Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / DataTemplate.cs / 2 / DataTemplate.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataTemplate describes how to display a single data item. // // Specs: http://avalon/coreui/Specs%20%20Property%20Engine/Styling%20Revisited.doc // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Controls; using System.Windows.Markup; namespace System.Windows { ////// DataTemplate describes how to display a single data item. /// [DictionaryKeyProperty("DataTemplateKey")] public class DataTemplate : FrameworkTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// DataTemplate Constructor /// public DataTemplate() { } ////// DataTemplate Constructor /// public DataTemplate(object dataType) { Exception ex = TemplateKey.ValidateDataType(dataType, "dataType"); if (ex != null) throw ex; _dataType = dataType; } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- ////// DataType for this DataTemplate. If the template is intended /// for object data, this is the Type of the data. If the /// template is intended for XML data, this is the tag name /// of the data (i.e. a string). /// [DefaultValue(null)] public object DataType { get { return _dataType; } set { Exception ex = TemplateKey.ValidateDataType(value, "value"); if (ex != null) throw ex; CheckSealed(); _dataType = value; } } ////// Collection of Triggers /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] [DependsOn("VisualTree")] public TriggerCollection Triggers { get { if (_triggers == null) { _triggers = new TriggerCollection(); // If the template has been sealed prior to this the newly // created TriggerCollection also needs to be sealed if (IsSealed) { _triggers.Seal(); } } return _triggers; } } ////// The key that will be used if the DataTemplate is added to a /// ResourceDictionary in Xaml without a specified Key (x:Key). /// public object DataTemplateKey { get { return (DataType != null) ? new DataTemplateKey(DataType) : null; } } #endregion PublicProperties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- // // TargetType for DataTemplate. This is override is // so FrameworkTemplate can see this property. // internal override Type TargetTypeInternal { get { return DefaultTargetType; } } // Subclasses must provide a way for the parser to directly set the // target type. For DataTemplate, this is not allowed. internal override void SetTargetTypeInternal(Type targetType) { throw new InvalidOperationException(SR.Get(SRID.TemplateNotTargetType)); } // // DataType for DataTemplate. This is override is // so FrameworkTemplate can see this property. // internal override object DataTypeInternal { get { return DataType; } } // // Collection of Triggers for a DataTemplate. This is // override is so FrameworkTemplate can see this property. // internal override TriggerCollection TriggersInternal { get { return Triggers; } } // Target type of DataTrigger is ContentPresenter static internal Type DefaultTargetType { get { return typeof(ContentPresenter); } } #endregion Internal Properties #region Protected Methods //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- ////// Validate against the following rules /// 1. Must have a non-null feTemplatedParent /// 2. A DataTemplate must be applied to a ContentPresenter /// protected override void ValidateTemplatedParent(FrameworkElement templatedParent) { // Must have a non-null feTemplatedParent if (templatedParent == null) { throw new ArgumentNullException("templatedParent"); } // A DataTemplate must be applied to a ContentPresenter if (!(templatedParent is ContentPresenter)) { throw new ArgumentException(SR.Get(SRID.TemplateTargetTypeMismatch, "ContentPresenter", templatedParent.GetType().Name)); } } #endregion Protected Methods #region Data private object _dataType; private TriggerCollection _triggers; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataTemplate describes how to display a single data item. // // Specs: http://avalon/coreui/Specs%20%20Property%20Engine/Styling%20Revisited.doc // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Controls; using System.Windows.Markup; namespace System.Windows { ////// DataTemplate describes how to display a single data item. /// [DictionaryKeyProperty("DataTemplateKey")] public class DataTemplate : FrameworkTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// DataTemplate Constructor /// public DataTemplate() { } ////// DataTemplate Constructor /// public DataTemplate(object dataType) { Exception ex = TemplateKey.ValidateDataType(dataType, "dataType"); if (ex != null) throw ex; _dataType = dataType; } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- ////// DataType for this DataTemplate. If the template is intended /// for object data, this is the Type of the data. If the /// template is intended for XML data, this is the tag name /// of the data (i.e. a string). /// [DefaultValue(null)] public object DataType { get { return _dataType; } set { Exception ex = TemplateKey.ValidateDataType(value, "value"); if (ex != null) throw ex; CheckSealed(); _dataType = value; } } ////// Collection of Triggers /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] [DependsOn("VisualTree")] public TriggerCollection Triggers { get { if (_triggers == null) { _triggers = new TriggerCollection(); // If the template has been sealed prior to this the newly // created TriggerCollection also needs to be sealed if (IsSealed) { _triggers.Seal(); } } return _triggers; } } ////// The key that will be used if the DataTemplate is added to a /// ResourceDictionary in Xaml without a specified Key (x:Key). /// public object DataTemplateKey { get { return (DataType != null) ? new DataTemplateKey(DataType) : null; } } #endregion PublicProperties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- // // TargetType for DataTemplate. This is override is // so FrameworkTemplate can see this property. // internal override Type TargetTypeInternal { get { return DefaultTargetType; } } // Subclasses must provide a way for the parser to directly set the // target type. For DataTemplate, this is not allowed. internal override void SetTargetTypeInternal(Type targetType) { throw new InvalidOperationException(SR.Get(SRID.TemplateNotTargetType)); } // // DataType for DataTemplate. This is override is // so FrameworkTemplate can see this property. // internal override object DataTypeInternal { get { return DataType; } } // // Collection of Triggers for a DataTemplate. This is // override is so FrameworkTemplate can see this property. // internal override TriggerCollection TriggersInternal { get { return Triggers; } } // Target type of DataTrigger is ContentPresenter static internal Type DefaultTargetType { get { return typeof(ContentPresenter); } } #endregion Internal Properties #region Protected Methods //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- ////// Validate against the following rules /// 1. Must have a non-null feTemplatedParent /// 2. A DataTemplate must be applied to a ContentPresenter /// protected override void ValidateTemplatedParent(FrameworkElement templatedParent) { // Must have a non-null feTemplatedParent if (templatedParent == null) { throw new ArgumentNullException("templatedParent"); } // A DataTemplate must be applied to a ContentPresenter if (!(templatedParent is ContentPresenter)) { throw new ArgumentException(SR.Get(SRID.TemplateTargetTypeMismatch, "ContentPresenter", templatedParent.GetType().Name)); } } #endregion Protected Methods #region Data private object _dataType; private TriggerCollection _triggers; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
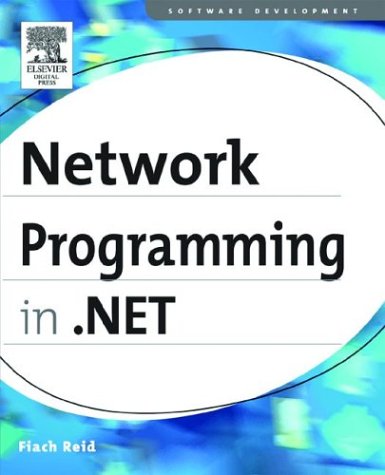
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Intellisense.cs
- ProgressBar.cs
- XmlWrappingWriter.cs
- EmbeddedMailObjectsCollection.cs
- MemberInitExpression.cs
- LeaseManager.cs
- TrackingStringDictionary.cs
- BinaryCommonClasses.cs
- ProcessModule.cs
- XmlSchemaImporter.cs
- CodeSubDirectoriesCollection.cs
- StreamGeometry.cs
- XPathDocumentIterator.cs
- httpserverutility.cs
- OleAutBinder.cs
- InvariantComparer.cs
- VirtualPathUtility.cs
- TagMapCollection.cs
- ToolboxDataAttribute.cs
- ToolBarTray.cs
- XsltException.cs
- SystemInfo.cs
- _ListenerRequestStream.cs
- EntityRecordInfo.cs
- _SslSessionsCache.cs
- InputLanguage.cs
- InkCanvasFeedbackAdorner.cs
- SchemaHelper.cs
- MultiplexingDispatchMessageFormatter.cs
- DataGridSortCommandEventArgs.cs
- LazyTextWriterCreator.cs
- Assert.cs
- SpotLight.cs
- FrameDimension.cs
- InputLanguageProfileNotifySink.cs
- ColorTranslator.cs
- LinqDataSourceStatusEventArgs.cs
- ProcessStartInfo.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- XmlHelper.cs
- MethodMessage.cs
- BooleanExpr.cs
- SudsWriter.cs
- Type.cs
- COAUTHINFO.cs
- XmlSchemaObjectCollection.cs
- Section.cs
- SafeCryptoHandles.cs
- SqlCommandSet.cs
- FactoryRecord.cs
- ValueQuery.cs
- OutputChannel.cs
- TransactionOptions.cs
- SystemIPInterfaceStatistics.cs
- CodeSubDirectory.cs
- Composition.cs
- CroppedBitmap.cs
- Atom10FormatterFactory.cs
- InkCanvasFeedbackAdorner.cs
- Vector3dCollection.cs
- ParagraphVisual.cs
- DateTimeConverter.cs
- RadioButton.cs
- RealizationDrawingContextWalker.cs
- CategoryGridEntry.cs
- ValueType.cs
- DataBoundControl.cs
- IPAddress.cs
- EntityContainerEmitter.cs
- MouseGestureValueSerializer.cs
- StringUtil.cs
- NativeMethods.cs
- OciEnlistContext.cs
- Effect.cs
- Choices.cs
- InvalidPipelineStoreException.cs
- VoiceObjectToken.cs
- DependencyPropertyChangedEventArgs.cs
- EdmSchemaAttribute.cs
- CurrentChangedEventManager.cs
- DescendentsWalker.cs
- Object.cs
- GACMembershipCondition.cs
- PropertyValueUIItem.cs
- FtpWebRequest.cs
- messageonlyhwndwrapper.cs
- ClosureBinding.cs
- NotifyParentPropertyAttribute.cs
- XmlSchemaSubstitutionGroup.cs
- login.cs
- RecordsAffectedEventArgs.cs
- WindowsEditBox.cs
- DesignTimeVisibleAttribute.cs
- Types.cs
- __ConsoleStream.cs
- LinqDataSourceDisposeEventArgs.cs
- EditBehavior.cs
- CompModSwitches.cs
- PackageDigitalSignature.cs
- Message.cs