Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Ink / StylusEditingBehavior.cs / 1 / StylusEditingBehavior.cs
using System; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design; using System.Collections; using System.Collections.Generic; using System.Windows.Input; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Navigation; using System.Windows.Media; using System.Security; using System.Security.Permissions; namespace MS.Internal.Ink { ////// IStylusEditing Interface /// internal interface IStylusEditing { ////// AddStylusPoints /// /// stylusPoints /// only true if eventArgs.UserInitiated is true void AddStylusPoints(StylusPointCollection stylusPoints, bool userInitiated); } ////// StylusEditingBehavior - a base class for all stylus related editing behaviors /// internal abstract class StylusEditingBehavior : EditingBehavior, IStylusEditing { //-------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// /// /// internal StylusEditingBehavior(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) : base(editingCoordinator, inkCanvas) { } #endregion Constructors //------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods ////// An internal method which performs a mode change in mid-stroke. /// /// internal void SwitchToMode(InkCanvasEditingMode mode) { // NTRAID:WINDOWSOS#1464481-2006/01/30/-WAYNEZEN, // The dispather frames can be entered. If one calls InkCanvas.Select/Paste from a dispather frame // during the user editing, this method will be called. But before the method is processed completely, // the user input could kick in AddStylusPoints. So EditingCoordinator.UserIsEditing flag may be messed up. // Now we use _disableInput to disable the input during changing the mode in mid-stroke. _disableInput = true; try { OnSwitchToMode(mode); } finally { _disableInput = false; } } #endregion Internal Methods //-------------------------------------------------------------------------------- // // IStylusEditing Interface // //------------------------------------------------------------------------------- #region IStylusEditing Interface ////// IStylusEditing.AddStylusPoints /// /// stylusPoints /// true if the eventArgs source had UserInitiated set to true ////// Critical: Calls critical methods StylusInputBegin and StylusInputContinue /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] void IStylusEditing.AddStylusPoints(StylusPointCollection stylusPoints, bool userInitiated) { EditingCoordinator.DebugCheckActiveBehavior(this); // Don't process if SwitchToMode is called during the mid-stroke. if ( _disableInput ) { return; } if ( !EditingCoordinator.UserIsEditing ) { EditingCoordinator.UserIsEditing = true; StylusInputBegin(stylusPoints, userInitiated); } else { StylusInputContinue(stylusPoints, userInitiated); } } #endregion IStylusEditing Interface //-------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// An abstract method which performs a mode change in mid-stroke. /// /// protected abstract void OnSwitchToMode(InkCanvasEditingMode mode); ////// Called when the InkEditingBehavior is activated. /// protected override void OnActivate() { } ////// Called when the InkEditingBehavior is deactivated. /// protected override void OnDeactivate() { } ////// OnCommit /// /// protected sealed override void OnCommit(bool commit) { // Make sure that user is still editing if ( EditingCoordinator.UserIsEditing ) { EditingCoordinator.UserIsEditing = false; // The follow code raises variety editing events. // The out-side code could throw exception in the their handlers. We use try/finally block to protect our status. StylusInputEnd(commit); } else { // If user isn't editing, we should still call the derive class. // So the dynamic behavior like LSB can be self deactivated when it has been commited. OnCommitWithoutStylusInput(commit); } } ////// StylusInputBegin /// /// stylusPoints /// true if the source eventArgs.UserInitiated flag is true ////// Critical: Handles critical data stylusPoints, which is critical /// when InkCollectionBehavior uses it to call critical method /// InkCanvas.RaiseGestureOrStrokeCollected /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] protected virtual void StylusInputBegin(StylusPointCollection stylusPoints, bool userInitiated) { //defer to derived classes } ////// StylusInputContinue /// /// stylusPoints /// true if the source eventArgs.UserInitiated flag is true ////// Critical: Handles critical data stylusPoints, which is critical /// when InkCollectionBehavior uses it to call critical method InkCanvas.RaiseGestureOrStrokeCollected /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] protected virtual void StylusInputContinue(StylusPointCollection stylusPoints, bool userInitiated) { //defer to derived classes } ////// StylusInputEnd /// /// protected virtual void StylusInputEnd(bool commit) { //defer to derived classes } ////// OnCommitWithoutStylusInput /// /// protected virtual void OnCommitWithoutStylusInput(bool commit) { //defer to derived classes } #endregion Protected Methods //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private bool _disableInput; // No need for initializing. The default value is false. #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design; using System.Collections; using System.Collections.Generic; using System.Windows.Input; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Interop; using System.Windows.Navigation; using System.Windows.Media; using System.Security; using System.Security.Permissions; namespace MS.Internal.Ink { ////// IStylusEditing Interface /// internal interface IStylusEditing { ////// AddStylusPoints /// /// stylusPoints /// only true if eventArgs.UserInitiated is true void AddStylusPoints(StylusPointCollection stylusPoints, bool userInitiated); } ////// StylusEditingBehavior - a base class for all stylus related editing behaviors /// internal abstract class StylusEditingBehavior : EditingBehavior, IStylusEditing { //-------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// /// /// internal StylusEditingBehavior(EditingCoordinator editingCoordinator, InkCanvas inkCanvas) : base(editingCoordinator, inkCanvas) { } #endregion Constructors //------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods ////// An internal method which performs a mode change in mid-stroke. /// /// internal void SwitchToMode(InkCanvasEditingMode mode) { // NTRAID:WINDOWSOS#1464481-2006/01/30/-WAYNEZEN, // The dispather frames can be entered. If one calls InkCanvas.Select/Paste from a dispather frame // during the user editing, this method will be called. But before the method is processed completely, // the user input could kick in AddStylusPoints. So EditingCoordinator.UserIsEditing flag may be messed up. // Now we use _disableInput to disable the input during changing the mode in mid-stroke. _disableInput = true; try { OnSwitchToMode(mode); } finally { _disableInput = false; } } #endregion Internal Methods //-------------------------------------------------------------------------------- // // IStylusEditing Interface // //------------------------------------------------------------------------------- #region IStylusEditing Interface ////// IStylusEditing.AddStylusPoints /// /// stylusPoints /// true if the eventArgs source had UserInitiated set to true ////// Critical: Calls critical methods StylusInputBegin and StylusInputContinue /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] void IStylusEditing.AddStylusPoints(StylusPointCollection stylusPoints, bool userInitiated) { EditingCoordinator.DebugCheckActiveBehavior(this); // Don't process if SwitchToMode is called during the mid-stroke. if ( _disableInput ) { return; } if ( !EditingCoordinator.UserIsEditing ) { EditingCoordinator.UserIsEditing = true; StylusInputBegin(stylusPoints, userInitiated); } else { StylusInputContinue(stylusPoints, userInitiated); } } #endregion IStylusEditing Interface //-------------------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------------------- #region Protected Methods ////// An abstract method which performs a mode change in mid-stroke. /// /// protected abstract void OnSwitchToMode(InkCanvasEditingMode mode); ////// Called when the InkEditingBehavior is activated. /// protected override void OnActivate() { } ////// Called when the InkEditingBehavior is deactivated. /// protected override void OnDeactivate() { } ////// OnCommit /// /// protected sealed override void OnCommit(bool commit) { // Make sure that user is still editing if ( EditingCoordinator.UserIsEditing ) { EditingCoordinator.UserIsEditing = false; // The follow code raises variety editing events. // The out-side code could throw exception in the their handlers. We use try/finally block to protect our status. StylusInputEnd(commit); } else { // If user isn't editing, we should still call the derive class. // So the dynamic behavior like LSB can be self deactivated when it has been commited. OnCommitWithoutStylusInput(commit); } } ////// StylusInputBegin /// /// stylusPoints /// true if the source eventArgs.UserInitiated flag is true ////// Critical: Handles critical data stylusPoints, which is critical /// when InkCollectionBehavior uses it to call critical method /// InkCanvas.RaiseGestureOrStrokeCollected /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] protected virtual void StylusInputBegin(StylusPointCollection stylusPoints, bool userInitiated) { //defer to derived classes } ////// StylusInputContinue /// /// stylusPoints /// true if the source eventArgs.UserInitiated flag is true ////// Critical: Handles critical data stylusPoints, which is critical /// when InkCollectionBehavior uses it to call critical method InkCanvas.RaiseGestureOrStrokeCollected /// /// Note that for InkCollectionBehavior, which inherits from this class, the stylusPoints /// passed through this API are critical. For EraserBehavior and LassoSelectionBehavior, which /// also inherit from this class, the stylusPoints are not critical. This is because only /// InkCollectionBehavior calls a critical method with the stylusPoints as an argument. /// [SecurityCritical] protected virtual void StylusInputContinue(StylusPointCollection stylusPoints, bool userInitiated) { //defer to derived classes } ////// StylusInputEnd /// /// protected virtual void StylusInputEnd(bool commit) { //defer to derived classes } ////// OnCommitWithoutStylusInput /// /// protected virtual void OnCommitWithoutStylusInput(bool commit) { //defer to derived classes } #endregion Protected Methods //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private bool _disableInput; // No need for initializing. The default value is false. #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
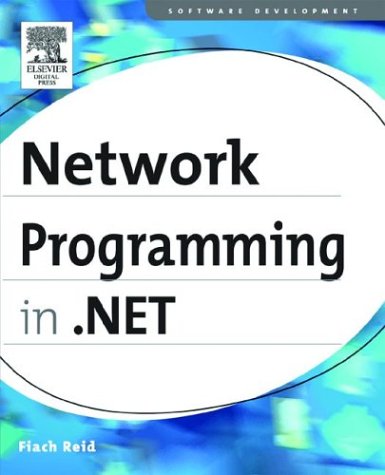
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Image.cs
- TdsParserStateObject.cs
- ConfigurationPermission.cs
- CustomValidator.cs
- EventWaitHandle.cs
- ObjectSecurityT.cs
- FileSystemEventArgs.cs
- XmlLangPropertyAttribute.cs
- XsdBuilder.cs
- UIPermission.cs
- WorkflowTransactionService.cs
- FlowLayoutSettings.cs
- NavigatorOutput.cs
- DataTrigger.cs
- DelegatingTypeDescriptionProvider.cs
- HtmlTableRowCollection.cs
- TextBoxAutomationPeer.cs
- TextSimpleMarkerProperties.cs
- ToolStrip.cs
- DataServices.cs
- AutoSizeComboBox.cs
- ZipIOExtraField.cs
- StateRuntime.cs
- HelpEvent.cs
- ObjectConverter.cs
- DataControlField.cs
- ExpressionParser.cs
- TcpTransportManager.cs
- TrayIconDesigner.cs
- OracleBFile.cs
- Run.cs
- SQLMoneyStorage.cs
- VisualTreeHelper.cs
- SequentialOutput.cs
- Cloud.cs
- ViewLoader.cs
- DBSchemaTable.cs
- WebHttpSecurity.cs
- XPathCompileException.cs
- Variable.cs
- CurrentChangingEventArgs.cs
- MulticastDelegate.cs
- SecurityTokenProvider.cs
- _SSPIWrapper.cs
- Source.cs
- TrackingParameters.cs
- GridViewPageEventArgs.cs
- ScriptingScriptResourceHandlerSection.cs
- FontStyle.cs
- TextClipboardData.cs
- DbConnectionFactory.cs
- DataGridViewCellCancelEventArgs.cs
- LiteralControl.cs
- X509Extension.cs
- HtmlImage.cs
- Win32PrintDialog.cs
- UInt64Converter.cs
- FontFamily.cs
- BinarySerializer.cs
- RegexFCD.cs
- Unit.cs
- InvalidAsynchronousStateException.cs
- IResourceProvider.cs
- ReadOnlyMetadataCollection.cs
- SizeAnimationUsingKeyFrames.cs
- TextPattern.cs
- ObjectIDGenerator.cs
- ObjectDisposedException.cs
- SymbolPair.cs
- ToolStripItemRenderEventArgs.cs
- DesignSurfaceServiceContainer.cs
- IPAddress.cs
- BrowserCapabilitiesFactoryBase.cs
- Column.cs
- ActivityWithResultConverter.cs
- ParallelTimeline.cs
- FtpRequestCacheValidator.cs
- InstanceLockQueryResult.cs
- FormsAuthentication.cs
- WebResponse.cs
- HttpWriter.cs
- FrameSecurityDescriptor.cs
- PopupControlService.cs
- TypedReference.cs
- RegistryDataKey.cs
- PrivilegeNotHeldException.cs
- HttpResponseWrapper.cs
- GPRECTF.cs
- CustomAttribute.cs
- EditorPartCollection.cs
- EntityDataSourceState.cs
- DataListItemEventArgs.cs
- ReferenceEqualityComparer.cs
- SiteOfOriginPart.cs
- DeclarativeCatalogPart.cs
- CornerRadiusConverter.cs
- Positioning.cs
- FontFamily.cs
- ProfileService.cs
- ClientUrlResolverWrapper.cs