Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / tx / System / Transactions / Oletx / OletxDependentTransaction.cs / 1305376 / OletxDependentTransaction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Threading; using System.Transactions.Diagnostics; namespace System.Transactions.Oletx { [Serializable] internal class OletxDependentTransaction : OletxTransaction { private OletxVolatileEnlistmentContainer volatileEnlistmentContainer; private int completed = 0; internal OletxDependentTransaction( RealOletxTransaction realTransaction, bool delayCommit ) : base( realTransaction ) { if ( null == realTransaction ) { throw new ArgumentNullException( "realTransaction" ); } this.volatileEnlistmentContainer = realOletxTransaction.AddDependentClone( delayCommit ); if ( DiagnosticTrace.Information ) { DependentCloneCreatedTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), this.TransactionTraceId, delayCommit ? DependentCloneOption.BlockCommitUntilComplete : DependentCloneOption.RollbackIfNotComplete ); } } public void Complete() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "DependentTransaction.Complete" ); } Debug.Assert( ( 0 == this.disposed ), "OletxTransction object is disposed" ); int localCompleted = Interlocked.CompareExchange( ref this.completed, 1, 0 ); if ( 1 == localCompleted ) { throw TransactionException.CreateTransactionCompletedException( SR.GetString( SR.TraceSourceOletx ) ); } if ( DiagnosticTrace.Information ) { DependentCloneCompleteTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), this.TransactionTraceId ); } this.volatileEnlistmentContainer.DependentCloneCompleted(); if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "DependentTransaction.Complete" ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Threading; using System.Transactions.Diagnostics; namespace System.Transactions.Oletx { [Serializable] internal class OletxDependentTransaction : OletxTransaction { private OletxVolatileEnlistmentContainer volatileEnlistmentContainer; private int completed = 0; internal OletxDependentTransaction( RealOletxTransaction realTransaction, bool delayCommit ) : base( realTransaction ) { if ( null == realTransaction ) { throw new ArgumentNullException( "realTransaction" ); } this.volatileEnlistmentContainer = realOletxTransaction.AddDependentClone( delayCommit ); if ( DiagnosticTrace.Information ) { DependentCloneCreatedTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), this.TransactionTraceId, delayCommit ? DependentCloneOption.BlockCommitUntilComplete : DependentCloneOption.RollbackIfNotComplete ); } } public void Complete() { if ( DiagnosticTrace.Verbose ) { MethodEnteredTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "DependentTransaction.Complete" ); } Debug.Assert( ( 0 == this.disposed ), "OletxTransction object is disposed" ); int localCompleted = Interlocked.CompareExchange( ref this.completed, 1, 0 ); if ( 1 == localCompleted ) { throw TransactionException.CreateTransactionCompletedException( SR.GetString( SR.TraceSourceOletx ) ); } if ( DiagnosticTrace.Information ) { DependentCloneCompleteTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), this.TransactionTraceId ); } this.volatileEnlistmentContainer.DependentCloneCompleted(); if ( DiagnosticTrace.Verbose ) { MethodExitedTraceRecord.Trace( SR.GetString( SR.TraceSourceOletx ), "DependentTransaction.Complete" ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
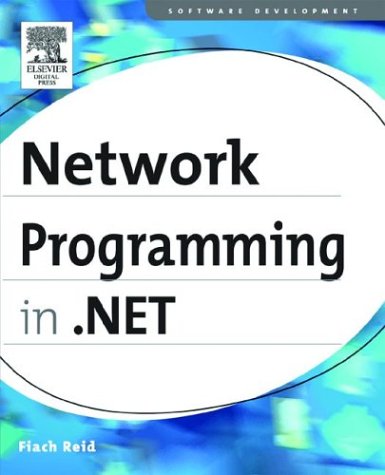
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetWebProxyFinder.cs
- SchemaTableOptionalColumn.cs
- SystemIPInterfaceProperties.cs
- ScrollContentPresenter.cs
- FrameworkElementAutomationPeer.cs
- BigInt.cs
- Oci.cs
- NamespaceMapping.cs
- PropertyDescriptors.cs
- BevelBitmapEffect.cs
- SemanticValue.cs
- StateDesignerConnector.cs
- StructuralCache.cs
- Assembly.cs
- CommonProperties.cs
- PlatformCulture.cs
- ProcessThreadCollection.cs
- InternalBufferOverflowException.cs
- DocumentPageHost.cs
- XmlUtil.cs
- ToolStripSystemRenderer.cs
- ConfigurationStrings.cs
- TransactionScopeDesigner.cs
- PerspectiveCamera.cs
- _IPv4Address.cs
- ConstructorBuilder.cs
- BinaryWriter.cs
- NamedPipeWorkerProcess.cs
- UIEndRequest.cs
- AutomationIdentifier.cs
- EtwTrace.cs
- GacUtil.cs
- XmlSchemaAttributeGroup.cs
- SafeHandles.cs
- CodeTypeMember.cs
- DataBoundControl.cs
- SQLMembershipProvider.cs
- SqlMultiplexer.cs
- NameValueFileSectionHandler.cs
- Guid.cs
- WriteTimeStream.cs
- AnimationClockResource.cs
- ScriptResourceInfo.cs
- FileDetails.cs
- ControlValuePropertyAttribute.cs
- SchemaManager.cs
- CompressStream.cs
- Double.cs
- DragEventArgs.cs
- FusionWrap.cs
- UnmanagedMarshal.cs
- EpmContentSerializerBase.cs
- Lease.cs
- ProcessHostMapPath.cs
- ObjectPersistData.cs
- TableHeaderCell.cs
- AnnotationAuthorChangedEventArgs.cs
- CroppedBitmap.cs
- SiteOfOriginContainer.cs
- XmlILConstructAnalyzer.cs
- LicenseContext.cs
- SafeSecurityHelper.cs
- XmlSchemaComplexType.cs
- CompressionTracing.cs
- TextWriter.cs
- PlatformNotSupportedException.cs
- ListCollectionView.cs
- EntityCodeGenerator.cs
- MapPathBasedVirtualPathProvider.cs
- HMACMD5.cs
- VectorKeyFrameCollection.cs
- TimeSpanValidatorAttribute.cs
- WebPartVerbsEventArgs.cs
- TextTreeRootNode.cs
- Exception.cs
- VerticalAlignConverter.cs
- Selection.cs
- NamedPermissionSet.cs
- DataGridCellsPresenter.cs
- WindowsGraphicsCacheManager.cs
- EventDriven.cs
- QueryStringConverter.cs
- BufferedWebEventProvider.cs
- ConfigurationConverterBase.cs
- ReverseInheritProperty.cs
- DelayDesigner.cs
- TimeZoneInfo.cs
- EventLogEntryCollection.cs
- FreezableDefaultValueFactory.cs
- TypeConverterAttribute.cs
- RequestCachePolicy.cs
- InvokeMethod.cs
- CheckBox.cs
- CheckPair.cs
- MasterPageParser.cs
- URI.cs
- InstanceKeyView.cs
- DataColumn.cs
- WCFServiceClientProxyGenerator.cs
- CommittableTransaction.cs