Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Drawing.cs / 1 / Drawing.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Drawing is the base Drawing class that defines the standard // interface which Drawing's must implement. // // History: // 2003/07/16 : adsmith - Created it. // 2003/07/28 : adsmith - Renamed it and hooked it up to the unmanaged code. // 2004/11/17 : timothyc - Repurposed Drawing from being a Changeable // implementation that contains render data into the base // class for the enumerable & modifiable Drawing subclasses. // 2005/01/26 : timothyc - Made Drawing abstract & removed legacy Drawing // support, including .Open() & IAddChild. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Resources; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; namespace System.Windows.Media { ////// Base class for the enumerable and modifiable Drawing subclasses. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public abstract partial class Drawing : Animatable, IDrawingContent, DUCE.IResource { #region Constructors ////// Constructor for Drawing /// ////// This constructor is internal to prevent external subclassing. /// internal Drawing() { } #endregion Constructors #region Public Properties ////// Bounds - the axis-aligned bounds of this Drawing. /// ////// Rect - the axis-aligned bounds of this Drawing. /// public Rect Bounds { get { ReadPreamble(); return GetBounds(); } } #endregion Public Properties #region Internal Methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal abstract void UpdateRealizations(RealizationContext realizationContext); ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal abstract void WalkCurrentValue(DrawingContextWalker ctx); #endregion Internal Methods #region IDrawingContent implementation void IDrawingContent.PrecomputeContent() { PrecomputeCore(); } bool IDrawingContent.ContentRequiresRealizationUpdates { get { return this.RequiresRealizationUpdates; } } ////// True if this content can introduce graphness /// bool IDrawingContent.ContentIntroducesGraphness { get { return CanIntroduceGraphness(); } } #endregion IDrawingContent implementation ////// Precompute updates cached data on the Drawings. Currently it is only caching the /// realization flag. /// internal override void Precompute() { PrecomputeCore(); } ////// Derived classes implement the caching of the RequiresRealizationUpdates flag /// in this method. /// ////// internal abstract void PrecomputeCore(); /// /// True if and only if the Drawing requires realization updates. /// internal override bool RequiresRealizationUpdates { get { return m_requiresRealizationUpdates; } set { m_requiresRealizationUpdates = value; } } ////// Returns the bounding box occupied by the content /// ////// Bounding box occupied by the content /// Rect IDrawingContent.GetContentBounds(BoundsDrawingContextWalker ctx) { Debug.Assert(ctx != null); WalkCurrentValue(ctx); return ctx.Bounds; } ////// Forward the current value of the content to the DrawingContextWalker /// methods. /// /// DrawingContextWalker to forward content to. void IDrawingContent.WalkContent(DrawingContextWalker ctx) { ((Drawing)this).WalkCurrentValue(ctx); } ////// Determines whether or not a point exists within the content /// /// Point to hit-test for. ////// 'true' if the point exists within the content, 'false' otherwise /// bool IDrawingContent.HitTestPoint(Point point) { return DrawingServices.HitTestPoint(this, point); } ////// Hit-tests a geometry against this content /// /// PathGeometry to hit-test for. ////// IntersectionDetail describing the result of the hit-test /// IntersectionDetail IDrawingContent.HitTestGeometry(PathGeometry geometry) { return DrawingServices.HitTestGeometry(this, geometry); } ////// Propagates an event handler to Freezables referenced by /// the content. /// /// Event handler to propagate /// 'true' to add the handler, 'false' to remove it void IDrawingContent.PropagateChangedHandler(EventHandler handler, bool adding) { if (!IsFrozen) { if (adding) { ((Drawing)this).Changed += handler; } else { ((Drawing)this).Changed -= handler; } } } ////// Calls UpdatesRealization on GlyphRun's referenced by the content /// /// Context to create realization updates for void IDrawingContent.UpdateRealizations(RealizationContext realizationContext) { ((Drawing)this).UpdateRealizations(realizationContext); } ////// GetBounds to calculate the bounds of this drawing. /// internal Rect GetBounds() { BoundsDrawingContextWalker ctx = new BoundsDrawingContextWalker(); WalkCurrentValue(ctx); return ctx.Bounds; } // private bool m_requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Drawing is the base Drawing class that defines the standard // interface which Drawing's must implement. // // History: // 2003/07/16 : adsmith - Created it. // 2003/07/28 : adsmith - Renamed it and hooked it up to the unmanaged code. // 2004/11/17 : timothyc - Repurposed Drawing from being a Changeable // implementation that contains render data into the base // class for the enumerable & modifiable Drawing subclasses. // 2005/01/26 : timothyc - Made Drawing abstract & removed legacy Drawing // support, including .Open() & IAddChild. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Resources; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; namespace System.Windows.Media { ////// Base class for the enumerable and modifiable Drawing subclasses. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] public abstract partial class Drawing : Animatable, IDrawingContent, DUCE.IResource { #region Constructors ////// Constructor for Drawing /// ////// This constructor is internal to prevent external subclassing. /// internal Drawing() { } #endregion Constructors #region Public Properties ////// Bounds - the axis-aligned bounds of this Drawing. /// ////// Rect - the axis-aligned bounds of this Drawing. /// public Rect Bounds { get { ReadPreamble(); return GetBounds(); } } #endregion Public Properties #region Internal Methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { return true; } ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal abstract void UpdateRealizations(RealizationContext realizationContext); ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal abstract void WalkCurrentValue(DrawingContextWalker ctx); #endregion Internal Methods #region IDrawingContent implementation void IDrawingContent.PrecomputeContent() { PrecomputeCore(); } bool IDrawingContent.ContentRequiresRealizationUpdates { get { return this.RequiresRealizationUpdates; } } ////// True if this content can introduce graphness /// bool IDrawingContent.ContentIntroducesGraphness { get { return CanIntroduceGraphness(); } } #endregion IDrawingContent implementation ////// Precompute updates cached data on the Drawings. Currently it is only caching the /// realization flag. /// internal override void Precompute() { PrecomputeCore(); } ////// Derived classes implement the caching of the RequiresRealizationUpdates flag /// in this method. /// ////// internal abstract void PrecomputeCore(); /// /// True if and only if the Drawing requires realization updates. /// internal override bool RequiresRealizationUpdates { get { return m_requiresRealizationUpdates; } set { m_requiresRealizationUpdates = value; } } ////// Returns the bounding box occupied by the content /// ////// Bounding box occupied by the content /// Rect IDrawingContent.GetContentBounds(BoundsDrawingContextWalker ctx) { Debug.Assert(ctx != null); WalkCurrentValue(ctx); return ctx.Bounds; } ////// Forward the current value of the content to the DrawingContextWalker /// methods. /// /// DrawingContextWalker to forward content to. void IDrawingContent.WalkContent(DrawingContextWalker ctx) { ((Drawing)this).WalkCurrentValue(ctx); } ////// Determines whether or not a point exists within the content /// /// Point to hit-test for. ////// 'true' if the point exists within the content, 'false' otherwise /// bool IDrawingContent.HitTestPoint(Point point) { return DrawingServices.HitTestPoint(this, point); } ////// Hit-tests a geometry against this content /// /// PathGeometry to hit-test for. ////// IntersectionDetail describing the result of the hit-test /// IntersectionDetail IDrawingContent.HitTestGeometry(PathGeometry geometry) { return DrawingServices.HitTestGeometry(this, geometry); } ////// Propagates an event handler to Freezables referenced by /// the content. /// /// Event handler to propagate /// 'true' to add the handler, 'false' to remove it void IDrawingContent.PropagateChangedHandler(EventHandler handler, bool adding) { if (!IsFrozen) { if (adding) { ((Drawing)this).Changed += handler; } else { ((Drawing)this).Changed -= handler; } } } ////// Calls UpdatesRealization on GlyphRun's referenced by the content /// /// Context to create realization updates for void IDrawingContent.UpdateRealizations(RealizationContext realizationContext) { ((Drawing)this).UpdateRealizations(realizationContext); } ////// GetBounds to calculate the bounds of this drawing. /// internal Rect GetBounds() { BoundsDrawingContextWalker ctx = new BoundsDrawingContextWalker(); WalkCurrentValue(ctx); return ctx.Bounds; } // private bool m_requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
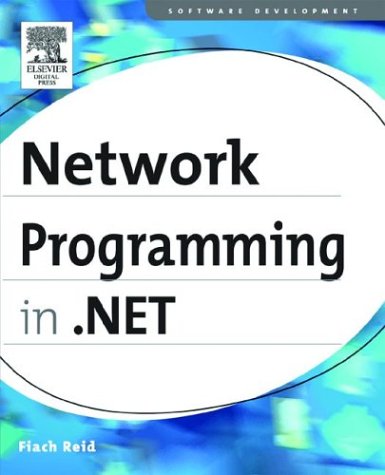
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vector3D.cs
- InvalidPrinterException.cs
- NumericUpDown.cs
- ApplicationFileCodeDomTreeGenerator.cs
- DataGridViewCellCancelEventArgs.cs
- CompilationRelaxations.cs
- DeadCharTextComposition.cs
- TransformerTypeCollection.cs
- PropertyFilter.cs
- SiteMapNodeCollection.cs
- SchemaEntity.cs
- DrawingCollection.cs
- XmlEntity.cs
- DateTimeAutomationPeer.cs
- HtmlInputHidden.cs
- ProfileManager.cs
- WizardSideBarListControlItem.cs
- DockAndAnchorLayout.cs
- ProcessingInstructionAction.cs
- LazyTextWriterCreator.cs
- HttpCapabilitiesEvaluator.cs
- PhysicalOps.cs
- ContextStaticAttribute.cs
- PageSettings.cs
- JsonObjectDataContract.cs
- CollectionChangedEventManager.cs
- PartialTrustHelpers.cs
- ListBindableAttribute.cs
- Int16Converter.cs
- Profiler.cs
- XmlSchemaSimpleTypeUnion.cs
- CapabilitiesState.cs
- WebPartAddingEventArgs.cs
- TcpTransportManager.cs
- PinnedBufferMemoryStream.cs
- ProfilePropertyNameValidator.cs
- NoPersistProperty.cs
- CultureInfo.cs
- ListCommandEventArgs.cs
- DataBindingExpressionBuilder.cs
- HtmlHead.cs
- SafeFindHandle.cs
- TypographyProperties.cs
- Listen.cs
- ToolStripSeparator.cs
- InlineUIContainer.cs
- RuleDefinitions.cs
- ComplusTypeValidator.cs
- CurrencyManager.cs
- HtmlInputButton.cs
- OptimizedTemplateContentHelper.cs
- SqlCacheDependencyDatabaseCollection.cs
- KeyboardDevice.cs
- SerializationException.cs
- FormViewPageEventArgs.cs
- ByteKeyFrameCollection.cs
- EdmItemCollection.cs
- Viewport3DVisual.cs
- ListBoxItem.cs
- URLEditor.cs
- ByteKeyFrameCollection.cs
- MatchingStyle.cs
- NotifyInputEventArgs.cs
- RowsCopiedEventArgs.cs
- RangeContentEnumerator.cs
- MenuEventArgs.cs
- XmlSchemaValidationException.cs
- CoreSwitches.cs
- XmlSchemaAnnotation.cs
- WorkingDirectoryEditor.cs
- OdbcUtils.cs
- RawStylusSystemGestureInputReport.cs
- BinaryParser.cs
- Regex.cs
- GridItemProviderWrapper.cs
- XPathArrayIterator.cs
- BatchParser.cs
- WebConvert.cs
- DrawListViewColumnHeaderEventArgs.cs
- XPathNavigatorKeyComparer.cs
- MergePropertyDescriptor.cs
- BrushConverter.cs
- DesignerCapabilities.cs
- ImageField.cs
- NameTable.cs
- CompilerResults.cs
- TdsEnums.cs
- WindowsScroll.cs
- SqlUserDefinedTypeAttribute.cs
- ToggleButtonAutomationPeer.cs
- ActivityDesignerAccessibleObject.cs
- MessagePartSpecification.cs
- SizeConverter.cs
- ForceCopyBuildProvider.cs
- ProgressBar.cs
- DocumentScope.cs
- CollectionChange.cs
- DATA_BLOB.cs
- DocumentNUp.cs
- SqlTypeSystemProvider.cs