Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Shapes / Line.cs / 1 / Line.cs
//---------------------------------------------------------------------------- // File: Line.cs // // Description: // Implementation of Line shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The line shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Line : Shape { #region Constructors ////// Instantiates a new instance of a line. /// public Line() { } #endregion Constructors #region Dynamic Properties ////// X1 property /// public static readonly DependencyProperty X1Property = DependencyProperty.Register( "X1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X1 property /// [TypeConverter(typeof(LengthConverter))] public double X1 { get { return (double)GetValue(X1Property); } set { SetValue(X1Property, value); } } ////// Y1 property /// public static readonly DependencyProperty Y1Property = DependencyProperty.Register( "Y1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y1 property /// [TypeConverter(typeof(LengthConverter))] public double Y1 { get { return (double)GetValue(Y1Property); } set { SetValue(Y1Property, value); } } ////// X2 property /// public static readonly DependencyProperty X2Property = DependencyProperty.Register( "X2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X2 property /// [TypeConverter(typeof(LengthConverter))] public double X2 { get { return (double)GetValue(X2Property); } set { SetValue(X2Property, value); } } ////// Y2 property /// public static readonly DependencyProperty Y2Property = DependencyProperty.Register( "Y2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y2 property /// [TypeConverter(typeof(LengthConverter))] public double Y2 { get { return (double)GetValue(Y2Property); } set { SetValue(Y2Property, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the line that defines this shape /// protected override Geometry DefiningGeometry { get { return _lineGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { Point point1 = new Point(X1, Y1); Point point2 = new Point(X2, Y2); // Create the Line geometry _lineGeometry = new LineGeometry(point1, point2); } #endregion Internal Methods #region Private Methods and Members private LineGeometry _lineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Line.cs // // Description: // Implementation of Line shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The line shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Line : Shape { #region Constructors ////// Instantiates a new instance of a line. /// public Line() { } #endregion Constructors #region Dynamic Properties ////// X1 property /// public static readonly DependencyProperty X1Property = DependencyProperty.Register( "X1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X1 property /// [TypeConverter(typeof(LengthConverter))] public double X1 { get { return (double)GetValue(X1Property); } set { SetValue(X1Property, value); } } ////// Y1 property /// public static readonly DependencyProperty Y1Property = DependencyProperty.Register( "Y1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y1 property /// [TypeConverter(typeof(LengthConverter))] public double Y1 { get { return (double)GetValue(Y1Property); } set { SetValue(Y1Property, value); } } ////// X2 property /// public static readonly DependencyProperty X2Property = DependencyProperty.Register( "X2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X2 property /// [TypeConverter(typeof(LengthConverter))] public double X2 { get { return (double)GetValue(X2Property); } set { SetValue(X2Property, value); } } ////// Y2 property /// public static readonly DependencyProperty Y2Property = DependencyProperty.Register( "Y2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y2 property /// [TypeConverter(typeof(LengthConverter))] public double Y2 { get { return (double)GetValue(Y2Property); } set { SetValue(Y2Property, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the line that defines this shape /// protected override Geometry DefiningGeometry { get { return _lineGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { Point point1 = new Point(X1, Y1); Point point2 = new Point(X2, Y2); // Create the Line geometry _lineGeometry = new LineGeometry(point1, point2); } #endregion Internal Methods #region Private Methods and Members private LineGeometry _lineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
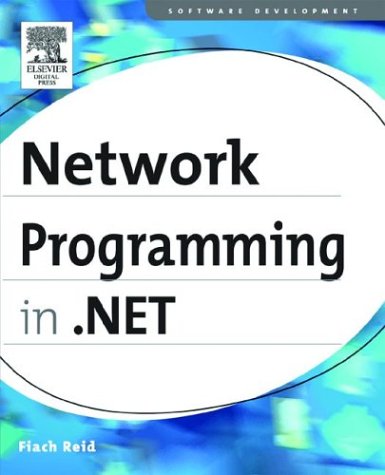
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpCapabilitiesBase.cs
- UrlPath.cs
- DataException.cs
- BaseResourcesBuildProvider.cs
- XpsS0ValidatingLoader.cs
- CodeConditionStatement.cs
- EFAssociationProvider.cs
- WCFServiceClientProxyGenerator.cs
- ProfessionalColorTable.cs
- listitem.cs
- IPPacketInformation.cs
- TextRangeEdit.cs
- RequestCacheValidator.cs
- CdpEqualityComparer.cs
- SecurityUtils.cs
- ScrollProperties.cs
- BlockUIContainer.cs
- Binding.cs
- OledbConnectionStringbuilder.cs
- FullTextBreakpoint.cs
- TreeNodeSelectionProcessor.cs
- TextEndOfSegment.cs
- ListItemConverter.cs
- KeyValuePairs.cs
- FixedPosition.cs
- GACMembershipCondition.cs
- NumberFormatInfo.cs
- PriorityBindingExpression.cs
- ProfileManager.cs
- VirtualDirectoryMapping.cs
- AstNode.cs
- Ops.cs
- CacheVirtualItemsEvent.cs
- EmptyControlCollection.cs
- ListenerPerfCounters.cs
- FontFamilyIdentifier.cs
- PrinterSettings.cs
- PublisherIdentityPermission.cs
- EncoderReplacementFallback.cs
- HtmlTextArea.cs
- ConnectorDragDropGlyph.cs
- ArrayTypeMismatchException.cs
- ProviderException.cs
- BaseCollection.cs
- EtwTrackingParticipant.cs
- SharedConnectionInfo.cs
- DesignTimeValidationFeature.cs
- WebPartUtil.cs
- ResourceDictionary.cs
- ArgIterator.cs
- Bitmap.cs
- DoubleKeyFrameCollection.cs
- MachineKeyValidationConverter.cs
- EntityType.cs
- Control.cs
- ConfigXmlText.cs
- ImageDrawing.cs
- VirtualDirectoryMappingCollection.cs
- ActiveDocumentEvent.cs
- FontFamilyValueSerializer.cs
- TemplateXamlParser.cs
- ImportDesigner.xaml.cs
- LocalizabilityAttribute.cs
- SHA512Managed.cs
- TextRangeEditTables.cs
- PropertyPathWorker.cs
- DataViewManagerListItemTypeDescriptor.cs
- RequestValidator.cs
- OpacityConverter.cs
- JoinElimination.cs
- GridLengthConverter.cs
- FrameworkElementFactory.cs
- webclient.cs
- WebPartConnectionsConnectVerb.cs
- CatalogZoneDesigner.cs
- MenuItemBindingCollection.cs
- SmiMetaDataProperty.cs
- ExtensionDataReader.cs
- GridSplitter.cs
- ClientSettingsProvider.cs
- ImplicitInputBrush.cs
- MarshalByRefObject.cs
- ItemDragEvent.cs
- JulianCalendar.cs
- TabItemAutomationPeer.cs
- HasCopySemanticsAttribute.cs
- CompositeCollectionView.cs
- ArglessEventHandlerProxy.cs
- BaseParser.cs
- SQLByteStorage.cs
- FamilyTypefaceCollection.cs
- CompositionAdorner.cs
- ColorTransform.cs
- ConstraintConverter.cs
- DataTableReaderListener.cs
- MembershipPasswordException.cs
- Vector3DKeyFrameCollection.cs
- EntityDataSourceColumn.cs
- UInt64Converter.cs
- _NestedMultipleAsyncResult.cs