Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / ClientServices / ClientFormsIdentity.cs / 1305376 / ClientFormsIdentity.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ClientServices { using System; using System.Net; using System.Security.Principal; using System.Runtime.InteropServices; using System.Security; using System.Web.Security; using System.Diagnostics.CodeAnalysis; public class ClientFormsIdentity : IIdentity, IDisposable { public string Name { get { return _Name; }} public bool IsAuthenticated { get { return _IsAuthenticated; }} public string AuthenticationType { get { return _AuthenticationType; } } public CookieContainer AuthenticationCookies { get { return _AuthenticationCookies; } } public MembershipProvider Provider { get { return _Provider; } } public ClientFormsIdentity(string name, string password, MembershipProvider provider, string authenticationType, bool isAuthenticated, CookieContainer authenticationCookies) { _Name = name; _AuthenticationType = authenticationType; _IsAuthenticated = isAuthenticated; _AuthenticationCookies = authenticationCookies; _Password = GetSecureStringFromString(password); _Provider = provider; } public void RevalidateUser() { if (_Disposed) { throw new ObjectDisposedException(this.GetType().FullName); } _Provider.ValidateUser(_Name, GetStringFromSecureString(_Password)); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { if (disposing) { if (_Password != null) { _Password.Dispose(); } } _Disposed = true; } private string _Name; private bool _IsAuthenticated; private string _AuthenticationType; private CookieContainer _AuthenticationCookies; private SecureString _Password; private MembershipProvider _Provider; private bool _Disposed; private static SecureString GetSecureStringFromString(string password) { char[] passwordChars = password.ToCharArray(); SecureString ss = new SecureString(); for (int iter = 0; iter < passwordChars.Length; iter++) ss.AppendChar(passwordChars[iter]); ss.MakeReadOnly(); return ss; } [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Justification="Reviewed and approved by feature crew")] [SecuritySafeCritical] private static string GetStringFromSecureString(SecureString securePass) { IntPtr bstr = IntPtr.Zero; try { bstr = Marshal.SecureStringToBSTR(securePass); return Marshal.PtrToStringBSTR(bstr); } finally { if (bstr != IntPtr.Zero) Marshal.FreeBSTR(bstr); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.ClientServices { using System; using System.Net; using System.Security.Principal; using System.Runtime.InteropServices; using System.Security; using System.Web.Security; using System.Diagnostics.CodeAnalysis; public class ClientFormsIdentity : IIdentity, IDisposable { public string Name { get { return _Name; }} public bool IsAuthenticated { get { return _IsAuthenticated; }} public string AuthenticationType { get { return _AuthenticationType; } } public CookieContainer AuthenticationCookies { get { return _AuthenticationCookies; } } public MembershipProvider Provider { get { return _Provider; } } public ClientFormsIdentity(string name, string password, MembershipProvider provider, string authenticationType, bool isAuthenticated, CookieContainer authenticationCookies) { _Name = name; _AuthenticationType = authenticationType; _IsAuthenticated = isAuthenticated; _AuthenticationCookies = authenticationCookies; _Password = GetSecureStringFromString(password); _Provider = provider; } public void RevalidateUser() { if (_Disposed) { throw new ObjectDisposedException(this.GetType().FullName); } _Provider.ValidateUser(_Name, GetStringFromSecureString(_Password)); } public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { if (disposing) { if (_Password != null) { _Password.Dispose(); } } _Disposed = true; } private string _Name; private bool _IsAuthenticated; private string _AuthenticationType; private CookieContainer _AuthenticationCookies; private SecureString _Password; private MembershipProvider _Provider; private bool _Disposed; private static SecureString GetSecureStringFromString(string password) { char[] passwordChars = password.ToCharArray(); SecureString ss = new SecureString(); for (int iter = 0; iter < passwordChars.Length; iter++) ss.AppendChar(passwordChars[iter]); ss.MakeReadOnly(); return ss; } [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Justification="Reviewed and approved by feature crew")] [SecuritySafeCritical] private static string GetStringFromSecureString(SecureString securePass) { IntPtr bstr = IntPtr.Zero; try { bstr = Marshal.SecureStringToBSTR(securePass); return Marshal.PtrToStringBSTR(bstr); } finally { if (bstr != IntPtr.Zero) Marshal.FreeBSTR(bstr); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
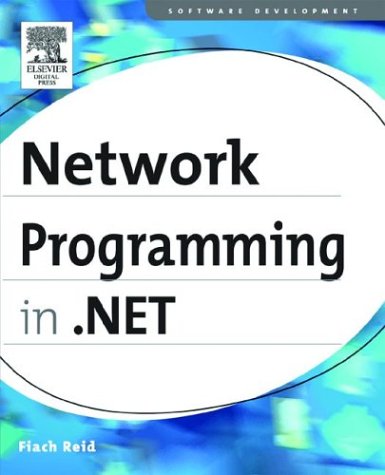
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuAutoFormat.cs
- LongValidatorAttribute.cs
- PagedControl.cs
- PtsPage.cs
- PassportAuthentication.cs
- TextEffect.cs
- ConnectivityStatus.cs
- DynamicDiscoSearcher.cs
- HtmlForm.cs
- TextPatternIdentifiers.cs
- PackageRelationshipCollection.cs
- DrawToolTipEventArgs.cs
- EvidenceTypeDescriptor.cs
- StateManagedCollection.cs
- EncoderParameter.cs
- Compiler.cs
- HintTextConverter.cs
- ToolBarButton.cs
- FixedNode.cs
- HtmlInputImage.cs
- brushes.cs
- BinHexDecoder.cs
- TextProviderWrapper.cs
- GuidTagList.cs
- StopStoryboard.cs
- RangeContentEnumerator.cs
- OneToOneMappingSerializer.cs
- RequestQueue.cs
- ImageFormatConverter.cs
- BinaryObjectReader.cs
- RadioButtonStandardAdapter.cs
- FormatterServices.cs
- Base64Encoding.cs
- X509Certificate.cs
- CursorInteropHelper.cs
- TraceListener.cs
- SchemaTableOptionalColumn.cs
- MarshalDirectiveException.cs
- Pkcs7Recipient.cs
- CacheSection.cs
- wmiutil.cs
- Interlocked.cs
- LassoSelectionBehavior.cs
- FormViewUpdatedEventArgs.cs
- InnerItemCollectionView.cs
- WebControlAdapter.cs
- EndpointBehaviorElement.cs
- Pair.cs
- UIElementPropertyUndoUnit.cs
- NameSpaceEvent.cs
- PerformanceCounterPermissionAttribute.cs
- DataChangedEventManager.cs
- HandledMouseEvent.cs
- SystemBrushes.cs
- HostedElements.cs
- SlipBehavior.cs
- TextDataBindingHandler.cs
- LockCookie.cs
- FunctionUpdateCommand.cs
- PermissionAttributes.cs
- MailWebEventProvider.cs
- VolatileResourceManager.cs
- ConfigXmlComment.cs
- IsolatedStorageFileStream.cs
- ToolStripSplitButton.cs
- PrintController.cs
- ToolBarTray.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- ToolStripScrollButton.cs
- Button.cs
- DataGridTable.cs
- ToolStripDropDownItem.cs
- LicenseProviderAttribute.cs
- MimeTypeMapper.cs
- CompositeDispatchFormatter.cs
- EnumCodeDomSerializer.cs
- JavaScriptObjectDeserializer.cs
- DesignerProperties.cs
- ZipIOModeEnforcingStream.cs
- SyndicationDeserializer.cs
- ChannelManager.cs
- PathGeometry.cs
- UInt16Storage.cs
- SkinIDTypeConverter.cs
- ObjectItemCollection.cs
- CustomAssemblyResolver.cs
- RecordsAffectedEventArgs.cs
- TabControlCancelEvent.cs
- PropertyTabAttribute.cs
- NullReferenceException.cs
- InplaceBitmapMetadataWriter.cs
- BaseCodePageEncoding.cs
- CopyNodeSetAction.cs
- MetadataItem.cs
- StreamAsIStream.cs
- DeleteMemberBinder.cs
- CodeExporter.cs
- Compiler.cs
- TextEditorParagraphs.cs
- SocketAddress.cs