Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlILStorageConverter.cs / 1 / XmlILStorageConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// This is a simple convenience wrapper internal class that contains static helper methods that get a value /// converter from XmlQueryRuntime and use it convert among several physical Clr representations for /// the same logical Xml type. For example, an external function might have an argument typed as /// xs:integer, with Clr type Decimal. Since ILGen stores xs:integer as Clr type Int64 instead of /// Decimal, a conversion to the desired storage type must take place. /// [EditorBrowsable(EditorBrowsableState.Never)] public static class XmlILStorageConverter { //----------------------------------------------- // ToAtomicValue //----------------------------------------------- public static XmlAtomicValue StringToAtomicValue(string value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DecimalToAtomicValue(decimal value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int64ToAtomicValue(long value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int32ToAtomicValue(int value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BooleanToAtomicValue(bool value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DoubleToAtomicValue(double value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue SingleToAtomicValue(float value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DateTimeToAtomicValue(DateTime value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue XmlQualifiedNameToAtomicValue(XmlQualifiedName value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue TimeSpanToAtomicValue(TimeSpan value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BytesToAtomicValue(byte[] value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static IListNavigatorsToItems(IList listNavigators) { // Check to see if the navigator cache implements IList IList listItems = listNavigators as IList ; if (listItems != null) return listItems; // Create XmlQueryNodeSequence, which does implement IList return new XmlQueryNodeSequence(listNavigators); } public static IList ItemsToNavigators(IList listItems) { // Check to see if the navigator cache implements IList IList listNavs = listItems as IList ; if (listNavs != null) return listNavs; // Create XmlQueryNodeSequence, which does implement IList XmlQueryNodeSequence seq = new XmlQueryNodeSequence(listItems.Count); for (int i = 0; i < listItems.Count; i++) seq.Add((XPathNavigator) listItems[i]); return seq; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Xml; using System.Xml.XPath; using System.Xml.Schema; using System.Xml.Xsl; using System.ComponentModel; namespace System.Xml.Xsl.Runtime { ////// This is a simple convenience wrapper internal class that contains static helper methods that get a value /// converter from XmlQueryRuntime and use it convert among several physical Clr representations for /// the same logical Xml type. For example, an external function might have an argument typed as /// xs:integer, with Clr type Decimal. Since ILGen stores xs:integer as Clr type Int64 instead of /// Decimal, a conversion to the desired storage type must take place. /// [EditorBrowsable(EditorBrowsableState.Never)] public static class XmlILStorageConverter { //----------------------------------------------- // ToAtomicValue //----------------------------------------------- public static XmlAtomicValue StringToAtomicValue(string value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DecimalToAtomicValue(decimal value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int64ToAtomicValue(long value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue Int32ToAtomicValue(int value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BooleanToAtomicValue(bool value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DoubleToAtomicValue(double value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue SingleToAtomicValue(float value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue DateTimeToAtomicValue(DateTime value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue XmlQualifiedNameToAtomicValue(XmlQualifiedName value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue TimeSpanToAtomicValue(TimeSpan value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static XmlAtomicValue BytesToAtomicValue(byte[] value, int index, XmlQueryRuntime runtime) { return new XmlAtomicValue(runtime.GetXmlType(index).SchemaType, value); } public static IListNavigatorsToItems(IList listNavigators) { // Check to see if the navigator cache implements IList IList listItems = listNavigators as IList ; if (listItems != null) return listItems; // Create XmlQueryNodeSequence, which does implement IList return new XmlQueryNodeSequence(listNavigators); } public static IList ItemsToNavigators(IList listItems) { // Check to see if the navigator cache implements IList IList listNavs = listItems as IList ; if (listNavs != null) return listNavs; // Create XmlQueryNodeSequence, which does implement IList XmlQueryNodeSequence seq = new XmlQueryNodeSequence(listItems.Count); for (int i = 0; i < listItems.Count; i++) seq.Add((XPathNavigator) listItems[i]); return seq; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
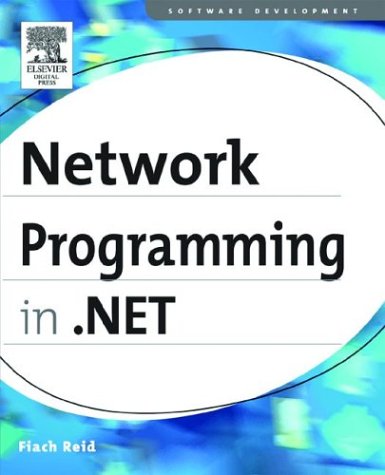
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailSettingsSection.cs
- EntityDataSourceSelectingEventArgs.cs
- WaitForChangedResult.cs
- Int32CollectionConverter.cs
- Exceptions.cs
- ScriptingSectionGroup.cs
- ZipIOLocalFileDataDescriptor.cs
- StrongBox.cs
- serverconfig.cs
- DES.cs
- WindowsScrollBarBits.cs
- XmlDocumentViewSchema.cs
- DispatcherProcessingDisabled.cs
- ReadOnlyMetadataCollection.cs
- HtmlMeta.cs
- HttpHeaderCollection.cs
- CanExecuteRoutedEventArgs.cs
- ErrorWebPart.cs
- CommentEmitter.cs
- SQLInt32Storage.cs
- DeploymentSection.cs
- ExpressionBuilder.cs
- GuidelineSet.cs
- ToolStripSystemRenderer.cs
- MaskDescriptors.cs
- IxmlLineInfo.cs
- BitmapCodecInfoInternal.cs
- LocalizationParserHooks.cs
- XmlMemberMapping.cs
- Publisher.cs
- WebBrowser.cs
- Material.cs
- FlowLayoutSettings.cs
- _IPv4Address.cs
- SmiMetaData.cs
- ModelUtilities.cs
- ScriptModule.cs
- SelectManyQueryOperator.cs
- MeasureData.cs
- TrackingWorkflowEventArgs.cs
- BoundingRectTracker.cs
- HashAlgorithm.cs
- CodeTypeReferenceCollection.cs
- PackageRelationshipSelector.cs
- ResolveMatchesMessageCD1.cs
- DataView.cs
- RootBrowserWindow.cs
- StateDesignerConnector.cs
- ModelService.cs
- ResourceWriter.cs
- Version.cs
- DataSetMappper.cs
- CollectionChangeEventArgs.cs
- DocumentGrid.cs
- Polygon.cs
- XmlValidatingReader.cs
- EndpointConfigContainer.cs
- BaseTemplateParser.cs
- GenericTypeParameterBuilder.cs
- MultipartIdentifier.cs
- TimeIntervalCollection.cs
- XmlHierarchyData.cs
- OrderedEnumerableRowCollection.cs
- WebPartCollection.cs
- PublisherMembershipCondition.cs
- TimeSpanOrInfiniteConverter.cs
- PrincipalPermission.cs
- WeakReferenceList.cs
- ProxyGenerationError.cs
- SimpleTextLine.cs
- ProcessHostFactoryHelper.cs
- SizeF.cs
- SafeCancelMibChangeNotify.cs
- EventDrivenDesigner.cs
- DataFormat.cs
- VarRefManager.cs
- CommandHelpers.cs
- XmlNamespaceManager.cs
- TypeReference.cs
- LinqDataSourceStatusEventArgs.cs
- OdbcConnectionString.cs
- Instrumentation.cs
- ToolStripItemEventArgs.cs
- PersistenceMetadataNamespace.cs
- SmtpMail.cs
- CodeTypeDelegate.cs
- XmlIterators.cs
- AppSecurityManager.cs
- XmlToDatasetMap.cs
- HandleCollector.cs
- Control.cs
- ListViewDeletedEventArgs.cs
- AdapterDictionary.cs
- IISUnsafeMethods.cs
- DefaultBindingPropertyAttribute.cs
- OAVariantLib.cs
- RootBrowserWindowProxy.cs
- DLinqDataModelProvider.cs
- FileClassifier.cs
- XpsS0ValidatingLoader.cs