Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SystemIdentity.cs / 1 / SystemIdentity.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.ComponentModel; using System.Globalization; using System.Runtime.InteropServices; using System.Security.Principal; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; internal sealed class SystemIdentity : IDisposable { bool m_isDisposed; WindowsIdentity m_identity; object m_sync; public static readonly IdentityReference LsaIdentityReference = new SecurityIdentifier( "SY" ); public SystemIdentity( bool throwIfAlreadySystem ) { m_sync = new object(); WindowsIdentity identity = WindowsIdentity.GetCurrent(); if( identity.IsSystem && throwIfAlreadySystem ) { // // This is an internal fatal error. // throw IDT.ThrowHelperError( new InvalidOperationException( SR.GetString( SR.UserIdentityEqualSystemNotSupported ) ) ); } else if( !identity.IsSystem ) { m_identity = identity; #pragma warning suppress 56523 if( !NativeMethods.RevertToSelf() ) { IDT.Assert( false, "Identity management failure" ); } } else { // // Do nothing. we are LSA already and throwIfAlreadySystem == false // IDT.Assert( null == m_identity, "m_identity should be null when we are system and throwIfAlreadySystem == false" ); } } void IDisposable.Dispose() { if ( m_isDisposed ) { return; } lock( m_sync ) { if( m_isDisposed ) { return; } m_isDisposed = true; if( null != m_identity ) { // // Impersonate the user // if( !NativeMethods.ImpersonateLoggedOnUser( m_identity.Token ) ) { int hr = Marshal.GetHRForLastWin32Error(); // // This exception is fatal to our service. // it could corrupt the flow of identity that the service expects. // Failfast here. // Diagnostics.InfoCardTrace.FailFast( String.Format( CultureInfo.InvariantCulture, SR.GetString( SR.StoreImpersonateLoggedOnUserFailed ), hr ) ); } m_identity.Dispose(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
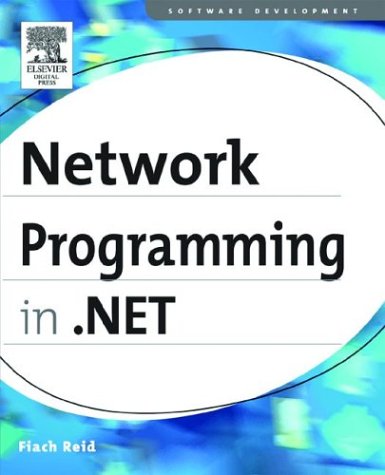
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DependencyPropertyHelper.cs
- ApplicationInfo.cs
- CompoundFileDeflateTransform.cs
- safelinkcollection.cs
- PeerValidationBehavior.cs
- Typography.cs
- Error.cs
- shaper.cs
- DataServiceRequestOfT.cs
- SoapHelper.cs
- ChildrenQuery.cs
- BatchWriter.cs
- CapabilitiesRule.cs
- StaticExtension.cs
- Hex.cs
- OleDbParameterCollection.cs
- FullTextLine.cs
- TdsValueSetter.cs
- ECDiffieHellmanCng.cs
- ImportCatalogPart.cs
- DeviceOverridableAttribute.cs
- SoapWriter.cs
- FillBehavior.cs
- QilGenerator.cs
- ZoneLinkButton.cs
- XmlRootAttribute.cs
- SchemaImporterExtensionElement.cs
- _SSPISessionCache.cs
- GenericPrincipal.cs
- NavigationWindow.cs
- TrackingMemoryStream.cs
- DrawingAttributeSerializer.cs
- ConfigurationSchemaErrors.cs
- Schema.cs
- RoutedEvent.cs
- ExpressionBuilderCollection.cs
- PipeSecurity.cs
- AdjustableArrowCap.cs
- WebPartVerbCollection.cs
- CommandHelper.cs
- Unit.cs
- MessageHeaders.cs
- EntitySetDataBindingList.cs
- ImageField.cs
- CubicEase.cs
- CertificateManager.cs
- RichTextBoxAutomationPeer.cs
- ADRoleFactoryConfiguration.cs
- AppDomainFactory.cs
- ShapeTypeface.cs
- EpmContentSerializerBase.cs
- PhoneCallDesigner.cs
- GatewayDefinition.cs
- VerticalAlignConverter.cs
- ImageAnimator.cs
- EntityDataSourceQueryBuilder.cs
- OptimisticConcurrencyException.cs
- SafeCoTaskMem.cs
- MimePart.cs
- FtpCachePolicyElement.cs
- ScriptManager.cs
- RtfControlWordInfo.cs
- MapPathBasedVirtualPathProvider.cs
- ColorContextHelper.cs
- SrgsGrammar.cs
- SQLInt32Storage.cs
- SerializationBinder.cs
- TouchPoint.cs
- ByteConverter.cs
- ListBoxItemWrapperAutomationPeer.cs
- InfoCardXmlSerializer.cs
- CodeParameterDeclarationExpressionCollection.cs
- TrackBar.cs
- And.cs
- BaseValidator.cs
- ModelPerspective.cs
- IsolatedStorageFileStream.cs
- Point3DAnimationBase.cs
- processwaithandle.cs
- _FtpDataStream.cs
- ItemsControlAutomationPeer.cs
- DbParameterHelper.cs
- ExpressionConverter.cs
- JsonReaderWriterFactory.cs
- ZoneLinkButton.cs
- ConnectionPointGlyph.cs
- FtpRequestCacheValidator.cs
- SpecialNameAttribute.cs
- Msec.cs
- xamlnodes.cs
- controlskin.cs
- Header.cs
- TextBoxBase.cs
- FontSourceCollection.cs
- WorkflowPrinting.cs
- RealizationDrawingContextWalker.cs
- AutomationPattern.cs
- SystemInfo.cs
- DynamicDataExtensions.cs
- HostExecutionContextManager.cs