Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / DemultiplexingDispatchMessageFormatter.cs / 1 / DemultiplexingDispatchMessageFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Globalization; using System.Text; class DemultiplexingDispatchMessageFormatter : IDispatchMessageFormatter { IDispatchMessageFormatter defaultFormatter; Dictionaryformatters; string supportedFormats; public DemultiplexingDispatchMessageFormatter(IDictionary formatters, IDispatchMessageFormatter defaultFormatter) { if (formatters == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("formatters"); } this.formatters = new Dictionary (); foreach (WebContentFormat key in formatters.Keys) { this.formatters.Add(key, formatters[key]); } this.defaultFormatter = defaultFormatter; } public void DeserializeRequest(Message message, object[] parameters) { if (message == null) { return; } WebContentFormat format; IDispatchMessageFormatter selectedFormatter; if (TryGetEncodingFormat(message, out format)) { this.formatters.TryGetValue(format, out selectedFormatter); if (selectedFormatter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR2.GetString(SR2.UnrecognizedHttpMessageFormat, format, GetSupportedFormats()))); } } else { selectedFormatter = this.defaultFormatter; if (selectedFormatter == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR2.GetString(SR2.MessageFormatPropertyNotFound3))); } } selectedFormatter.DeserializeRequest(message, parameters); } public Message SerializeReply(MessageVersion messageVersion, object[] parameters, object result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR2.GetString(SR2.SerializingReplyNotSupportedByFormatter, this))); } internal static string GetSupportedFormats(IEnumerable formats) { StringBuilder sb = new StringBuilder(); int i = 0; foreach (WebContentFormat format in formats) { if (i > 0) { sb.Append(CultureInfo.CurrentCulture.TextInfo.ListSeparator); sb.Append(" "); } sb.Append("'" + format.ToString() + "'"); ++i; } return sb.ToString(); } internal static bool TryGetEncodingFormat(Message message, out WebContentFormat format) { object prop; message.Properties.TryGetValue(WebBodyFormatMessageProperty.Name, out prop); WebBodyFormatMessageProperty formatProperty = prop as WebBodyFormatMessageProperty; if (formatProperty == null) { format = WebContentFormat.Default; return false; } format = formatProperty.Format; return true; } string GetSupportedFormats() { if (this.supportedFormats == null) { this.supportedFormats = GetSupportedFormats(this.formatters.Keys); } return this.supportedFormats; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
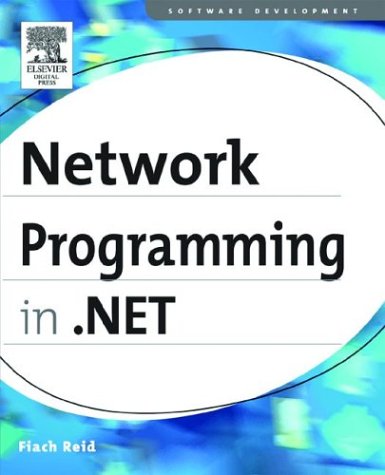
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectConverter.cs
- Dynamic.cs
- BinarySerializer.cs
- MappingModelBuildProvider.cs
- XmlExpressionDumper.cs
- UrlParameterReader.cs
- ExpressionPrinter.cs
- OpenTypeLayout.cs
- FamilyMapCollection.cs
- ExtendedPropertyDescriptor.cs
- ScaleTransform3D.cs
- SetterBaseCollection.cs
- returneventsaver.cs
- DataGridViewColumnStateChangedEventArgs.cs
- sqlmetadatafactory.cs
- StringExpressionSet.cs
- DetailsViewCommandEventArgs.cs
- ScriptResourceAttribute.cs
- BaseTemplateCodeDomTreeGenerator.cs
- HashAlgorithm.cs
- WebErrorHandler.cs
- DesigntimeLicenseContextSerializer.cs
- XmlSerializationGeneratedCode.cs
- HandlerBase.cs
- XmlSerializableWriter.cs
- X509WindowsSecurityToken.cs
- MultipartContentParser.cs
- loginstatus.cs
- StylusEditingBehavior.cs
- GeneratedCodeAttribute.cs
- WebFormsRootDesigner.cs
- UICuesEvent.cs
- IDataContractSurrogate.cs
- CodeTypeReferenceExpression.cs
- BitmapEffectDrawing.cs
- MembershipUser.cs
- PartBasedPackageProperties.cs
- SessionParameter.cs
- TopClause.cs
- SiteMapHierarchicalDataSourceView.cs
- TableItemPattern.cs
- BCryptSafeHandles.cs
- WorkflowQueue.cs
- CfgArc.cs
- XmlnsCache.cs
- SurrogateEncoder.cs
- MenuDesigner.cs
- DataControlPagerLinkButton.cs
- SchemaManager.cs
- UnknownBitmapEncoder.cs
- ApplicationException.cs
- ThreadStartException.cs
- ConfigXmlSignificantWhitespace.cs
- HtmlLink.cs
- XmlElementAttributes.cs
- BufferedStream.cs
- DataGridLinkButton.cs
- UiaCoreApi.cs
- ContractsBCL.cs
- ForeignKeyConstraint.cs
- WindowsScrollBarBits.cs
- StrokeFIndices.cs
- AttributeInfo.cs
- WsdlInspector.cs
- WmlObjectListAdapter.cs
- TrustManagerPromptUI.cs
- TypeSystem.cs
- WebRequestModulesSection.cs
- MergeLocalizationDirectives.cs
- COMException.cs
- SiteIdentityPermission.cs
- FormatConvertedBitmap.cs
- UIAgentAsyncEndRequest.cs
- PlaceHolder.cs
- BamlStream.cs
- DPTypeDescriptorContext.cs
- ProxyHwnd.cs
- StringInfo.cs
- SelectionGlyph.cs
- DrawingVisualDrawingContext.cs
- WindowsBrush.cs
- RightsManagementEncryptedStream.cs
- WindowsListViewGroup.cs
- BlockCollection.cs
- GlyphTypeface.cs
- CharUnicodeInfo.cs
- Attribute.cs
- XPathQueryGenerator.cs
- WeakReadOnlyCollection.cs
- DataTableReaderListener.cs
- RsaSecurityTokenParameters.cs
- SHA384.cs
- XPathNode.cs
- TextTreeRootNode.cs
- CodeDomConfigurationHandler.cs
- iisPickupDirectory.cs
- RuntimeArgumentHandle.cs
- DependencyObjectType.cs
- ValueProviderWrapper.cs
- COM2ColorConverter.cs