Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / HitTestParameters3D.cs / 1 / HitTestParameters3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // History: // 03/16/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using MS.Internal.Media3D; using CultureInfo = System.Globalization.CultureInfo; namespace System.Windows.Media.Media3D { ////// Encapsulates a set parameters for performing a 3D hit test. This is an /// abstract base class. /// ////// Internally the HitTestParameters3D is double as the hit testing "context" /// which includes such things as the current LocalToWorld transform, etc. /// public abstract class HitTestParameters3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal to prevent 3rd parties from extending this abstract base class. internal HitTestParameters3D() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal void PushVisualTransform(Transform3D transform) { Debug.Assert(!HasModelTransformMatrix, "ModelTransform stack should be empty when pusing a visual transform"); if (transform != null && transform != Transform3D.Identity) { _visualTransformStack.Push(transform.Value); } } internal void PushModelTransform(Transform3D transform) { if (transform != null && transform != Transform3D.Identity) { _modelTransformStack.Push(transform.Value); } } internal void PopTransform(Transform3D transform) { if (transform != null && transform != Transform3D.Identity) { if (_modelTransformStack.Count > 0) { _modelTransformStack.Pop(); } else { _visualTransformStack.Pop(); } } } //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal bool HasWorldTransformMatrix { get { return _visualTransformStack.Count > 0 || _modelTransformStack.Count > 0; } } internal Matrix3D WorldTransformMatrix { get { Debug.Assert(HasWorldTransformMatrix, "Check HasWorldTransformMatrix before accessing WorldTransformMatrix."); if (_modelTransformStack.IsEmpty) { return _visualTransformStack.Top; } else if (_visualTransformStack.IsEmpty) { return _modelTransformStack.Top; } else { return _modelTransformStack.Top * _visualTransformStack.Top; } } } internal bool HasModelTransformMatrix { get { return _modelTransformStack.Count > 0; } } ////// The ModelTransformMatrix is the transform in the coordinate system /// of the last Visual hit. /// internal Matrix3D ModelTransformMatrix { get { Debug.Assert(HasModelTransformMatrix, "Check HasModelTransformMatrix before accessing ModelTransformMatrix."); return _modelTransformStack.Top; } } ////// True if the hit test origined in 2D (i.e., we projected a /// the ray from a point on a Viewport3DVisual.) /// internal bool HasHitTestProjectionMatrix { get { return _hitTestProjectionMatrix != null; } } ////// The projection matrix can be set to give additional /// information about a hit test that originated from a camera. /// When any 3D point is projected using the matrix it ends up /// at an x,y location (after homogeneous divide) that is a /// constant translation of its location in the camera's /// viewpoint. /// /// All points on the ray project to 0,0 /// /// The RayFromViewportPoint methods will set this matrix up. /// This matrix is needed to implement hit testing of the magic /// line, which is not intersected by any rays not produced /// using RayFromViewportPoint. /// /// It is being added to HitTestParameters3D rather than /// RayHitTestParameters3D because it could be generally useful /// to any sort of hit testing that originates from a camera, /// including cone hit testing or the full generalization of 3D /// shape hit testing. /// internal Matrix3D HitTestProjectionMatrix { get { Debug.Assert(HasHitTestProjectionMatrix, "Check HasHitTestProjectionMatrix before accessing HitTestProjectionMatrix."); return _hitTestProjectionMatrix.Value; } set { _hitTestProjectionMatrix = new Matrix3D?(value); } } internal Visual3D CurrentVisual; internal Model3D CurrentModel; internal GeometryModel3D CurrentGeometry; #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private Matrix3D? _hitTestProjectionMatrix = null; private Matrix3DStack _visualTransformStack = new Matrix3DStack(); private Matrix3DStack _modelTransformStack = new Matrix3DStack(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // History: // 03/16/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using MS.Internal.Media3D; using CultureInfo = System.Globalization.CultureInfo; namespace System.Windows.Media.Media3D { ////// Encapsulates a set parameters for performing a 3D hit test. This is an /// abstract base class. /// ////// Internally the HitTestParameters3D is double as the hit testing "context" /// which includes such things as the current LocalToWorld transform, etc. /// public abstract class HitTestParameters3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal to prevent 3rd parties from extending this abstract base class. internal HitTestParameters3D() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal void PushVisualTransform(Transform3D transform) { Debug.Assert(!HasModelTransformMatrix, "ModelTransform stack should be empty when pusing a visual transform"); if (transform != null && transform != Transform3D.Identity) { _visualTransformStack.Push(transform.Value); } } internal void PushModelTransform(Transform3D transform) { if (transform != null && transform != Transform3D.Identity) { _modelTransformStack.Push(transform.Value); } } internal void PopTransform(Transform3D transform) { if (transform != null && transform != Transform3D.Identity) { if (_modelTransformStack.Count > 0) { _modelTransformStack.Pop(); } else { _visualTransformStack.Pop(); } } } //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal bool HasWorldTransformMatrix { get { return _visualTransformStack.Count > 0 || _modelTransformStack.Count > 0; } } internal Matrix3D WorldTransformMatrix { get { Debug.Assert(HasWorldTransformMatrix, "Check HasWorldTransformMatrix before accessing WorldTransformMatrix."); if (_modelTransformStack.IsEmpty) { return _visualTransformStack.Top; } else if (_visualTransformStack.IsEmpty) { return _modelTransformStack.Top; } else { return _modelTransformStack.Top * _visualTransformStack.Top; } } } internal bool HasModelTransformMatrix { get { return _modelTransformStack.Count > 0; } } ////// The ModelTransformMatrix is the transform in the coordinate system /// of the last Visual hit. /// internal Matrix3D ModelTransformMatrix { get { Debug.Assert(HasModelTransformMatrix, "Check HasModelTransformMatrix before accessing ModelTransformMatrix."); return _modelTransformStack.Top; } } ////// True if the hit test origined in 2D (i.e., we projected a /// the ray from a point on a Viewport3DVisual.) /// internal bool HasHitTestProjectionMatrix { get { return _hitTestProjectionMatrix != null; } } ////// The projection matrix can be set to give additional /// information about a hit test that originated from a camera. /// When any 3D point is projected using the matrix it ends up /// at an x,y location (after homogeneous divide) that is a /// constant translation of its location in the camera's /// viewpoint. /// /// All points on the ray project to 0,0 /// /// The RayFromViewportPoint methods will set this matrix up. /// This matrix is needed to implement hit testing of the magic /// line, which is not intersected by any rays not produced /// using RayFromViewportPoint. /// /// It is being added to HitTestParameters3D rather than /// RayHitTestParameters3D because it could be generally useful /// to any sort of hit testing that originates from a camera, /// including cone hit testing or the full generalization of 3D /// shape hit testing. /// internal Matrix3D HitTestProjectionMatrix { get { Debug.Assert(HasHitTestProjectionMatrix, "Check HasHitTestProjectionMatrix before accessing HitTestProjectionMatrix."); return _hitTestProjectionMatrix.Value; } set { _hitTestProjectionMatrix = new Matrix3D?(value); } } internal Visual3D CurrentVisual; internal Model3D CurrentModel; internal GeometryModel3D CurrentGeometry; #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private Matrix3D? _hitTestProjectionMatrix = null; private Matrix3DStack _visualTransformStack = new Matrix3DStack(); private Matrix3DStack _modelTransformStack = new Matrix3DStack(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
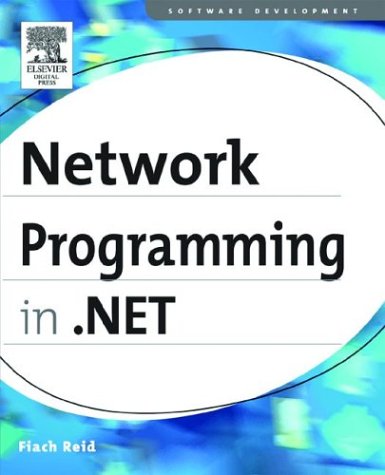
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartChrome.cs
- CodeTypeMember.cs
- WindowsIdentity.cs
- Cloud.cs
- Opcode.cs
- ResizeGrip.cs
- RSAOAEPKeyExchangeFormatter.cs
- SecurityManager.cs
- PerformanceCounterPermissionAttribute.cs
- PrinterUnitConvert.cs
- FillErrorEventArgs.cs
- RMEnrollmentPage3.cs
- BezierSegment.cs
- Console.cs
- QueryGenerator.cs
- LockedHandleGlyph.cs
- AnnotationService.cs
- ElementHostPropertyMap.cs
- ResourceSet.cs
- CryptoStream.cs
- AbandonedMutexException.cs
- JapaneseCalendar.cs
- StreamWriter.cs
- Set.cs
- NullReferenceException.cs
- NetworkInformationException.cs
- StandardToolWindows.cs
- Token.cs
- ToolStripContentPanel.cs
- XmlDataProvider.cs
- ConnectionPoolManager.cs
- ASCIIEncoding.cs
- OutOfMemoryException.cs
- SingleTagSectionHandler.cs
- DesignSurfaceManager.cs
- SafeCertificateStore.cs
- PropertyDescriptorGridEntry.cs
- X509ServiceCertificateAuthentication.cs
- UDPClient.cs
- DesignerSerializerAttribute.cs
- _ListenerResponseStream.cs
- ReadOnlyDictionary.cs
- BlurBitmapEffect.cs
- FixedPageStructure.cs
- DynamicAttribute.cs
- DataGridViewComboBoxColumn.cs
- TCPClient.cs
- ProcessingInstructionAction.cs
- HMACSHA1.cs
- TableLayoutStyleCollection.cs
- IfAction.cs
- DataGridViewRowHeaderCell.cs
- BamlBinaryReader.cs
- CodeExpressionCollection.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- TraceContextRecord.cs
- HttpProcessUtility.cs
- UnaryExpression.cs
- Transform3D.cs
- Pool.cs
- MetadataCacheItem.cs
- milexports.cs
- ScriptRegistrationManager.cs
- SettingsPropertyIsReadOnlyException.cs
- PathStreamGeometryContext.cs
- ObjectDataSourceSelectingEventArgs.cs
- RuntimeHandles.cs
- NonBatchDirectoryCompiler.cs
- ErrorWebPart.cs
- DocumentPageViewAutomationPeer.cs
- RegexWorker.cs
- MappedMetaModel.cs
- EnumMember.cs
- ProcessHostFactoryHelper.cs
- ListViewEditEventArgs.cs
- InstancePersistenceEvent.cs
- DataViewManagerListItemTypeDescriptor.cs
- DataSourceView.cs
- NetSectionGroup.cs
- ListViewGroupCollectionEditor.cs
- HtmlElementCollection.cs
- EntryWrittenEventArgs.cs
- DataGrid.cs
- JsonQNameDataContract.cs
- TextInfo.cs
- ToolStripAdornerWindowService.cs
- ListItem.cs
- BmpBitmapDecoder.cs
- CodeAttributeArgumentCollection.cs
- DocumentPageViewAutomationPeer.cs
- SQLMoneyStorage.cs
- WindowsGraphics.cs
- BufferedResponseStream.cs
- DataGridTextColumn.cs
- IBuiltInEvidence.cs
- TagNameToTypeMapper.cs
- AttributedMetaModel.cs
- updateconfighost.cs
- TextServicesCompartmentEventSink.cs
- PolicyException.cs