Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / XamlIntegration / TypeConverterBase.cs / 1305376 / TypeConverterBase.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.XamlIntegration { using System; using System.Collections.Concurrent; using System.ComponentModel; using System.Globalization; using System.Runtime; using System.Threading; using System.Xaml; public abstract class TypeConverterBase : TypeConverter { Lazy> helpers = new Lazy >(); TypeConverterHelper helper; Type baseType; Type helperType; internal TypeConverterBase(Type baseType, Type helperType) { this.baseType = baseType; this.helperType = helperType; } internal TypeConverterBase(Type targetType, Type baseType, Type helperType) { this.helper = GetTypeConverterHelper(targetType, baseType, helperType); } public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == TypeHelper.StringType) { return true; } return base.CanConvertFrom(context, sourceType); } public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == TypeHelper.StringType) { return false; } return base.CanConvertTo(context, destinationType); } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string stringValue = value as string; if (stringValue != null) { TypeConverterHelper currentHelper = helper; if (currentHelper == null) { IDestinationTypeProvider targetService = context.GetService(typeof(IDestinationTypeProvider)) as IDestinationTypeProvider; Type targetType = targetService.GetDestinationType(); if (!this.helpers.Value.TryGetValue(targetType, out currentHelper)) { currentHelper = GetTypeConverterHelper(targetType, this.baseType, this.helperType); if (!this.helpers.Value.TryAdd(targetType, currentHelper)) { if (!this.helpers.Value.TryGetValue(targetType, out currentHelper)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeConverterHelperCacheAddFailed(targetType))); } } } } object result = currentHelper.UntypedConvertFromString(stringValue, context); return result; } return base.ConvertFrom(context, culture, value); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { return base.ConvertTo(context, culture, value, destinationType); } TypeConverterHelper GetTypeConverterHelper(Type targetType, Type baseType, Type helperType) { Type[] genericTypeArguments; if (baseType.BaseType == targetType) { // support non-generic ActivityWithResult, In/Out/InOutArgument genericTypeArguments = new Type[] { TypeHelper.ObjectType }; } else { // Find baseType in the base class list of targetType while (!targetType.IsGenericType || !(targetType.GetGenericTypeDefinition() == baseType)) { if (targetType == TypeHelper.ObjectType) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidTypeConverterUsage)); } targetType = targetType.BaseType; } genericTypeArguments = targetType.GetGenericArguments(); } Type concreteHelperType = helperType.MakeGenericType(genericTypeArguments); return (TypeConverterHelper)Activator.CreateInstance(concreteHelperType); } internal abstract class TypeConverterHelper { public abstract object UntypedConvertFromString(string text, ITypeDescriptorContext context); public static T GetService (ITypeDescriptorContext context) where T : class { T service = (T)context.GetService(typeof(T)); if (service == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidTypeConverterUsage)); } return service; } } internal abstract class TypeConverterHelper : TypeConverterHelper { public abstract T ConvertFromString(string text, ITypeDescriptorContext context); public sealed override object UntypedConvertFromString(string text, ITypeDescriptorContext context) { return ConvertFromString(text, context); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
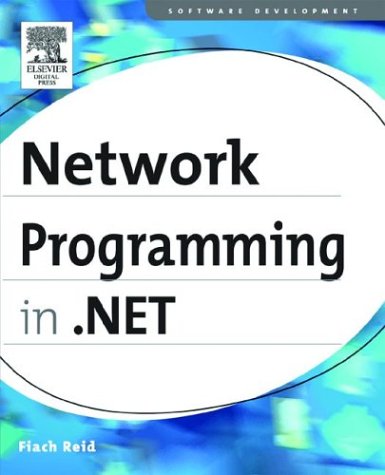
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NavigationEventArgs.cs
- TabletCollection.cs
- ColumnWidthChangingEvent.cs
- XmlNamespaceManager.cs
- ExpressionBinding.cs
- StopStoryboard.cs
- PropertyMetadata.cs
- DataServiceProviderWrapper.cs
- ServiceEndpoint.cs
- X509RawDataKeyIdentifierClause.cs
- ImageField.cs
- AtomicFile.cs
- CreateUserWizard.cs
- FunctionUpdateCommand.cs
- ThicknessAnimation.cs
- FixedSOMTable.cs
- HtmlElement.cs
- ParameterModifier.cs
- TextTreeRootNode.cs
- DbMetaDataFactory.cs
- SystemDiagnosticsSection.cs
- SupportsPreviewControlAttribute.cs
- RadialGradientBrush.cs
- MultiBindingExpression.cs
- SubMenuStyle.cs
- PagedDataSource.cs
- ListViewGroupConverter.cs
- BitmapCodecInfoInternal.cs
- BooleanFacetDescriptionElement.cs
- RootBuilder.cs
- RichTextBoxConstants.cs
- Point3DConverter.cs
- XmlSortKey.cs
- X509ChainPolicy.cs
- Tablet.cs
- ProxyAttribute.cs
- EntityTypeBase.cs
- URLIdentityPermission.cs
- PropertyOrder.cs
- DataGridViewElement.cs
- _HTTPDateParse.cs
- FacetDescription.cs
- WebPartCatalogCloseVerb.cs
- RoutedEventConverter.cs
- DefaultValueMapping.cs
- ObjectQueryState.cs
- UserMapPath.cs
- DataSpaceManager.cs
- CurrentChangingEventManager.cs
- PackageDigitalSignatureManager.cs
- CollectionsUtil.cs
- BreakSafeBase.cs
- HostingPreferredMapPath.cs
- ObjectParameter.cs
- OutputCacheSection.cs
- SQLGuid.cs
- SafeSecurityHandles.cs
- SafeWaitHandle.cs
- AnimationClockResource.cs
- SendSecurityHeaderElementContainer.cs
- CodeIterationStatement.cs
- Variable.cs
- EndpointInfoCollection.cs
- UnwrappedTypesXmlSerializerManager.cs
- AssertSection.cs
- _SafeNetHandles.cs
- DbProviderManifest.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- WinCategoryAttribute.cs
- FontSourceCollection.cs
- xml.cs
- EnumConverter.cs
- RawStylusInputCustomDataList.cs
- ConfigXmlElement.cs
- DispatcherExceptionFilterEventArgs.cs
- CopyCodeAction.cs
- TextSpan.cs
- FixedTextContainer.cs
- PointAnimationUsingKeyFrames.cs
- ObjRef.cs
- DataContract.cs
- ImageSource.cs
- Block.cs
- CapabilitiesAssignment.cs
- SoapHttpTransportImporter.cs
- TextSearch.cs
- GlyphInfoList.cs
- ProvidePropertyAttribute.cs
- PageThemeParser.cs
- ThreadPool.cs
- SocketStream.cs
- WebBrowsableAttribute.cs
- ProviderIncompatibleException.cs
- RemoteCryptoTokenProvider.cs
- SafePEFileHandle.cs
- CopyNamespacesAction.cs
- DbDataReader.cs
- SectionVisual.cs
- SafeRightsManagementQueryHandle.cs
- DictionaryKeyPropertyAttribute.cs