Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Runtime / Remoting / ContextProperty.cs / 1 / ContextProperty.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: ContextProperty.cs ** ** A contextProperty is a name-value pair holding the property ** name and the object representing the property in a context. ** An array of these is returned by Context::GetContextProperties() ** ** ** ===========================================================*/ namespace System.Runtime.Remoting.Contexts { using System; using System.Threading; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.Remoting.Activation; using System.Security.Permissions; ///[SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] [SecurityPermissionAttribute(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.Infrastructure)] [System.Runtime.InteropServices.ComVisible(true)] public class ContextProperty { internal String _name; // property name internal Object _property; // property object /// public virtual String Name { get { return _name; } } /// public virtual Object Property { get { return _property; } } /* can't create outside the package */ internal ContextProperty(String name, Object prop) { _name = name; _property = prop; } } // The IContextAttribute interface is implemented by attribute classes. // The attributes contribute a property which resides in a context and // enforces a specific policy for the objects created in that context. /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextAttribute { /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsContextOK(Context ctx, IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void GetPropertiesForNewContext(IConstructionCallMessage msg); } // This interface is exposed by the property contributed to a context // by an attribute. By default, it is also implemented by the ContextAttribute // base class which every attribute class must extend from. /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextProperty { /// // This is the name under which the property will be added // to the {name,property} table in a context. String Name { [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] get; } /// // After forming the newCtx, we ask each property if it is happy // with the context. We expect most implementations to say yes. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsNewContextOK(Context newCtx); /// // New method. All properties are notified when the context // they are in is frozen. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void Freeze(Context newContext); } /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextPropertyActivator { /// // This method lets properties in the current context have a say in // whether an activation may be done 'here' or not. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsOKToActivate(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void CollectFromClientContext(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool DeliverClientContextToServerContext(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void CollectFromServerContext(IConstructionReturnMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool DeliverServerContextToClientContext(IConstructionReturnMessage msg); } // All context attribute classes must extend from this base class. // This class provides the base implementations which the derived // classes are free to over-ride. The base implementations provide // the default answers to various questions. /// [AttributeUsage(AttributeTargets.Class), Serializable] [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] [SecurityPermissionAttribute(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.Infrastructure)] [System.Runtime.InteropServices.ComVisible(true)] public class ContextAttribute : Attribute, IContextAttribute, IContextProperty { /// protected String AttributeName; // The derived class must call: base(name); /// public ContextAttribute(String name) { AttributeName = name; } // IContextPropery::Name // Default implementation provides AttributeName as the property name. /// public virtual String Name { get {return AttributeName;} } // IContextProperty::IsNewContextOK /// public virtual bool IsNewContextOK(Context newCtx) { // This will be called before entering the newCtx // Default implementation says OK. return true; } // IContextProperty::Freeze // Default implementation does nothing /// public virtual void Freeze(Context newContext) { BCLDebug.Log("ContextAttribute::ContextProperty::Freeze"+ " for context " + newContext ); } // Object::Equals // Default implementation just compares the names /// public override bool Equals(Object o) { IContextProperty prop = o as IContextProperty; return (null != prop) && AttributeName.Equals(prop.Name); } /// public override int GetHashCode() { return this.AttributeName.GetHashCode(); } // IContextAttribute::IsContextOK // Default calls Object::Equals on the property and does not // bother with the ctorMsg. /// public virtual bool IsContextOK( Context ctx, IConstructionCallMessage ctorMsg) { if (ctx == null) throw new ArgumentNullException("ctx"); if (ctorMsg == null) throw new ArgumentNullException("ctorMsg"); BCLDebug.Assert(ctorMsg.ActivationType.IsMarshalByRef, "Activation on a non MarshalByRef object"); if (!ctorMsg.ActivationType.IsContextful) { return true; } Object prop = ctx.GetProperty(AttributeName); if ((prop!=null) && (Equals(prop))) { return true; } return false; } // IContextAttribute::GetPropertiesForNewContext // Default adds the attribute itself w/o regard to the current // list of properties /// public virtual void GetPropertiesForNewContext( IConstructionCallMessage ctorMsg) { if (ctorMsg == null) throw new ArgumentNullException("ctorMsg"); ctorMsg.ContextProperties.Add((IContextProperty)this); } } #if SIMPLEXAACTIVATION /// [AttributeUsage(AttributeTargets.Class)] [System.Runtime.InteropServices.ComVisible(true)] public class new_appdomain : ContextAttribute { internal static int _domain_no = 0; /// public new_appdomain() : base("new_appdomain") {} /// [System.Runtime.InteropServices.ComVisible(true)] public override bool IsContextOK(Context ctx, IConstructionCallMessage msg) { return false; } /// [System.Runtime.InteropServices.ComVisible(true)] public override void GetPropertiesForNewContext(IConstructionCallMessage ctorMsg) { ctorMsg.GetProperties()["__new_appdomain"] = true; } /// [System.Runtime.InteropServices.ComVisible(true)] public static IConstructionReturnMessage DoSimpleXADActivation(IConstructionCallMessage msg) { int domain_no = Interlocked.Increment(ref _domain_no); AppDomain ad = AppDomain.CreateDomain("AutoDomain #" + domain_no, null, null); activator a = (activator) (ad.CreateInstance(null, typeof(activator).FullName)).Unwrap(); return a.Activate(msg); } /// [System.Runtime.InteropServices.ComVisible(true)] public class activator : MarshalByRefObject, IActivator { /// [System.Runtime.InteropServices.ComVisible(true)] public IConstructionReturnMessage Activate(IConstructionCallMessage msg) { return RemotingServices.DoCrossContextActivation(msg); } /// [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public void LoadAssembly(AssemblyName an) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly a = Assembly.InternalLoad(an, false, null, ref stackMark); if (a == null) { throw new RemotingException( String.Format( Environment.GetResourceString( "Remoting_AssemblyLoadFailed"), an)); } } } } /// [new_appdomain] [System.Runtime.InteropServices.ComVisible(true)] public class MBR : MarshalByRefObject { /// public String MyAppDomain() { return Thread.GetDomain().GetFriendlyName(); } } /// [new_appdomain] [System.Runtime.InteropServices.ComVisible(true)] public class CB : ContextBoundObject { /// public String MyAppDomain() { return Thread.GetDomain().GetFriendlyName(); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** File: ContextProperty.cs ** ** A contextProperty is a name-value pair holding the property ** name and the object representing the property in a context. ** An array of these is returned by Context::GetContextProperties() ** ** ** ===========================================================*/ namespace System.Runtime.Remoting.Contexts { using System; using System.Threading; using System.Reflection; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.Remoting.Activation; using System.Security.Permissions; /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] [SecurityPermissionAttribute(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.Infrastructure)] [System.Runtime.InteropServices.ComVisible(true)] public class ContextProperty { internal String _name; // property name internal Object _property; // property object /// public virtual String Name { get { return _name; } } /// public virtual Object Property { get { return _property; } } /* can't create outside the package */ internal ContextProperty(String name, Object prop) { _name = name; _property = prop; } } // The IContextAttribute interface is implemented by attribute classes. // The attributes contribute a property which resides in a context and // enforces a specific policy for the objects created in that context. /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextAttribute { /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsContextOK(Context ctx, IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void GetPropertiesForNewContext(IConstructionCallMessage msg); } // This interface is exposed by the property contributed to a context // by an attribute. By default, it is also implemented by the ContextAttribute // base class which every attribute class must extend from. /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextProperty { /// // This is the name under which the property will be added // to the {name,property} table in a context. String Name { [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] get; } /// // After forming the newCtx, we ask each property if it is happy // with the context. We expect most implementations to say yes. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsNewContextOK(Context newCtx); /// // New method. All properties are notified when the context // they are in is frozen. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void Freeze(Context newContext); } /// [System.Runtime.InteropServices.ComVisible(true)] public interface IContextPropertyActivator { /// // This method lets properties in the current context have a say in // whether an activation may be done 'here' or not. [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool IsOKToActivate(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void CollectFromClientContext(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool DeliverClientContextToServerContext(IConstructionCallMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] void CollectFromServerContext(IConstructionReturnMessage msg); /// [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] bool DeliverServerContextToClientContext(IConstructionReturnMessage msg); } // All context attribute classes must extend from this base class. // This class provides the base implementations which the derived // classes are free to over-ride. The base implementations provide // the default answers to various questions. /// [AttributeUsage(AttributeTargets.Class), Serializable] [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.Infrastructure)] [SecurityPermissionAttribute(SecurityAction.InheritanceDemand, Flags=SecurityPermissionFlag.Infrastructure)] [System.Runtime.InteropServices.ComVisible(true)] public class ContextAttribute : Attribute, IContextAttribute, IContextProperty { /// protected String AttributeName; // The derived class must call: base(name); /// public ContextAttribute(String name) { AttributeName = name; } // IContextPropery::Name // Default implementation provides AttributeName as the property name. /// public virtual String Name { get {return AttributeName;} } // IContextProperty::IsNewContextOK /// public virtual bool IsNewContextOK(Context newCtx) { // This will be called before entering the newCtx // Default implementation says OK. return true; } // IContextProperty::Freeze // Default implementation does nothing /// public virtual void Freeze(Context newContext) { BCLDebug.Log("ContextAttribute::ContextProperty::Freeze"+ " for context " + newContext ); } // Object::Equals // Default implementation just compares the names /// public override bool Equals(Object o) { IContextProperty prop = o as IContextProperty; return (null != prop) && AttributeName.Equals(prop.Name); } /// public override int GetHashCode() { return this.AttributeName.GetHashCode(); } // IContextAttribute::IsContextOK // Default calls Object::Equals on the property and does not // bother with the ctorMsg. /// public virtual bool IsContextOK( Context ctx, IConstructionCallMessage ctorMsg) { if (ctx == null) throw new ArgumentNullException("ctx"); if (ctorMsg == null) throw new ArgumentNullException("ctorMsg"); BCLDebug.Assert(ctorMsg.ActivationType.IsMarshalByRef, "Activation on a non MarshalByRef object"); if (!ctorMsg.ActivationType.IsContextful) { return true; } Object prop = ctx.GetProperty(AttributeName); if ((prop!=null) && (Equals(prop))) { return true; } return false; } // IContextAttribute::GetPropertiesForNewContext // Default adds the attribute itself w/o regard to the current // list of properties /// public virtual void GetPropertiesForNewContext( IConstructionCallMessage ctorMsg) { if (ctorMsg == null) throw new ArgumentNullException("ctorMsg"); ctorMsg.ContextProperties.Add((IContextProperty)this); } } #if SIMPLEXAACTIVATION /// [AttributeUsage(AttributeTargets.Class)] [System.Runtime.InteropServices.ComVisible(true)] public class new_appdomain : ContextAttribute { internal static int _domain_no = 0; /// public new_appdomain() : base("new_appdomain") {} /// [System.Runtime.InteropServices.ComVisible(true)] public override bool IsContextOK(Context ctx, IConstructionCallMessage msg) { return false; } /// [System.Runtime.InteropServices.ComVisible(true)] public override void GetPropertiesForNewContext(IConstructionCallMessage ctorMsg) { ctorMsg.GetProperties()["__new_appdomain"] = true; } /// [System.Runtime.InteropServices.ComVisible(true)] public static IConstructionReturnMessage DoSimpleXADActivation(IConstructionCallMessage msg) { int domain_no = Interlocked.Increment(ref _domain_no); AppDomain ad = AppDomain.CreateDomain("AutoDomain #" + domain_no, null, null); activator a = (activator) (ad.CreateInstance(null, typeof(activator).FullName)).Unwrap(); return a.Activate(msg); } /// [System.Runtime.InteropServices.ComVisible(true)] public class activator : MarshalByRefObject, IActivator { /// [System.Runtime.InteropServices.ComVisible(true)] public IConstructionReturnMessage Activate(IConstructionCallMessage msg) { return RemotingServices.DoCrossContextActivation(msg); } /// [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public void LoadAssembly(AssemblyName an) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly a = Assembly.InternalLoad(an, false, null, ref stackMark); if (a == null) { throw new RemotingException( String.Format( Environment.GetResourceString( "Remoting_AssemblyLoadFailed"), an)); } } } } /// [new_appdomain] [System.Runtime.InteropServices.ComVisible(true)] public class MBR : MarshalByRefObject { /// public String MyAppDomain() { return Thread.GetDomain().GetFriendlyName(); } } /// [new_appdomain] [System.Runtime.InteropServices.ComVisible(true)] public class CB : ContextBoundObject { /// public String MyAppDomain() { return Thread.GetDomain().GetFriendlyName(); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
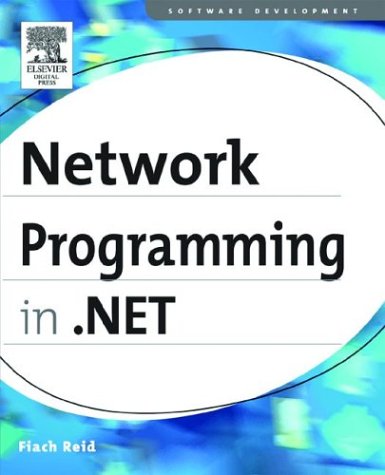
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlMappingsModule.cs
- CounterSampleCalculator.cs
- DataContractJsonSerializer.cs
- SemaphoreSecurity.cs
- XmlAtomicValue.cs
- ValueTypeFixupInfo.cs
- XmlEventCache.cs
- AttributeCollection.cs
- StyleModeStack.cs
- DependencyObject.cs
- Compress.cs
- ConfigPathUtility.cs
- CodeEntryPointMethod.cs
- CancelEventArgs.cs
- GenerateTemporaryTargetAssembly.cs
- ScrollViewerAutomationPeer.cs
- OperationDescription.cs
- ValueTypeFixupInfo.cs
- HttpWebRequestElement.cs
- WindowsStreamSecurityElement.cs
- RoleManagerEventArgs.cs
- IDQuery.cs
- XmlElementList.cs
- CachedCompositeFamily.cs
- WizardForm.cs
- BamlBinaryWriter.cs
- Rotation3DAnimationUsingKeyFrames.cs
- SimplePropertyEntry.cs
- PreservationFileReader.cs
- SqlDuplicator.cs
- NullEntityWrapper.cs
- DataGridViewBand.cs
- DiscardableAttribute.cs
- GeometryCollection.cs
- MatrixKeyFrameCollection.cs
- AnnotationService.cs
- XmlSchemaAnnotation.cs
- ToolStripItemCollection.cs
- TemplateBindingExtension.cs
- ErrorLog.cs
- DataSourceXmlSubItemAttribute.cs
- UpdateCompiler.cs
- TreeNode.cs
- UnsafeNativeMethods.cs
- SoapCodeExporter.cs
- ThreadAbortException.cs
- FatalException.cs
- TcpActivation.cs
- TreeNodeEventArgs.cs
- CheckBoxList.cs
- MemberPath.cs
- IntegerFacetDescriptionElement.cs
- WSFederationHttpBindingElement.cs
- ColorMatrix.cs
- QilFunction.cs
- CalendarItem.cs
- CategoryNameCollection.cs
- PasswordPropertyTextAttribute.cs
- HashSetEqualityComparer.cs
- EntityDataSourceMemberPath.cs
- SynchronizedPool.cs
- XmlSchemaCompilationSettings.cs
- X509ChainElement.cs
- FacetDescriptionElement.cs
- XmlCountingReader.cs
- ThreadAttributes.cs
- StateDesigner.CommentLayoutGlyph.cs
- SchemaMapping.cs
- ProgressChangedEventArgs.cs
- CompModSwitches.cs
- HttpRequest.cs
- TdsParserSessionPool.cs
- SegmentInfo.cs
- DtdParser.cs
- ParameterBuilder.cs
- DrawingAttributeSerializer.cs
- ByteKeyFrameCollection.cs
- ArrangedElementCollection.cs
- OSEnvironmentHelper.cs
- WebReferenceOptions.cs
- NotifyCollectionChangedEventArgs.cs
- XmlSecureResolver.cs
- PackageProperties.cs
- OverloadGroupAttribute.cs
- HostedTcpTransportManager.cs
- PersonalizationStateInfo.cs
- SqlUtil.cs
- CodeBlockBuilder.cs
- localization.cs
- XmlQueryContext.cs
- AnnotationResourceCollection.cs
- TraceContextEventArgs.cs
- Drawing.cs
- _IPv6Address.cs
- CompModSwitches.cs
- SearchForVirtualItemEventArgs.cs
- TextSelectionHelper.cs
- Variant.cs
- WmlLabelAdapter.cs
- UnsafeNativeMethods.cs