Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / FixedSOMLineCollection.cs / 1 / FixedSOMLineCollection.cs
/*++ File: FixedSOMLineCollection.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: Internal helper class that can store a set of sorted horizontal and vertical FixedSOMLineRanges. These ranges are used in construction of FixedBlocks and Tables History: 05/17/2005: agurcan - Created --*/ namespace System.Windows.Documents { using System.Collections.Generic; using System.Windows.Shapes; using System.Windows.Media; using System.Diagnostics; using System.Windows; //Stores a collection of horizontal and vertical lines sorted by y and x axis respectively // internal sealed class FixedSOMLineCollection { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors public FixedSOMLineCollection() { _verticals = new List(); _horizontals = new List (); } #endregion Constructors //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Public Methods public bool IsVerticallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_verticals, left, top, right, bottom); } public bool IsHorizontallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_horizontals, top, left, bottom, right); } public void AddVertical(Point point1, Point point2) { Debug.Assert(point1.X == point2.X); _AddLineToRanges(_verticals, point1.X, point1.Y, point2.Y); } public void AddHorizontal(Point point1, Point point2) { Debug.Assert(point1.Y == point2.Y); _AddLineToRanges(_horizontals, point1.Y, point1.X, point2.X); } #endregion Public Methods #region Private Methods //Merge line 2 into line 1 private void _AddLineToRanges(List ranges, double line, double start, double end) { if (start > end) { double temp = start; start = end; end = temp; } FixedSOMLineRanges range; double maxSeparation = .5 * FixedSOMLineRanges.MinLineSeparation; for (int i=0; i < ranges.Count; i++) { if (line < ranges[i].Line - maxSeparation) { range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Insert(i, range); return; } else if (line < ranges[i].Line + maxSeparation) { ranges[i].AddRange(start, end); return; } } // add to end range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Add(range); return; } //Generic function that decides whether or not a rectangle as spefied by the points is //divided by any of the lines in the line ranges in the passed in list private bool _IsSeparated(List lines, double parallelLowEnd, double perpLowEnd, double parallelHighEnd, double perpHighEnd) { int startIndex = 0; int endIndex = lines.Count; if (endIndex == 0) { return false; } int i = 0; while (endIndex > startIndex) { i = (startIndex + endIndex) >> 1; if (lines[i].Line < parallelLowEnd) { startIndex = i + 1; } else { if (lines[i].Line <= parallelHighEnd) { break; } endIndex = i; } } if (lines[i].Line >= parallelLowEnd && lines[i].Line <= parallelHighEnd) { do { i--; } while (i>=0 && lines[i].Line >= parallelLowEnd); i++; while (i =0) { double end = lines[i].End[rangeIndex]; if (end >= perpHighEnd - allowedMargin) { return true; } } i++; }; } return false; } #endregion Private Methods #region Public Properties public List HorizontalLines { get { return _horizontals; } } public List VerticalLines { get { return _verticals; } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private List _horizontals; private List _verticals; private const double _fudgeFactor = 0.1; // We allow 10% margin at each end #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /*++ File: FixedSOMLineCollection.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: Internal helper class that can store a set of sorted horizontal and vertical FixedSOMLineRanges. These ranges are used in construction of FixedBlocks and Tables History: 05/17/2005: agurcan - Created --*/ namespace System.Windows.Documents { using System.Collections.Generic; using System.Windows.Shapes; using System.Windows.Media; using System.Diagnostics; using System.Windows; //Stores a collection of horizontal and vertical lines sorted by y and x axis respectively // internal sealed class FixedSOMLineCollection { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors public FixedSOMLineCollection() { _verticals = new List (); _horizontals = new List (); } #endregion Constructors //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Public Methods public bool IsVerticallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_verticals, left, top, right, bottom); } public bool IsHorizontallySeparated(double left, double top, double right, double bottom) { return _IsSeparated(_horizontals, top, left, bottom, right); } public void AddVertical(Point point1, Point point2) { Debug.Assert(point1.X == point2.X); _AddLineToRanges(_verticals, point1.X, point1.Y, point2.Y); } public void AddHorizontal(Point point1, Point point2) { Debug.Assert(point1.Y == point2.Y); _AddLineToRanges(_horizontals, point1.Y, point1.X, point2.X); } #endregion Public Methods #region Private Methods //Merge line 2 into line 1 private void _AddLineToRanges(List ranges, double line, double start, double end) { if (start > end) { double temp = start; start = end; end = temp; } FixedSOMLineRanges range; double maxSeparation = .5 * FixedSOMLineRanges.MinLineSeparation; for (int i=0; i < ranges.Count; i++) { if (line < ranges[i].Line - maxSeparation) { range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Insert(i, range); return; } else if (line < ranges[i].Line + maxSeparation) { ranges[i].AddRange(start, end); return; } } // add to end range = new FixedSOMLineRanges(); range.Line = line; range.AddRange(start, end); ranges.Add(range); return; } //Generic function that decides whether or not a rectangle as spefied by the points is //divided by any of the lines in the line ranges in the passed in list private bool _IsSeparated(List lines, double parallelLowEnd, double perpLowEnd, double parallelHighEnd, double perpHighEnd) { int startIndex = 0; int endIndex = lines.Count; if (endIndex == 0) { return false; } int i = 0; while (endIndex > startIndex) { i = (startIndex + endIndex) >> 1; if (lines[i].Line < parallelLowEnd) { startIndex = i + 1; } else { if (lines[i].Line <= parallelHighEnd) { break; } endIndex = i; } } if (lines[i].Line >= parallelLowEnd && lines[i].Line <= parallelHighEnd) { do { i--; } while (i>=0 && lines[i].Line >= parallelLowEnd); i++; while (i =0) { double end = lines[i].End[rangeIndex]; if (end >= perpHighEnd - allowedMargin) { return true; } } i++; }; } return false; } #endregion Private Methods #region Public Properties public List HorizontalLines { get { return _horizontals; } } public List VerticalLines { get { return _verticals; } } #endregion Public Properties //-------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private List _horizontals; private List _verticals; private const double _fudgeFactor = 0.1; // We allow 10% margin at each end #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
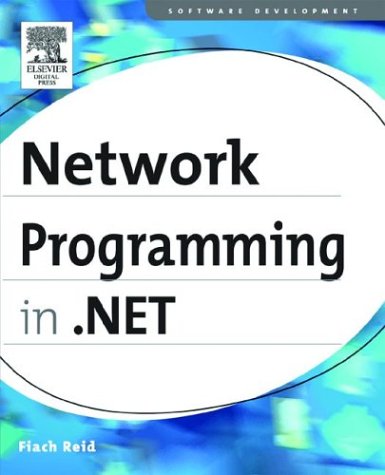
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Convert.cs
- DmlSqlGenerator.cs
- XmlDomTextWriter.cs
- TextSpanModifier.cs
- MetadataFile.cs
- EventLogPermissionEntryCollection.cs
- HttpHandlerAction.cs
- SecurityHeaderTokenResolver.cs
- FacetValues.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- XmlBindingWorker.cs
- TableRow.cs
- Debug.cs
- Simplifier.cs
- DoubleLink.cs
- SuppressMergeCheckAttribute.cs
- Util.cs
- HttpModuleAction.cs
- TrackingStringDictionary.cs
- SmiEventStream.cs
- OutputScopeManager.cs
- FirstMatchCodeGroup.cs
- TreeNodeSelectionProcessor.cs
- TemplateLookupAction.cs
- HostExecutionContextManager.cs
- DefaultHttpHandler.cs
- MSAAEventDispatcher.cs
- ToolStripDropTargetManager.cs
- XmlElementAttribute.cs
- XomlCompilerParameters.cs
- HttpClientCertificate.cs
- DataGridViewAccessibleObject.cs
- FlowDocument.cs
- precedingquery.cs
- CompModSwitches.cs
- EnumDataContract.cs
- PartialCachingControl.cs
- DependencyPropertyAttribute.cs
- CryptoApi.cs
- Rijndael.cs
- SignedInfo.cs
- QueueProcessor.cs
- DiscoveryOperationContext.cs
- SqlDataReader.cs
- CounterCreationDataCollection.cs
- FontStyle.cs
- SecurityUtils.cs
- CachedPathData.cs
- filewebresponse.cs
- ObjectListFieldCollection.cs
- StoreItemCollection.Loader.cs
- Pair.cs
- ToolboxCategoryItems.cs
- InstanceDataCollection.cs
- OdbcParameterCollection.cs
- RequestTimeoutManager.cs
- SystemWebCachingSectionGroup.cs
- StrongNameUtility.cs
- TreeViewImageIndexConverter.cs
- MultiTrigger.cs
- Application.cs
- MarkupCompilePass2.cs
- LoggedException.cs
- HttpPostedFile.cs
- MailAddressCollection.cs
- Journaling.cs
- Win32SafeHandles.cs
- RestHandler.cs
- ToolStripRendererSwitcher.cs
- TreeNodeMouseHoverEvent.cs
- ZipIORawDataFileBlock.cs
- UrlMappingCollection.cs
- Utils.cs
- CodeConstructor.cs
- DESCryptoServiceProvider.cs
- DelegateTypeInfo.cs
- AccessorTable.cs
- SqlRetyper.cs
- NotCondition.cs
- IOException.cs
- SortQuery.cs
- DataGridViewRowCancelEventArgs.cs
- DbSetClause.cs
- NativeMethods.cs
- SByteConverter.cs
- SqlDataSource.cs
- RecordsAffectedEventArgs.cs
- WorkflowRuntimeEndpoint.cs
- Wrapper.cs
- Wizard.cs
- BuildResult.cs
- AxisAngleRotation3D.cs
- TraceHandler.cs
- Utils.cs
- OpCopier.cs
- BezierSegment.cs
- PackageDigitalSignature.cs
- EmptyElement.cs
- IsolatedStorageFilePermission.cs
- SecurityContext.cs