Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / filewebresponse.cs / 1 / filewebresponse.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Runtime.Serialization; using System.IO; using System.Globalization; using System.Security.Permissions; [Serializable] public class FileWebResponse : WebResponse, ISerializable, ICloseEx { const int DefaultFileStreamBufferSize = 8192; const string DefaultFileContentType = "application/octet-stream"; // fields bool m_closed; long m_contentLength; FileAccess m_fileAccess; WebHeaderCollection m_headers; Stream m_stream; Uri m_uri; // constructors internal FileWebResponse(FileWebRequest request, Uri uri, FileAccess access, bool asyncHint) { GlobalLog.Enter("FileWebResponse::FileWebResponse", "uri="+uri+", access="+access+", asyncHint="+asyncHint); try { m_fileAccess = access; if (access == FileAccess.Write) { m_stream = Stream.Null; } else { // // apparently, specifying async when the stream will be read // synchronously, or vice versa, can lead to a 10x perf hit. // While we don't know how the app will read the stream, we // use the hint from whether the app called BeginGetResponse // or GetResponse to supply the async flag to the stream ctor // m_stream = new FileWebStream(request, uri.LocalPath, FileMode.Open, FileAccess.Read, FileShare.Read, DefaultFileStreamBufferSize, asyncHint ); m_contentLength = m_stream.Length; } m_headers = new WebHeaderCollection(WebHeaderCollectionType.FileWebResponse); m_headers.AddInternal(HttpKnownHeaderNames.ContentLength, m_contentLength.ToString(NumberFormatInfo.InvariantInfo)); m_headers.AddInternal(HttpKnownHeaderNames.ContentType, DefaultFileContentType); m_uri = uri; } catch (Exception e) { Exception ex = new WebException(e.Message, e, WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } catch { Exception ex = new WebException(string.Empty, new Exception(SR.GetString(SR.net_nonClsCompliantException)), WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } GlobalLog.Leave("FileWebResponse::FileWebResponse"); } // // ISerializable constructor // [Obsolete("Serialization is obsoleted for this type. http://go.microsoft.com/fwlink/?linkid=14202")] protected FileWebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext):base(serializationInfo, streamingContext) { m_headers = (WebHeaderCollection)serializationInfo.GetValue("headers", typeof(WebHeaderCollection)); m_uri = (Uri)serializationInfo.GetValue("uri", typeof(Uri)); m_contentLength = serializationInfo.GetInt64("contentLength"); m_fileAccess = (FileAccess )serializationInfo.GetInt32("fileAccess"); } // // ISerializable method // ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide some way for derived classes to access GetObjectData even if the derived class // explicitly re-inherits ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { serializationInfo.AddValue("headers", m_headers, typeof(WebHeaderCollection)); serializationInfo.AddValue("uri", m_uri, typeof(Uri)); serializationInfo.AddValue("contentLength", m_contentLength); serializationInfo.AddValue("fileAccess", m_fileAccess); base.GetObjectData(serializationInfo, streamingContext); } // properties public override long ContentLength { get { CheckDisposed(); return m_contentLength; } } public override string ContentType { get { CheckDisposed(); return DefaultFileContentType; } } public override WebHeaderCollection Headers { get { CheckDisposed(); return m_headers; } } public override Uri ResponseUri { get { CheckDisposed(); return m_uri; } } // methods private void CheckDisposed() { if (m_closed) { throw new ObjectDisposedException(this.GetType().FullName); } } public override void Close() { ((ICloseEx)this).CloseEx(CloseExState.Normal); } void ICloseEx.CloseEx(CloseExState closeState) { GlobalLog.Enter("FileWebResponse::Close()"); try { if (!m_closed) { m_closed = true; Stream chkStream = m_stream; if (chkStream!=null) { if (chkStream is ICloseEx) ((ICloseEx)chkStream).CloseEx(closeState); else chkStream.Close(); m_stream = null; } } } finally { GlobalLog.Leave("FileWebResponse::Close()"); } } public override Stream GetResponseStream() { GlobalLog.Enter("FileWebResponse::GetResponseStream()"); try { CheckDisposed(); } finally { GlobalLog.Leave("FileWebResponse::GetResponseStream()"); } return m_stream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Runtime.Serialization; using System.IO; using System.Globalization; using System.Security.Permissions; [Serializable] public class FileWebResponse : WebResponse, ISerializable, ICloseEx { const int DefaultFileStreamBufferSize = 8192; const string DefaultFileContentType = "application/octet-stream"; // fields bool m_closed; long m_contentLength; FileAccess m_fileAccess; WebHeaderCollection m_headers; Stream m_stream; Uri m_uri; // constructors internal FileWebResponse(FileWebRequest request, Uri uri, FileAccess access, bool asyncHint) { GlobalLog.Enter("FileWebResponse::FileWebResponse", "uri="+uri+", access="+access+", asyncHint="+asyncHint); try { m_fileAccess = access; if (access == FileAccess.Write) { m_stream = Stream.Null; } else { // // apparently, specifying async when the stream will be read // synchronously, or vice versa, can lead to a 10x perf hit. // While we don't know how the app will read the stream, we // use the hint from whether the app called BeginGetResponse // or GetResponse to supply the async flag to the stream ctor // m_stream = new FileWebStream(request, uri.LocalPath, FileMode.Open, FileAccess.Read, FileShare.Read, DefaultFileStreamBufferSize, asyncHint ); m_contentLength = m_stream.Length; } m_headers = new WebHeaderCollection(WebHeaderCollectionType.FileWebResponse); m_headers.AddInternal(HttpKnownHeaderNames.ContentLength, m_contentLength.ToString(NumberFormatInfo.InvariantInfo)); m_headers.AddInternal(HttpKnownHeaderNames.ContentType, DefaultFileContentType); m_uri = uri; } catch (Exception e) { Exception ex = new WebException(e.Message, e, WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } catch { Exception ex = new WebException(string.Empty, new Exception(SR.GetString(SR.net_nonClsCompliantException)), WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } GlobalLog.Leave("FileWebResponse::FileWebResponse"); } // // ISerializable constructor // [Obsolete("Serialization is obsoleted for this type. http://go.microsoft.com/fwlink/?linkid=14202")] protected FileWebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext):base(serializationInfo, streamingContext) { m_headers = (WebHeaderCollection)serializationInfo.GetValue("headers", typeof(WebHeaderCollection)); m_uri = (Uri)serializationInfo.GetValue("uri", typeof(Uri)); m_contentLength = serializationInfo.GetInt64("contentLength"); m_fileAccess = (FileAccess )serializationInfo.GetInt32("fileAccess"); } // // ISerializable method // ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide some way for derived classes to access GetObjectData even if the derived class // explicitly re-inherits ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { serializationInfo.AddValue("headers", m_headers, typeof(WebHeaderCollection)); serializationInfo.AddValue("uri", m_uri, typeof(Uri)); serializationInfo.AddValue("contentLength", m_contentLength); serializationInfo.AddValue("fileAccess", m_fileAccess); base.GetObjectData(serializationInfo, streamingContext); } // properties public override long ContentLength { get { CheckDisposed(); return m_contentLength; } } public override string ContentType { get { CheckDisposed(); return DefaultFileContentType; } } public override WebHeaderCollection Headers { get { CheckDisposed(); return m_headers; } } public override Uri ResponseUri { get { CheckDisposed(); return m_uri; } } // methods private void CheckDisposed() { if (m_closed) { throw new ObjectDisposedException(this.GetType().FullName); } } public override void Close() { ((ICloseEx)this).CloseEx(CloseExState.Normal); } void ICloseEx.CloseEx(CloseExState closeState) { GlobalLog.Enter("FileWebResponse::Close()"); try { if (!m_closed) { m_closed = true; Stream chkStream = m_stream; if (chkStream!=null) { if (chkStream is ICloseEx) ((ICloseEx)chkStream).CloseEx(closeState); else chkStream.Close(); m_stream = null; } } } finally { GlobalLog.Leave("FileWebResponse::Close()"); } } public override Stream GetResponseStream() { GlobalLog.Enter("FileWebResponse::GetResponseStream()"); try { CheckDisposed(); } finally { GlobalLog.Leave("FileWebResponse::GetResponseStream()"); } return m_stream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
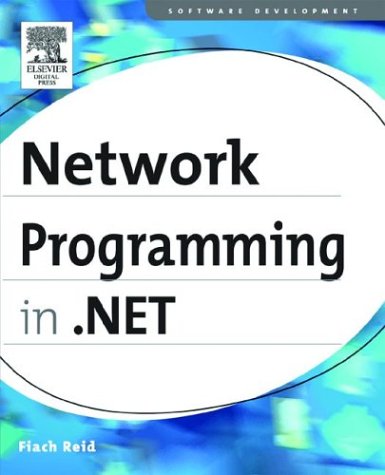
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultParameterValueAttribute.cs
- XmlSchemaValidator.cs
- CreateRefExpr.cs
- WebServiceClientProxyGenerator.cs
- HierarchicalDataTemplate.cs
- UserControlCodeDomTreeGenerator.cs
- WindowsListViewScroll.cs
- PublisherMembershipCondition.cs
- ModifyActivitiesPropertyDescriptor.cs
- GroupItemAutomationPeer.cs
- CallContext.cs
- ExplicitDiscriminatorMap.cs
- WmpBitmapDecoder.cs
- ExpressionConverter.cs
- Tokenizer.cs
- CreateUserWizardStep.cs
- DLinqAssociationProvider.cs
- QuinticEase.cs
- Validator.cs
- Timeline.cs
- TransformerInfo.cs
- Matrix3DStack.cs
- FeatureManager.cs
- BrowserInteropHelper.cs
- WebPartZone.cs
- PropertyDescriptorComparer.cs
- BinaryCommonClasses.cs
- BrowserInteropHelper.cs
- CharacterMetricsDictionary.cs
- DocumentViewerBaseAutomationPeer.cs
- entitydatasourceentitysetnameconverter.cs
- MenuTracker.cs
- DrawListViewItemEventArgs.cs
- DetailsView.cs
- MemoryMappedViewAccessor.cs
- ProcessModelSection.cs
- TextElementEnumerator.cs
- StrokeNodeEnumerator.cs
- BlurBitmapEffect.cs
- BinHexDecoder.cs
- MethodBuilderInstantiation.cs
- ParserOptions.cs
- StylusPointCollection.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- Thread.cs
- PointF.cs
- PerformanceCounterCategory.cs
- DataSourceHelper.cs
- Hash.cs
- ZoneButton.cs
- AsymmetricAlgorithm.cs
- CacheSection.cs
- sqlpipe.cs
- Rules.cs
- RegionData.cs
- XmlAttributes.cs
- HandleExceptionArgs.cs
- ScrollItemPattern.cs
- UpdateProgress.cs
- EditorPartCollection.cs
- ContentFileHelper.cs
- GlyphTypeface.cs
- TitleStyle.cs
- SecurityCriticalDataForSet.cs
- ListViewDeletedEventArgs.cs
- SafeReadContext.cs
- DataKeyCollection.cs
- DefaultValueAttribute.cs
- MemoryMappedViewStream.cs
- ProcessingInstructionAction.cs
- PositiveTimeSpanValidatorAttribute.cs
- cookie.cs
- HtmlAnchor.cs
- LayoutInformation.cs
- BamlTreeUpdater.cs
- SiteMap.cs
- ArraySortHelper.cs
- documentation.cs
- AccessibleObject.cs
- XhtmlBasicFormAdapter.cs
- LinkConverter.cs
- ContainerFilterService.cs
- RawStylusSystemGestureInputReport.cs
- __Filters.cs
- ImageField.cs
- SplineQuaternionKeyFrame.cs
- EntityExpressionVisitor.cs
- NotCondition.cs
- DocumentSchemaValidator.cs
- GridViewDeleteEventArgs.cs
- XmlWrappingWriter.cs
- Rect3D.cs
- AmbientValueAttribute.cs
- SystemFonts.cs
- Query.cs
- DayRenderEvent.cs
- HwndSourceParameters.cs
- FileDialogCustomPlacesCollection.cs
- SequentialOutput.cs
- JulianCalendar.cs