Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Cache / RequestCacheManager.cs / 1 / RequestCacheManager.cs
/*++ Copyright (c) Microsoft Corporation Module Name: RequestCacheManager.cs Abstract: The class implements the app domain wide configuration for request cache aware clients Author: Alexei Vopilov 21-Dec-2002 Revision History: Jan 25 2004 - Changed the visibility of the class from public to internal, compressed unused logic. --*/ namespace System.Net.Cache { using System; using System.Collections; using System.Net.Configuration; using System.Configuration; ///Specifies app domain-wide default settings for request caching internal sealed class RequestCacheManager { private RequestCacheManager() {} private static RequestCachingSectionInternal s_CacheConfigSettings; private static readonly RequestCacheBinding s_BypassCacheBinding = new RequestCacheBinding (null, null, new RequestCachePolicy(RequestCacheLevel.BypassCache)); private static RequestCacheBinding s_DefaultGlobalBinding; private static RequestCacheBinding s_DefaultHttpBinding; private static RequestCacheBinding s_DefaultFtpBinding; // // ATN: the caller MUST use uri.Scheme as a parameter, otheriwse cannot do schme ref comparision // internal static RequestCacheBinding GetBinding(string internedScheme) { if (internedScheme == null) throw new ArgumentNullException("uriScheme"); if (s_CacheConfigSettings == null) LoadConfigSettings(); if(s_CacheConfigSettings.DisableAllCaching) return s_BypassCacheBinding; if (internedScheme.Length == 0) return s_DefaultGlobalBinding; if ((object)internedScheme == (object)Uri.UriSchemeHttp || (object)internedScheme == (object)Uri.UriSchemeHttps) return s_DefaultHttpBinding; if ((object)internedScheme == (object)Uri.UriSchemeFtp) return s_DefaultFtpBinding; return s_BypassCacheBinding; } internal static bool IsCachingEnabled { get { if (s_CacheConfigSettings == null) LoadConfigSettings(); return !s_CacheConfigSettings.DisableAllCaching; } } // internal static void SetBinding(string uriScheme, RequestCacheBinding binding) { if (uriScheme == null) throw new ArgumentNullException("uriScheme"); if (s_CacheConfigSettings == null) LoadConfigSettings(); if(s_CacheConfigSettings.DisableAllCaching) return; if (uriScheme.Length == 0) s_DefaultGlobalBinding = binding; else if (uriScheme == Uri.UriSchemeHttp || uriScheme == Uri.UriSchemeHttps) s_DefaultHttpBinding = binding; else if (uriScheme == Uri.UriSchemeFtp) s_DefaultFtpBinding = binding; } // private static void LoadConfigSettings() { // Any concurent access shall go here and block until we've grabbed the config settings lock (s_BypassCacheBinding) { if (s_CacheConfigSettings == null) { RequestCachingSectionInternal settings = RequestCachingSectionInternal.GetSection(); s_DefaultGlobalBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultHttpValidator, settings.DefaultCachePolicy); s_DefaultHttpBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultHttpValidator, settings.DefaultHttpCachePolicy); s_DefaultFtpBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultFtpValidator, settings.DefaultFtpCachePolicy); s_CacheConfigSettings = settings; } } } } // // internal class RequestCacheBinding { private RequestCache m_RequestCache; private RequestCacheValidator m_CacheValidator; private RequestCachePolicy m_Policy; internal RequestCacheBinding (RequestCache requestCache, RequestCacheValidator cacheValidator, RequestCachePolicy policy) { m_RequestCache = requestCache; m_CacheValidator = cacheValidator; m_Policy = policy; } internal RequestCache Cache { get {return m_RequestCache;} } internal RequestCacheValidator Validator { get {return m_CacheValidator;} } internal RequestCachePolicy Policy { get {return m_Policy;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /*++ Copyright (c) Microsoft Corporation Module Name: RequestCacheManager.cs Abstract: The class implements the app domain wide configuration for request cache aware clients Author: Alexei Vopilov 21-Dec-2002 Revision History: Jan 25 2004 - Changed the visibility of the class from public to internal, compressed unused logic. --*/ namespace System.Net.Cache { using System; using System.Collections; using System.Net.Configuration; using System.Configuration; ///Specifies app domain-wide default settings for request caching internal sealed class RequestCacheManager { private RequestCacheManager() {} private static RequestCachingSectionInternal s_CacheConfigSettings; private static readonly RequestCacheBinding s_BypassCacheBinding = new RequestCacheBinding (null, null, new RequestCachePolicy(RequestCacheLevel.BypassCache)); private static RequestCacheBinding s_DefaultGlobalBinding; private static RequestCacheBinding s_DefaultHttpBinding; private static RequestCacheBinding s_DefaultFtpBinding; // // ATN: the caller MUST use uri.Scheme as a parameter, otheriwse cannot do schme ref comparision // internal static RequestCacheBinding GetBinding(string internedScheme) { if (internedScheme == null) throw new ArgumentNullException("uriScheme"); if (s_CacheConfigSettings == null) LoadConfigSettings(); if(s_CacheConfigSettings.DisableAllCaching) return s_BypassCacheBinding; if (internedScheme.Length == 0) return s_DefaultGlobalBinding; if ((object)internedScheme == (object)Uri.UriSchemeHttp || (object)internedScheme == (object)Uri.UriSchemeHttps) return s_DefaultHttpBinding; if ((object)internedScheme == (object)Uri.UriSchemeFtp) return s_DefaultFtpBinding; return s_BypassCacheBinding; } internal static bool IsCachingEnabled { get { if (s_CacheConfigSettings == null) LoadConfigSettings(); return !s_CacheConfigSettings.DisableAllCaching; } } // internal static void SetBinding(string uriScheme, RequestCacheBinding binding) { if (uriScheme == null) throw new ArgumentNullException("uriScheme"); if (s_CacheConfigSettings == null) LoadConfigSettings(); if(s_CacheConfigSettings.DisableAllCaching) return; if (uriScheme.Length == 0) s_DefaultGlobalBinding = binding; else if (uriScheme == Uri.UriSchemeHttp || uriScheme == Uri.UriSchemeHttps) s_DefaultHttpBinding = binding; else if (uriScheme == Uri.UriSchemeFtp) s_DefaultFtpBinding = binding; } // private static void LoadConfigSettings() { // Any concurent access shall go here and block until we've grabbed the config settings lock (s_BypassCacheBinding) { if (s_CacheConfigSettings == null) { RequestCachingSectionInternal settings = RequestCachingSectionInternal.GetSection(); s_DefaultGlobalBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultHttpValidator, settings.DefaultCachePolicy); s_DefaultHttpBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultHttpValidator, settings.DefaultHttpCachePolicy); s_DefaultFtpBinding = new RequestCacheBinding (settings.DefaultCache, settings.DefaultFtpValidator, settings.DefaultFtpCachePolicy); s_CacheConfigSettings = settings; } } } } // // internal class RequestCacheBinding { private RequestCache m_RequestCache; private RequestCacheValidator m_CacheValidator; private RequestCachePolicy m_Policy; internal RequestCacheBinding (RequestCache requestCache, RequestCacheValidator cacheValidator, RequestCachePolicy policy) { m_RequestCache = requestCache; m_CacheValidator = cacheValidator; m_Policy = policy; } internal RequestCache Cache { get {return m_RequestCache;} } internal RequestCacheValidator Validator { get {return m_CacheValidator;} } internal RequestCachePolicy Policy { get {return m_Policy;} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
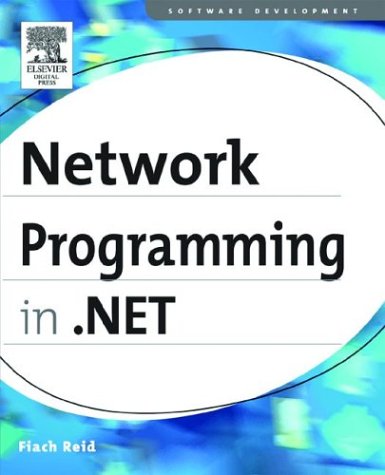
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CipherData.cs
- HeaderPanel.cs
- BooleanExpr.cs
- PassportAuthenticationEventArgs.cs
- DefaultSection.cs
- AddressHeaderCollectionElement.cs
- ShaderEffect.cs
- InputProcessorProfiles.cs
- ActivityXRefConverter.cs
- PassportAuthentication.cs
- AnnotationElement.cs
- Table.cs
- SchemaNamespaceManager.cs
- UnlockCardRequest.cs
- FieldInfo.cs
- ResourceCategoryAttribute.cs
- WindowsFormsHelpers.cs
- SiteMapSection.cs
- InputChannelBinder.cs
- WebConfigurationFileMap.cs
- StateItem.cs
- CodeTypeReferenceExpression.cs
- MetricEntry.cs
- PasswordDeriveBytes.cs
- RegexCompiler.cs
- TakeOrSkipWhileQueryOperator.cs
- HttpPostedFile.cs
- StringUtil.cs
- SapiAttributeParser.cs
- WindowsAltTab.cs
- ProgressPage.cs
- ReplyChannelAcceptor.cs
- ClrPerspective.cs
- WebBaseEventKeyComparer.cs
- Vector.cs
- StrongNameHelpers.cs
- QilParameter.cs
- XmlSchemaGroupRef.cs
- CurrentChangingEventArgs.cs
- ObjectTag.cs
- DefaultAsyncDataDispatcher.cs
- EntityUtil.cs
- TemplateInstanceAttribute.cs
- IdleTimeoutMonitor.cs
- UrlAuthorizationModule.cs
- ObjectTag.cs
- CacheChildrenQuery.cs
- ServiceAppDomainAssociationProvider.cs
- DataControlPagerLinkButton.cs
- VirtualizingPanel.cs
- StylusDownEventArgs.cs
- VisualCollection.cs
- ConvertersCollection.cs
- Visual.cs
- StringBuilder.cs
- RoleGroupCollection.cs
- FactorySettingsElement.cs
- WebServiceResponseDesigner.cs
- SafeLocalMemHandle.cs
- MethodToken.cs
- DefaultEventAttribute.cs
- ExpressionPrefixAttribute.cs
- ReflectionPermission.cs
- StringAnimationBase.cs
- OutputCacheSettings.cs
- FragmentNavigationEventArgs.cs
- PropertyIDSet.cs
- PersonalizableAttribute.cs
- Token.cs
- SystemFonts.cs
- FileAccessException.cs
- OutputCacheSection.cs
- CheckBoxStandardAdapter.cs
- ListViewItem.cs
- SiteMapProvider.cs
- ParseNumbers.cs
- DateTimeOffset.cs
- ManifestSignatureInformation.cs
- MetabaseServerConfig.cs
- CharacterBuffer.cs
- ObjectDataSource.cs
- NamespaceList.cs
- DataDocumentXPathNavigator.cs
- ConfigurationPropertyCollection.cs
- SQLDecimal.cs
- CodeAttributeArgument.cs
- AsymmetricSignatureFormatter.cs
- IndexingContentUnit.cs
- AbstractExpressions.cs
- UIElement.cs
- StandardCommands.cs
- GroupPartitionExpr.cs
- WizardStepCollectionEditor.cs
- ListItemDetailViewAttribute.cs
- DisplayInformation.cs
- RepeaterItem.cs
- TableLayoutPanelCodeDomSerializer.cs
- ZipFileInfo.cs
- contentDescriptor.cs
- DataGridViewColumnCollectionEditor.cs