Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / FormCollection.cs / 1305376 / FormCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Globalization; ////// /// public class FormCollection : ReadOnlyCollectionBase { internal static object CollectionSyncRoot = new object(); ////// This is a read only collection of Forms exposed as a static property of the /// Application class. This is used to store all the currently loaded forms in an app. /// ////// /// public virtual Form this[string name] { get { if (name != null) { lock (CollectionSyncRoot) { foreach(Form form in InnerList) { if (string.Equals(form.Name, name, StringComparison.OrdinalIgnoreCase)) { return form; } } } } return null; } } ////// Gets a form specified by name, if present, else returns null. If there are multiple /// forms with matching names, the first form found is returned. /// ////// /// public virtual Form this[int index] { get { Form f = null; lock (CollectionSyncRoot) { f = (Form) InnerList[index]; } return f; } } ////// Gets a form specified by index. /// ////// Used internally to add a Form to the FormCollection /// internal void Add(Form form) { lock (CollectionSyncRoot) { InnerList.Add(form); } } ////// Used internally to check if a Form is in the FormCollection /// internal bool Contains(Form form) { bool inCollection = false; lock (CollectionSyncRoot) { inCollection = InnerList.Contains(form); } return inCollection; } ////// Used internally to add a Form to the FormCollection /// internal void Remove(Form form) { lock (CollectionSyncRoot) { InnerList.Remove(form); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
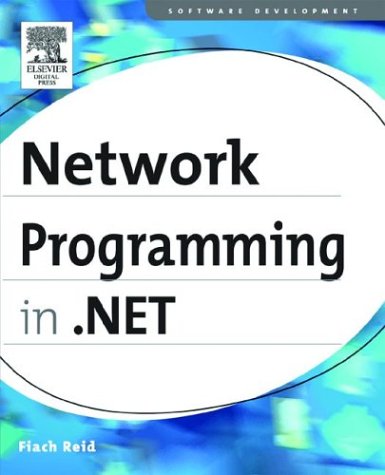
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509SecurityToken.cs
- CommandLibraryHelper.cs
- ThrowHelper.cs
- Ipv6Element.cs
- IdentifierCollection.cs
- unitconverter.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SplineKeyFrames.cs
- CompilerLocalReference.cs
- ListMarkerSourceInfo.cs
- NativeMethods.cs
- CharStorage.cs
- Html32TextWriter.cs
- GridViewRowEventArgs.cs
- DataServiceHost.cs
- SecurityRuntime.cs
- ComponentCollection.cs
- ReverseComparer.cs
- InstanceKeyCollisionException.cs
- HScrollProperties.cs
- PersistenceTypeAttribute.cs
- Compensation.cs
- ResourceProviderFactory.cs
- FormView.cs
- Calendar.cs
- FormatVersion.cs
- DataControlPagerLinkButton.cs
- Debugger.cs
- LinkLabelLinkClickedEvent.cs
- CodeCommentStatement.cs
- XmlSchemaAttribute.cs
- SafeNativeMethods.cs
- FileSystemEventArgs.cs
- SpellerInterop.cs
- UIElementIsland.cs
- InstallerTypeAttribute.cs
- columnmapfactory.cs
- WebPartZoneBaseDesigner.cs
- SchemeSettingElement.cs
- DesignerDeviceConfig.cs
- MembershipPasswordException.cs
- AlignmentXValidation.cs
- MsdtcWrapper.cs
- ColorConvertedBitmapExtension.cs
- MappingModelBuildProvider.cs
- ExecutionPropertyManager.cs
- HttpCookieCollection.cs
- SessionEndedEventArgs.cs
- PolyBezierSegmentFigureLogic.cs
- TextEditorMouse.cs
- ApplicationActivator.cs
- TextEditorContextMenu.cs
- FileLogRecord.cs
- DefaultExpressionVisitor.cs
- TrackBarDesigner.cs
- DbProviderFactories.cs
- GraphicsPathIterator.cs
- TimeSpanValidator.cs
- ObjectListTitleAttribute.cs
- SqlMethods.cs
- ResourceDefaultValueAttribute.cs
- METAHEADER.cs
- Cell.cs
- VersionedStream.cs
- File.cs
- TypeUsage.cs
- LiteralText.cs
- SqlConnectionPoolProviderInfo.cs
- TdsRecordBufferSetter.cs
- OverrideMode.cs
- XmlQueryContext.cs
- BufferAllocator.cs
- ImageListStreamer.cs
- AutomationPatternInfo.cs
- References.cs
- PlatformNotSupportedException.cs
- X509CertificateChain.cs
- AdornerDecorator.cs
- GlobalProxySelection.cs
- BrowserTree.cs
- PropertyCondition.cs
- TaskCanceledException.cs
- BinaryObjectInfo.cs
- ToolBar.cs
- PeerIPHelper.cs
- WindowsScroll.cs
- DATA_BLOB.cs
- InArgument.cs
- NavigationHelper.cs
- SqlClientPermission.cs
- ObjectItemCollection.cs
- ScrollChrome.cs
- MultiSelectRootGridEntry.cs
- DisplayToken.cs
- UnsafeNetInfoNativeMethods.cs
- VisualStyleRenderer.cs
- RecognizedAudio.cs
- SmiSettersStream.cs
- DataGridViewCellConverter.cs
- RsaSecurityToken.cs