Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / HierarchicalDataTemplate.cs / 1 / HierarchicalDataTemplate.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: HierarchicalDataTemplate adds hierarchy support to DataTemplate. // // Specs: http://avalon/coreui/Specs%20%20Property%20Engine/Styling%20Revisited.doc // //--------------------------------------------------------------------------- using System.Windows.Controls; using System.Windows.Data; namespace System.Windows { ////// HierarchicalDataTemplate adds hierarchy support to DataTemplate. /// public class HierarchicalDataTemplate : DataTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// HierarchicalDataTemplate Constructor /// public HierarchicalDataTemplate() { } ////// HierarchicalDataTemplate Constructor /// public HierarchicalDataTemplate(object dataType) : base(dataType) { } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- ////// ItemsSource binding for this DataTemplate. This is applied /// to the ItemsSource property on a generated HeaderedItemsControl, /// to indicate where to find the collection that represents the /// next level in the data hierarchy. /// public BindingBase ItemsSource { get { return _itemsSourceBinding; } set { CheckSealed(); _itemsSourceBinding = value; } } ////// ItemTemplate for this DataTemplate. This is applied /// to the ItemTemplate property on a generated HeaderedItemsControl, /// to indicate how to display items from the next level in the /// data hierarchy. /// public DataTemplate ItemTemplate { get { return _itemTemplate; } set { CheckSealed(); _itemTemplate = value; _itemTemplateSet = true; } } ////// ItemTemplateSelector for this DataTemplate. This is applied /// to the ItemTemplateSelector property on a generated HeaderedItemsControl, /// to indicate how to select a template to display items from the /// next level in the data hierarchy. /// public DataTemplateSelector ItemTemplateSelector { get { return _itemTemplateSelector; } set { CheckSealed(); _itemTemplateSelector = value; _itemTemplateSelectorSet = true; } } ////// ItemContainerStyle for this DataTemplate. This is applied /// to the ItemContainerStyle property on a generated HeaderedItemsControl, /// to indicate a style for the containers it generates. /// public Style ItemContainerStyle { get { return _itemContainerStyle; } set { CheckSealed(); _itemContainerStyle = value; _itemContainerStyleSet = true; } } ////// ItemContainerStyleSelector for this DataTemplate. This is applied /// to the ItemContainerStyleSelector property on a generated HeaderedItemsControl, /// to indicate how to select a style for the containers it generates. /// public StyleSelector ItemContainerStyleSelector { get { return _itemContainerStyleSelector; } set { CheckSealed(); _itemContainerStyleSelector = value; _itemContainerStyleSelectorSet = true; } } ////// ItemStringFormat for this DataTemplate. This is applied /// to the ItemStringFormat property on a generated HeaderedItemsControl, /// to indicate how to format items from the /// next level in the data hierarchy. /// public string ItemStringFormat { get { return _itemStringFormat; } set { CheckSealed(); _itemStringFormat = value; _itemStringFormatSet = true; } } ////// AlternationCount for this DataTemplate. This is applied /// to the AlternationCount property on a generated HeaderedItemsControl, /// to control the setting of AlternationIndex at the /// next level in the data hierarchy. /// public int AlternationCount { get { return _alternationCount; } set { CheckSealed(); _alternationCount = value; _alternationCountSet = true; } } ////// ItemBindingGroup for this DataTemplate. This is applied /// to the ItemBindingGroup property on a generated HeaderedItemsControl, /// to define the binding group used at the /// next level in the data hierarchy. /// public BindingGroup ItemBindingGroup { get { return _itemBindingGroup; } set { CheckSealed(); _itemBindingGroup = value; _itemBindingGroupSet = true; } } #endregion Public Properties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- internal bool IsItemTemplateSet { get { return _itemTemplateSet; } } internal bool IsItemTemplateSelectorSet { get { return _itemTemplateSelectorSet; } } internal bool IsItemContainerStyleSet { get { return _itemContainerStyleSet; } } internal bool IsItemContainerStyleSelectorSet { get { return _itemContainerStyleSelectorSet; } } internal bool IsItemStringFormatSet { get { return _itemStringFormatSet; } } internal bool IsAlternationCountSet { get { return _alternationCountSet; } } internal bool IsItemBindingGroupSet { get { return _itemBindingGroupSet; } } #endregion Internal Properties #region Data private BindingBase _itemsSourceBinding; private DataTemplate _itemTemplate; private DataTemplateSelector _itemTemplateSelector; private Style _itemContainerStyle; private StyleSelector _itemContainerStyleSelector; private string _itemStringFormat; private int _alternationCount; private BindingGroup _itemBindingGroup; private bool _itemTemplateSet; private bool _itemTemplateSelectorSet; private bool _itemContainerStyleSet; private bool _itemContainerStyleSelectorSet; private bool _itemStringFormatSet; private bool _alternationCountSet; private bool _itemBindingGroupSet; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: HierarchicalDataTemplate adds hierarchy support to DataTemplate. // // Specs: http://avalon/coreui/Specs%20%20Property%20Engine/Styling%20Revisited.doc // //--------------------------------------------------------------------------- using System.Windows.Controls; using System.Windows.Data; namespace System.Windows { ////// HierarchicalDataTemplate adds hierarchy support to DataTemplate. /// public class HierarchicalDataTemplate : DataTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// HierarchicalDataTemplate Constructor /// public HierarchicalDataTemplate() { } ////// HierarchicalDataTemplate Constructor /// public HierarchicalDataTemplate(object dataType) : base(dataType) { } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- ////// ItemsSource binding for this DataTemplate. This is applied /// to the ItemsSource property on a generated HeaderedItemsControl, /// to indicate where to find the collection that represents the /// next level in the data hierarchy. /// public BindingBase ItemsSource { get { return _itemsSourceBinding; } set { CheckSealed(); _itemsSourceBinding = value; } } ////// ItemTemplate for this DataTemplate. This is applied /// to the ItemTemplate property on a generated HeaderedItemsControl, /// to indicate how to display items from the next level in the /// data hierarchy. /// public DataTemplate ItemTemplate { get { return _itemTemplate; } set { CheckSealed(); _itemTemplate = value; _itemTemplateSet = true; } } ////// ItemTemplateSelector for this DataTemplate. This is applied /// to the ItemTemplateSelector property on a generated HeaderedItemsControl, /// to indicate how to select a template to display items from the /// next level in the data hierarchy. /// public DataTemplateSelector ItemTemplateSelector { get { return _itemTemplateSelector; } set { CheckSealed(); _itemTemplateSelector = value; _itemTemplateSelectorSet = true; } } ////// ItemContainerStyle for this DataTemplate. This is applied /// to the ItemContainerStyle property on a generated HeaderedItemsControl, /// to indicate a style for the containers it generates. /// public Style ItemContainerStyle { get { return _itemContainerStyle; } set { CheckSealed(); _itemContainerStyle = value; _itemContainerStyleSet = true; } } ////// ItemContainerStyleSelector for this DataTemplate. This is applied /// to the ItemContainerStyleSelector property on a generated HeaderedItemsControl, /// to indicate how to select a style for the containers it generates. /// public StyleSelector ItemContainerStyleSelector { get { return _itemContainerStyleSelector; } set { CheckSealed(); _itemContainerStyleSelector = value; _itemContainerStyleSelectorSet = true; } } ////// ItemStringFormat for this DataTemplate. This is applied /// to the ItemStringFormat property on a generated HeaderedItemsControl, /// to indicate how to format items from the /// next level in the data hierarchy. /// public string ItemStringFormat { get { return _itemStringFormat; } set { CheckSealed(); _itemStringFormat = value; _itemStringFormatSet = true; } } ////// AlternationCount for this DataTemplate. This is applied /// to the AlternationCount property on a generated HeaderedItemsControl, /// to control the setting of AlternationIndex at the /// next level in the data hierarchy. /// public int AlternationCount { get { return _alternationCount; } set { CheckSealed(); _alternationCount = value; _alternationCountSet = true; } } ////// ItemBindingGroup for this DataTemplate. This is applied /// to the ItemBindingGroup property on a generated HeaderedItemsControl, /// to define the binding group used at the /// next level in the data hierarchy. /// public BindingGroup ItemBindingGroup { get { return _itemBindingGroup; } set { CheckSealed(); _itemBindingGroup = value; _itemBindingGroupSet = true; } } #endregion Public Properties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- internal bool IsItemTemplateSet { get { return _itemTemplateSet; } } internal bool IsItemTemplateSelectorSet { get { return _itemTemplateSelectorSet; } } internal bool IsItemContainerStyleSet { get { return _itemContainerStyleSet; } } internal bool IsItemContainerStyleSelectorSet { get { return _itemContainerStyleSelectorSet; } } internal bool IsItemStringFormatSet { get { return _itemStringFormatSet; } } internal bool IsAlternationCountSet { get { return _alternationCountSet; } } internal bool IsItemBindingGroupSet { get { return _itemBindingGroupSet; } } #endregion Internal Properties #region Data private BindingBase _itemsSourceBinding; private DataTemplate _itemTemplate; private DataTemplateSelector _itemTemplateSelector; private Style _itemContainerStyle; private StyleSelector _itemContainerStyleSelector; private string _itemStringFormat; private int _alternationCount; private BindingGroup _itemBindingGroup; private bool _itemTemplateSet; private bool _itemTemplateSelectorSet; private bool _itemContainerStyleSet; private bool _itemContainerStyleSelectorSet; private bool _itemStringFormatSet; private bool _alternationCountSet; private bool _itemBindingGroupSet; #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
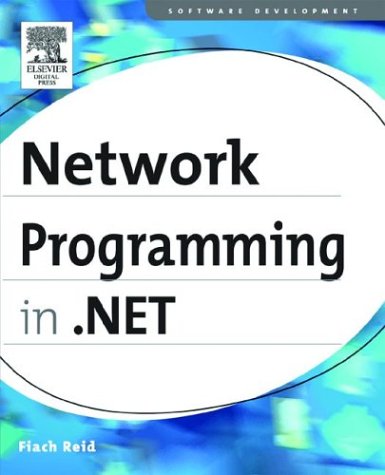
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AbstractExpressions.cs
- AbstractDataSvcMapFileLoader.cs
- XmlRootAttribute.cs
- TitleStyle.cs
- DetailsViewInsertEventArgs.cs
- Link.cs
- Path.cs
- HttpResponseHeader.cs
- CharConverter.cs
- MethodImplAttribute.cs
- FrameworkElement.cs
- CompilationRelaxations.cs
- BitmapEffectrendercontext.cs
- COM2Enum.cs
- ObjectDataSourceWizardForm.cs
- Source.cs
- ByteStreamGeometryContext.cs
- AdapterDictionary.cs
- TextRange.cs
- MimeMapping.cs
- RightsManagementPermission.cs
- OutOfMemoryException.cs
- ForceCopyBuildProvider.cs
- SecureConversationServiceElement.cs
- Container.cs
- TcpTransportSecurityElement.cs
- Nodes.cs
- CacheMode.cs
- PerformanceCounterLib.cs
- ResponseStream.cs
- DiscoveryEndpointElement.cs
- ParseHttpDate.cs
- SyndicationItemFormatter.cs
- FrameworkContentElement.cs
- PageAsyncTaskManager.cs
- MouseDevice.cs
- FontWeights.cs
- SwitchLevelAttribute.cs
- VisualProxy.cs
- ClientRolePrincipal.cs
- webbrowsersite.cs
- ExpressionBuilderCollection.cs
- invalidudtexception.cs
- AQNBuilder.cs
- LogicalExpr.cs
- DataGridPageChangedEventArgs.cs
- EnumerationRangeValidationUtil.cs
- ButtonChrome.cs
- DrawingContext.cs
- CommandEventArgs.cs
- OrderByExpression.cs
- RadioButtonList.cs
- EntityContainerEntitySet.cs
- LinqDataSourceValidationException.cs
- Tuple.cs
- EncryptedType.cs
- IndependentlyAnimatedPropertyMetadata.cs
- ToolStripMenuItemCodeDomSerializer.cs
- DebuggerAttributes.cs
- SQLRoleProvider.cs
- AppDomainFactory.cs
- ControlAdapter.cs
- ObjectHandle.cs
- ToolStripStatusLabel.cs
- EdmError.cs
- NavigationPropertyEmitter.cs
- NotFiniteNumberException.cs
- ISFTagAndGuidCache.cs
- RowBinding.cs
- UInt64.cs
- CultureInfoConverter.cs
- FocusChangedEventArgs.cs
- AutomationAttributeInfo.cs
- BindingGraph.cs
- DesignSurface.cs
- HtmlInputCheckBox.cs
- SplitterDesigner.cs
- ServiceParser.cs
- WarningException.cs
- XamlSerializerUtil.cs
- CapabilitiesAssignment.cs
- StrokeCollection2.cs
- DemultiplexingDispatchMessageFormatter.cs
- DataGridAddNewRow.cs
- QuerySafeNavigator.cs
- TextEditorParagraphs.cs
- QilValidationVisitor.cs
- AuthStoreRoleProvider.cs
- ToolStripItemImageRenderEventArgs.cs
- Version.cs
- DecimalStorage.cs
- WmlObjectListAdapter.cs
- CodeVariableReferenceExpression.cs
- OleStrCAMarshaler.cs
- GatewayDefinition.cs
- AutoGeneratedFieldProperties.cs
- CellIdBoolean.cs
- CollectionViewProxy.cs
- DataGridViewTextBoxEditingControl.cs
- CompressionTransform.cs