Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Win32 / SafeHandles / BCryptSafeHandles.cs / 1305376 / BCryptSafeHandles.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Diagnostics.Contracts; namespace Microsoft.Win32.SafeHandles { ////// SafeHandle representing a BCRYPT_ALG_HANDLE /// //// #pragma warning disable 618 // Have not migrated to v4 transparency yet [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] #pragma warning restore 618 internal sealed class SafeBCryptAlgorithmHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeBCryptAlgorithmHandle() : base(true) { } [DllImport("bcrypt")] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern BCryptNative.ErrorCode BCryptCloseAlgorithmProvider(IntPtr hAlgorithm, int flags); protected override bool ReleaseHandle() { return BCryptCloseAlgorithmProvider(handle, 0) == BCryptNative.ErrorCode.Success; } } ///// /// Safe handle representing a BCRYPT_HASH_HANDLE and the associated buffer holding the hash object /// //// #pragma warning disable 618 // Have not migrated to v4 transparency yet [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] #pragma warning restore 618 internal sealed class SafeBCryptHashHandle : SafeHandleZeroOrMinusOneIsInvalid { private IntPtr m_hashObject; private SafeBCryptHashHandle() : base(true) { } ///// /// Buffer holding the hash object. This buffer should be allocated with Marshal.AllocCoTaskMem. /// internal IntPtr HashObject { get { return m_hashObject; } set { Contract.Requires(value != IntPtr.Zero); m_hashObject = value; } } [DllImport("bcrypt")] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern BCryptNative.ErrorCode BCryptDestroyHash(IntPtr hHash); protected override bool ReleaseHandle() { bool success = BCryptDestroyHash(handle) == BCryptNative.ErrorCode.Success; // The hash object buffer must be released only after destroying the hash handle if (m_hashObject != IntPtr.Zero) { Marshal.FreeCoTaskMem(m_hashObject); } return success; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security; using System.Security.Cryptography; using System.Diagnostics.Contracts; namespace Microsoft.Win32.SafeHandles { ////// SafeHandle representing a BCRYPT_ALG_HANDLE /// //// #pragma warning disable 618 // Have not migrated to v4 transparency yet [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] #pragma warning restore 618 internal sealed class SafeBCryptAlgorithmHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeBCryptAlgorithmHandle() : base(true) { } [DllImport("bcrypt")] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern BCryptNative.ErrorCode BCryptCloseAlgorithmProvider(IntPtr hAlgorithm, int flags); protected override bool ReleaseHandle() { return BCryptCloseAlgorithmProvider(handle, 0) == BCryptNative.ErrorCode.Success; } } ///// /// Safe handle representing a BCRYPT_HASH_HANDLE and the associated buffer holding the hash object /// //// #pragma warning disable 618 // Have not migrated to v4 transparency yet [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] #pragma warning restore 618 internal sealed class SafeBCryptHashHandle : SafeHandleZeroOrMinusOneIsInvalid { private IntPtr m_hashObject; private SafeBCryptHashHandle() : base(true) { } ///// /// Buffer holding the hash object. This buffer should be allocated with Marshal.AllocCoTaskMem. /// internal IntPtr HashObject { get { return m_hashObject; } set { Contract.Requires(value != IntPtr.Zero); m_hashObject = value; } } [DllImport("bcrypt")] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [SuppressUnmanagedCodeSecurity] private static extern BCryptNative.ErrorCode BCryptDestroyHash(IntPtr hHash); protected override bool ReleaseHandle() { bool success = BCryptDestroyHash(handle) == BCryptNative.ErrorCode.Success; // The hash object buffer must be released only after destroying the hash handle if (m_hashObject != IntPtr.Zero) { Marshal.FreeCoTaskMem(m_hashObject); } return success; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
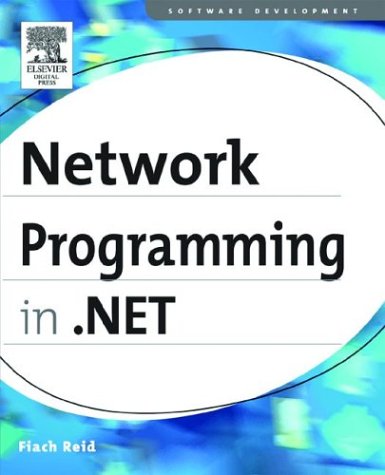
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReceiveActivity.cs
- XmlConvert.cs
- DelegatingTypeDescriptionProvider.cs
- ToolStripDesignerAvailabilityAttribute.cs
- ListViewTableCell.cs
- TextWriterTraceListener.cs
- WebPartHeaderCloseVerb.cs
- SoapAttributeOverrides.cs
- HtmlLink.cs
- TextEditorThreadLocalStore.cs
- DesignerSelectionListAdapter.cs
- ActivityExecutorDelegateInfo.cs
- JsonWriter.cs
- EdmSchemaAttribute.cs
- FileDialog_Vista.cs
- RequestQueue.cs
- DrawingServices.cs
- DataFieldCollectionEditor.cs
- Wildcard.cs
- EntityModelBuildProvider.cs
- CommonDialog.cs
- DropShadowBitmapEffect.cs
- Tablet.cs
- SourceFileBuildProvider.cs
- cache.cs
- OdbcConnectionPoolProviderInfo.cs
- X509CertificateValidator.cs
- OutputCacheSection.cs
- PropertyIDSet.cs
- InvalidPrinterException.cs
- Schedule.cs
- HeaderedItemsControl.cs
- XmlWellformedWriter.cs
- DesignerView.cs
- DataObjectMethodAttribute.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- NativeWindow.cs
- SqlNodeAnnotations.cs
- sqlmetadatafactory.cs
- Types.cs
- PageThemeBuildProvider.cs
- _FtpControlStream.cs
- RefreshPropertiesAttribute.cs
- ObjectDataSourceDisposingEventArgs.cs
- COM2Properties.cs
- ObservableDictionary.cs
- MenuCommand.cs
- DataSvcMapFileSerializer.cs
- translator.cs
- RelationshipManager.cs
- FrameworkContextData.cs
- X509ClientCertificateAuthentication.cs
- CellNormalizer.cs
- UserInitiatedNavigationPermission.cs
- WizardForm.cs
- InvalidDocumentContentsException.cs
- ColorBuilder.cs
- MD5CryptoServiceProvider.cs
- XmlAnyElementAttributes.cs
- AppDomain.cs
- BitVector32.cs
- FixedSOMFixedBlock.cs
- HWStack.cs
- SymDocumentType.cs
- EntitySqlQueryCacheKey.cs
- BuildResultCache.cs
- WebConfigurationManager.cs
- MarkupCompilePass2.cs
- WorkflowMarkupSerializationException.cs
- WebScriptMetadataMessage.cs
- ScalarConstant.cs
- DataGridComboBoxColumn.cs
- WebPartZoneBase.cs
- LinqDataSourceHelper.cs
- UIElementParaClient.cs
- VBCodeProvider.cs
- PreloadedPackages.cs
- StringBuilder.cs
- DataGridViewAccessibleObject.cs
- Int32Rect.cs
- ExclusiveNamedPipeTransportManager.cs
- ContextMenuAutomationPeer.cs
- ProtectedConfigurationProviderCollection.cs
- DockAndAnchorLayout.cs
- MatchNoneMessageFilter.cs
- MexTcpBindingElement.cs
- UIElementPropertyUndoUnit.cs
- DesignerActionKeyboardBehavior.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- EntityDataSourceReferenceGroup.cs
- ListViewTableRow.cs
- TraceSwitch.cs
- TokenizerHelper.cs
- NativeMethods.cs
- SelectorItemAutomationPeer.cs
- InfoCardBaseException.cs
- SessionPageStateSection.cs
- DynamicHyperLink.cs
- CredentialCache.cs
- RuntimeHandles.cs