Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / TypeSystem.cs / 2 / TypeSystem.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Utility functions for processing Expression trees // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Collections; using System.Text; using System.Reflection; using System.Diagnostics; using System.Linq; ///Utility functions for processing Expression trees internal static class TypeSystem { ///Method map for methods in URI query options private static readonly DictionaryexpressionMethodMap; /// VB Method map for methods in URI query options private static readonly DictionaryexpressionVBMethodMap; /// Properties that should be represented as methods private static readonly DictionarypropertiesAsMethodsMap; /// VB Assembly name #if !ASTORIA_LIGHT private const string VisualBasicAssemblyFullName = "Microsoft.VisualBasic, Version=8.0.0.0, Culture=neutral, PublicKeyToken=" + AssemblyRef.MicrosoftPublicKeyToken; #else private const string VisualBasicAssemblyFullName = "Microsoft.VisualBasic, Version=2.0.5.0, Culture=neutral, PublicKeyToken=" + AssemblyRef.MicrosoftSilverlightPublicKeyToken; #endif ////// Initializes method map /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1810:InitializeReferenceTypeStaticFieldsInline", Justification = "Cleaner code")] static TypeSystem() { // string functions #if !ASTORIA_LIGHT const int ExpectedCount = 24; #else const int ExpectedCount = 22; #endif expressionMethodMap = new Dictionary(ExpectedCount); expressionMethodMap.Add(typeof(string).GetMethod("Contains", new Type[] { typeof(string) }), @"substringof"); expressionMethodMap.Add(typeof(string).GetMethod("EndsWith", new Type[] { typeof(string) }), @"endswith"); expressionMethodMap.Add(typeof(string).GetMethod("StartsWith", new Type[] { typeof(string) }), @"startswith"); expressionMethodMap.Add(typeof(string).GetMethod("IndexOf", new Type[] { typeof(string) }), @"indexof"); expressionMethodMap.Add(typeof(string).GetMethod("Replace", new Type[] { typeof(string), typeof(string) }), @"replace"); expressionMethodMap.Add(typeof(string).GetMethod("Substring", new Type[] { typeof(int) }), @"substring"); expressionMethodMap.Add(typeof(string).GetMethod("Substring", new Type[] { typeof(int), typeof(int) }), @"substring"); expressionMethodMap.Add(typeof(string).GetMethod("ToLower", Type.EmptyTypes), @"tolower"); expressionMethodMap.Add(typeof(string).GetMethod("ToUpper", Type.EmptyTypes), @"toupper"); expressionMethodMap.Add(typeof(string).GetMethod("Trim", Type.EmptyTypes), @"trim"); expressionMethodMap.Add(typeof(string).GetMethod("Concat", new Type[] { typeof(string), typeof(string) }, null), @"concat"); expressionMethodMap.Add(typeof(string).GetProperty("Length", typeof(int)).GetGetMethod(), @"length"); // datetime methods expressionMethodMap.Add(typeof(DateTime).GetProperty("Day", typeof(int)).GetGetMethod(), @"day"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Hour", typeof(int)).GetGetMethod(), @"hour"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Month", typeof(int)).GetGetMethod(), @"month"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Minute", typeof(int)).GetGetMethod(), @"minute"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Second", typeof(int)).GetGetMethod(), @"second"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Year", typeof(int)).GetGetMethod(), @"year"); // math methods expressionMethodMap.Add(typeof(Math).GetMethod("Round", new Type[] { typeof(double) }), @"round"); expressionMethodMap.Add(typeof(Math).GetMethod("Round", new Type[] { typeof(decimal) }), @"round"); expressionMethodMap.Add(typeof(Math).GetMethod("Floor", new Type[] { typeof(double) }), @"floor"); #if !ASTORIA_LIGHT // Math.Floor(Decimal) not available expressionMethodMap.Add(typeof(Math).GetMethod("Floor", new Type[] { typeof(decimal) }), @"floor"); #endif expressionMethodMap.Add(typeof(Math).GetMethod("Ceiling", new Type[] { typeof(double) }), @"ceiling"); #if !ASTORIA_LIGHT // Math.Ceiling(Decimal) not available expressionMethodMap.Add(typeof(Math).GetMethod("Ceiling", new Type[] { typeof(decimal) }), @"ceiling"); #endif Debug.Assert(expressionMethodMap.Count == ExpectedCount, "expressionMethodMap.Count == ExpectedCount"); // vb methods // lookup these by type name + method name expressionVBMethodMap = new Dictionary (); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Trim", @"trim"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Len", @"length"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Mid", @"substring"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.UCase", @"toupper"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.LCase", @"tolower"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Year", @"year"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Month", @"month"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Day", @"day"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Hour", @"hour"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Minute", @"minute"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Second", @"second"); Debug.Assert(expressionVBMethodMap.Count == 11, "expressionVBMethodMap.Count == 11"); propertiesAsMethodsMap = new Dictionary (); propertiesAsMethodsMap.Add( typeof(string).GetProperty("Length", typeof(int)), typeof(string).GetProperty("Length", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Day", typeof(int)), typeof(DateTime).GetProperty("Day", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Hour", typeof(int)), typeof(DateTime).GetProperty("Hour", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Minute", typeof(int)), typeof(DateTime).GetProperty("Minute", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Second", typeof(int)), typeof(DateTime).GetProperty("Second", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Month", typeof(int)), typeof(DateTime).GetProperty("Month", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Year", typeof(int)), typeof(DateTime).GetProperty("Year", typeof(int)).GetGetMethod()); Debug.Assert(propertiesAsMethodsMap.Count == 7, "propertiesAsMethodsMap.Count == 7"); } /// /// Sees if method has URI equivalent /// /// The method info /// uri method name ///true/ false internal static bool TryGetQueryOptionMethod(MethodInfo mi, out string methodName) { return (expressionMethodMap.TryGetValue(mi, out methodName) || (mi.DeclaringType.Assembly.FullName == VisualBasicAssemblyFullName && expressionVBMethodMap.TryGetValue(mi.DeclaringType.FullName + "." + mi.Name, out methodName))); } ////// Sees if property can be represented as method for translation to URI /// /// The property info /// get method for property ///true/ false internal static bool TryGetPropertyAsMethod(PropertyInfo pi, out MethodInfo mi) { return propertiesAsMethodsMap.TryGetValue(pi, out mi); } ////// Gets the elementtype for a sequence /// /// The sequence type ///The element type internal static Type GetElementType(Type seqType) { Type ienum = FindIEnumerable(seqType); if (ienum == null) { return seqType; } return ienum.GetGenericArguments()[0]; } ////// Determines whether a property is private /// /// The PropertyInfo structure for the property ///true/ false if property is private internal static bool IsPrivate(PropertyInfo pi) { MethodInfo mi = pi.GetGetMethod() ?? pi.GetSetMethod(); if (mi != null) { return mi.IsPrivate; } return true; } ////// Finds type that implements IEnumerable so can get elemtent type /// /// The Type to check ///returns the type which implements IEnumerable internal static Type FindIEnumerable(Type seqType) { if (seqType == null || seqType == typeof(string)) { return null; } if (seqType.IsArray) { return typeof(IEnumerable<>).MakeGenericType(seqType.GetElementType()); } if (seqType.IsGenericType) { foreach (Type arg in seqType.GetGenericArguments()) { Type ienum = typeof(IEnumerable<>).MakeGenericType(arg); if (ienum.IsAssignableFrom(seqType)) { return ienum; } } } Type[] ifaces = seqType.GetInterfaces(); if (ifaces != null && ifaces.Length > 0) { foreach (Type iface in ifaces) { Type ienum = FindIEnumerable(iface); if (ienum != null) { return ienum; } } } if (seqType.BaseType != null && seqType.BaseType != typeof(object)) { return FindIEnumerable(seqType.BaseType); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Utility functions for processing Expression trees // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Collections; using System.Text; using System.Reflection; using System.Diagnostics; using System.Linq; ///Utility functions for processing Expression trees internal static class TypeSystem { ///Method map for methods in URI query options private static readonly DictionaryexpressionMethodMap; /// VB Method map for methods in URI query options private static readonly DictionaryexpressionVBMethodMap; /// Properties that should be represented as methods private static readonly DictionarypropertiesAsMethodsMap; /// VB Assembly name #if !ASTORIA_LIGHT private const string VisualBasicAssemblyFullName = "Microsoft.VisualBasic, Version=8.0.0.0, Culture=neutral, PublicKeyToken=" + AssemblyRef.MicrosoftPublicKeyToken; #else private const string VisualBasicAssemblyFullName = "Microsoft.VisualBasic, Version=2.0.5.0, Culture=neutral, PublicKeyToken=" + AssemblyRef.MicrosoftSilverlightPublicKeyToken; #endif ////// Initializes method map /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1810:InitializeReferenceTypeStaticFieldsInline", Justification = "Cleaner code")] static TypeSystem() { // string functions #if !ASTORIA_LIGHT const int ExpectedCount = 24; #else const int ExpectedCount = 22; #endif expressionMethodMap = new Dictionary(ExpectedCount); expressionMethodMap.Add(typeof(string).GetMethod("Contains", new Type[] { typeof(string) }), @"substringof"); expressionMethodMap.Add(typeof(string).GetMethod("EndsWith", new Type[] { typeof(string) }), @"endswith"); expressionMethodMap.Add(typeof(string).GetMethod("StartsWith", new Type[] { typeof(string) }), @"startswith"); expressionMethodMap.Add(typeof(string).GetMethod("IndexOf", new Type[] { typeof(string) }), @"indexof"); expressionMethodMap.Add(typeof(string).GetMethod("Replace", new Type[] { typeof(string), typeof(string) }), @"replace"); expressionMethodMap.Add(typeof(string).GetMethod("Substring", new Type[] { typeof(int) }), @"substring"); expressionMethodMap.Add(typeof(string).GetMethod("Substring", new Type[] { typeof(int), typeof(int) }), @"substring"); expressionMethodMap.Add(typeof(string).GetMethod("ToLower", Type.EmptyTypes), @"tolower"); expressionMethodMap.Add(typeof(string).GetMethod("ToUpper", Type.EmptyTypes), @"toupper"); expressionMethodMap.Add(typeof(string).GetMethod("Trim", Type.EmptyTypes), @"trim"); expressionMethodMap.Add(typeof(string).GetMethod("Concat", new Type[] { typeof(string), typeof(string) }, null), @"concat"); expressionMethodMap.Add(typeof(string).GetProperty("Length", typeof(int)).GetGetMethod(), @"length"); // datetime methods expressionMethodMap.Add(typeof(DateTime).GetProperty("Day", typeof(int)).GetGetMethod(), @"day"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Hour", typeof(int)).GetGetMethod(), @"hour"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Month", typeof(int)).GetGetMethod(), @"month"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Minute", typeof(int)).GetGetMethod(), @"minute"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Second", typeof(int)).GetGetMethod(), @"second"); expressionMethodMap.Add(typeof(DateTime).GetProperty("Year", typeof(int)).GetGetMethod(), @"year"); // math methods expressionMethodMap.Add(typeof(Math).GetMethod("Round", new Type[] { typeof(double) }), @"round"); expressionMethodMap.Add(typeof(Math).GetMethod("Round", new Type[] { typeof(decimal) }), @"round"); expressionMethodMap.Add(typeof(Math).GetMethod("Floor", new Type[] { typeof(double) }), @"floor"); #if !ASTORIA_LIGHT // Math.Floor(Decimal) not available expressionMethodMap.Add(typeof(Math).GetMethod("Floor", new Type[] { typeof(decimal) }), @"floor"); #endif expressionMethodMap.Add(typeof(Math).GetMethod("Ceiling", new Type[] { typeof(double) }), @"ceiling"); #if !ASTORIA_LIGHT // Math.Ceiling(Decimal) not available expressionMethodMap.Add(typeof(Math).GetMethod("Ceiling", new Type[] { typeof(decimal) }), @"ceiling"); #endif Debug.Assert(expressionMethodMap.Count == ExpectedCount, "expressionMethodMap.Count == ExpectedCount"); // vb methods // lookup these by type name + method name expressionVBMethodMap = new Dictionary (); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Trim", @"trim"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Len", @"length"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.Mid", @"substring"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.UCase", @"toupper"); expressionVBMethodMap.Add("Microsoft.VisualBasic.Strings.LCase", @"tolower"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Year", @"year"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Month", @"month"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Day", @"day"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Hour", @"hour"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Minute", @"minute"); expressionVBMethodMap.Add("Microsoft.VisualBasic.DateAndTime.Second", @"second"); Debug.Assert(expressionVBMethodMap.Count == 11, "expressionVBMethodMap.Count == 11"); propertiesAsMethodsMap = new Dictionary (); propertiesAsMethodsMap.Add( typeof(string).GetProperty("Length", typeof(int)), typeof(string).GetProperty("Length", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Day", typeof(int)), typeof(DateTime).GetProperty("Day", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Hour", typeof(int)), typeof(DateTime).GetProperty("Hour", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Minute", typeof(int)), typeof(DateTime).GetProperty("Minute", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Second", typeof(int)), typeof(DateTime).GetProperty("Second", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Month", typeof(int)), typeof(DateTime).GetProperty("Month", typeof(int)).GetGetMethod()); propertiesAsMethodsMap.Add( typeof(DateTime).GetProperty("Year", typeof(int)), typeof(DateTime).GetProperty("Year", typeof(int)).GetGetMethod()); Debug.Assert(propertiesAsMethodsMap.Count == 7, "propertiesAsMethodsMap.Count == 7"); } /// /// Sees if method has URI equivalent /// /// The method info /// uri method name ///true/ false internal static bool TryGetQueryOptionMethod(MethodInfo mi, out string methodName) { return (expressionMethodMap.TryGetValue(mi, out methodName) || (mi.DeclaringType.Assembly.FullName == VisualBasicAssemblyFullName && expressionVBMethodMap.TryGetValue(mi.DeclaringType.FullName + "." + mi.Name, out methodName))); } ////// Sees if property can be represented as method for translation to URI /// /// The property info /// get method for property ///true/ false internal static bool TryGetPropertyAsMethod(PropertyInfo pi, out MethodInfo mi) { return propertiesAsMethodsMap.TryGetValue(pi, out mi); } ////// Gets the elementtype for a sequence /// /// The sequence type ///The element type internal static Type GetElementType(Type seqType) { Type ienum = FindIEnumerable(seqType); if (ienum == null) { return seqType; } return ienum.GetGenericArguments()[0]; } ////// Determines whether a property is private /// /// The PropertyInfo structure for the property ///true/ false if property is private internal static bool IsPrivate(PropertyInfo pi) { MethodInfo mi = pi.GetGetMethod() ?? pi.GetSetMethod(); if (mi != null) { return mi.IsPrivate; } return true; } ////// Finds type that implements IEnumerable so can get elemtent type /// /// The Type to check ///returns the type which implements IEnumerable internal static Type FindIEnumerable(Type seqType) { if (seqType == null || seqType == typeof(string)) { return null; } if (seqType.IsArray) { return typeof(IEnumerable<>).MakeGenericType(seqType.GetElementType()); } if (seqType.IsGenericType) { foreach (Type arg in seqType.GetGenericArguments()) { Type ienum = typeof(IEnumerable<>).MakeGenericType(arg); if (ienum.IsAssignableFrom(seqType)) { return ienum; } } } Type[] ifaces = seqType.GetInterfaces(); if (ifaces != null && ifaces.Length > 0) { foreach (Type iface in ifaces) { Type ienum = FindIEnumerable(iface); if (ienum != null) { return ienum; } } } if (seqType.BaseType != null && seqType.BaseType != typeof(object)) { return FindIEnumerable(seqType.BaseType); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
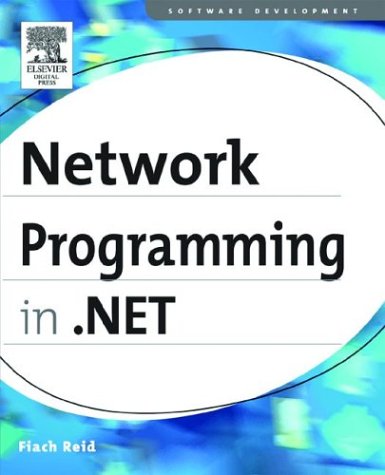
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DrawTreeNodeEventArgs.cs
- CodeTypeDelegate.cs
- CommandValueSerializer.cs
- Point.cs
- RepeatBehavior.cs
- EventSourceCreationData.cs
- DataColumnPropertyDescriptor.cs
- XamlTemplateSerializer.cs
- HandlerBase.cs
- DependencyPropertyHelper.cs
- OperationPickerDialog.designer.cs
- ClientType.cs
- SqlFlattener.cs
- WebBrowserBase.cs
- _ConnectOverlappedAsyncResult.cs
- TableChangeProcessor.cs
- PrintPreviewControl.cs
- ConfigXmlCDataSection.cs
- SoapProtocolReflector.cs
- XmlSchemaComplexContentRestriction.cs
- TemplatedEditableDesignerRegion.cs
- RotateTransform.cs
- SecurityTokenException.cs
- QueryOptionExpression.cs
- CheckPair.cs
- CommonRemoteMemoryBlock.cs
- TreeViewItem.cs
- DmlSqlGenerator.cs
- WS2007HttpBinding.cs
- Identity.cs
- SqlDataSourceSummaryPanel.cs
- XmlDocumentFragment.cs
- NotFiniteNumberException.cs
- SamlConstants.cs
- ContentIterators.cs
- WebPartEditVerb.cs
- WebPartConnectionsDisconnectVerb.cs
- EditingScope.cs
- ElementHostAutomationPeer.cs
- ScrollableControlDesigner.cs
- FontDriver.cs
- SkewTransform.cs
- Calendar.cs
- EdmConstants.cs
- PreservationFileReader.cs
- DecimalStorage.cs
- ValueUtilsSmi.cs
- CheckBox.cs
- IApplicationTrustManager.cs
- XmlSchemaAnnotation.cs
- CodeIterationStatement.cs
- ResourceAssociationTypeEnd.cs
- DataGridViewAccessibleObject.cs
- ModelItemImpl.cs
- ProcessModelSection.cs
- RowParagraph.cs
- TextUtf8RawTextWriter.cs
- ChtmlCalendarAdapter.cs
- BitVec.cs
- AliasGenerator.cs
- xsdvalidator.cs
- WindowsRichEdit.cs
- ValueSerializerAttribute.cs
- OuterGlowBitmapEffect.cs
- TypedElement.cs
- ContextStack.cs
- UpdateEventArgs.cs
- ExpressionVisitor.cs
- _SecureChannel.cs
- Int16AnimationBase.cs
- RPIdentityRequirement.cs
- ClrProviderManifest.cs
- GraphicsPath.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- listitem.cs
- TableChangeProcessor.cs
- AccessorTable.cs
- DBConcurrencyException.cs
- StylusTip.cs
- WindowsRegion.cs
- ConfigXmlSignificantWhitespace.cs
- DataGridViewCellFormattingEventArgs.cs
- QueryResponse.cs
- SocketInformation.cs
- DateTimeSerializationSection.cs
- SpeakCompletedEventArgs.cs
- SqlConnectionFactory.cs
- EnumUnknown.cs
- WindowsListViewItem.cs
- CollectionConverter.cs
- TrustManager.cs
- MultiView.cs
- TypeNameConverter.cs
- TypedRowHandler.cs
- IndexingContentUnit.cs
- ScriptControl.cs
- InheritanceAttribute.cs
- WindowsListViewItemCheckBox.cs
- unsafenativemethodstextservices.cs
- Rect3D.cs