Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / CharacterBufferReference.cs / 1 / CharacterBufferReference.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2003 // // File: CharacterBufferReference.cs // // Contents: Text Character buffer reference // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-5-2004 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using MS.Internal; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// Text character buffer reference /// public struct CharacterBufferReference : IEquatable{ private CharacterBuffer _charBuffer; private int _offsetToFirstChar; #region Constructor /// /// Construct character buffer reference from character array /// /// character array /// character buffer offset to the first character public CharacterBufferReference( char[] characterArray, int offsetToFirstChar ) : this( new CharArrayCharacterBuffer(characterArray), offsetToFirstChar ) {} ////// Construct character buffer reference from string /// /// character string /// character buffer offset to the first character public CharacterBufferReference( string characterString, int offsetToFirstChar ) : this( new StringCharacterBuffer(characterString), offsetToFirstChar ) {} ////// Construct character buffer reference from unsafe character string /// /// pointer to character string /// character length of unsafe string ////// Critical: This manipulates unsafe pointers and calls into the critical UnsafeStringCharacterBuffer ctor. /// PublicOK: The caller needs unmanaged code permission in order to pass unsafe pointers to us. /// [SecurityCritical] [CLSCompliant(false)] public unsafe CharacterBufferReference( char* unsafeCharacterString, int characterLength ) : this(new UnsafeStringCharacterBuffer(unsafeCharacterString, characterLength), 0) {} ////// Construct character buffer reference from memory buffer /// internal CharacterBufferReference( CharacterBuffer charBuffer, int offsetToFirstChar ) { if (offsetToFirstChar < 0) { throw new ArgumentOutOfRangeException("offsetToFirstChar", SR.Get(SRID.ParameterCannotBeNegative)); } // maximum offset is one less than CharacterBuffer.Count, except that zero is always a valid offset // even in the case of an empty or null character buffer int maxOffset = (charBuffer == null) ? 0 : Math.Max(0, charBuffer.Count - 1); if (offsetToFirstChar > maxOffset) { throw new ArgumentOutOfRangeException("offsetToFirstChar", SR.Get(SRID.ParameterCannotBeGreaterThan, maxOffset)); } _charBuffer = charBuffer; _offsetToFirstChar = offsetToFirstChar; } #endregion ////// Compute hash code /// public override int GetHashCode() { return _charBuffer != null ? _charBuffer.GetHashCode() ^ _offsetToFirstChar : 0; } ////// Test equality with the input object /// /// The object to test. public override bool Equals(object obj) { if (obj is CharacterBufferReference) { return Equals((CharacterBufferReference)obj); } return false; } ////// Test equality with the input CharacterBufferReference /// /// The characterBufferReference value to test public bool Equals(CharacterBufferReference value) { return _charBuffer == value._charBuffer && _offsetToFirstChar == value._offsetToFirstChar; } ////// Compare two CharacterBufferReference for equality /// /// left operand /// right operand ///whether or not two operands are equal public static bool operator == ( CharacterBufferReference left, CharacterBufferReference right ) { return left.Equals(right); } ////// Compare two CharacterBufferReference for inequality /// /// left operand /// right operand ///whether or not two operands are equal public static bool operator != ( CharacterBufferReference left, CharacterBufferReference right ) { return !(left == right); } internal CharacterBuffer CharacterBuffer { get { return _charBuffer; } } internal int OffsetToFirstChar { get { return _offsetToFirstChar; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
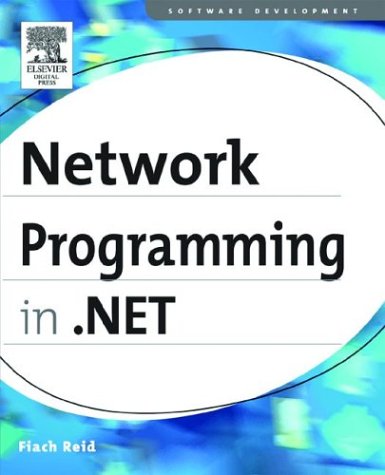
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlNode.cs
- HttpDictionary.cs
- SystemParameters.cs
- DesignerObjectListAdapter.cs
- Perspective.cs
- MergeFailedEvent.cs
- ApplyImportsAction.cs
- SafeProcessHandle.cs
- HttpBufferlessInputStream.cs
- SpoolingTask.cs
- ObjectDataSourceMethodEventArgs.cs
- Rethrow.cs
- webbrowsersite.cs
- CatalogPartChrome.cs
- CodeGroup.cs
- ExpressionVisitor.cs
- Internal.cs
- HttpGetProtocolImporter.cs
- X509SecurityTokenProvider.cs
- SerializeAbsoluteContext.cs
- DataGridViewEditingControlShowingEventArgs.cs
- ArglessEventHandlerProxy.cs
- SerializationEventsCache.cs
- FrameDimension.cs
- ProxyWebPartConnectionCollection.cs
- OutKeywords.cs
- HwndSourceParameters.cs
- CodeDefaultValueExpression.cs
- AccessControlList.cs
- Serializer.cs
- SqlIdentifier.cs
- DataListItemEventArgs.cs
- CssTextWriter.cs
- DbDataAdapter.cs
- StyleBamlRecordReader.cs
- StylusPointPropertyInfoDefaults.cs
- TextCollapsingProperties.cs
- initElementDictionary.cs
- WindowsPrincipal.cs
- EdmItemError.cs
- MethodBody.cs
- HttpStreamMessage.cs
- DynamicValueConverter.cs
- ToolboxSnapDragDropEventArgs.cs
- SimpleTypeResolver.cs
- ColorDialog.cs
- StoreItemCollection.Loader.cs
- ExceptionUtil.cs
- MissingMethodException.cs
- RepeaterItemCollection.cs
- NewItemsContextMenuStrip.cs
- UnsafeNativeMethods.cs
- BamlLocalizationDictionary.cs
- GetPageCompletedEventArgs.cs
- KeyFrames.cs
- TypeSource.cs
- UInt64Storage.cs
- CompressEmulationStream.cs
- XamlSerializationHelper.cs
- WindowsAuthenticationEventArgs.cs
- SystemFonts.cs
- ValidationErrorEventArgs.cs
- RectValueSerializer.cs
- PasswordRecoveryDesigner.cs
- XmlLinkedNode.cs
- DocumentManager.cs
- FontDifferentiator.cs
- XPathMultyIterator.cs
- GeneralTransform3D.cs
- UnsafeNativeMethodsCLR.cs
- StylusButtonCollection.cs
- pingexception.cs
- Activator.cs
- ImageBrush.cs
- coordinator.cs
- MetadataSerializer.cs
- SelectingProviderEventArgs.cs
- WebConfigurationManager.cs
- ThreadSafeList.cs
- Drawing.cs
- StaticContext.cs
- MemberDescriptor.cs
- DataViewManager.cs
- _IPv6Address.cs
- FrameworkTemplate.cs
- Deserializer.cs
- UserControlFileEditor.cs
- Double.cs
- TransactionScope.cs
- XmlElementAttributes.cs
- CodeSnippetTypeMember.cs
- AlignmentXValidation.cs
- AuthenticationModeHelper.cs
- ResourceDescriptionAttribute.cs
- EventRouteFactory.cs
- WhitespaceRule.cs
- ClaimComparer.cs
- UInt16.cs
- OperandQuery.cs
- Empty.cs