Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / ProcessActivityTreeOptions.cs / 1305376 / ProcessActivityTreeOptions.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System.Collections.Generic; using System.Activities.Validation; using System.Runtime; class ProcessActivityTreeOptions { static ProcessActivityTreeOptions validationOptions; static ProcessActivityTreeOptions singleLevelValidationOptions; static ProcessActivityTreeOptions fullCachingOptions; static ProcessActivityTreeOptions finishCachingSubtreeOptionsWithCreateEmptyBindings; static ProcessActivityTreeOptions finishCachingSubtreeOptionsWithoutCreateEmptyBindings; ProcessActivityTreeOptions() { } public static ProcessActivityTreeOptions FullCachingOptions { get { if (fullCachingOptions == null) { fullCachingOptions = new ProcessActivityTreeOptions { SkipIfCached = true, CreateEmptyBindings = true, OnlyCallCallbackForDeclarations = true }; } return fullCachingOptions; } } public static ProcessActivityTreeOptions ValidationOptions { get { if (validationOptions == null) { validationOptions = new ProcessActivityTreeOptions { SkipPrivateChildren = false, // We don't want to interfere with activities doing null-checks // by creating empty bindings. CreateEmptyBindings = false }; } return validationOptions; } } static ProcessActivityTreeOptions SingleLevelValidationOptions { get { if (singleLevelValidationOptions == null) { singleLevelValidationOptions = new ProcessActivityTreeOptions { SkipPrivateChildren = false, // We don't want to interfere with activities doing null-checks // by creating empty bindings. CreateEmptyBindings = false, OnlyVisitSingleLevel = true }; } return singleLevelValidationOptions; } } static ProcessActivityTreeOptions FinishCachingSubtreeOptionsWithoutCreateEmptyBindings { get { if (finishCachingSubtreeOptionsWithoutCreateEmptyBindings == null) { // We don't want to run constraints and we only want to hit // the public path. finishCachingSubtreeOptionsWithoutCreateEmptyBindings = new ProcessActivityTreeOptions { SkipConstraints = true, StoreTempViolations = true }; } return finishCachingSubtreeOptionsWithoutCreateEmptyBindings; } } static ProcessActivityTreeOptions FinishCachingSubtreeOptionsWithCreateEmptyBindings { get { if (finishCachingSubtreeOptionsWithCreateEmptyBindings == null) { // We don't want to run constraints and we only want to hit // the public path. finishCachingSubtreeOptionsWithCreateEmptyBindings = new ProcessActivityTreeOptions { SkipConstraints = true, CreateEmptyBindings = true, StoreTempViolations = true }; } return finishCachingSubtreeOptionsWithCreateEmptyBindings; } } public static ProcessActivityTreeOptions GetFinishCachingSubtreeOptions(ProcessActivityTreeOptions originalOptions) { if (originalOptions.CreateEmptyBindings) { return ProcessActivityTreeOptions.FinishCachingSubtreeOptionsWithCreateEmptyBindings; } else { return ProcessActivityTreeOptions.FinishCachingSubtreeOptionsWithoutCreateEmptyBindings; } } public static ProcessActivityTreeOptions GetValidationOptions(ValidationSettings settings) { if (settings.SingleLevel) { return ProcessActivityTreeOptions.SingleLevelValidationOptions; } else { return ProcessActivityTreeOptions.ValidationOptions; } } public bool SkipIfCached { get; private set; } public bool CreateEmptyBindings { get; private set; } public bool SkipPrivateChildren { get; private set; } public bool OnlyCallCallbackForDeclarations { get; private set; } public bool SkipConstraints { get; private set; } public bool OnlyVisitSingleLevel { get; private set; } public bool StoreTempViolations { get; private set; } public bool IsRuntimeReadyOptions { get { // We don't really support progressive caching at runtime so we only set ourselves // as runtime ready if we cached the whole workflow and created empty bindings. // In order to support progressive caching we need to deal with the following // issues: // * We need a mechanism for supporting activities which supply extensions // * We need to understand when we haven't created empty bindings so that // we can progressively create them return !this.SkipPrivateChildren && this.CreateEmptyBindings; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
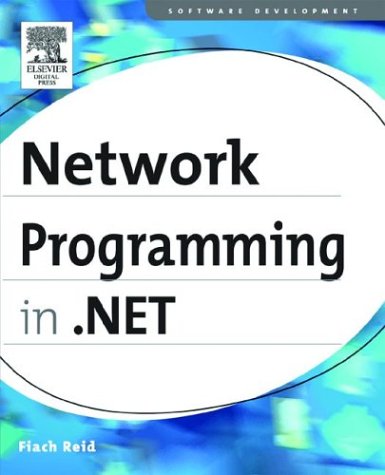
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Model3D.cs
- WebPermission.cs
- BaseParser.cs
- DataGridCellAutomationPeer.cs
- TextElementEditingBehaviorAttribute.cs
- ListItemCollection.cs
- FlatButtonAppearance.cs
- MD5.cs
- IncrementalCompileAnalyzer.cs
- CheckBoxStandardAdapter.cs
- Site.cs
- TextDecorationUnitValidation.cs
- DefaultValueAttribute.cs
- HttpHandlerActionCollection.cs
- ResourceAttributes.cs
- ImageField.cs
- ModelFactory.cs
- GetWinFXPath.cs
- MetabaseReader.cs
- CatalogPartChrome.cs
- FlowDocumentView.cs
- DecoratedNameAttribute.cs
- Comparer.cs
- Schema.cs
- EntityClassGenerator.cs
- securitymgrsite.cs
- BindingNavigator.cs
- ExtendedPropertyCollection.cs
- ValidatorCollection.cs
- ObfuscateAssemblyAttribute.cs
- SafeFreeMibTable.cs
- Size.cs
- ReadOnlyHierarchicalDataSourceView.cs
- HttpProfileGroupBase.cs
- CodeTryCatchFinallyStatement.cs
- ViewLoader.cs
- DataGridViewDataConnection.cs
- SamlSecurityTokenAuthenticator.cs
- XPathPatternParser.cs
- DependencyObjectType.cs
- Site.cs
- HttpStreams.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SiteMapDataSourceDesigner.cs
- baseaxisquery.cs
- Timer.cs
- SafeJobHandle.cs
- ExcCanonicalXml.cs
- OleDbStruct.cs
- SmtpTransport.cs
- RoleGroupCollection.cs
- XmlSchemaException.cs
- XNodeSchemaApplier.cs
- InkCanvasInnerCanvas.cs
- Int32RectConverter.cs
- MemberMaps.cs
- RepeatButton.cs
- HostExecutionContextManager.cs
- WrappedIUnknown.cs
- ConnectionStringSettings.cs
- ProfileService.cs
- WebDescriptionAttribute.cs
- PackWebResponse.cs
- QuaternionKeyFrameCollection.cs
- WinFormsSpinner.cs
- DataGridColumnStyleMappingNameEditor.cs
- AspNetPartialTrustHelpers.cs
- InternalCompensate.cs
- AssertFilter.cs
- MultiPageTextView.cs
- BamlTreeMap.cs
- TagPrefixCollection.cs
- ListViewGroupCollectionEditor.cs
- DesignerSerializationOptionsAttribute.cs
- ContextStaticAttribute.cs
- BinaryFormatter.cs
- UnicastIPAddressInformationCollection.cs
- PageStatePersister.cs
- Bezier.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- BezierSegment.cs
- Currency.cs
- CoTaskMemSafeHandle.cs
- HtmlButton.cs
- InlineCollection.cs
- Schema.cs
- IndexerNameAttribute.cs
- TreeNodeSelectionProcessor.cs
- LinqDataSourceStatusEventArgs.cs
- XmlExtensionFunction.cs
- Int16.cs
- IssuanceTokenProviderState.cs
- XmlSchemaProviderAttribute.cs
- TextTrailingCharacterEllipsis.cs
- OneOfTypeConst.cs
- Font.cs
- PerspectiveCamera.cs
- ThreadInterruptedException.cs
- BindingEntityInfo.cs
- Empty.cs