Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / X509ServiceCertificateAuthentication.cs / 1 / X509ServiceCertificateAuthentication.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Security { using System.IdentityModel.Selectors; using System.ServiceModel; using System.Security.Cryptography.X509Certificates; public class X509ServiceCertificateAuthentication { internal const X509CertificateValidationMode DefaultCertificateValidationMode = X509CertificateValidationMode.ChainTrust; internal const X509RevocationMode DefaultRevocationMode = X509RevocationMode.Online; internal const StoreLocation DefaultTrustedStoreLocation = StoreLocation.CurrentUser; static X509CertificateValidator defaultCertificateValidator; X509CertificateValidationMode certificateValidationMode = DefaultCertificateValidationMode; X509RevocationMode revocationMode = DefaultRevocationMode; StoreLocation trustedStoreLocation = DefaultTrustedStoreLocation; X509CertificateValidator customCertificateValidator = null; bool isReadOnly; internal X509ServiceCertificateAuthentication() { } internal X509ServiceCertificateAuthentication(X509ServiceCertificateAuthentication other) { this.certificateValidationMode = other.certificateValidationMode; this.customCertificateValidator = other.customCertificateValidator; this.revocationMode = other.revocationMode; this.trustedStoreLocation = other.trustedStoreLocation; this.isReadOnly = other.isReadOnly; } internal static X509CertificateValidator DefaultCertificateValidator { get { if (defaultCertificateValidator == null) { bool useMachineContext = DefaultTrustedStoreLocation == StoreLocation.LocalMachine; X509ChainPolicy chainPolicy = new X509ChainPolicy(); chainPolicy.RevocationMode = DefaultRevocationMode; defaultCertificateValidator = X509CertificateValidator.CreateChainTrustValidator(useMachineContext, chainPolicy); } return defaultCertificateValidator; } } public X509CertificateValidationMode CertificateValidationMode { get { return this.certificateValidationMode; } set { X509CertificateValidationModeHelper.Validate(value); ThrowIfImmutable(); this.certificateValidationMode = value; } } public X509RevocationMode RevocationMode { get { return this.revocationMode; } set { ThrowIfImmutable(); this.revocationMode = value; } } public StoreLocation TrustedStoreLocation { get { return this.trustedStoreLocation; } set { ThrowIfImmutable(); this.trustedStoreLocation = value; } } public X509CertificateValidator CustomCertificateValidator { get { return this.customCertificateValidator; } set { ThrowIfImmutable(); this.customCertificateValidator = value; } } internal bool TryGetCertificateValidator(out X509CertificateValidator validator) { validator = null; if (this.certificateValidationMode == X509CertificateValidationMode.None) { validator = X509CertificateValidator.None; } else if (this.certificateValidationMode == X509CertificateValidationMode.PeerTrust) { validator = X509CertificateValidator.PeerTrust; } else if (this.certificateValidationMode == X509CertificateValidationMode.Custom) { validator = this.customCertificateValidator; } else { bool useMachineContext = this.trustedStoreLocation == StoreLocation.LocalMachine; X509ChainPolicy chainPolicy = new X509ChainPolicy(); chainPolicy.RevocationMode = this.revocationMode; if (this.certificateValidationMode == X509CertificateValidationMode.ChainTrust) { validator = X509CertificateValidator.CreateChainTrustValidator(useMachineContext, chainPolicy); } else { validator = X509CertificateValidator.CreatePeerOrChainTrustValidator(useMachineContext, chainPolicy); } } return (validator != null); } internal X509CertificateValidator GetCertificateValidator() { X509CertificateValidator result; if (!TryGetCertificateValidator(out result)) { DiagnosticUtility.DebugAssert(this.customCertificateValidator == null, ""); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.MissingCustomCertificateValidator))); } return result; } internal void MakeReadOnly() { this.isReadOnly = true; } void ThrowIfImmutable() { if (this.isReadOnly) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
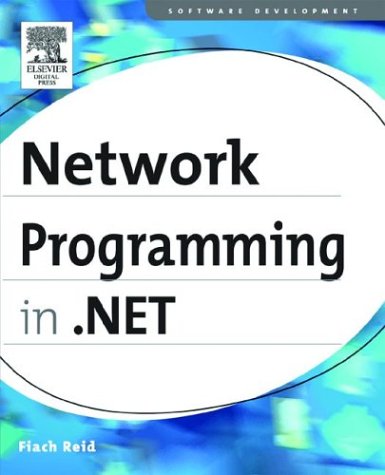
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingEnvironmentSection.cs
- EastAsianLunisolarCalendar.cs
- DataControlFieldHeaderCell.cs
- MissingMethodException.cs
- ButtonChrome.cs
- TypeConverterHelper.cs
- Stylesheet.cs
- UnsafeNativeMethods.cs
- ToolStripMenuItemCodeDomSerializer.cs
- bindurihelper.cs
- StylesEditorDialog.cs
- CssTextWriter.cs
- DataGridViewRowsRemovedEventArgs.cs
- QueryOutputWriter.cs
- PlatformCulture.cs
- XmlParserContext.cs
- AssociationEndMember.cs
- SafeEventLogWriteHandle.cs
- Imaging.cs
- ProfileModule.cs
- CacheAxisQuery.cs
- DiagnosticsConfiguration.cs
- SingletonInstanceContextProvider.cs
- DPTypeDescriptorContext.cs
- XmlSignatureManifest.cs
- CodeTypeDelegate.cs
- TimeSpanValidatorAttribute.cs
- ConfigUtil.cs
- WindowsButton.cs
- QilIterator.cs
- SplitterPanel.cs
- Journal.cs
- CommentAction.cs
- FontConverter.cs
- HtmlInputReset.cs
- Control.cs
- PropertyDescriptorGridEntry.cs
- TraceHandlerErrorFormatter.cs
- DependencyObjectProvider.cs
- Trigger.cs
- Bitmap.cs
- MemberDescriptor.cs
- RoleBoolean.cs
- SystemTcpConnection.cs
- ImageButton.cs
- TextElementAutomationPeer.cs
- UncommonField.cs
- TypeBrowserDialog.cs
- InitiatorServiceModelSecurityTokenRequirement.cs
- NameTable.cs
- ExtendedProtectionPolicyElement.cs
- ConfigurationPropertyAttribute.cs
- ToolStripItemEventArgs.cs
- SafeViewOfFileHandle.cs
- AssociationTypeEmitter.cs
- VisualProxy.cs
- TransformGroup.cs
- EncoderParameters.cs
- ConfigXmlReader.cs
- BitmapCache.cs
- ContextMenuService.cs
- DataGridViewCheckBoxCell.cs
- SafeNativeMethods.cs
- CultureNotFoundException.cs
- AccessibleObject.cs
- Scene3D.cs
- SystemIdentity.cs
- XmlTextReaderImplHelpers.cs
- ChangePassword.cs
- BindingMemberInfo.cs
- StrokeCollection2.cs
- SmiRequestExecutor.cs
- ISO2022Encoding.cs
- VScrollBar.cs
- QueryCursorEventArgs.cs
- RectangleGeometry.cs
- HelpProvider.cs
- Vector3D.cs
- SigningCredentials.cs
- ToolStripContentPanel.cs
- SqlTypesSchemaImporter.cs
- GestureRecognizer.cs
- OdbcParameter.cs
- NullRuntimeConfig.cs
- Column.cs
- XmlSchemaSimpleContent.cs
- GPStream.cs
- Substitution.cs
- GridToolTip.cs
- EntityStoreSchemaGenerator.cs
- SmuggledIUnknown.cs
- MultiBindingExpression.cs
- BooleanProjectedSlot.cs
- HtmlLinkAdapter.cs
- DeclaredTypeElementCollection.cs
- DataBinding.cs
- Stream.cs
- ClassImporter.cs
- ExpressionBinding.cs
- XmlNodeChangedEventManager.cs