Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Advanced / AdjustableArrowCap.cs / 2 / AdjustableArrowCap.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; ////// /// Represents an adjustable arrow-shaped line /// cap. /// public sealed class AdjustableArrowCap : CustomLineCap { internal AdjustableArrowCap(IntPtr nativeCap) : base(nativeCap) {} ////// /// Initializes a new instance of the public AdjustableArrowCap(float width, float height) : this(width, height, true) {} ///class with the specified width and /// height. /// /// /// public AdjustableArrowCap(float width, float height, bool isFilled) { IntPtr nativeCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateAdjustableArrowCap( height, width, isFilled, out nativeCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeLineCap(nativeCap); } private void _SetHeight(float height) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapHeight(new HandleRef(this, nativeCap), height); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetHeight() { float height; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapHeight(new HandleRef(this, nativeCap), out height); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return height; } ////// Initializes a new instance of the ///class with the specified width, /// height, and fill property. /// /// /// Gets or sets the height of the arrow cap. /// public float Height { get { return _GetHeight(); } set { _SetHeight(value); } } private void _SetWidth(float width) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapWidth(new HandleRef(this, nativeCap), width); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetWidth() { float width; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapWidth(new HandleRef(this, nativeCap), out width); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return width; } ////// /// Gets or sets the width of the arrow cap. /// public float Width { get { return _GetWidth(); } set { _SetWidth(value); } } private void _SetMiddleInset(float middleInset) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapMiddleInset(new HandleRef(this, nativeCap), middleInset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetMiddleInset() { float middleInset; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapMiddleInset(new HandleRef(this, nativeCap), out middleInset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return middleInset; } ////// /// public float MiddleInset { get { return _GetMiddleInset(); } set { _SetMiddleInset(value); } } private void _SetFillState(bool isFilled) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapFillState(new HandleRef(this, nativeCap), isFilled); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private bool _IsFilled() { bool isFilled = false; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapFillState(new HandleRef(this, nativeCap), out isFilled); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isFilled; } ////// Gets or set the number of pixels between the outline of the arrow cap and the fill. /// ////// /// Gets or sets a value indicating whether the /// arrow cap is filled. /// public bool Filled { get { return _IsFilled(); } set { _SetFillState(value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; ////// /// Represents an adjustable arrow-shaped line /// cap. /// public sealed class AdjustableArrowCap : CustomLineCap { internal AdjustableArrowCap(IntPtr nativeCap) : base(nativeCap) {} ////// /// Initializes a new instance of the public AdjustableArrowCap(float width, float height) : this(width, height, true) {} ///class with the specified width and /// height. /// /// /// public AdjustableArrowCap(float width, float height, bool isFilled) { IntPtr nativeCap = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateAdjustableArrowCap( height, width, isFilled, out nativeCap); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeLineCap(nativeCap); } private void _SetHeight(float height) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapHeight(new HandleRef(this, nativeCap), height); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetHeight() { float height; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapHeight(new HandleRef(this, nativeCap), out height); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return height; } ////// Initializes a new instance of the ///class with the specified width, /// height, and fill property. /// /// /// Gets or sets the height of the arrow cap. /// public float Height { get { return _GetHeight(); } set { _SetHeight(value); } } private void _SetWidth(float width) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapWidth(new HandleRef(this, nativeCap), width); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetWidth() { float width; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapWidth(new HandleRef(this, nativeCap), out width); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return width; } ////// /// Gets or sets the width of the arrow cap. /// public float Width { get { return _GetWidth(); } set { _SetWidth(value); } } private void _SetMiddleInset(float middleInset) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapMiddleInset(new HandleRef(this, nativeCap), middleInset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private float _GetMiddleInset() { float middleInset; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapMiddleInset(new HandleRef(this, nativeCap), out middleInset); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return middleInset; } ////// /// public float MiddleInset { get { return _GetMiddleInset(); } set { _SetMiddleInset(value); } } private void _SetFillState(bool isFilled) { int status = SafeNativeMethods.Gdip.GdipSetAdjustableArrowCapFillState(new HandleRef(this, nativeCap), isFilled); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } private bool _IsFilled() { bool isFilled = false; int status = SafeNativeMethods.Gdip.GdipGetAdjustableArrowCapFillState(new HandleRef(this, nativeCap), out isFilled); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isFilled; } ////// Gets or set the number of pixels between the outline of the arrow cap and the fill. /// ////// /// Gets or sets a value indicating whether the /// arrow cap is filled. /// public bool Filled { get { return _IsFilled(); } set { _SetFillState(value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
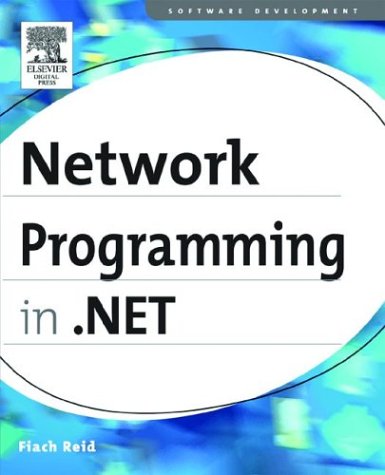
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- File.cs
- Style.cs
- EventData.cs
- LockedActivityGlyph.cs
- OdbcConnectionOpen.cs
- SiteMapNodeCollection.cs
- TextAction.cs
- ScrollContentPresenter.cs
- ColorComboBox.cs
- FunctionNode.cs
- EmptyEnumerator.cs
- DateTimeAutomationPeer.cs
- RegistryDataKey.cs
- AudioStateChangedEventArgs.cs
- BitmapDecoder.cs
- XamlTreeBuilderBamlRecordWriter.cs
- List.cs
- PolicyException.cs
- AnnotationHighlightLayer.cs
- CompilationLock.cs
- KeyMatchBuilder.cs
- TableRowGroup.cs
- ConfigurationValue.cs
- ConfigurationStrings.cs
- EntityDataSourceWizardForm.cs
- EntityContainerEmitter.cs
- MessageQueueKey.cs
- WebPartsSection.cs
- CompilationPass2Task.cs
- ConvertersCollection.cs
- DrawToolTipEventArgs.cs
- BuilderPropertyEntry.cs
- IdentityReference.cs
- Visual3DCollection.cs
- WsdlBuildProvider.cs
- LayoutInformation.cs
- XamlTreeBuilderBamlRecordWriter.cs
- Vars.cs
- SiteMapPathDesigner.cs
- Rules.cs
- PathGeometry.cs
- WSHttpSecurity.cs
- GlobalProxySelection.cs
- WhileDesigner.cs
- AppDomain.cs
- ObjectConverter.cs
- ResourcesBuildProvider.cs
- BitmapVisualManager.cs
- LOSFormatter.cs
- StateDesigner.CommentLayoutGlyph.cs
- DataServiceRequest.cs
- Pen.cs
- RowUpdatingEventArgs.cs
- NumberFunctions.cs
- COM2IDispatchConverter.cs
- ResourceContainer.cs
- TdsParserStateObject.cs
- IncomingWebRequestContext.cs
- Size.cs
- LocalizationParserHooks.cs
- LockCookie.cs
- FixedSOMLineCollection.cs
- TdsRecordBufferSetter.cs
- BoolLiteral.cs
- VisualStyleRenderer.cs
- XmlMessageFormatter.cs
- DataGridViewCellCancelEventArgs.cs
- SqlClientMetaDataCollectionNames.cs
- StyleSelector.cs
- HelpFileFileNameEditor.cs
- WebPartZone.cs
- BuildProviderAppliesToAttribute.cs
- IndentedWriter.cs
- ListViewItemSelectionChangedEvent.cs
- ConfigurationSection.cs
- WebRequestModulesSection.cs
- CapacityStreamGeometryContext.cs
- regiisutil.cs
- CommandBindingCollection.cs
- SelectionPattern.cs
- DataControlReferenceCollection.cs
- ReadOnlyHierarchicalDataSource.cs
- Rotation3DAnimation.cs
- SamlAssertion.cs
- COSERVERINFO.cs
- ApplicationActivator.cs
- RichTextBoxConstants.cs
- JournalEntryStack.cs
- Evidence.cs
- OleDbConnectionFactory.cs
- DataStreamFromComStream.cs
- Missing.cs
- CounterSetInstance.cs
- HttpGetClientProtocol.cs
- MarshalByValueComponent.cs
- RedirectionProxy.cs
- PlaceHolder.cs
- ObjectManager.cs
- DateTimeConstantAttribute.cs