Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / VerticalAlignConverter.cs / 1305376 / VerticalAlignConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; internal class VerticalAlignConverter : EnumConverter { static string[] stringValues = new String[(int) VerticalAlign.Bottom + 1]; static VerticalAlignConverter () { stringValues[(int) VerticalAlign.NotSet] = "NotSet"; stringValues[(int) VerticalAlign.Top] = "Top"; stringValues[(int) VerticalAlign.Middle] = "Middle"; stringValues[(int) VerticalAlign.Bottom] = "Bottom"; } // this constructor needs to be public despite the fact that it's in an internal // class so it can be created by Activator.CreateInstance. public VerticalAlignConverter () : base(typeof(VerticalAlign)) {} public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return base.CanConvertFrom(context, sourceType); } } public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) return null; if (value is string) { string textValue = ((string)value).Trim(); if (textValue.Length == 0) return VerticalAlign.NotSet; switch (textValue) { case "NotSet": return VerticalAlign.NotSet; case "Top": return VerticalAlign.Top; case "Middle": return VerticalAlign.Middle; case "Bottom": return VerticalAlign.Bottom; } } return base.ConvertFrom(context, culture, value); } public override bool CanConvertTo(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertTo(context, sourceType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == typeof(string) && ((int) value <= (int)VerticalAlign.Bottom)) { return stringValues[(int) value]; } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
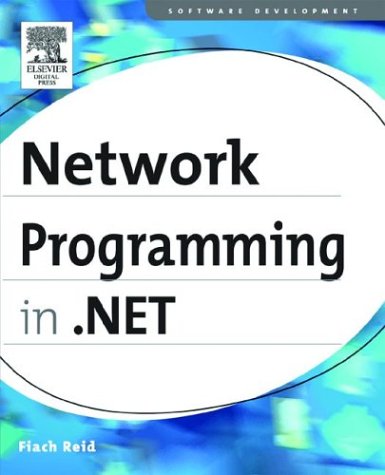
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlAliasesReferenced.cs
- externdll.cs
- DataDocumentXPathNavigator.cs
- BindingMAnagerBase.cs
- TdsParser.cs
- IRCollection.cs
- TextFormatterContext.cs
- InstalledVoice.cs
- DBDataPermissionAttribute.cs
- WithParamAction.cs
- File.cs
- UserControl.cs
- InstancePersistenceContext.cs
- BuildProviderAppliesToAttribute.cs
- ComPlusThreadInitializer.cs
- PeerNameRecordCollection.cs
- InputDevice.cs
- ObjectStateManager.cs
- AssociationTypeEmitter.cs
- DomainLiteralReader.cs
- BasicKeyConstraint.cs
- Size3DConverter.cs
- ToolStripDropDownDesigner.cs
- CounterCreationDataConverter.cs
- KoreanLunisolarCalendar.cs
- FileEnumerator.cs
- AsyncMethodInvoker.cs
- VisualStyleTypesAndProperties.cs
- ShaperBuffers.cs
- DescendentsWalkerBase.cs
- OracleConnectionString.cs
- CodeAttributeArgument.cs
- ResizeBehavior.cs
- XmlNamespaceMappingCollection.cs
- EdmProviderManifest.cs
- userdatakeys.cs
- CheckoutException.cs
- SQLByteStorage.cs
- Exceptions.cs
- TracePayload.cs
- Version.cs
- CharacterBufferReference.cs
- RangeValidator.cs
- ObjectDataSourceEventArgs.cs
- QilValidationVisitor.cs
- Repeater.cs
- PipelineModuleStepContainer.cs
- AuthenticateEventArgs.cs
- DataRecordInternal.cs
- EdmItemCollection.OcAssemblyCache.cs
- ProfessionalColorTable.cs
- CustomErrorCollection.cs
- RepeaterCommandEventArgs.cs
- StringFormat.cs
- PriorityRange.cs
- OleDbInfoMessageEvent.cs
- CopyNodeSetAction.cs
- NavigatorOutput.cs
- HttpCacheVaryByContentEncodings.cs
- ThreadStaticAttribute.cs
- MultipartContentParser.cs
- FieldInfo.cs
- UInt16Storage.cs
- BaseHashHelper.cs
- SelectionItemProviderWrapper.cs
- WebBrowserProgressChangedEventHandler.cs
- DesignerVerbCollection.cs
- ScopelessEnumAttribute.cs
- CssTextWriter.cs
- TextBoxBaseDesigner.cs
- HostingEnvironmentException.cs
- NativeMethods.cs
- WorkflowApplicationCompletedEventArgs.cs
- BinaryWriter.cs
- XmlSchemaValidator.cs
- CodeTypeConstructor.cs
- CodeIdentifier.cs
- _LoggingObject.cs
- LiteralTextParser.cs
- ProfileSettingsCollection.cs
- XmlBinaryReaderSession.cs
- FormViewDeleteEventArgs.cs
- CharacterHit.cs
- CodeCompiler.cs
- GorillaCodec.cs
- SchemaAttDef.cs
- ComboBoxRenderer.cs
- RegexCompilationInfo.cs
- NonceToken.cs
- DataServiceEntityAttribute.cs
- DataRecordInfo.cs
- RegexTree.cs
- EndpointDiscoveryMetadataCD1.cs
- ChangeProcessor.cs
- CounterCreationDataCollection.cs
- ToolStripSeparatorRenderEventArgs.cs
- CodeTypeReference.cs
- XmlSerializerSection.cs
- UncommonField.cs
- RelationshipNavigation.cs