Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / Collections / Specialized / StringDictionary.cs / 1 / StringDictionary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; ////// [Serializable] [DesignerSerializer("System.Diagnostics.Design.StringDictionaryCodeDomSerializer, " + AssemblyRef.SystemDesign, "System.ComponentModel.Design.Serialization.CodeDomSerializer, " + AssemblyRef.SystemDesign)] public class StringDictionary : IEnumerable { internal Hashtable contents = new Hashtable(); ///Implements a hashtable with the key strongly typed to be /// a string rather than an object. ////// public StringDictionary() { } ///Initializes a new instance of the System.Windows.Forms.StringDictionary class. ////// public virtual int Count { get { return contents.Count; } } ///Gets the number of key-and-value pairs in the System.Windows.Forms.StringDictionary. ////// public virtual bool IsSynchronized { get { return contents.IsSynchronized; } } ///Indicates whether access to the System.Windows.Forms.StringDictionary is synchronized (thread-safe). This property is /// read-only. ////// public virtual string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key.ToLower(CultureInfo.InvariantCulture)]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key.ToLower(CultureInfo.InvariantCulture)] = value; } } ///Gets or sets the value associated with the specified key. ////// public virtual ICollection Keys { get { return contents.Keys; } } ///Gets a collection of keys in the System.Windows.Forms.StringDictionary. ////// public virtual object SyncRoot { get { return contents.SyncRoot; } } ///Gets an object that can be used to synchronize access to the System.Windows.Forms.StringDictionary. ////// public virtual ICollection Values { get { return contents.Values; } } ///Gets a collection of values in the System.Windows.Forms.StringDictionary. ////// public virtual void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key.ToLower(CultureInfo.InvariantCulture), value); } ///Adds an entry with the specified key and value into the System.Windows.Forms.StringDictionary. ////// public virtual void Clear() { contents.Clear(); } ///Removes all entries from the System.Windows.Forms.StringDictionary. ////// public virtual bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key.ToLower(CultureInfo.InvariantCulture)); } ///Determines if the string dictionary contains a specific key ////// public virtual bool ContainsValue(string value) { return contents.ContainsValue(value); } ///Determines if the System.Windows.Forms.StringDictionary contains a specific value. ////// public virtual void CopyTo(Array array, int index) { contents.CopyTo(array, index); } ///Copies the string dictionary values to a one-dimensional ///instance at the /// specified index. /// public virtual IEnumerator GetEnumerator() { return contents.GetEnumerator(); } ///Returns an enumerator that can iterate through the string dictionary. ////// public virtual void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key.ToLower(CultureInfo.InvariantCulture)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Removes the entry with the specified key from the string dictionary. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; ////// [Serializable] [DesignerSerializer("System.Diagnostics.Design.StringDictionaryCodeDomSerializer, " + AssemblyRef.SystemDesign, "System.ComponentModel.Design.Serialization.CodeDomSerializer, " + AssemblyRef.SystemDesign)] public class StringDictionary : IEnumerable { internal Hashtable contents = new Hashtable(); ///Implements a hashtable with the key strongly typed to be /// a string rather than an object. ////// public StringDictionary() { } ///Initializes a new instance of the System.Windows.Forms.StringDictionary class. ////// public virtual int Count { get { return contents.Count; } } ///Gets the number of key-and-value pairs in the System.Windows.Forms.StringDictionary. ////// public virtual bool IsSynchronized { get { return contents.IsSynchronized; } } ///Indicates whether access to the System.Windows.Forms.StringDictionary is synchronized (thread-safe). This property is /// read-only. ////// public virtual string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key.ToLower(CultureInfo.InvariantCulture)]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key.ToLower(CultureInfo.InvariantCulture)] = value; } } ///Gets or sets the value associated with the specified key. ////// public virtual ICollection Keys { get { return contents.Keys; } } ///Gets a collection of keys in the System.Windows.Forms.StringDictionary. ////// public virtual object SyncRoot { get { return contents.SyncRoot; } } ///Gets an object that can be used to synchronize access to the System.Windows.Forms.StringDictionary. ////// public virtual ICollection Values { get { return contents.Values; } } ///Gets a collection of values in the System.Windows.Forms.StringDictionary. ////// public virtual void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key.ToLower(CultureInfo.InvariantCulture), value); } ///Adds an entry with the specified key and value into the System.Windows.Forms.StringDictionary. ////// public virtual void Clear() { contents.Clear(); } ///Removes all entries from the System.Windows.Forms.StringDictionary. ////// public virtual bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key.ToLower(CultureInfo.InvariantCulture)); } ///Determines if the string dictionary contains a specific key ////// public virtual bool ContainsValue(string value) { return contents.ContainsValue(value); } ///Determines if the System.Windows.Forms.StringDictionary contains a specific value. ////// public virtual void CopyTo(Array array, int index) { contents.CopyTo(array, index); } ///Copies the string dictionary values to a one-dimensional ///instance at the /// specified index. /// public virtual IEnumerator GetEnumerator() { return contents.GetEnumerator(); } ///Returns an enumerator that can iterate through the string dictionary. ////// public virtual void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key.ToLower(CultureInfo.InvariantCulture)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Removes the entry with the specified key from the string dictionary. ///
Link Menu
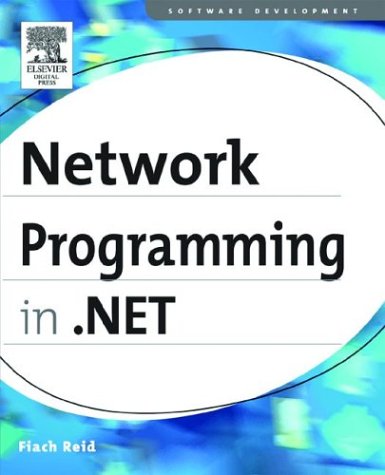
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GetIsBrowserClientRequest.cs
- TakeQueryOptionExpression.cs
- HtmlInputImage.cs
- DynamicEndpoint.cs
- DataContractSerializerElement.cs
- LogicalExpr.cs
- ProcessHostFactoryHelper.cs
- ProgressChangedEventArgs.cs
- LogFlushAsyncResult.cs
- TheQuery.cs
- DesignBindingPicker.cs
- BordersPage.cs
- UnknownBitmapDecoder.cs
- SymLanguageType.cs
- RuntimeResourceSet.cs
- ColumnMapProcessor.cs
- ContainerAction.cs
- DrawTreeNodeEventArgs.cs
- GeneralTransform.cs
- VersionedStreamOwner.cs
- StandardOleMarshalObject.cs
- SubclassTypeValidator.cs
- _OverlappedAsyncResult.cs
- Rfc4050KeyFormatter.cs
- DataObjectSettingDataEventArgs.cs
- ListItemConverter.cs
- CodeExpressionCollection.cs
- Bits.cs
- ColumnMapCopier.cs
- DockEditor.cs
- _AuthenticationState.cs
- InvalidWMPVersionException.cs
- FileCodeGroup.cs
- ExtensionSimplifierMarkupObject.cs
- ToolStripRenderEventArgs.cs
- ProfileProvider.cs
- AsyncCompletedEventArgs.cs
- grammarelement.cs
- RegisteredHiddenField.cs
- Directory.cs
- FixedNode.cs
- XPathExpr.cs
- RepeaterDataBoundAdapter.cs
- CodeDirectionExpression.cs
- ErrorFormatterPage.cs
- SqlDataSourceFilteringEventArgs.cs
- linebase.cs
- ObjectDataSourceEventArgs.cs
- GridViewPageEventArgs.cs
- BinaryReader.cs
- DoubleKeyFrameCollection.cs
- ValidatedControlConverter.cs
- ProjectionCamera.cs
- Nodes.cs
- WebPartConnectionsConfigureVerb.cs
- _FtpControlStream.cs
- RequiredAttributeAttribute.cs
- NativeMethods.cs
- PassportIdentity.cs
- SqlMethodAttribute.cs
- IisNotInstalledException.cs
- Cursors.cs
- DescendentsWalkerBase.cs
- SiteMapNodeItemEventArgs.cs
- FamilyMapCollection.cs
- KeyPullup.cs
- Int16AnimationUsingKeyFrames.cs
- XsltQilFactory.cs
- ConfigXmlSignificantWhitespace.cs
- PingReply.cs
- EtwTrace.cs
- ObjectList.cs
- StateDesigner.Helpers.cs
- RSACryptoServiceProvider.cs
- TextFormattingConverter.cs
- IsolatedStorageFileStream.cs
- MemberExpression.cs
- ComPlusTraceRecord.cs
- Input.cs
- UnsignedPublishLicense.cs
- BooleanConverter.cs
- UseAttributeSetsAction.cs
- DataServiceQuery.cs
- ImageSource.cs
- CompilerInfo.cs
- ResourcePool.cs
- DataGridColumnHeaderCollection.cs
- RoutedEventArgs.cs
- SqlDataSourceCommandEventArgs.cs
- HttpModule.cs
- HebrewCalendar.cs
- CodeGenerator.cs
- AncillaryOps.cs
- TextRenderer.cs
- Compiler.cs
- StatusBarItem.cs
- SuppressIldasmAttribute.cs
- ImageAnimator.cs
- TypeToStringValueConverter.cs
- MappedMetaModel.cs