Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / ManagedLibraries / Remoting / Channels / IPC / PipeStream.cs / 1305376 / PipeStream.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //========================================================================== // File: PipeStream.cs // // Summary: Stream used for reading from a named pipe // Author: [....]@microsoft.com //========================================================================= using System; using System.IO; using System.Runtime.Remoting; namespace System.Runtime.Remoting.Channels.Ipc { // Basically the same as NetworkStream, but adds support for timeouts. internal sealed class PipeStream : Stream { private IpcPort _port; private int _timeout = 0; // throw timout exception if a read takes longer than this many milliseconds public PipeStream(IpcPort port) { if (port == null) throw new ArgumentNullException("port"); _port = port; } // SocketStream // Stream implementation public override bool CanRead { get { return true; } } public override bool CanSeek { get { return false; } } public override bool CanWrite { get { return true; } } public override long Length { get { throw new NotSupportedException(); } } public override long Position { get { throw new NotSupportedException(); } set { throw new NotSupportedException(); } } // Position public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(); } public override int Read(byte[] buffer, int offset, int size) { if (_timeout <= 0) { return _port.Read(buffer, offset, size); } else { IAsyncResult ar = _port.BeginRead(buffer, offset, size, null, null); if (_timeout>0 && !ar.IsCompleted) { ar.AsyncWaitHandle.WaitOne(_timeout, false); if (!ar.IsCompleted) throw new RemotingTimeoutException(); } return _port.EndRead(ar); } } // Read public override void Write(byte[] buffer, int offset, int count) { _port.Write(buffer, offset, count); } // Write protected override void Dispose(bool disposing) { try { if (disposing) _port.Dispose(); } finally { base.Dispose(disposing); } } public override void Flush() { } public override IAsyncResult BeginRead( byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { IAsyncResult asyncResult = _port.BeginRead( buffer, offset, size, callback, state); return asyncResult; } // BeginRead public override int EndRead(IAsyncResult asyncResult) { return _port.EndRead(asyncResult); } // EndRead public override IAsyncResult BeginWrite( byte[] buffer, int offset, int size, AsyncCallback callback, Object state) { throw new NotSupportedException(); } // BeginWrite public override void EndWrite(IAsyncResult asyncResult) { throw new NotSupportedException(); } // EndWrite public override void SetLength(long value) { throw new NotSupportedException(); } } // class SocketStream } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
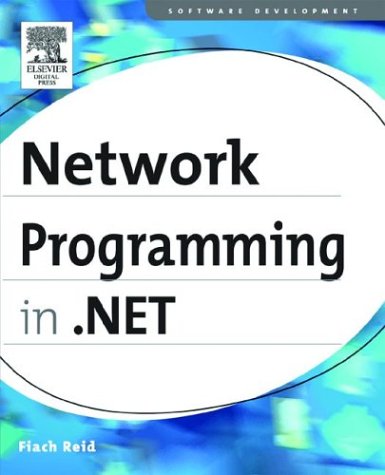
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InkCanvasSelection.cs
- BindingListCollectionView.cs
- WSSecureConversation.cs
- MonthChangedEventArgs.cs
- WebBrowserNavigatedEventHandler.cs
- Walker.cs
- Sentence.cs
- IODescriptionAttribute.cs
- EnterpriseServicesHelper.cs
- UserPreferenceChangedEventArgs.cs
- PagePropertiesChangingEventArgs.cs
- ObjectItemLoadingSessionData.cs
- XmlDocument.cs
- OpCellTreeNode.cs
- WebPart.cs
- BasicHttpMessageSecurity.cs
- HostedHttpContext.cs
- HttpCacheVary.cs
- _NtlmClient.cs
- DBSqlParserTable.cs
- SourceSwitch.cs
- SystemGatewayIPAddressInformation.cs
- ProvidePropertyAttribute.cs
- ScriptResourceAttribute.cs
- NavigationFailedEventArgs.cs
- ProtocolsConfigurationEntry.cs
- XmlDictionaryReader.cs
- CollectionViewProxy.cs
- ChtmlSelectionListAdapter.cs
- IdnMapping.cs
- DependencyObjectType.cs
- CapacityStreamGeometryContext.cs
- FrameworkTemplate.cs
- ThreadStartException.cs
- EventLogPermissionEntry.cs
- Expression.DebuggerProxy.cs
- HwndSourceKeyboardInputSite.cs
- XsdValidatingReader.cs
- SeparatorAutomationPeer.cs
- EntityDataSourceSelectedEventArgs.cs
- SettingsBindableAttribute.cs
- PenLineCapValidation.cs
- ResXBuildProvider.cs
- IndentedTextWriter.cs
- CodeLabeledStatement.cs
- DataGridTextBoxColumn.cs
- KeysConverter.cs
- Subtree.cs
- PrintController.cs
- RuleSettings.cs
- CodeComment.cs
- UICuesEvent.cs
- Point4D.cs
- ChtmlFormAdapter.cs
- RouteData.cs
- PaperSize.cs
- TrackingDataItem.cs
- Nodes.cs
- AddInBase.cs
- HtmlHead.cs
- UserNameSecurityToken.cs
- ListControl.cs
- DateRangeEvent.cs
- DATA_BLOB.cs
- UnsafeNetInfoNativeMethods.cs
- RegexWorker.cs
- CapiNative.cs
- SocketCache.cs
- XappLauncher.cs
- ContextMenu.cs
- Serializer.cs
- odbcmetadatacolumnnames.cs
- EmbeddedMailObject.cs
- FixedDocument.cs
- EnumDataContract.cs
- HttpContext.cs
- IndentedWriter.cs
- DataGridClipboardCellContent.cs
- MatchAttribute.cs
- SqlDataSourceEnumerator.cs
- EndEvent.cs
- SimpleTableProvider.cs
- HtmlWindow.cs
- Track.cs
- ToolStripItemTextRenderEventArgs.cs
- _DomainName.cs
- UrlParameterWriter.cs
- DbLambda.cs
- ManagedIStream.cs
- TraceUtils.cs
- JapaneseCalendar.cs
- StandardBindingElementCollection.cs
- HitTestResult.cs
- DocumentStatusResources.cs
- PropertyGeneratedEventArgs.cs
- _ContextAwareResult.cs
- DataGridViewRowCollection.cs
- DataListItemCollection.cs
- ZipIOExtraFieldPaddingElement.cs
- Geometry3D.cs