Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / DependencyPropertyHelper.cs / 1 / DependencyPropertyHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Helper class for miscellaneous framework-level features related // to DependencyProperties. // // See spec at [....]/sites/Avalon/Specs/GetValueSource.mht // //--------------------------------------------------------------------------- using System; namespace System.Windows { ////// Source of a DependencyProperty value. /// public enum BaseValueSource { ///The source is not known by the Framework. Unknown = BaseValueSourceInternal.Unknown, ///Default value, as defined by property metadata. Default = BaseValueSourceInternal.Default, ///Inherited from an ancestor. Inherited = BaseValueSourceInternal.Inherited, ///Default Style for the current theme. DefaultStyle = BaseValueSourceInternal.ThemeStyle, ///Trigger in the default Style for the current theme. DefaultStyleTrigger = BaseValueSourceInternal.ThemeStyleTrigger, ///Style setter. Style = BaseValueSourceInternal.Style, ///Trigger in the Template. TemplateTrigger = BaseValueSourceInternal.TemplateTrigger, ///Trigger in the Style. StyleTrigger = BaseValueSourceInternal.StyleTrigger, ///Implicit Style reference. ImplicitStyleReference = BaseValueSourceInternal.ImplicitReference, ///Template that created the element. ParentTemplate = BaseValueSourceInternal.ParentTemplate, ///Trigger in the Template that created the element. ParentTemplateTrigger = BaseValueSourceInternal.ParentTemplateTrigger, ///Local value. Local = BaseValueSourceInternal.Local, } ////// This struct contains the information returned from /// DependencyPropertyHelper.GetValueSource. /// public struct ValueSource { internal ValueSource(BaseValueSourceInternal source, bool isExpression, bool isAnimated, bool isCoerced) { // this cast is justified because the public BaseValueSource enum // values agree with the internal BaseValueSourceInternal enum values. _baseValueSource = (BaseValueSource)source; _isExpression = isExpression; _isAnimated = isAnimated; _isCoerced = isCoerced; } ////// The base value source. /// public BaseValueSource BaseValueSource { get { return _baseValueSource; } } ////// True if the value came from an Expression. /// public bool IsExpression { get { return _isExpression; } } ////// True if the value came from an animation. /// public bool IsAnimated { get { return _isAnimated; } } ////// True if the value was coerced. /// public bool IsCoerced { get { return _isCoerced; } } #region Object overrides - required by FxCop ////// Return the hash code for this ValueSource. /// public override int GetHashCode() { return _baseValueSource.GetHashCode(); } ////// True if this ValueSource equals the argument. /// public override bool Equals(object o) { if (o is ValueSource) { ValueSource that = (ValueSource)o; return this._baseValueSource == that._baseValueSource && this._isExpression == that._isExpression && this._isAnimated == that._isAnimated && this._isCoerced == that._isCoerced; } else { return false; } } ////// True if the two arguments are equal. /// public static bool operator==(ValueSource vs1, ValueSource vs2) { return vs1.Equals(vs2); } ////// True if the two arguments are unequal. /// public static bool operator!=(ValueSource vs1, ValueSource vs2) { return !vs1.Equals(vs2); } #endregion Object overrides - required by FxCop BaseValueSource _baseValueSource; bool _isExpression; bool _isAnimated; bool _isCoerced; } ////// Helper class for miscellaneous framework-level features related /// to DependencyProperties. /// public static class DependencyPropertyHelper { ////// Return the source of the value for the given property. /// public static ValueSource GetValueSource(DependencyObject dependencyObject, DependencyProperty dependencyProperty) { if (dependencyObject == null) throw new ArgumentNullException("dependencyObject"); if (dependencyProperty == null) throw new ArgumentNullException("dependencyProperty"); dependencyObject.VerifyAccess(); bool hasModifiers, isExpression, isAnimated, isCoerced; BaseValueSourceInternal source = dependencyObject.GetValueSource(dependencyProperty, null, out hasModifiers, out isExpression, out isAnimated, out isCoerced); return new ValueSource(source, isExpression, isAnimated, isCoerced); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
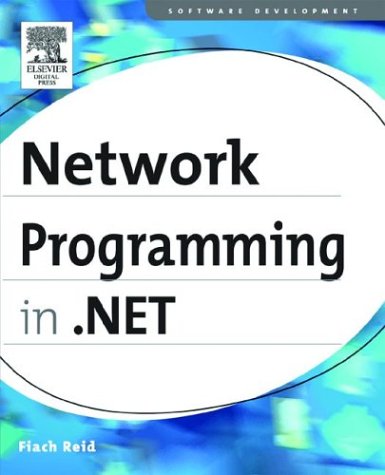
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IntSecurity.cs
- MsmqIntegrationInputChannel.cs
- PermissionRequestEvidence.cs
- DataSourceGroupCollection.cs
- FlowDocumentPage.cs
- Bold.cs
- WpfPayload.cs
- ProjectionCamera.cs
- LogicalExpr.cs
- SizeKeyFrameCollection.cs
- DocumentApplication.cs
- BooleanStorage.cs
- QueryStringParameter.cs
- BitmapDecoder.cs
- Encoder.cs
- _NegoStream.cs
- TracedNativeMethods.cs
- HttpCapabilitiesEvaluator.cs
- CallContext.cs
- DataGridViewColumnEventArgs.cs
- PersonalizationState.cs
- SchemaManager.cs
- ParseChildrenAsPropertiesAttribute.cs
- RemoteWebConfigurationHostServer.cs
- RequestCacheManager.cs
- CqlErrorHelper.cs
- SelectedPathEditor.cs
- Interlocked.cs
- InteropAutomationProvider.cs
- ConnectionPoint.cs
- BindingList.cs
- ReturnValue.cs
- FormattedTextSymbols.cs
- AlternateView.cs
- BigInt.cs
- ErrorHandler.cs
- XmlSchemaImporter.cs
- TCPListener.cs
- ObjectContext.cs
- DescendantQuery.cs
- VersionedStream.cs
- ExternalException.cs
- HandledMouseEvent.cs
- TextEndOfParagraph.cs
- CallSiteBinder.cs
- CustomCategoryAttribute.cs
- QuadraticBezierSegment.cs
- VectorAnimationUsingKeyFrames.cs
- XamlDesignerSerializationManager.cs
- ExecutionContext.cs
- TrustLevel.cs
- CurrencyWrapper.cs
- PerformanceCounter.cs
- SmtpClient.cs
- GroupAggregateExpr.cs
- PointValueSerializer.cs
- WebDescriptionAttribute.cs
- ToolStripItemEventArgs.cs
- ThreadPoolTaskScheduler.cs
- PenLineCapValidation.cs
- SrgsOneOf.cs
- MessageQueueKey.cs
- ResourceAssociationTypeEnd.cs
- ErrorRuntimeConfig.cs
- DesigntimeLicenseContext.cs
- SqlMethodCallConverter.cs
- ChineseLunisolarCalendar.cs
- Helpers.cs
- BaseWebProxyFinder.cs
- ProcessModuleCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- keycontainerpermission.cs
- SoapSchemaImporter.cs
- DrawItemEvent.cs
- DSGeneratorProblem.cs
- WithStatement.cs
- IdnElement.cs
- ExtentKey.cs
- TriState.cs
- LinearQuaternionKeyFrame.cs
- ResXFileRef.cs
- CodeTypeReference.cs
- WindowsTitleBar.cs
- RuleElement.cs
- KeysConverter.cs
- RowsCopiedEventArgs.cs
- SiteMapNodeItem.cs
- TextBoxBase.cs
- PartManifestEntry.cs
- DataTable.cs
- DomainUpDown.cs
- NativeMethods.cs
- DeobfuscatingStream.cs
- UiaCoreApi.cs
- base64Transforms.cs
- _ServiceNameStore.cs
- AtomMaterializer.cs
- ErrorFormatter.cs
- Vector3DAnimationBase.cs
- HtmlGenericControl.cs