Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / TableRowGroup.cs / 1 / TableRowGroup.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table row group implementation // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- //In order to avoid generating warnings about unknown message numbers and //unknown pragmas when compiling your C# source code with the actual C# compiler, //you need to disable warnings 1634 and 1691. (Presharp Documentation) #pragma warning disable 1634, 1691 using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Utility; using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Markup; using MS.Internal; using MS.Internal.Data; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Table row group implementation /// [ContentProperty("Rows")] public class TableRowGroup : TextElement, IAddChild { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of a RowGroup /// public TableRowGroup() : base() { Initialize(); } // common initialization for all constructors private void Initialize() { _rows = new TableRowCollection(this); _rowInsertionIndex = -1; _parentIndex = -1; } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// void IAddChild.AddChild(object value) { if (value == null) { throw new ArgumentNullException("value"); } TableRow row = value as TableRow; if (row != null) { Rows.Add(row); return; } throw (new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRow)), "value")); } ////// /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// Returns the row group's row collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public TableRowCollection Rows { get { return (_rows); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeRows() { return Rows.Count > 0; } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the RowGroup about entering model tree. /// internal void OnEnterParentTree() { if(Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnAfterExitParentTree(Table table) { table.OnStructureChanged(); } ////// ValidateStructure /// internal void ValidateStructure() { RowSpanVector rowSpanVector = new RowSpanVector(); _columnCount = 0; for (int i = 0; i < Rows.Count; ++i) { Rows[i].ValidateStructure(rowSpanVector); _columnCount = Math.Max(_columnCount, Rows[i].ColumnCount); } Table.EnsureColumnCount(_columnCount); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return Parent as Table; } } ////// RowGroup's index in the parents row group collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// Stores temporary data for where to insert a new row /// internal int InsertionIndex { get { return _rowInsertionIndex; } set { _rowInsertionIndex = value; } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Called when body receives a new parent (via OM or text tree) /// /// /// New parent of body /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; if (newParent != null && !(newParent is Table)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if (oldParent != null) { OnExitParentTree(); ((Table)oldParent).RowGroups.InternalRemove(this); OnAfterExitParentTree(oldParent as Table); } base.OnNewParent(newParent); if (newParent != null) { ((Table)newParent).RowGroups.InternalAdd(this); OnEnterParentTree(); } } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private TableRowCollection _rows; // children rows store private int _parentIndex; // row group's index in parent's children collection private int _rowInsertionIndex; // Insertion index for row private int _columnCount; // Column count. #endregion Private Fields //------------------------------------------------------ // // Private Structures / Classes // //----------------------------------------------------- } } #pragma warning restore 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table row group implementation // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // //--------------------------------------------------------------------------- //In order to avoid generating warnings about unknown message numbers and //unknown pragmas when compiling your C# source code with the actual C# compiler, //you need to disable warnings 1634 and 1691. (Presharp Documentation) #pragma warning disable 1634, 1691 using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Utility; using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Markup; using MS.Internal; using MS.Internal.Data; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Table row group implementation /// [ContentProperty("Rows")] public class TableRowGroup : TextElement, IAddChild { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an instance of a RowGroup /// public TableRowGroup() : base() { Initialize(); } // common initialization for all constructors private void Initialize() { _rows = new TableRowCollection(this); _rowInsertionIndex = -1; _parentIndex = -1; } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// void IAddChild.AddChild(object value) { if (value == null) { throw new ArgumentNullException("value"); } TableRow row = value as TableRow; if (row != null) { Rows.Add(row); return; } throw (new ArgumentException(SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(TableRow)), "value")); } ////// /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// Returns the row group's row collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public TableRowCollection Rows { get { return (_rows); } } ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeRows() { return Rows.Count > 0; } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion Protected Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the RowGroup about entering model tree. /// internal void OnEnterParentTree() { if(Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnAfterExitParentTree(Table table) { table.OnStructureChanged(); } ////// ValidateStructure /// internal void ValidateStructure() { RowSpanVector rowSpanVector = new RowSpanVector(); _columnCount = 0; for (int i = 0; i < Rows.Count; ++i) { Rows[i].ValidateStructure(rowSpanVector); _columnCount = Math.Max(_columnCount, Rows[i].ColumnCount); } Table.EnsureColumnCount(_columnCount); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Table owner accessor /// internal Table Table { get { return Parent as Table; } } ////// RowGroup's index in the parents row group collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// Stores temporary data for where to insert a new row /// internal int InsertionIndex { get { return _rowInsertionIndex; } set { _rowInsertionIndex = value; } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Called when body receives a new parent (via OM or text tree) /// /// /// New parent of body /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; if (newParent != null && !(newParent is Table)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if (oldParent != null) { OnExitParentTree(); ((Table)oldParent).RowGroups.InternalRemove(this); OnAfterExitParentTree(oldParent as Table); } base.OnNewParent(newParent); if (newParent != null) { ((Table)newParent).RowGroups.InternalAdd(this); OnEnterParentTree(); } } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private TableRowCollection _rows; // children rows store private int _parentIndex; // row group's index in parent's children collection private int _rowInsertionIndex; // Insertion index for row private int _columnCount; // Column count. #endregion Private Fields //------------------------------------------------------ // // Private Structures / Classes // //----------------------------------------------------- } } #pragma warning restore 1634, 1691 // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
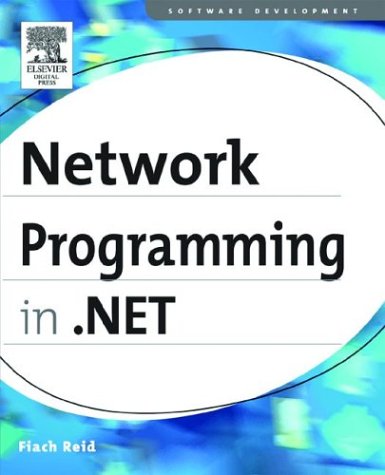
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EffectiveValueEntry.cs
- HandlerWithFactory.cs
- SoapBinding.cs
- CardSpacePolicyElement.cs
- RandomNumberGenerator.cs
- PrintPreviewGraphics.cs
- Main.cs
- MenuItem.cs
- Bold.cs
- WebPartConnectionsConfigureVerb.cs
- AccessControlEntry.cs
- RegexRunner.cs
- SystemIPv6InterfaceProperties.cs
- ObjectPropertyMapping.cs
- WebBrowserDesigner.cs
- QueryAccessibilityHelpEvent.cs
- SingleKeyFrameCollection.cs
- TypeCollectionDesigner.xaml.cs
- TabletDevice.cs
- VirtualDirectoryMappingCollection.cs
- MemberRelationshipService.cs
- DataMemberListEditor.cs
- RelationshipEndMember.cs
- DataGridViewCellStyleChangedEventArgs.cs
- CompatibleComparer.cs
- TransformerConfigurationWizardBase.cs
- _DisconnectOverlappedAsyncResult.cs
- KnownTypes.cs
- MinimizableAttributeTypeConverter.cs
- StringStorage.cs
- XmlResolver.cs
- ObjectContextServiceProvider.cs
- DbSourceCommand.cs
- HttpServerVarsCollection.cs
- DataGridCell.cs
- TriggerAction.cs
- TypeSystem.cs
- WpfWebRequestHelper.cs
- SelectionProcessor.cs
- ObjectList.cs
- LineServicesCallbacks.cs
- BinHexEncoder.cs
- XamlStackWriter.cs
- ControlParameter.cs
- TextTreeUndoUnit.cs
- KeyGestureConverter.cs
- HttpCacheVary.cs
- WaitForChangedResult.cs
- HtmlElementEventArgs.cs
- PeerResolverSettings.cs
- ExpandSegment.cs
- MimeTextImporter.cs
- HandlerFactoryCache.cs
- ListMarkerLine.cs
- WindowsScrollBarBits.cs
- EntityDataSourceViewSchema.cs
- SqlWebEventProvider.cs
- ErrorStyle.cs
- BaseDataList.cs
- FormView.cs
- Composition.cs
- HashCoreRequest.cs
- XmlSchemaNotation.cs
- DesignerImageAdapter.cs
- RegexWriter.cs
- TextTreeRootTextBlock.cs
- CompiledQuery.cs
- LinqDataSourceSelectEventArgs.cs
- DllNotFoundException.cs
- Page.cs
- ResolveCompletedEventArgs.cs
- HwndHostAutomationPeer.cs
- FixedTextContainer.cs
- EditBehavior.cs
- Metadata.cs
- StringDictionaryCodeDomSerializer.cs
- QueueProcessor.cs
- EventLogInformation.cs
- grammarelement.cs
- BaseTemplateBuildProvider.cs
- DataSetUtil.cs
- BaseValidator.cs
- TextBox.cs
- Menu.cs
- Pair.cs
- PerfCounters.cs
- RemotingConfigParser.cs
- DataGridViewHitTestInfo.cs
- DesignTimeVisibleAttribute.cs
- ServicePrincipalNameElement.cs
- ItemCollectionEditor.cs
- RestHandler.cs
- ChtmlTextWriter.cs
- ClientSettingsStore.cs
- DataSourceCache.cs
- CustomSignedXml.cs
- PeerCollaboration.cs
- TreeNode.cs
- SpellerInterop.cs
- GeometryGroup.cs