Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Annotations / LocatorPart.cs / 1 / LocatorPart.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorPart represents a set of name/value pairs that identify a // piece of data within a certain context. The names and values are // strings. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 05/06/2004: ssimova: Created // 06/30/2004: rruiz: Added change notifications to parent, clean-up //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Xml; using MS.Internal.Annotations; using MS.Internal.Annotations.Anchoring; namespace System.Windows.Annotations { ////// ContentLocatorPart represents a set of name/value pairs that identify a /// piece of data within a certain context. The names and values are /// all strings. /// public sealed class ContentLocatorPart : INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a ContentLocatorPart with the specified type name and namespace. /// /// fully qualified locator part's type ///partType is null ///partType.Namespace or partType.Name is null or empty string public ContentLocatorPart(XmlQualifiedName partType) { if (partType == null) { throw new ArgumentNullException("partType"); } if (String.IsNullOrEmpty(partType.Name)) { throw new ArgumentException(SR.Get(SRID.TypeNameMustBeSpecified), "partType.Name"); } if (String.IsNullOrEmpty(partType.Namespace)) { throw new ArgumentException(SR.Get(SRID.TypeNameMustBeSpecified), "partType.Namespace"); } _type = partType; _nameValues = new ObservableDictionary(); _nameValues.PropertyChanged += OnPropertyChanged; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two ContentLocatorParts for equality. They are equal if they /// contain the same set of name/value pairs. /// /// second locator part ///true - the ContentLocatorParts are equal, false - different public override bool Equals(object obj) { ContentLocatorPart part = obj as ContentLocatorPart; string otherValue; // We are equal to ourselves if (part == this) { return true; } // Not a locator part if (part == null) { return false; } // Have different type names if (!_type.Equals(part.PartType)) { return false; } // Have different number of name/value pairs if (part.NameValuePairs.Count != _nameValues.Count) { return false; } foreach (KeyValuePairk_v in _nameValues) { // A name/value pair isn't present or has a different value if (!part._nameValues.TryGetValue(k_v.Key, out otherValue)) { return false; } if (k_v.Value != otherValue) { return false; } } return true; } /// /// Returns the hashcode for this ContentLocatorPart. /// ///hashcode public override int GetHashCode() { return base.GetHashCode(); } ////// Create a deep clone of this ContentLocatorPart. The returned ContentLocatorPart /// is equal to this ContentLocatorPart. /// ///a deep clone of this ContentLocatorPart; never returns null public object Clone() { ContentLocatorPart newPart = new ContentLocatorPart(_type); foreach (KeyValuePairk_v in _nameValues) { newPart.NameValuePairs.Add(k_v.Key, k_v.Value); } return newPart; } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties /// /// /// public IDictionaryNameValuePairs { get { return _nameValues; } } /// /// Returns the ContentLocatorPart's type name. /// ///qualified type name for this ContentLocatorPart public XmlQualifiedName PartType { get { return _type; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Determines if a locator part matches this locator part. Matches is /// different from equals because a locator part may be defined to match /// a range of locator parts, not just exact replicas. /// internal bool Matches(ContentLocatorPart part) { bool overlaps = false; string overlapsString; _nameValues.TryGetValue(TextSelectionProcessor.IncludeOverlaps, out overlapsString); // If IncludeOverlaps is true, a match is any locator part // whose range overlaps with ours if (Boolean.TryParse(overlapsString, out overlaps) && overlaps) { // We match ourselves if (part == this) { return true; } // Have different type names if (!_type.Equals(part.PartType)) { return false; } int desiredStartOffset; int desiredEndOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(this, out desiredStartOffset, out desiredEndOffset); int startOffset; int endOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(part, out startOffset, out endOffset); // Take care of an exact match to us (which may include offset==MinValue // which we don't want to handle with the formula below. if (desiredStartOffset == startOffset && desiredEndOffset == endOffset) { return true; } // Take care of the special case of no content to match to if (desiredStartOffset == int.MinValue) { return false; } if ((startOffset >= desiredStartOffset && startOffset <= desiredEndOffset) || (startOffset < desiredStartOffset && endOffset >= desiredStartOffset)) { return true; } return false; } return this.Equals(part); } ////// Produces an XPath fragment that selects for matches to this ContentLocatorPart. /// /// namespace manager used to look up prefixes ///an XPath fragment that selects for matches to this ContentLocatorPart internal string GetQueryFragment(XmlNamespaceManager namespaceManager) { bool overlaps = false; string overlapsString; _nameValues.TryGetValue(TextSelectionProcessor.IncludeOverlaps, out overlapsString); if (Boolean.TryParse(overlapsString, out overlaps) && overlaps) { return GetOverlapQueryFragment(namespaceManager); } else { return GetExactQueryFragment(namespaceManager); } } #endregion Internal Methods //------------------------------------------------------ // // Internal Operators // //----------------------------------------------------- //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Notify the owner this ContentLocatorPart has changed. /// private void OnPropertyChanged(Object sender, System.ComponentModel.PropertyChangedEventArgs e) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs("NameValuePairs")); } } ////// Produces an XPath fragment that selects for ContentLocatorParts with an anchor that /// intersects with the range specified by this ContentLocatorPart. /// /// namespace manager used to look up prefixes private string GetOverlapQueryFragment(XmlNamespaceManager namespaceManager) { string corePrefix = namespaceManager.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); string prefix = namespaceManager.LookupPrefix(this.PartType.Namespace); string res = prefix == null ? "" : (prefix + ":"); res += TextSelectionProcessor.CharacterRangeElementName.Name + "/" + corePrefix + ":"+AnnotationXmlConstants.Elements.Item; int startOffset; int endOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(this, out startOffset, out endOffset); string startStr = startOffset.ToString(NumberFormatInfo.InvariantInfo); string endStr = endOffset.ToString(NumberFormatInfo.InvariantInfo); // Note: this will never match if offsetStr == 0. Which makes sense - there // is no content to get anchors for. res += "[starts-with(@" + AnnotationXmlConstants.Attributes.ItemName + ", \"" + TextSelectionProcessor.SegmentAttribute + "\") and " + " ((substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") >= " + startStr + " and substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") <= " + endStr + ") or " + " (substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") < " + startStr + " and substring-after(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") >= " + startStr + "))]"; return res; } ////// Produces an XPath fragment that selects ContentLocatorParts of the same type /// and containing the exact name/values this ContentLocatorPart contains. /// /// namespaceManager used to generate the XPath fragment private string GetExactQueryFragment(XmlNamespaceManager namespaceManager) { string corePrefix = namespaceManager.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); string prefix = namespaceManager.LookupPrefix(this.PartType.Namespace); string res = prefix == null ? "" : (prefix + ":"); res += this.PartType.Name; bool and = false; foreach (KeyValuePairk_v in ((ICollection >)this.NameValuePairs)) { if (and) { res += "/parent::*/" + corePrefix + ":" + AnnotationXmlConstants.Elements.Item + "["; } else { and = true; res += "/" + corePrefix + ":" + AnnotationXmlConstants.Elements.Item + "["; } res += "@" + AnnotationXmlConstants.Attributes.ItemName + "=\"" + k_v.Key + "\" and @" + AnnotationXmlConstants.Attributes.ItemValue + "=\"" + k_v.Value + "\"]"; } if (and) { res += "/parent::*"; } return res; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields /// /// private bool _owned; ////// The ContentLocatorPart's type name. /// private XmlQualifiedName _type; ////// The internal data structure. /// private ObservableDictionary _nameValues; /// private event PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorPart represents a set of name/value pairs that identify a // piece of data within a certain context. The names and values are // strings. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 05/06/2004: ssimova: Created // 06/30/2004: rruiz: Added change notifications to parent, clean-up //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Xml; using MS.Internal.Annotations; using MS.Internal.Annotations.Anchoring; namespace System.Windows.Annotations { ////// ContentLocatorPart represents a set of name/value pairs that identify a /// piece of data within a certain context. The names and values are /// all strings. /// public sealed class ContentLocatorPart : INotifyPropertyChanged2, IOwnedObject { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a ContentLocatorPart with the specified type name and namespace. /// /// fully qualified locator part's type ///partType is null ///partType.Namespace or partType.Name is null or empty string public ContentLocatorPart(XmlQualifiedName partType) { if (partType == null) { throw new ArgumentNullException("partType"); } if (String.IsNullOrEmpty(partType.Name)) { throw new ArgumentException(SR.Get(SRID.TypeNameMustBeSpecified), "partType.Name"); } if (String.IsNullOrEmpty(partType.Namespace)) { throw new ArgumentException(SR.Get(SRID.TypeNameMustBeSpecified), "partType.Namespace"); } _type = partType; _nameValues = new ObservableDictionary(); _nameValues.PropertyChanged += OnPropertyChanged; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two ContentLocatorParts for equality. They are equal if they /// contain the same set of name/value pairs. /// /// second locator part ///true - the ContentLocatorParts are equal, false - different public override bool Equals(object obj) { ContentLocatorPart part = obj as ContentLocatorPart; string otherValue; // We are equal to ourselves if (part == this) { return true; } // Not a locator part if (part == null) { return false; } // Have different type names if (!_type.Equals(part.PartType)) { return false; } // Have different number of name/value pairs if (part.NameValuePairs.Count != _nameValues.Count) { return false; } foreach (KeyValuePairk_v in _nameValues) { // A name/value pair isn't present or has a different value if (!part._nameValues.TryGetValue(k_v.Key, out otherValue)) { return false; } if (k_v.Value != otherValue) { return false; } } return true; } /// /// Returns the hashcode for this ContentLocatorPart. /// ///hashcode public override int GetHashCode() { return base.GetHashCode(); } ////// Create a deep clone of this ContentLocatorPart. The returned ContentLocatorPart /// is equal to this ContentLocatorPart. /// ///a deep clone of this ContentLocatorPart; never returns null public object Clone() { ContentLocatorPart newPart = new ContentLocatorPart(_type); foreach (KeyValuePairk_v in _nameValues) { newPart.NameValuePairs.Add(k_v.Key, k_v.Value); } return newPart; } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties /// /// /// public IDictionaryNameValuePairs { get { return _nameValues; } } /// /// Returns the ContentLocatorPart's type name. /// ///qualified type name for this ContentLocatorPart public XmlQualifiedName PartType { get { return _type; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ #region Public Events ////// /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add{ _propertyChanged += value; } remove{ _propertyChanged -= value; } } #endregion Public Events //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Determines if a locator part matches this locator part. Matches is /// different from equals because a locator part may be defined to match /// a range of locator parts, not just exact replicas. /// internal bool Matches(ContentLocatorPart part) { bool overlaps = false; string overlapsString; _nameValues.TryGetValue(TextSelectionProcessor.IncludeOverlaps, out overlapsString); // If IncludeOverlaps is true, a match is any locator part // whose range overlaps with ours if (Boolean.TryParse(overlapsString, out overlaps) && overlaps) { // We match ourselves if (part == this) { return true; } // Have different type names if (!_type.Equals(part.PartType)) { return false; } int desiredStartOffset; int desiredEndOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(this, out desiredStartOffset, out desiredEndOffset); int startOffset; int endOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(part, out startOffset, out endOffset); // Take care of an exact match to us (which may include offset==MinValue // which we don't want to handle with the formula below. if (desiredStartOffset == startOffset && desiredEndOffset == endOffset) { return true; } // Take care of the special case of no content to match to if (desiredStartOffset == int.MinValue) { return false; } if ((startOffset >= desiredStartOffset && startOffset <= desiredEndOffset) || (startOffset < desiredStartOffset && endOffset >= desiredStartOffset)) { return true; } return false; } return this.Equals(part); } ////// Produces an XPath fragment that selects for matches to this ContentLocatorPart. /// /// namespace manager used to look up prefixes ///an XPath fragment that selects for matches to this ContentLocatorPart internal string GetQueryFragment(XmlNamespaceManager namespaceManager) { bool overlaps = false; string overlapsString; _nameValues.TryGetValue(TextSelectionProcessor.IncludeOverlaps, out overlapsString); if (Boolean.TryParse(overlapsString, out overlaps) && overlaps) { return GetOverlapQueryFragment(namespaceManager); } else { return GetExactQueryFragment(namespaceManager); } } #endregion Internal Methods //------------------------------------------------------ // // Internal Operators // //----------------------------------------------------- //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// bool IOwnedObject.Owned { get { return _owned; } set { _owned = value; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Notify the owner this ContentLocatorPart has changed. /// private void OnPropertyChanged(Object sender, System.ComponentModel.PropertyChangedEventArgs e) { if (_propertyChanged != null) { _propertyChanged(this, new System.ComponentModel.PropertyChangedEventArgs("NameValuePairs")); } } ////// Produces an XPath fragment that selects for ContentLocatorParts with an anchor that /// intersects with the range specified by this ContentLocatorPart. /// /// namespace manager used to look up prefixes private string GetOverlapQueryFragment(XmlNamespaceManager namespaceManager) { string corePrefix = namespaceManager.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); string prefix = namespaceManager.LookupPrefix(this.PartType.Namespace); string res = prefix == null ? "" : (prefix + ":"); res += TextSelectionProcessor.CharacterRangeElementName.Name + "/" + corePrefix + ":"+AnnotationXmlConstants.Elements.Item; int startOffset; int endOffset; TextSelectionProcessor.GetMaxMinLocatorPartValues(this, out startOffset, out endOffset); string startStr = startOffset.ToString(NumberFormatInfo.InvariantInfo); string endStr = endOffset.ToString(NumberFormatInfo.InvariantInfo); // Note: this will never match if offsetStr == 0. Which makes sense - there // is no content to get anchors for. res += "[starts-with(@" + AnnotationXmlConstants.Attributes.ItemName + ", \"" + TextSelectionProcessor.SegmentAttribute + "\") and " + " ((substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") >= " + startStr + " and substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") <= " + endStr + ") or " + " (substring-before(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") < " + startStr + " and substring-after(@" + AnnotationXmlConstants.Attributes.ItemValue + ",\",\") >= " + startStr + "))]"; return res; } ////// Produces an XPath fragment that selects ContentLocatorParts of the same type /// and containing the exact name/values this ContentLocatorPart contains. /// /// namespaceManager used to generate the XPath fragment private string GetExactQueryFragment(XmlNamespaceManager namespaceManager) { string corePrefix = namespaceManager.LookupPrefix(AnnotationXmlConstants.Namespaces.CoreSchemaNamespace); string prefix = namespaceManager.LookupPrefix(this.PartType.Namespace); string res = prefix == null ? "" : (prefix + ":"); res += this.PartType.Name; bool and = false; foreach (KeyValuePairk_v in ((ICollection >)this.NameValuePairs)) { if (and) { res += "/parent::*/" + corePrefix + ":" + AnnotationXmlConstants.Elements.Item + "["; } else { and = true; res += "/" + corePrefix + ":" + AnnotationXmlConstants.Elements.Item + "["; } res += "@" + AnnotationXmlConstants.Attributes.ItemName + "=\"" + k_v.Key + "\" and @" + AnnotationXmlConstants.Attributes.ItemValue + "=\"" + k_v.Value + "\"]"; } if (and) { res += "/parent::*"; } return res; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields /// /// private bool _owned; ////// The ContentLocatorPart's type name. /// private XmlQualifiedName _type; ////// The internal data structure. /// private ObservableDictionary _nameValues; /// private event PropertyChangedEventHandler _propertyChanged; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
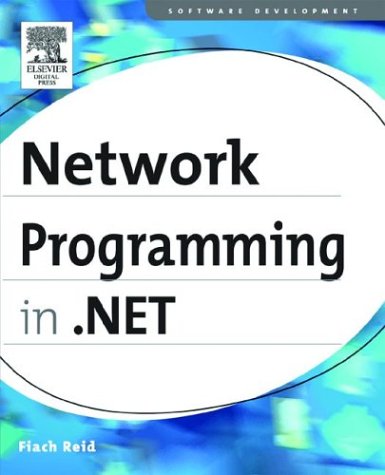
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqIntegrationOutputChannel.cs
- TypeConverterBase.cs
- FocusWithinProperty.cs
- WindowsToolbar.cs
- HotSpotCollectionEditor.cs
- VisualTreeHelper.cs
- SafeLibraryHandle.cs
- CultureTable.cs
- SqlMethodAttribute.cs
- Rect.cs
- ColumnHeaderConverter.cs
- PropertyPath.cs
- MetadataException.cs
- QilValidationVisitor.cs
- CmsInterop.cs
- WindowsProgressbar.cs
- SafeNativeMethods.cs
- RayMeshGeometry3DHitTestResult.cs
- WebControl.cs
- SQLUtility.cs
- SoapSchemaMember.cs
- SchemaImporterExtensionsSection.cs
- InfiniteIntConverter.cs
- MetafileHeaderWmf.cs
- TextUtf8RawTextWriter.cs
- CancellableEnumerable.cs
- KeyManager.cs
- ServiceHttpHandlerFactory.cs
- PointAnimationUsingKeyFrames.cs
- DisplayMemberTemplateSelector.cs
- ConfigurationLoaderException.cs
- PngBitmapEncoder.cs
- WebPartDisplayModeCancelEventArgs.cs
- VariableReference.cs
- ObjectDisposedException.cs
- BitmapMetadata.cs
- BevelBitmapEffect.cs
- XmlILStorageConverter.cs
- PolyQuadraticBezierSegment.cs
- UnauthorizedAccessException.cs
- ContentValidator.cs
- KeyPressEvent.cs
- AttachedAnnotation.cs
- URI.cs
- ClientSession.cs
- ResXResourceWriter.cs
- ControllableStoryboardAction.cs
- EnumerableCollectionView.cs
- RightsManagementPermission.cs
- FactoryMaker.cs
- ControlCachePolicy.cs
- StreamWithDictionary.cs
- PenThread.cs
- RowUpdatingEventArgs.cs
- ConsumerConnectionPointCollection.cs
- SqlInternalConnectionTds.cs
- EntityDataReader.cs
- PhonemeEventArgs.cs
- UInt16.cs
- TagPrefixAttribute.cs
- SymmetricKeyWrap.cs
- ComEventsMethod.cs
- FixedSOMImage.cs
- LinearQuaternionKeyFrame.cs
- InProcStateClientManager.cs
- XmlSchemaNotation.cs
- OciHandle.cs
- SafeUserTokenHandle.cs
- ServiceHttpHandlerFactory.cs
- LinqDataSourceUpdateEventArgs.cs
- safex509handles.cs
- XsdDateTime.cs
- BeginCreateSecurityTokenRequest.cs
- SafeLibraryHandle.cs
- WmpBitmapEncoder.cs
- NetworkInterface.cs
- TimeSpanValidator.cs
- Size3DConverter.cs
- StructuredProperty.cs
- _UncName.cs
- odbcmetadatacolumnnames.cs
- SafeMILHandle.cs
- SoapHttpTransportImporter.cs
- GenericWebPart.cs
- QilFactory.cs
- PageAction.cs
- DataTemplateSelector.cs
- RemotingConfigParser.cs
- CompositeDataBoundControl.cs
- autovalidator.cs
- DiagnosticTraceSource.cs
- NetPipeSectionData.cs
- MenuScrollingVisibilityConverter.cs
- WorkflowInstanceProvider.cs
- FactoryGenerator.cs
- ObjectDataProvider.cs
- sitestring.cs
- EventListenerClientSide.cs
- RenderingEventArgs.cs
- Util.cs