Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Management / BufferedWebEventProvider.cs / 1 / BufferedWebEventProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Management { using System; using System.Web; using System.Diagnostics; using System.Web.Util; using System.Web.Configuration; using System.Configuration.Provider; using System.Collections; using System.Collections.Specialized; using System.Configuration; using System.Security; using Debug=System.Web.Util.Debug; using System.Security.Permissions; // Interface for buffered event provider [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class BufferedWebEventProvider : WebEventProvider { bool _buffer = true; string _bufferMode; WebEventBuffer _webEventBuffer; public override void Initialize(string name, NameValueCollection config) { // create buffer according to the buffer mode settings specified, like we do in sql/mail providers // wire up the delegate to the ProcessEventFlush method Debug.Trace("BufferedWebEventProvider", "Initializing: name=" + name); ProviderUtil.GetAndRemoveBooleanAttribute(config, "buffer", name, ref _buffer); if (_buffer) { ProviderUtil.GetAndRemoveRequiredNonEmptyStringAttribute(config, "bufferMode", name, ref _bufferMode); _webEventBuffer = new WebEventBuffer(this, _bufferMode, new WebEventBufferFlushCallback(this.ProcessEventFlush)); } else { ProviderUtil.GetAndRemoveStringAttribute(config, "bufferMode", name, ref _bufferMode); } base.Initialize(name, config); ProviderUtil.CheckUnrecognizedAttributes(config, name); } public bool UseBuffering { get { return _buffer; } } public string BufferMode { get { return _bufferMode; } } public override void ProcessEvent(WebBaseEvent eventRaised) { if (_buffer) { // register the event with the buffer instead of writing it out Debug.Trace("BufferedWebEventProvider", "Saving event to buffer: event=" + eventRaised.GetType().Name); _webEventBuffer.AddEvent(eventRaised); } else { WebEventBufferFlushInfo flushInfo = new WebEventBufferFlushInfo( new WebBaseEventCollection(eventRaised), EventNotificationType.Unbuffered, 0, DateTime.MinValue, 0, 0); ProcessEventFlush(flushInfo); } } public abstract void ProcessEventFlush(WebEventBufferFlushInfo flushInfo); public override void Flush() { if (_buffer) { _webEventBuffer.Flush(Int32.MaxValue, FlushCallReason.StaticFlush); } } public override void Shutdown() { if (_webEventBuffer != null) { _webEventBuffer.Shutdown(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Management { using System; using System.Web; using System.Diagnostics; using System.Web.Util; using System.Web.Configuration; using System.Configuration.Provider; using System.Collections; using System.Collections.Specialized; using System.Configuration; using System.Security; using Debug=System.Web.Util.Debug; using System.Security.Permissions; // Interface for buffered event provider [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class BufferedWebEventProvider : WebEventProvider { bool _buffer = true; string _bufferMode; WebEventBuffer _webEventBuffer; public override void Initialize(string name, NameValueCollection config) { // create buffer according to the buffer mode settings specified, like we do in sql/mail providers // wire up the delegate to the ProcessEventFlush method Debug.Trace("BufferedWebEventProvider", "Initializing: name=" + name); ProviderUtil.GetAndRemoveBooleanAttribute(config, "buffer", name, ref _buffer); if (_buffer) { ProviderUtil.GetAndRemoveRequiredNonEmptyStringAttribute(config, "bufferMode", name, ref _bufferMode); _webEventBuffer = new WebEventBuffer(this, _bufferMode, new WebEventBufferFlushCallback(this.ProcessEventFlush)); } else { ProviderUtil.GetAndRemoveStringAttribute(config, "bufferMode", name, ref _bufferMode); } base.Initialize(name, config); ProviderUtil.CheckUnrecognizedAttributes(config, name); } public bool UseBuffering { get { return _buffer; } } public string BufferMode { get { return _bufferMode; } } public override void ProcessEvent(WebBaseEvent eventRaised) { if (_buffer) { // register the event with the buffer instead of writing it out Debug.Trace("BufferedWebEventProvider", "Saving event to buffer: event=" + eventRaised.GetType().Name); _webEventBuffer.AddEvent(eventRaised); } else { WebEventBufferFlushInfo flushInfo = new WebEventBufferFlushInfo( new WebBaseEventCollection(eventRaised), EventNotificationType.Unbuffered, 0, DateTime.MinValue, 0, 0); ProcessEventFlush(flushInfo); } } public abstract void ProcessEventFlush(WebEventBufferFlushInfo flushInfo); public override void Flush() { if (_buffer) { _webEventBuffer.Flush(Int32.MaxValue, FlushCallReason.StaticFlush); } } public override void Shutdown() { if (_webEventBuffer != null) { _webEventBuffer.Shutdown(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
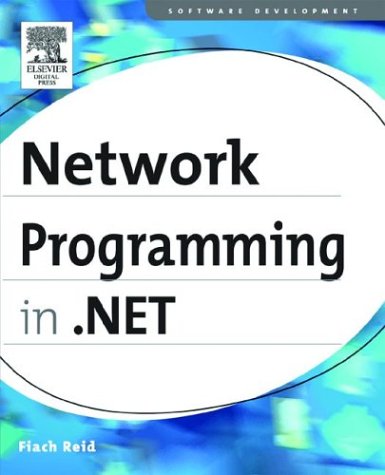
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdapterUtil.cs
- Camera.cs
- ErrorWrapper.cs
- ColumnMapCopier.cs
- LicFileLicenseProvider.cs
- VerticalAlignConverter.cs
- XmlIncludeAttribute.cs
- SecurityHelper.cs
- XmlCharacterData.cs
- SqlServices.cs
- CodeMemberEvent.cs
- Policy.cs
- QueryTask.cs
- OdbcErrorCollection.cs
- TransactedBatchContext.cs
- XmlTextReader.cs
- Cursor.cs
- FocusChangedEventArgs.cs
- HttpPostedFile.cs
- HttpServerProtocol.cs
- FormatException.cs
- LocatorPartList.cs
- Schema.cs
- EntitySqlException.cs
- OleDbConnection.cs
- GeometryCollection.cs
- EmptyCollection.cs
- VisualBasicHelper.cs
- PasswordPropertyTextAttribute.cs
- PrintDialog.cs
- LambdaCompiler.cs
- XamlVector3DCollectionSerializer.cs
- PriorityItem.cs
- SecurityMode.cs
- RIPEMD160.cs
- MeasurementDCInfo.cs
- RowBinding.cs
- IxmlLineInfo.cs
- SiteMapProvider.cs
- SafeNativeMethods.cs
- Relationship.cs
- InstanceLockLostException.cs
- TableCellCollection.cs
- DocumentsTrace.cs
- ServiceInstanceProvider.cs
- AlignmentXValidation.cs
- NumberFormatter.cs
- TextEditorSpelling.cs
- httpapplicationstate.cs
- NumericPagerField.cs
- UpdateManifestForBrowserApplication.cs
- XmlSchemaImporter.cs
- RoutedEvent.cs
- FtpRequestCacheValidator.cs
- HtmlTableCellCollection.cs
- XmlQuerySequence.cs
- BitmapEffectGeneralTransform.cs
- ConditionCollection.cs
- List.cs
- WpfWebRequestHelper.cs
- CollectionViewProxy.cs
- BypassElement.cs
- XmlSchemaFacet.cs
- ComponentChangingEvent.cs
- NavigationHelper.cs
- DbConnectionPoolGroupProviderInfo.cs
- BinaryWriter.cs
- MD5HashHelper.cs
- EventSinkHelperWriter.cs
- WorkflowStateRollbackService.cs
- VisualStyleInformation.cs
- ReachFixedDocumentSerializerAsync.cs
- PageCatalogPartDesigner.cs
- FileDialog_Vista.cs
- ProfileSection.cs
- TypedServiceChannelBuilder.cs
- RegexFCD.cs
- _RequestCacheProtocol.cs
- IntAverageAggregationOperator.cs
- DecimalKeyFrameCollection.cs
- CredentialCache.cs
- StdValidatorsAndConverters.cs
- MethodRental.cs
- IdentifierCollection.cs
- ValidationSummary.cs
- ButtonField.cs
- IteratorDescriptor.cs
- TablePattern.cs
- RoutedEventConverter.cs
- SqlConnectionString.cs
- ExpressionNormalizer.cs
- ColumnMapVisitor.cs
- UrlAuthFailedErrorFormatter.cs
- HashAlgorithm.cs
- DesignerForm.cs
- PrincipalPermission.cs
- IdentityHolder.cs
- _NativeSSPI.cs
- Atom10ItemFormatter.cs
- CssTextWriter.cs