Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / PersonalizableAttribute.cs / 1 / PersonalizableAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Security.Permissions; using System.Reflection; using System.Web; using System.Web.Util; ////// Used to mark a property as personalizable. /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AttributeUsage(AttributeTargets.Property)] public sealed class PersonalizableAttribute : Attribute { internal static readonly Type PersonalizableAttributeType = typeof(PersonalizableAttribute); private static readonly IDictionary PersonalizableTypeTable = Hashtable.Synchronized(new Hashtable()); ////// Indicates that the property is not personalizable. /// public static readonly PersonalizableAttribute NotPersonalizable = new PersonalizableAttribute(false); ////// Indicates that the property is personalizable. /// public static readonly PersonalizableAttribute Personalizable = new PersonalizableAttribute(true); ////// Indicates that the property is personalizable and can be changed per user. /// public static readonly PersonalizableAttribute UserPersonalizable = new PersonalizableAttribute(PersonalizationScope.User); ////// Indicates that the property is personalizable that can only be changed for all users. /// public static readonly PersonalizableAttribute SharedPersonalizable = new PersonalizableAttribute(PersonalizationScope.Shared); ////// The default personalizable attribute for a property or type. The default /// is to indicate that a property or type is not personalizable. /// public static readonly PersonalizableAttribute Default = NotPersonalizable; private bool _isPersonalizable; private bool _isSensitive; private PersonalizationScope _scope; ////// Initializes an instance of PersonalizableAttribute to indicate /// a personalizable property. /// By default personalized properties can be personalized per user. /// public PersonalizableAttribute() : this(true, PersonalizationScope.User, false) { } ////// Initializes an instance of PersonalizableAttribute with the /// specified value. /// public PersonalizableAttribute(bool isPersonalizable) : this(isPersonalizable, PersonalizationScope.User, false) { } ////// Initializes an instance of PersonalizableAttribute to indicate /// a personalizable property along with the specified personalization scope. /// public PersonalizableAttribute(PersonalizationScope scope) : this(true, scope, false) { } ////// Initializes an instance of PersonalizableAttribute to indicate /// a personalizable property along with the specified personalization scope and sensitivity. /// public PersonalizableAttribute(PersonalizationScope scope, bool isSensitive) : this(true, scope, isSensitive) { } ////// /// Initializes an instance of PersonalizableAttribute with the specified values. /// private PersonalizableAttribute(bool isPersonalizable, PersonalizationScope scope, bool isSensitive) { Debug.Assert((isPersonalizable == true || isSensitive == false), "Only Personalizable properties can be sensitive"); _isPersonalizable = isPersonalizable; _isSensitive = isSensitive; if (_isPersonalizable) { _scope = scope; } } ////// Whether the property or the type has been marked as personalizable. /// public bool IsPersonalizable { get { return _isPersonalizable; } } ////// Whether the property or the type has been marked as sensitive. /// public bool IsSensitive { get { return _isSensitive; } } ////// The personalization scope associated with the personalizable property. /// This property only has meaning when IsPersonalizable is true. /// public PersonalizationScope Scope { get { return _scope; } } ///public override bool Equals(object obj) { if (obj == this) { return true; } PersonalizableAttribute other = obj as PersonalizableAttribute; if (other != null) { return (other.IsPersonalizable == IsPersonalizable) && (other.Scope == Scope) && (other.IsSensitive == IsSensitive); } return false; } /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(_isPersonalizable.GetHashCode(), _scope.GetHashCode(), _isSensitive.GetHashCode()); } /// /// Returns the list of personalizable properties as a collection of /// PropertyInfos for the specified type. /// public static ICollection GetPersonalizableProperties(Type type) { Debug.Assert(type != null); PersonalizableTypeEntry typeEntry = (PersonalizableTypeEntry)PersonalizableTypeTable[type]; if (typeEntry == null) { typeEntry = new PersonalizableTypeEntry(type); PersonalizableTypeTable[type] = typeEntry; } return typeEntry.PropertyInfos; } ////// Returns the list of personalizable properties as a collection of /// PropertyInfos for the specified type. /// internal static IDictionary GetPersonalizablePropertyEntries(Type type) { Debug.Assert(type != null); PersonalizableTypeEntry typeEntry = (PersonalizableTypeEntry)PersonalizableTypeTable[type]; if (typeEntry == null) { typeEntry = new PersonalizableTypeEntry(type); PersonalizableTypeTable[type] = typeEntry; } return typeEntry.PropertyEntries; } ////// internal static IDictionary GetPersonalizablePropertyValues(Control control, PersonalizationScope scope, bool excludeSensitive) { IDictionary propertyBag = null; IDictionary propertyEntries = GetPersonalizablePropertyEntries(control.GetType()); if (propertyEntries.Count != 0) { foreach (DictionaryEntry entry in propertyEntries) { string name = (string)entry.Key; PersonalizablePropertyEntry propEntry = (PersonalizablePropertyEntry)entry.Value; if (excludeSensitive && propEntry.IsSensitive) { continue; } if ((scope == PersonalizationScope.User) && (propEntry.Scope == PersonalizationScope.Shared)) { continue; } if (propertyBag == null) { propertyBag = new HybridDictionary(propertyEntries.Count, /* caseInsensitive */ false); } object value = FastPropertyAccessor.GetProperty(control, name); propertyBag[name] = new Pair(propEntry.PropertyInfo, value); } } if (propertyBag == null) { propertyBag = new HybridDictionary(/* caseInsensitive */ false); } return propertyBag; } ///public override bool IsDefaultAttribute() { return this.Equals(Default); } /// public override bool Match(object obj) { if (obj == this) { return true; } PersonalizableAttribute other = obj as PersonalizableAttribute; if (other != null) { return (other.IsPersonalizable == IsPersonalizable); } return false; } } }
Link Menu
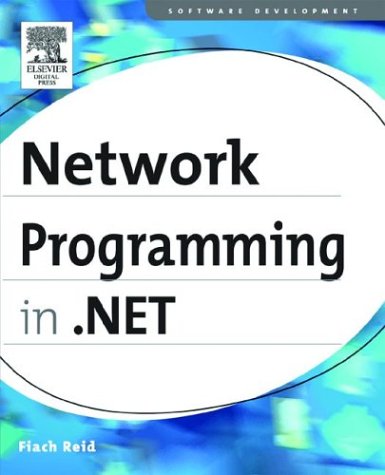
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataMemberFieldEditor.cs
- Image.cs
- HierarchicalDataBoundControl.cs
- HiddenField.cs
- GradientBrush.cs
- PerspectiveCamera.cs
- NativeMethods.cs
- EventLogConfiguration.cs
- TableMethodGenerator.cs
- ScaleTransform.cs
- TraceLog.cs
- LineUtil.cs
- DataSourceExpressionCollection.cs
- input.cs
- BinaryConverter.cs
- EventArgs.cs
- AbandonedMutexException.cs
- OptimalBreakSession.cs
- UrlAuthorizationModule.cs
- NativeMethods.cs
- LicenseContext.cs
- shaperfactory.cs
- XmlUtil.cs
- OwnerDrawPropertyBag.cs
- UnsafeNativeMethods.cs
- EventlogProvider.cs
- SqlConnection.cs
- HandleCollector.cs
- _ConnectStream.cs
- DynamicScriptObject.cs
- StrokeNodeOperations2.cs
- sqlnorm.cs
- EnumUnknown.cs
- HtmlShimManager.cs
- XPathDescendantIterator.cs
- ControlBuilder.cs
- ProxyAttribute.cs
- XmlElementAttribute.cs
- DesignerSelectionListAdapter.cs
- Error.cs
- ConnectorEditor.cs
- MaskInputRejectedEventArgs.cs
- XmlILConstructAnalyzer.cs
- XmlSchemaGroupRef.cs
- StickyNoteAnnotations.cs
- CodeTypeOfExpression.cs
- SettingsSection.cs
- RuntimeUtils.cs
- Menu.cs
- CompilerLocalReference.cs
- SymbolDocumentGenerator.cs
- MatrixKeyFrameCollection.cs
- VerticalAlignConverter.cs
- ListDictionary.cs
- InvalidComObjectException.cs
- WebConfigurationHost.cs
- FutureFactory.cs
- PropertyInfoSet.cs
- DiagnosticsConfiguration.cs
- IIS7WorkerRequest.cs
- BinaryObjectInfo.cs
- PointConverter.cs
- EventLogPermissionEntry.cs
- DBSqlParserTableCollection.cs
- DataGridCellsPresenter.cs
- SchemaMapping.cs
- EntityDataSourceSelectedEventArgs.cs
- BaseHashHelper.cs
- SingleTagSectionHandler.cs
- OleDbInfoMessageEvent.cs
- HtmlLink.cs
- SmtpSection.cs
- _Events.cs
- InteropAutomationProvider.cs
- RawStylusActions.cs
- PersonalizationDictionary.cs
- VisualBrush.cs
- InvalidCommandTreeException.cs
- LinqDataSourceContextData.cs
- CacheMemory.cs
- ProfileProvider.cs
- TextModifier.cs
- util.cs
- Container.cs
- EventLogEntryCollection.cs
- CompiledQueryCacheEntry.cs
- ConfigXmlSignificantWhitespace.cs
- PerformanceCountersElement.cs
- ProviderSettingsCollection.cs
- PolyLineSegment.cs
- InkCanvas.cs
- ADRoleFactoryConfiguration.cs
- WarningException.cs
- SqlConnectionStringBuilder.cs
- WebPartManagerInternals.cs
- XsltOutput.cs
- VersionUtil.cs
- RuntimeConfigLKG.cs
- AsyncDataRequest.cs
- DefaultSerializationProviderAttribute.cs