Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / ModelItemImpl.cs / 1305376 / ModelItemImpl.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Markup; using System.Activities.Presentation.Services; using System.Activities.Presentation.View; using System.Linq; using System.Runtime; using System.Dynamic; using System.Reflection; // This class provides the View facing ModelItem implementation, this works with the ModelTreeManager // and keeps the xaml up to date by intercepting every change to the model properties. class ModelItemImpl : ModelItem, IModelTreeItem, ICustomTypeDescriptor, IDynamicMetaObjectProvider { ModelProperty contentProperty; object instance; Type itemType; DictionarymodelPropertyStore; ModelTreeManager modelTreeManager; ModelProperty nameProperty; List parents; ModelPropertyCollectionImpl properties; List sources; List subTreeNodesThatNeedBackLinkPatching; DependencyObject view; public ModelItemImpl(ModelTreeManager modelTreeManager, Type itemType, object instance, ModelItem parent) { this.itemType = itemType; this.instance = instance; this.modelTreeManager = modelTreeManager; this.parents = new List (1); this.sources = new List (1); if (parent != null) { this.parents.Add(parent); } this.modelPropertyStore = new Dictionary (); this.subTreeNodesThatNeedBackLinkPatching = new List (); } public override event PropertyChangedEventHandler PropertyChanged; public override global::System.ComponentModel.AttributeCollection Attributes { get { return TypeDescriptor.GetAttributes(itemType); } } public override ModelProperty Content { get { if (this.contentProperty == null) { Fx.Assert(this.instance != null, "instance should not be null"); ContentPropertyAttribute contentAttribute = TypeDescriptor.GetAttributes(this.instance)[typeof(ContentPropertyAttribute)] as ContentPropertyAttribute; if (contentAttribute != null && !String.IsNullOrEmpty(contentAttribute.Name)) { this.contentProperty = this.Properties.Find(contentAttribute.Name); } } return contentProperty; } } public override Type ItemType { get { return this.itemType; } } public ModelItem ModelItem { get { return this; } } public override string Name { get { string name = null; if ((this.NameProperty != null) && (this.NameProperty.Value != null)) { name = (string)this.NameProperty.Value.GetCurrentValue(); } return name; } set { if (this.NameProperty != null) { this.NameProperty.SetValue(value); } } } public override ModelItem Parent { get { return (this.Parents.Count() > 0) ? this.Parents.First() : null; } } public override ModelPropertyCollection Properties { get { if (this.properties == null) { properties = new ModelPropertyCollectionImpl(this); } return properties; } } public override ModelItem Root { get { return this.modelTreeManager.Root; } } // This holds a reference to the modelproperty that is currently holding this ModelItem. public override ModelProperty Source { get { return (this.sources.Count > 0) ? this.sources.First() : null; } } public override DependencyObject View { get { return this.view; } } public override IEnumerable Parents { get { return this.parents.Concat( from source in this.sources select source.Parent); } } public override IEnumerable Sources { get { return this.sources; } } protected ModelProperty NameProperty { get { if (this.nameProperty == null) { Fx.Assert(this.instance != null, "instance should not be null"); RuntimeNamePropertyAttribute runtimeNamePropertyAttribute = TypeDescriptor.GetAttributes(this.instance)[typeof(RuntimeNamePropertyAttribute)] as RuntimeNamePropertyAttribute; if (runtimeNamePropertyAttribute != null && !String.IsNullOrEmpty(runtimeNamePropertyAttribute.Name)) { this.nameProperty = this.Properties.Find(runtimeNamePropertyAttribute.Name); } } return nameProperty; } } Dictionary IModelTreeItem.ModelPropertyStore { get { return modelPropertyStore; } } ModelTreeManager IModelTreeItem.ModelTreeManager { get { return modelTreeManager; } } void IModelTreeItem.SetCurrentView(DependencyObject view) { this.view = view; } public override ModelEditingScope BeginEdit(string description) { return this.modelTreeManager.CreateEditingScope(description); } public override ModelEditingScope BeginEdit() { return this.BeginEdit(null); } public override object GetCurrentValue() { return this.instance; } void IModelTreeItem.OnPropertyChanged(string propertyName) { this.OnPropertyChanged(propertyName); } void IModelTreeItem.SetParent(ModelItem dataModelItem) { if (dataModelItem != null && !this.parents.Contains(dataModelItem)) { this.parents.Add(dataModelItem); } } void IModelTreeItem.SetSource(ModelProperty property) { if (!this.sources.Contains(property)) { // also check if the same parent.property is in the list as a different instance of oldModelProperty ModelProperty foundProperty = this.sources.Find((modelProperty) => modelProperty.Name.Equals(property.Name) && property.Parent == modelProperty.Parent); if (foundProperty == null) { this.sources.Add(property); } } } IList IModelTreeItem.SubNodesThatNeedBackLinkUpdate { get { return this.subTreeNodesThatNeedBackLinkPatching; } } #region ICustomTypeDescriptor Members AttributeCollection ICustomTypeDescriptor.GetAttributes() { return this.Attributes; } string ICustomTypeDescriptor.GetClassName() { return TypeDescriptor.GetClassName(this); } string ICustomTypeDescriptor.GetComponentName() { return TypeDescriptor.GetComponentName(this); } TypeConverter ICustomTypeDescriptor.GetConverter() { return ModelUtilities.GetConverter(this); } EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { // we dont support events; return null; } PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return ModelUtilities.GetDefaultProperty(this); } object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { // we dont support editors return null; } EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { // we dont support events; return null; } EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { // we dont support events; return null; } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { return ModelUtilities.WrapProperties(this); } PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { // get model properties List properties = new List (); foreach (PropertyDescriptor modelPropertyDescriptor in ModelUtilities.WrapProperties(this)) { properties.Add(modelPropertyDescriptor); } // try to see if there are pseudo builtin properties for this type. AttachedPropertiesService AttachedPropertiesService = this.modelTreeManager.Context.Services.GetService (); if (AttachedPropertiesService != null) { var nonBrowsableAttachedProperties = from attachedProperty in AttachedPropertiesService.GetAttachedProperties(this.itemType) where !attachedProperty.IsBrowsable select attachedProperty; foreach (AttachedProperty AttachedProperty in nonBrowsableAttachedProperties) { properties.Add(new AttachedPropertyDescriptor(AttachedProperty, this)); } } return new PropertyDescriptorCollection(properties.ToArray(), true); } object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } #endregion void IModelTreeItem.RemoveParent(ModelItem oldParent) { if (this.parents.Contains(oldParent)) { this.parents.Remove(oldParent); } } void IModelTreeItem.RemoveSource(ModelProperty oldModelProperty) { if (this.sources.Contains(oldModelProperty)) { this.sources.Remove(oldModelProperty); } else { // also check if the same parent.property is in the list as a different instance of oldModelProperty ModelProperty foundProperty = this.sources.Find((modelProperty) => modelProperty.Name.Equals(oldModelProperty.Name) && modelProperty.Parent == oldModelProperty.Parent); if (foundProperty != null) { this.sources.Remove(foundProperty); } } } protected virtual void OnPropertyChanged(string propertyName) { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } DynamicMetaObject IDynamicMetaObjectProvider.GetMetaObject(System.Linq.Expressions.Expression parameter) { return new ModelItemMetaObject(parameter,this); } public object SetPropertyValue(string propertyName, object val) { ModelProperty modelProperty = this.Properties.Find(propertyName); if (modelProperty != null) { modelProperty.SetValue(val); } else { PropertyDescriptor descriptor = TypeDescriptor.GetProperties(this)[propertyName]; if (descriptor != null) { descriptor.SetValue(this, val); } } return GetPropertyValue(propertyName); } public object GetPropertyValue(string propertyName) { ModelProperty modelProperty = this.Properties.Find(propertyName); object value = null; if (modelProperty != null) { ModelItem valueModelitem = modelProperty.Value; if (valueModelitem == null) { value = null; } else { Type itemType = valueModelitem.ItemType; if (itemType.IsPrimitive || itemType.IsEnum || itemType.Equals(typeof(String))) { value = valueModelitem.GetCurrentValue(); } else { value = valueModelitem; } } } else { PropertyDescriptor descriptor = TypeDescriptor.GetProperties(this)[propertyName]; if (descriptor != null) { value = descriptor.GetValue(this); } } return value; } class ModelItemMetaObject : System.Dynamic.DynamicMetaObject { MethodInfo getPropertyValueMethodInfo = typeof(ModelItemImpl).GetMethod("GetPropertyValue"); MethodInfo setPropertyValueMethodInfo = typeof(ModelItemImpl).GetMethod("SetPropertyValue"); public ModelItemMetaObject(System.Linq.Expressions.Expression parameter, ModelItemImpl target) : base(parameter, BindingRestrictions.Empty,target) { } public override DynamicMetaObject BindGetMember(GetMemberBinder binder) { System.Linq.Expressions.Expression s = System.Linq.Expressions.Expression.Convert(this.Expression, typeof(ModelItemImpl)); System.Linq.Expressions.Expression value = System.Linq.Expressions.Expression.Call(s, getPropertyValueMethodInfo, System.Linq.Expressions.Expression.Constant(binder.Name)); return new DynamicMetaObject(value, BindingRestrictions.GetTypeRestriction(this.Expression, this.LimitType)); } public override DynamicMetaObject BindSetMember(SetMemberBinder binder, DynamicMetaObject value) { System.Linq.Expressions.Expression s = System.Linq.Expressions.Expression.Convert(this.Expression, typeof(ModelItemImpl)); System.Linq.Expressions.Expression objectValue = System.Linq.Expressions.Expression.Convert(value.Expression, typeof(object)); System.Linq.Expressions.Expression valueExp = System.Linq.Expressions.Expression.Call(s, setPropertyValueMethodInfo, System.Linq.Expressions.Expression.Constant(binder.Name), objectValue); return new DynamicMetaObject(valueExp, BindingRestrictions.GetTypeRestriction(this.Expression, this.LimitType)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
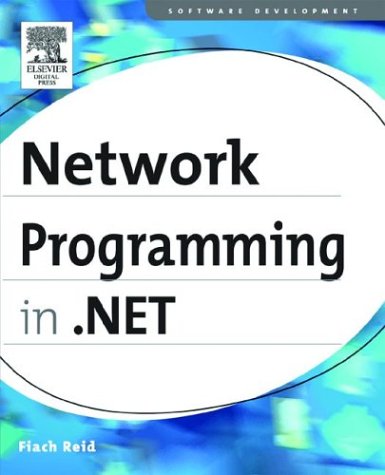
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNode.cs
- ConnectionStringSettingsCollection.cs
- MexHttpsBindingCollectionElement.cs
- ControlTemplate.cs
- Relationship.cs
- GridEntry.cs
- AvtEvent.cs
- StylusPlugInCollection.cs
- SafeArrayTypeMismatchException.cs
- MimeObjectFactory.cs
- MemoryMappedViewStream.cs
- XmlWriterTraceListener.cs
- NumberFormatInfo.cs
- GridViewColumn.cs
- MaskedTextBox.cs
- CompositeTypefaceMetrics.cs
- CommandManager.cs
- SolidColorBrush.cs
- CompilerTypeWithParams.cs
- ValidationErrorEventArgs.cs
- ResetableIterator.cs
- DataServiceKeyAttribute.cs
- FormViewPageEventArgs.cs
- CaseInsensitiveComparer.cs
- VirtualPathProvider.cs
- ActivityTrace.cs
- DispatcherOperation.cs
- TextContainerChangedEventArgs.cs
- QueueProcessor.cs
- Emitter.cs
- SynchronizedInputPattern.cs
- StringBuilder.cs
- PointConverter.cs
- HistoryEventArgs.cs
- FunctionImportMapping.cs
- ArithmeticException.cs
- ObjectDataSourceDisposingEventArgs.cs
- DocumentViewer.cs
- SqlCommandSet.cs
- SqlTransaction.cs
- codemethodreferenceexpression.cs
- JsonReaderWriterFactory.cs
- XmlSerializerNamespaces.cs
- ByeOperation11AsyncResult.cs
- DoWhile.cs
- DataGridToolTip.cs
- ResourceManagerWrapper.cs
- unsafenativemethodsother.cs
- TraceSection.cs
- GridProviderWrapper.cs
- DynamicValidator.cs
- XmlDataImplementation.cs
- NetSectionGroup.cs
- ContextMenuAutomationPeer.cs
- _HTTPDateParse.cs
- TreeViewHitTestInfo.cs
- HGlobalSafeHandle.cs
- TransformGroup.cs
- ETagAttribute.cs
- WsatAdminException.cs
- ExpandableObjectConverter.cs
- Array.cs
- DataGridViewDesigner.cs
- Setter.cs
- UnauthorizedWebPart.cs
- SetStoryboardSpeedRatio.cs
- QueryReaderSettings.cs
- ManipulationDeltaEventArgs.cs
- WebPermission.cs
- OpenFileDialog.cs
- LocalizabilityAttribute.cs
- XmlSerializationWriter.cs
- DataBoundControl.cs
- TransformProviderWrapper.cs
- SaveFileDialogDesigner.cs
- FunctionMappingTranslator.cs
- FixedStringLookup.cs
- DynamicILGenerator.cs
- ClrProviderManifest.cs
- DataSourceProvider.cs
- StorageMappingFragment.cs
- ImageClickEventArgs.cs
- ISessionStateStore.cs
- RestHandlerFactory.cs
- InternalsVisibleToAttribute.cs
- HtmlHistory.cs
- BooleanStorage.cs
- ReadOnlyDictionary.cs
- CellLabel.cs
- IApplicationTrustManager.cs
- DataGridAutomationPeer.cs
- Content.cs
- DbParameterHelper.cs
- FileLogRecordEnumerator.cs
- CopyAction.cs
- PnrpPeerResolverBindingElement.cs
- ToolboxItemFilterAttribute.cs
- EventSinkHelperWriter.cs
- ChineseLunisolarCalendar.cs
- ChainedAsyncResult.cs