Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / AttachedPropertyBrowsableForChildrenAttribute.cs / 1305600 / AttachedPropertyBrowsableForChildrenAttribute.cs
namespace System.Windows { using System; ////// This attribute declares that a property is visible when the /// property owner is a parent of another element. For example, /// Canvas.Left is only useful on elements parented within the /// canvas. The class supports two types of tree walks: a shallow /// walk, the default which requires that the immediate parent be the /// owner type of the property, and a deep walk, declared by setting /// IncludeDescendants to true and requires that the owner type be /// somewhere in the parenting hierarchy. /// [AttributeUsage(AttributeTargets.Method, AllowMultiple = false)] public sealed class AttachedPropertyBrowsableForChildrenAttribute : AttachedPropertyBrowsableAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Creates a new AttachedPropertyBrowsableForChildrenAttribute. /// public AttachedPropertyBrowsableForChildrenAttribute() { } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Gets or sets if the property should be browsable for just the /// immediate children (false) or all children (true). /// public bool IncludeDescendants { get { return _includeDescendants; } set { _includeDescendants = value; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Overrides Object.Equals to implement correct equality semantics for this /// attribute. /// public override bool Equals(object obj) { AttachedPropertyBrowsableForChildrenAttribute other = obj as AttachedPropertyBrowsableForChildrenAttribute; if (other == null) return false; return _includeDescendants == other._includeDescendants; } ////// Overrides Object.GetHashCode to implement correct hashing semantics. /// public override int GetHashCode() { return _includeDescendants.GetHashCode(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Returns true if the object provided is the immediate logical /// child (if IncludeDescendants is false) or any logical child /// (if IncludeDescendants is true). /// internal override bool IsBrowsable(DependencyObject d, DependencyProperty dp) { if (d == null) throw new ArgumentNullException("d"); if (dp == null) throw new ArgumentNullException("dp"); DependencyObject walk = d; Type ownerType = dp.OwnerType; do { walk = FrameworkElement.GetFrameworkParent(walk); if (walk != null && ownerType.IsInstanceOfType(walk)) { return true; } } while (_includeDescendants && walk != null); return false; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private bool _includeDescendants; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
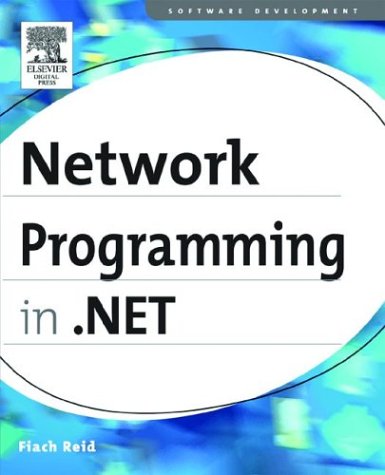
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintDialogException.cs
- PTUtility.cs
- StatusBarPanel.cs
- TrustSection.cs
- BufferBuilder.cs
- InertiaRotationBehavior.cs
- pingexception.cs
- MbpInfo.cs
- ToolStripDropDown.cs
- RemoteWebConfigurationHostServer.cs
- MetadataItemSerializer.cs
- DataGridViewColumnCollection.cs
- ObfuscationAttribute.cs
- FileLoadException.cs
- DateTimeValueSerializerContext.cs
- MissingMethodException.cs
- EntityContainer.cs
- DesignerContextDescriptor.cs
- TextEditorSpelling.cs
- ImportedPolicyConversionContext.cs
- OperatingSystem.cs
- EdmEntityTypeAttribute.cs
- FontWeightConverter.cs
- TranslateTransform.cs
- OracleConnectionFactory.cs
- BitmapEffect.cs
- DmlSqlGenerator.cs
- ApplicationBuildProvider.cs
- QueryStringHandler.cs
- SharedConnectionInfo.cs
- DiagnosticTrace.cs
- XmlAnyElementAttributes.cs
- SynchronizedInputPattern.cs
- DispatchWrapper.cs
- ArithmeticException.cs
- SchemaConstraints.cs
- SessionEndingCancelEventArgs.cs
- CharUnicodeInfo.cs
- DrawingContextWalker.cs
- XmlSchemaSimpleContentExtension.cs
- RouteParser.cs
- SortedDictionary.cs
- WebSysDisplayNameAttribute.cs
- FileAuthorizationModule.cs
- BuilderPropertyEntry.cs
- SocketAddress.cs
- NetworkInformationException.cs
- HttpWebResponse.cs
- CompositeDataBoundControl.cs
- PublisherIdentityPermission.cs
- CompilerResults.cs
- Keywords.cs
- ChannelManager.cs
- ReliableSessionElement.cs
- Substitution.cs
- DispatcherHooks.cs
- DataConnectionHelper.cs
- Vector.cs
- ProfileManager.cs
- SystemInformation.cs
- FullTextBreakpoint.cs
- TrustLevelCollection.cs
- DataGridBoolColumn.cs
- InternalConfigConfigurationFactory.cs
- DataGridView.cs
- IsolatedStoragePermission.cs
- ActivityExecutionContext.cs
- ColorConvertedBitmap.cs
- ExclusiveCanonicalizationTransform.cs
- UnsafeNativeMethods.cs
- ConstructorNeedsTagAttribute.cs
- XamlUtilities.cs
- Encoder.cs
- Dump.cs
- TableSectionStyle.cs
- Model3DGroup.cs
- SuspendDesigner.cs
- XPathDocument.cs
- DataGridTablesFactory.cs
- ReadOnlyDataSourceView.cs
- Viewport2DVisual3D.cs
- ReferenceSchema.cs
- CssClassPropertyAttribute.cs
- Rule.cs
- PointCollectionValueSerializer.cs
- SQLChars.cs
- SQLDouble.cs
- AppSecurityManager.cs
- DesignerSerializationManager.cs
- PermissionRequestEvidence.cs
- ImageAnimator.cs
- DiagnosticStrings.cs
- MessageBox.cs
- BasePropertyDescriptor.cs
- DateTimeParse.cs
- ClaimSet.cs
- XmlSchemaImporter.cs
- ClientEventManager.cs
- ErrorFormatterPage.cs
- MessageQueueAccessControlEntry.cs