Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Util / RequestValidator.cs / 1305376 / RequestValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Base class providing extensibility hooks for custom request validation * * Copyright (c) 2009 Microsoft Corporation */ namespace System.Web.Util { using System; using System.Diagnostics.CodeAnalysis; using System.Threading; using System.Web; using System.Web.Configuration; public class RequestValidator { private static RequestValidator _customValidator; private static readonly Lazy_customValidatorResolver = new Lazy (GetCustomValidatorFromConfig); public static RequestValidator Current { get { if (_customValidator == null) { _customValidator = _customValidatorResolver.Value; } return _customValidator; } set { if (value == null) { throw new ArgumentNullException("value"); } _customValidator = value; } } private static RequestValidator GetCustomValidatorFromConfig() { // App since this is static per AppDomain RuntimeConfig config = RuntimeConfig.GetAppConfig(); HttpRuntimeSection runtimeSection = config.HttpRuntime; string validatorTypeName = runtimeSection.RequestValidationType; // validate the type Type validatorType = ConfigUtil.GetType(validatorTypeName, "requestValidationType", runtimeSection); ConfigUtil.CheckBaseType(typeof(RequestValidator) /* expectedBaseType */, validatorType, "requestValidationType", runtimeSection); // instantiate RequestValidator validator = (RequestValidator)HttpRuntime.CreatePublicInstance(validatorType); return validator; } internal static void InitializeOnFirstRequest() { // instantiate the validator if it hasn't already been created RequestValidator validator = _customValidatorResolver.Value; } private static bool IsAtoZ(char c) { return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z'); } [SuppressMessage("Microsoft.Design", "CA1021:AvoidOutParameters", Justification = "This is an appropriate way to return multiple pieces of data.")] protected internal virtual bool IsValidRequestString(HttpContext context, string value, RequestValidationSource requestValidationSource, string collectionKey, out int validationFailureIndex) { if (requestValidationSource == RequestValidationSource.Headers) { validationFailureIndex = 0; return true; // Ignore Headers collection in the default implementation } return !CrossSiteScriptingValidation.IsDangerousString(value, out validationFailureIndex); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
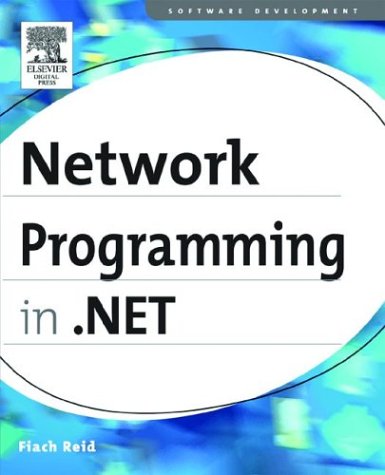
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeView.cs
- ListenerPerfCounters.cs
- XmlHelper.cs
- Int32Collection.cs
- ListBindingConverter.cs
- MailSettingsSection.cs
- ApplicationException.cs
- AVElementHelper.cs
- OleDbErrorCollection.cs
- ZipIOLocalFileDataDescriptor.cs
- Internal.cs
- WpfGeneratedKnownProperties.cs
- ObjectView.cs
- ToolStripSeparatorRenderEventArgs.cs
- WorkflowOwnerAsyncResult.cs
- EFTableProvider.cs
- Regex.cs
- PatternMatcher.cs
- DeliveryStrategy.cs
- ScriptReferenceBase.cs
- Asn1IntegerConverter.cs
- UserUseLicenseDictionaryLoader.cs
- AtomServiceDocumentSerializer.cs
- SafeThreadHandle.cs
- XmlMemberMapping.cs
- BindingsCollection.cs
- WebServiceHandlerFactory.cs
- NetworkInterface.cs
- InvariantComparer.cs
- ConfigurationProperty.cs
- ToggleButton.cs
- DocumentViewerBaseAutomationPeer.cs
- RoleManagerEventArgs.cs
- Vector.cs
- DbCommandTree.cs
- DataControlExtensions.cs
- MediaCommands.cs
- XmlUtil.cs
- FixedHighlight.cs
- HybridWebProxyFinder.cs
- Dispatcher.cs
- HtmlCommandAdapter.cs
- FormCollection.cs
- DataTablePropertyDescriptor.cs
- DataListCommandEventArgs.cs
- XmlBinaryReaderSession.cs
- _ConnectOverlappedAsyncResult.cs
- OracleCommandBuilder.cs
- XmlSchemaDatatype.cs
- SqlXml.cs
- RegexMatch.cs
- ScrollViewer.cs
- Container.cs
- CacheDependency.cs
- SignedInfo.cs
- Viewport2DVisual3D.cs
- SqlHelper.cs
- ImageAutomationPeer.cs
- PreloadedPackages.cs
- SystemResourceKey.cs
- FixedSOMTextRun.cs
- ReflectionPermission.cs
- SerTrace.cs
- WebPartChrome.cs
- MemoryFailPoint.cs
- ObjectDataSourceStatusEventArgs.cs
- AssemblyNameEqualityComparer.cs
- SmtpLoginAuthenticationModule.cs
- BinaryKeyIdentifierClause.cs
- codemethodreferenceexpression.cs
- SystemIPGlobalStatistics.cs
- ExpressionNormalizer.cs
- XamlDesignerSerializationManager.cs
- NotifyInputEventArgs.cs
- TextAutomationPeer.cs
- HttpWebResponse.cs
- FontUnit.cs
- CheckBoxRenderer.cs
- ContextInformation.cs
- DesignerGeometryHelper.cs
- HtmlEmptyTagControlBuilder.cs
- OracleTimeSpan.cs
- DataGridColumn.cs
- DataGridItem.cs
- BooleanAnimationBase.cs
- LineBreakRecord.cs
- SqlDataSourceConfigureSelectPanel.cs
- DocumentXmlWriter.cs
- ClientBuildManager.cs
- ToolStripComboBox.cs
- TreeNodeStyleCollection.cs
- Attributes.cs
- ProfileManager.cs
- FormViewDeletedEventArgs.cs
- FontInfo.cs
- Win32Exception.cs
- ColumnClickEvent.cs
- SHA512.cs
- TimeSpanParse.cs
- DataTransferEventArgs.cs