Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Policy / FirstMatchCodeGroup.cs / 1 / FirstMatchCodeGroup.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // FirstMatchCodeGroup.cs // // Representation for code groups used for the policy mechanism // namespace System.Security.Policy { using System; using System.Security.Util; using System.Security; using System.Collections; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class FirstMatchCodeGroup : CodeGroup { internal FirstMatchCodeGroup() : base() { } public FirstMatchCodeGroup( IMembershipCondition membershipCondition, PolicyStatement policy ) : base( membershipCondition, policy ) { } public override PolicyStatement Resolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { PolicyStatement childPolicy = null; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { childPolicy = ((CodeGroup)enumerator.Current).Resolve( evidence ); // If the child has a policy, we are done. if (childPolicy != null) break; } PolicyStatement thisPolicy = this.PolicyStatement; if (thisPolicy == null) { return childPolicy; } else if (childPolicy != null) { // Combine the child and this policy and return it. PolicyStatement combined = new PolicyStatement(); combined.SetPermissionSetNoCopy( thisPolicy.GetPermissionSetNoCopy().Union( childPolicy.GetPermissionSetNoCopy() ) ); // if both this group and matching child group are exclusive we need to throw an exception if (((thisPolicy.Attributes & childPolicy.Attributes) & PolicyStatementAttribute.Exclusive) == PolicyStatementAttribute.Exclusive) throw new PolicyException( Environment.GetResourceString( "Policy_MultipleExclusive" ) ); combined.Attributes = thisPolicy.Attributes | childPolicy.Attributes; return combined; } else { // Otherwise we just copy the this policy. return this.PolicyStatement; } } else { return null; } } public override CodeGroup ResolveMatchingCodeGroups( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); if (this.MembershipCondition.Check( evidence )) { CodeGroup retGroup = this.Copy(); retGroup.Children = new ArrayList(); IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { CodeGroup matchingGroups = ((CodeGroup)enumerator.Current).ResolveMatchingCodeGroups( evidence ); // If the child has a policy, we are done. if (matchingGroups != null) { retGroup.AddChild( matchingGroups ); break; } } return retGroup; } else { return null; } } public override CodeGroup Copy() { FirstMatchCodeGroup group = new FirstMatchCodeGroup(); group.MembershipCondition = this.MembershipCondition; group.PolicyStatement = this.PolicyStatement; group.Name = this.Name; group.Description = this.Description; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { group.AddChild( (CodeGroup)enumerator.Current ); } return group; } public override String MergeLogic { get { return Environment.GetResourceString( "MergeLogic_FirstMatch" ); } } internal override String GetTypeName() { return "System.Security.Policy.FirstMatchCodeGroup"; } } }
Link Menu
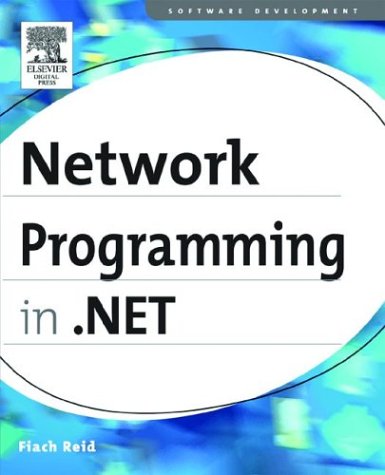
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Visual.cs
- ProviderException.cs
- StylusShape.cs
- TraceListener.cs
- RadioButtonStandardAdapter.cs
- bidPrivateBase.cs
- SecurityDescriptor.cs
- newinstructionaction.cs
- XmlCustomFormatter.cs
- CodeValidator.cs
- RtfToken.cs
- ResXFileRef.cs
- CapabilitiesUse.cs
- InstanceStore.cs
- PluralizationService.cs
- SynchronizedChannelCollection.cs
- DataTableCollection.cs
- MaterialCollection.cs
- TextBreakpoint.cs
- StateInitialization.cs
- PartialTrustVisibleAssembliesSection.cs
- XmlCharacterData.cs
- CodeMethodInvokeExpression.cs
- TransportationConfigurationTypeInstallComponent.cs
- GPStream.cs
- ReadOnlyHierarchicalDataSourceView.cs
- BufferedStream.cs
- AtomServiceDocumentSerializer.cs
- DataGridViewCellStyleEditor.cs
- RadioButton.cs
- DataKeyPropertyAttribute.cs
- SoapMessage.cs
- OuterGlowBitmapEffect.cs
- DataGridViewTopLeftHeaderCell.cs
- UpdatePanelTrigger.cs
- GraphicsContext.cs
- ContentPosition.cs
- TextStore.cs
- Polyline.cs
- PointLight.cs
- MachineKeySection.cs
- Compiler.cs
- ExchangeUtilities.cs
- XmlImplementation.cs
- XmlEntity.cs
- _StreamFramer.cs
- UInt64.cs
- ParserExtension.cs
- DocumentViewerBase.cs
- Route.cs
- XmlIncludeAttribute.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- PseudoWebRequest.cs
- PropertyIDSet.cs
- RightsManagementInformation.cs
- AbsoluteQuery.cs
- LinqMaximalSubtreeNominator.cs
- XmlChoiceIdentifierAttribute.cs
- Validator.cs
- AsnEncodedData.cs
- sqlnorm.cs
- Line.cs
- BezierSegment.cs
- CategoryAttribute.cs
- SmtpNtlmAuthenticationModule.cs
- CorrelationManager.cs
- SafeRightsManagementQueryHandle.cs
- WebContentFormatHelper.cs
- DataFormats.cs
- AccessDataSourceView.cs
- RepeatButton.cs
- EpmSourcePathSegment.cs
- TextBox.cs
- OpCodes.cs
- Aes.cs
- ThousandthOfEmRealPoints.cs
- _emptywebproxy.cs
- EntityViewContainer.cs
- BindingsCollection.cs
- XPathMultyIterator.cs
- ScopeElementCollection.cs
- Tablet.cs
- SqlConnectionFactory.cs
- WebPartConnectionsEventArgs.cs
- XMLSchema.cs
- ProfileSection.cs
- ExpressionParser.cs
- ObjectConverter.cs
- JpegBitmapEncoder.cs
- HelpKeywordAttribute.cs
- TrackBarRenderer.cs
- RepeaterItem.cs
- ImpersonationContext.cs
- IPHostEntry.cs
- DelegatingTypeDescriptionProvider.cs
- TCEAdapterGenerator.cs
- ProfilePropertySettingsCollection.cs
- XmlObjectSerializer.cs
- XamlPointCollectionSerializer.cs
- TdsParserStaticMethods.cs