Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / AsyncCompletedEventArgs.cs / 1 / AsyncCompletedEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; using System.Reflection; [HostProtection(SharedState = true)] public class AsyncCompletedEventArgs : System.EventArgs { private readonly Exception error; private readonly bool cancelled; private readonly object userState; public AsyncCompletedEventArgs(Exception error, bool cancelled, object userState) { this.error = error; this.cancelled = cancelled; this.userState = userState; } [ SRDescription(SR.Async_AsyncEventArgs_Cancelled) ] public bool Cancelled { get { return cancelled; } } [ SRDescription(SR.Async_AsyncEventArgs_Error) ] public Exception Error { get { return error; } } [ SRDescription(SR.Async_AsyncEventArgs_UserState) ] public object UserState { get { return userState; } } // Call from every result 'getter'. Will throw if there's an error or operation was cancelled // [SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")] protected void RaiseExceptionIfNecessary() { if (Error != null) { throw new TargetInvocationException(SR.GetString(SR.Async_ExceptionOccurred), Error); } else if (Cancelled) { throw new InvalidOperationException(SR.GetString(SR.Async_OperationCancelled)); } } } }
Link Menu
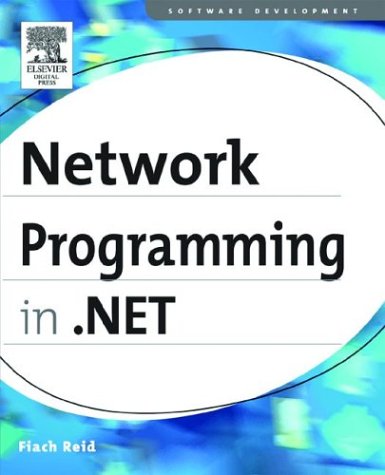
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerNearMe.cs
- FontCollection.cs
- SmiContext.cs
- ZipIOLocalFileDataDescriptor.cs
- UriParserTemplates.cs
- Preprocessor.cs
- DataSet.cs
- CryptoApi.cs
- MetadataPropertyAttribute.cs
- ProxyWebPartManager.cs
- WindowsFormsSynchronizationContext.cs
- MemberDomainMap.cs
- FontSizeConverter.cs
- NullReferenceException.cs
- WeakReadOnlyCollection.cs
- DesignerRegion.cs
- XamlPathDataSerializer.cs
- TextRunCacheImp.cs
- DurationConverter.cs
- DesignerListAdapter.cs
- querybuilder.cs
- SemanticKeyElement.cs
- LassoSelectionBehavior.cs
- InstanceData.cs
- RadioButtonList.cs
- RequiredArgumentAttribute.cs
- CodeVariableDeclarationStatement.cs
- AppDomainGrammarProxy.cs
- DispatcherExceptionFilterEventArgs.cs
- TimeSpan.cs
- AssemblyFilter.cs
- PartialClassGenerationTaskInternal.cs
- CommandCollectionEditor.cs
- PeerEndPoint.cs
- EventListenerClientSide.cs
- Point4DValueSerializer.cs
- HtmlLink.cs
- CompositeDataBoundControl.cs
- DataControlImageButton.cs
- CodeArrayCreateExpression.cs
- GuidelineSet.cs
- WebPartZoneBaseDesigner.cs
- TargetInvocationException.cs
- HttpCacheParams.cs
- CheckBoxList.cs
- RegexCaptureCollection.cs
- FullTextLine.cs
- ExpressionDumper.cs
- StandardCommands.cs
- X509KeyIdentifierClauseType.cs
- WorkflowDesignerColors.cs
- ExtensionQuery.cs
- XamlPathDataSerializer.cs
- StateElementCollection.cs
- SynchronizedDispatch.cs
- Int16.cs
- ChangesetResponse.cs
- DecimalAnimationUsingKeyFrames.cs
- ConsoleTraceListener.cs
- SqlParameterCollection.cs
- FixedTextPointer.cs
- Selection.cs
- SrgsElementFactory.cs
- DesignConnection.cs
- UnknownBitmapDecoder.cs
- ActivityTypeDesigner.xaml.cs
- NumberSubstitution.cs
- TypeGeneratedEventArgs.cs
- StorageRoot.cs
- PrintPreviewDialog.cs
- UxThemeWrapper.cs
- DrawingAttributeSerializer.cs
- MouseBinding.cs
- RichTextBox.cs
- PropertyItemInternal.cs
- FilteredDataSetHelper.cs
- LambdaCompiler.Statements.cs
- ComboBoxItem.cs
- ContextMenuStrip.cs
- SmiXetterAccessMap.cs
- RenderingBiasValidation.cs
- StandardCommands.cs
- CounterNameConverter.cs
- ToolStripDropDownMenu.cs
- MetadataArtifactLoader.cs
- VirtualizedItemProviderWrapper.cs
- SourceChangedEventArgs.cs
- Matrix.cs
- CodeDirectionExpression.cs
- SystemIPGlobalStatistics.cs
- HitTestFilterBehavior.cs
- SqlError.cs
- Accessible.cs
- XmlSerializerFactory.cs
- RenderingEventArgs.cs
- InheritanceAttribute.cs
- EntityDesignerDataSourceView.cs
- CacheRequest.cs
- WebPartDeleteVerb.cs
- TableProviderWrapper.cs