Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / SafeCryptoHandles.cs / 1305376 / SafeCryptoHandles.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // SafeCryptoHandles.cs // namespace System.Security.Cryptography { using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.Versioning; using Microsoft.Win32.SafeHandles; ////// Safe handle representing a mscorwks!CRYPT_PROV_CTX /// ////// Since we need sometimes to delete the key container created in the context of the CSP, the handle /// used in this class is actually a pointer to a CRYPT_PROV_CTX unmanaged structure defined in /// COMCryptography.h /// [System.Security.SecurityCritical] // auto-generated internal sealed class SafeProvHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeProvHandle() : base(true) { SetHandle(IntPtr.Zero); } private SafeProvHandle(IntPtr handle) : base (true) { SetHandle(handle); } internal static SafeProvHandle InvalidHandle { get { return new SafeProvHandle(); } } [DllImport(JitHelpers.QCall, CharSet = CharSet.Unicode)] [ResourceExposure(ResourceScope.None)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern void FreeCsp(IntPtr pProviderContext); [System.Security.SecurityCritical] protected override bool ReleaseHandle() { FreeCsp(handle); return true; } } #if FEATURE_CRYPTO ////// Safe handle representing a mscorkws!CRYPT_KEY_CTX /// ////// Since we need to delete the key handle before the provider is released we need to actually hold a /// pointer to a CRYPT_KEY_CTX unmanaged structure whose destructor decrements a refCount. Only when /// the provider refCount is 0 it is deleted. This way, we loose a ---- in the critical finalization /// of the key handle and provider handle. This also applies to hash handles, which point to a /// CRYPT_HASH_CTX. Those strucutres are defined in COMCryptography.h /// [System.Security.SecurityCritical] // auto-generated internal sealed class SafeKeyHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeKeyHandle() : base(true) { SetHandle(IntPtr.Zero); } private SafeKeyHandle(IntPtr handle) : base (true) { SetHandle(handle); } internal static SafeKeyHandle InvalidHandle { get { return new SafeKeyHandle(); } } [DllImport(JitHelpers.QCall, CharSet = CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] [SuppressUnmanagedCodeSecurity] private static extern void FreeKey(IntPtr pKeyCotext); [System.Security.SecurityCritical] protected override bool ReleaseHandle() { FreeKey(handle); return true; } } ////// SafeHandle representing a mscorwks!CRYPT_HASH_CTX /// ////// See code:System.Security.Cryptography.SafeKeyHandle for information about the release process /// for a CRYPT_HASH_CTX. /// [System.Security.SecurityCritical] // auto-generated internal sealed class SafeHashHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeHashHandle() : base(true) { SetHandle(IntPtr.Zero); } private SafeHashHandle(IntPtr handle) : base (true) { SetHandle(handle); } internal static SafeHashHandle InvalidHandle { get { return new SafeHashHandle(); } } [DllImport(JitHelpers.QCall, CharSet = CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [ResourceExposure(ResourceScope.None)] [SuppressUnmanagedCodeSecurity] private static extern void FreeHash(IntPtr pHashContext); [System.Security.SecurityCritical] protected override bool ReleaseHandle() { FreeHash(handle); return true; } } #endif // #if FEATURE_CRYPTO } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
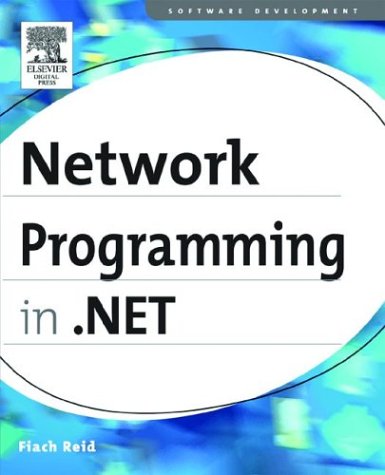
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PerformanceCounterPermission.cs
- Context.cs
- MetadataConversionError.cs
- ActiveXHelper.cs
- InstancePersistence.cs
- CharConverter.cs
- DebuggerAttributes.cs
- AdjustableArrowCap.cs
- EnumValidator.cs
- Matrix.cs
- HtmlControl.cs
- Stroke.cs
- ExceptionList.cs
- DesigntimeLicenseContext.cs
- XmlDocumentFragment.cs
- IPAddressCollection.cs
- CalendarDayButton.cs
- DbConnectionHelper.cs
- Stackframe.cs
- AssemblyNameUtility.cs
- XmlSerializationWriter.cs
- MessageQueueTransaction.cs
- Update.cs
- FixedDSBuilder.cs
- DataSourceControl.cs
- DefaultMemberAttribute.cs
- Slider.cs
- KnownTypeHelper.cs
- RoutedEvent.cs
- PriorityBindingExpression.cs
- xml.cs
- XMLSyntaxException.cs
- LockCookie.cs
- AppearanceEditorPart.cs
- WebServiceData.cs
- DataGridCell.cs
- PrimaryKeyTypeConverter.cs
- Message.cs
- MemoryRecordBuffer.cs
- BooleanProjectedSlot.cs
- RC2.cs
- XmlSchemaCollection.cs
- AccessedThroughPropertyAttribute.cs
- AutoGeneratedField.cs
- SystemPens.cs
- SocketPermission.cs
- TypeExtensionConverter.cs
- MetadataPropertyAttribute.cs
- VisualBasicSettingsHandler.cs
- ControlUtil.cs
- SpellerError.cs
- SelectionList.cs
- XmlNamespaceMapping.cs
- xml.cs
- URLAttribute.cs
- FloaterParaClient.cs
- SQLDecimalStorage.cs
- RuleSettings.cs
- AutoScrollHelper.cs
- DataObject.cs
- Color.cs
- ProjectionCamera.cs
- ServiceProviders.cs
- _BufferOffsetSize.cs
- DataGridViewButtonColumn.cs
- StoryFragments.cs
- XmlExpressionDumper.cs
- ReliabilityContractAttribute.cs
- CharEnumerator.cs
- VisualBrush.cs
- MembershipSection.cs
- OleDbInfoMessageEvent.cs
- ViewBase.cs
- BlockUIContainer.cs
- ContractAdapter.cs
- EntityContainerRelationshipSetEnd.cs
- TextFormatterContext.cs
- SingleStorage.cs
- Encoding.cs
- XPathSelectionIterator.cs
- activationcontext.cs
- SpellerHighlightLayer.cs
- mediapermission.cs
- ContentType.cs
- panel.cs
- EntityDataSource.cs
- AsyncInvokeOperation.cs
- CodeAttributeDeclarationCollection.cs
- CellParagraph.cs
- SecurityTokenRequirement.cs
- RadioButtonFlatAdapter.cs
- CodeSnippetExpression.cs
- PropertyMapper.cs
- SoapSchemaImporter.cs
- DesignerSelectionListAdapter.cs
- NodeInfo.cs
- ToolStripProgressBar.cs
- PersonalizationProviderCollection.cs
- ThreadStateException.cs
- XPathMessageFilter.cs