Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / DisplayToken.cs / 1 / DisplayToken.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using System.IO; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; enum DisplayClaimType :byte { NoDisplayToken = 0, // No display token was present in the RSTR DisplayClaimString = 1, // A display string token was present in the RSTR DisplayClaimList = 2 // A display list token was present in the RSTR } // // Summary // This class represents a display token returned from the IP/STS in the RSTR // class DisplayToken { string m_displayString; string m_mimeType; Listm_displayList; DisplayClaimType m_displayType; public IEnumerable ClaimList { get { return m_displayList; } } public DisplayToken() { m_displayType = DisplayClaimType.NoDisplayToken; } public DisplayToken( string displayString, string mimeType ) { m_displayString = displayString; m_mimeType = mimeType; m_displayType = DisplayClaimType.DisplayClaimString; } public DisplayToken( List claimList ) { m_displayList = claimList; m_displayType = DisplayClaimType.DisplayClaimList; } // // Summary // Serialize the displaytoken // // Parameter // The writer to which the serialized data is written. // public void Serialize( BinaryWriter writer ) { writer.Write( (byte)m_displayType ); switch( m_displayType ) { case DisplayClaimType.DisplayClaimList: IDT.TraceDebug( "Display token claim list was created." ); writer.Write( ( UInt32 )m_displayList.Count ); foreach( DisplayClaim clm in m_displayList ) { clm.Serialize( writer ); } break; case DisplayClaimType.DisplayClaimString: IDT.TraceDebug( "Display token string was created." ); Utility.SerializeString( writer, m_displayString ); Utility.SerializeString( writer, m_mimeType ); break; case DisplayClaimType.NoDisplayToken: IDT.TraceDebug( "No display token was created." ); break; default: IDT.ThrowInvalidArgumentConditional( true, "ClaimType" ); break; } } // // Summary // Deserialize the display claim // // Parameter // The reader from which the serialized data is read. // // REMARKS: // Used by tools only // public void Deserialize( BinaryReader reader ) { m_displayType = (DisplayClaimType) reader.ReadByte(); switch( m_displayType ) { case DisplayClaimType.DisplayClaimList: m_displayList.Clear(); UInt32 count = reader.ReadUInt32(); for( UInt32 i = 0; i < count; i++ ) { DisplayClaim clm = new DisplayClaim( reader ); m_displayList.Add( clm ); } break; case DisplayClaimType.DisplayClaimString: m_displayString = Utility.DeserializeString( reader ); m_mimeType = Utility.DeserializeString( reader ); break; case DisplayClaimType.NoDisplayToken: break; default: throw IDT.ThrowHelperError( new InvalidOperationException( SR.GetString( SR.InvalidDisplayClaimType ) ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
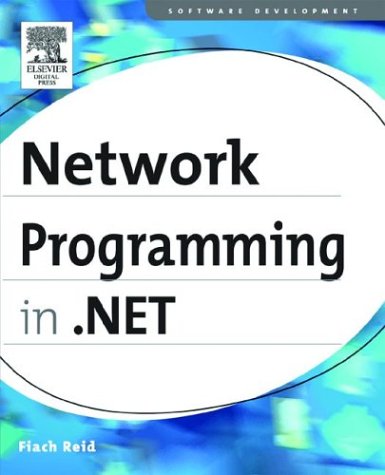
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckableControlBaseAdapter.cs
- TimeSpanValidator.cs
- HttpRawResponse.cs
- X509LogoTypeExtension.cs
- TranslateTransform3D.cs
- LockCookie.cs
- XPathNavigator.cs
- Privilege.cs
- DataColumnMapping.cs
- MethodToken.cs
- UIElementHelper.cs
- EventBuilder.cs
- Vector3DValueSerializer.cs
- ToolStripButton.cs
- DataStorage.cs
- HttpVersion.cs
- WebEncodingValidator.cs
- StylusPointPropertyId.cs
- XmlObjectSerializerReadContextComplex.cs
- SqlPersonalizationProvider.cs
- MobileUITypeEditor.cs
- EmptyElement.cs
- PipeStream.cs
- BaseTemplateParser.cs
- ByteAnimation.cs
- XmlnsCompatibleWithAttribute.cs
- ApplicationServicesHostFactory.cs
- RawMouseInputReport.cs
- Metafile.cs
- LookupTables.cs
- ProfileWorkflowElement.cs
- keycontainerpermission.cs
- InvalidPrinterException.cs
- ContainerSelectorGlyph.cs
- WebPartVerbsEventArgs.cs
- AlgoModule.cs
- PeekCompletedEventArgs.cs
- RemotingServices.cs
- Nullable.cs
- OpacityConverter.cs
- ZoneButton.cs
- ToolStripItemCollection.cs
- StyleCollection.cs
- Base64Decoder.cs
- Soap.cs
- NonSerializedAttribute.cs
- Transactions.cs
- EditorAttribute.cs
- StorageMappingFragment.cs
- DataGridViewEditingControlShowingEventArgs.cs
- CompressedStack.cs
- Simplifier.cs
- BamlBinaryReader.cs
- ResourceExpressionBuilder.cs
- EventSinkHelperWriter.cs
- NumericPagerField.cs
- TreeWalker.cs
- WhitespaceSignificantCollectionAttribute.cs
- Renderer.cs
- Timeline.cs
- PEFileReader.cs
- ControlDesigner.cs
- CornerRadius.cs
- DesignerView.cs
- AmbientLight.cs
- SqlDelegatedTransaction.cs
- Relationship.cs
- CrossContextChannel.cs
- HttpResponseInternalWrapper.cs
- ProtocolsConfiguration.cs
- Image.cs
- WeakHashtable.cs
- XslTransform.cs
- XsltFunctions.cs
- MaterialGroup.cs
- ItemsPresenter.cs
- AttachedAnnotationChangedEventArgs.cs
- ServiceDescriptionReflector.cs
- AutomationEventArgs.cs
- DesignTimeDataBinding.cs
- XmlSchemaGroupRef.cs
- TcpClientSocketManager.cs
- RuntimeConfigLKG.cs
- WebServiceEnumData.cs
- ByValueEqualityComparer.cs
- EmptyImpersonationContext.cs
- RangeValuePattern.cs
- connectionpool.cs
- SspiHelper.cs
- ResourceReader.cs
- SoapFormatterSinks.cs
- GlyphShapingProperties.cs
- ConfigurationManager.cs
- NetPipeSectionData.cs
- BitmapImage.cs
- ControlTemplate.cs
- NativeMethods.cs
- SelectionUIService.cs
- XmlWriterTraceListener.cs
- UnionExpr.cs