Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / DeliveryStrategy.cs / 1 / DeliveryStrategy.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Collections.Generic; using System.ServiceModel.Diagnostics; using System.Threading; abstract class DeliveryStrategy: IDisposable where ItemType : class, IDisposable { InputQueueChannel channel; ItemDequeuedCallback dequeueCallback; int quota; public DeliveryStrategy(InputQueueChannel channel, int quota) { if (quota <= 0) { DiagnosticUtility.DebugAssert("Argument quota must be positive."); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } this.channel = channel; this.quota = quota; } protected InputQueueChannel Channel { get { return this.channel; } } public ItemDequeuedCallback DequeueCallback { get { return this.dequeueCallback; } set { this.dequeueCallback = value; } } public abstract int EnqueuedCount { get; } protected int Quota { get { return this.quota; } } public abstract bool CanEnqueue(Int64 sequenceNumber); public virtual void Dispose() { } public abstract bool Enqueue(ItemType item, Int64 sequenceNumber); } class OrderedDeliveryStrategy : DeliveryStrategy where ItemType : class, IDisposable { bool isEnqueueInOrder; Dictionary items; WaitCallback onDispatchCallback; Int64 windowStart; public OrderedDeliveryStrategy( InputQueueChannel channel, int quota, bool isEnqueueInOrder) : base(channel, quota) { this.isEnqueueInOrder = isEnqueueInOrder; this.items = new Dictionary (); this.windowStart = 1; } public override int EnqueuedCount { get { return this.Channel.InternalPendingItems + this.items.Count; } } WaitCallback OnDispatchCallback { get { if (this.onDispatchCallback == null) { this.onDispatchCallback = this.OnDispatch; } return this.onDispatchCallback; } } public override bool CanEnqueue(long sequenceNumber) { if (this.EnqueuedCount >= this.Quota) { return false; } if (this.isEnqueueInOrder && (sequenceNumber > this.windowStart)) { return false; } return (this.Channel.InternalPendingItems + sequenceNumber - this.windowStart < this.Quota); } public override bool Enqueue(ItemType item, long sequenceNumber) { if (sequenceNumber > this.windowStart) { this.items.Add(sequenceNumber, item); return false; } this.windowStart++; while (this.items.ContainsKey(this.windowStart)) { if (this.Channel.EnqueueWithoutDispatch(item, this.DequeueCallback)) { IOThreadScheduler.ScheduleCallback(this.OnDispatchCallback, null); } item = this.items[this.windowStart]; this.items.Remove(this.windowStart); this.windowStart++; } return this.Channel.EnqueueWithoutDispatch(item, this.DequeueCallback); } static void DisposeItems(Dictionary .Enumerator items) { if (items.MoveNext()) { using (ItemType item = items.Current.Value) { DisposeItems(items); } } } public override void Dispose() { DisposeItems(this.items.GetEnumerator()); this.items.Clear(); base.Dispose(); } void OnDispatch(object state) { this.Channel.Dispatch(); } } class UnorderedDeliveryStrategy : DeliveryStrategy where ItemType : class, IDisposable { public UnorderedDeliveryStrategy(InputQueueChannel channel, int quota) : base(channel, quota) { } public override int EnqueuedCount { get { return this.Channel.InternalPendingItems; } } public override bool CanEnqueue(Int64 sequenceNumber) { return (this.EnqueuedCount < this.Quota); } public override bool Enqueue(ItemType item, long sequenceNumber) { return this.Channel.EnqueueWithoutDispatch(item, this.DequeueCallback); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
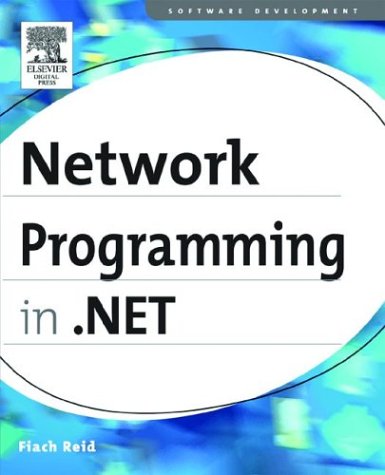
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridPagerStyle.cs
- RequestSecurityToken.cs
- ReceiveSecurityHeaderEntry.cs
- TypefaceCollection.cs
- TableCell.cs
- HitTestParameters3D.cs
- SoapAttributes.cs
- OletxDependentTransaction.cs
- BufferedGraphicsManager.cs
- PrintPageEvent.cs
- ImmutableObjectAttribute.cs
- RecognizeCompletedEventArgs.cs
- LocatorBase.cs
- EventSinkHelperWriter.cs
- ADConnectionHelper.cs
- Int32RectConverter.cs
- WebPartTracker.cs
- XPathQilFactory.cs
- ParallelSeparator.xaml.cs
- OpacityConverter.cs
- ButtonAutomationPeer.cs
- RegexWriter.cs
- PasswordDeriveBytes.cs
- ItemTypeToolStripMenuItem.cs
- DataServiceHost.cs
- WebReferenceOptions.cs
- CurrencyWrapper.cs
- MethodCallTranslator.cs
- Type.cs
- TraceListener.cs
- SystemDropShadowChrome.cs
- TaskHelper.cs
- COM2ICategorizePropertiesHandler.cs
- SchemaImporterExtensionElement.cs
- PackagePartCollection.cs
- mansign.cs
- LassoSelectionBehavior.cs
- DetailsViewUpdatedEventArgs.cs
- XmlDataSourceNodeDescriptor.cs
- EtwTrackingParticipant.cs
- LocalizedNameDescriptionPair.cs
- MeasureData.cs
- LayoutManager.cs
- LineInfo.cs
- METAHEADER.cs
- GenericWebPart.cs
- XmlReflectionMember.cs
- SizeFConverter.cs
- RMEnrollmentPage3.cs
- InstanceDescriptor.cs
- MemberHolder.cs
- DoubleLinkListEnumerator.cs
- ValueCollectionParameterReader.cs
- InProcStateClientManager.cs
- UnsafeNativeMethods.cs
- DiscoveryDocumentLinksPattern.cs
- RecordsAffectedEventArgs.cs
- XPathNavigatorKeyComparer.cs
- DocumentGridPage.cs
- ITextView.cs
- RemotingAttributes.cs
- GroupByExpressionRewriter.cs
- DropSource.cs
- PerfCounters.cs
- _AuthenticationState.cs
- BitConverter.cs
- Property.cs
- WinEventHandler.cs
- SelectionItemProviderWrapper.cs
- RightsManagementPermission.cs
- XmlDataSource.cs
- FontUnitConverter.cs
- DecoderFallback.cs
- InfoCardUIAgent.cs
- OperandQuery.cs
- SchemaImporterExtensionElementCollection.cs
- ProfileProvider.cs
- StreamDocument.cs
- GeometryGroup.cs
- TextCollapsingProperties.cs
- AvtEvent.cs
- StylusPointPropertyId.cs
- FastEncoder.cs
- SapiGrammar.cs
- DebugViewWriter.cs
- ProxyAssemblyNotLoadedException.cs
- DataGridViewMethods.cs
- DtdParser.cs
- RC2.cs
- MsmqHostedTransportManager.cs
- OutputCacheSettingsSection.cs
- UnmanagedMemoryStream.cs
- Point3DIndependentAnimationStorage.cs
- DataGridViewCellEventArgs.cs
- ScriptModule.cs
- ApplicationGesture.cs
- AnonymousIdentificationModule.cs
- CatalogPart.cs
- COM2Enum.cs
- LinqDataSourceView.cs