Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ProcessingInstructionAction.cs / 1 / ProcessingInstructionAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ProcessingInstructionAction : ContainerAction { private const int NameEvaluated = 2; private const int NameReady = 3; private Avt nameAvt; // Compile time precalculated AVT private string name; private const char CharX = 'X'; private const char Charx = 'x'; private const char CharM = 'M'; private const char Charm = 'm'; private const char CharL = 'L'; private const char Charl = 'l'; internal ProcessingInstructionAction() {} internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); if(this.nameAvt.IsConstant) { this.name = this.nameAvt.Evaluate(null, null); this.nameAvt = null; if (! IsProcessingInstructionName(this.name)) { // For Now: set to null to ignore action late; this.name = null; } } if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.nameAvt == null) { frame.StoredOutput = this.name; if(this.name == null) { // name was static but was bad; frame.Finished(); break; } } else { frame.StoredOutput = this.nameAvt.Evaluate(processor, frame); if (! IsProcessingInstructionName(frame.StoredOutput)) { frame.Finished(); break; } } goto case NameReady; case NameReady: Debug.Assert(frame.StoredOutput != null); if (processor.BeginEvent(XPathNodeType.ProcessingInstruction, string.Empty, frame.StoredOutput, string.Empty, false) == false) { // Come back later frame.State = NameReady; break; } processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run case ProcessingChildren: if (processor.EndEvent(XPathNodeType.ProcessingInstruction) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); frame.Finished(); break; } } internal static bool IsProcessingInstructionName(string name) { if (name == null) { return false; } int nameLength = name.Length; int position = 0; XmlCharType xmlCharType = XmlCharType.Instance; while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position >= nameLength) { return false; } if (position < nameLength && ! xmlCharType.IsStartNCNameChar(name[position])) { return false; } while (position < nameLength && xmlCharType.IsNCNameChar(name[position])) { position ++; } while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position < nameLength) { return false; } if (nameLength == 3 && (name[0] == CharX || name[0] == Charx) && (name[1] == CharM || name[1] == Charm) && (name[2] == CharL || name[2] == Charl) ) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ProcessingInstructionAction : ContainerAction { private const int NameEvaluated = 2; private const int NameReady = 3; private Avt nameAvt; // Compile time precalculated AVT private string name; private const char CharX = 'X'; private const char Charx = 'x'; private const char CharM = 'M'; private const char Charm = 'm'; private const char CharL = 'L'; private const char Charl = 'l'; internal ProcessingInstructionAction() {} internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); if(this.nameAvt.IsConstant) { this.name = this.nameAvt.Evaluate(null, null); this.nameAvt = null; if (! IsProcessingInstructionName(this.name)) { // For Now: set to null to ignore action late; this.name = null; } } if (compiler.Recurse()) { CompileTemplate(compiler); compiler.ToParent(); } } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.nameAvt == null) { frame.StoredOutput = this.name; if(this.name == null) { // name was static but was bad; frame.Finished(); break; } } else { frame.StoredOutput = this.nameAvt.Evaluate(processor, frame); if (! IsProcessingInstructionName(frame.StoredOutput)) { frame.Finished(); break; } } goto case NameReady; case NameReady: Debug.Assert(frame.StoredOutput != null); if (processor.BeginEvent(XPathNodeType.ProcessingInstruction, string.Empty, frame.StoredOutput, string.Empty, false) == false) { // Come back later frame.State = NameReady; break; } processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run case ProcessingChildren: if (processor.EndEvent(XPathNodeType.ProcessingInstruction) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); frame.Finished(); break; } } internal static bool IsProcessingInstructionName(string name) { if (name == null) { return false; } int nameLength = name.Length; int position = 0; XmlCharType xmlCharType = XmlCharType.Instance; while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position >= nameLength) { return false; } if (position < nameLength && ! xmlCharType.IsStartNCNameChar(name[position])) { return false; } while (position < nameLength && xmlCharType.IsNCNameChar(name[position])) { position ++; } while (position < nameLength && xmlCharType.IsWhiteSpace(name[position])) { position ++; } if (position < nameLength) { return false; } if (nameLength == 3 && (name[0] == CharX || name[0] == Charx) && (name[1] == CharM || name[1] == Charm) && (name[2] == CharL || name[2] == Charl) ) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
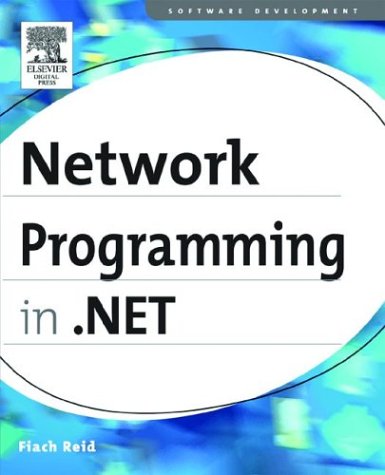
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpCapabilitiesEvaluator.cs
- ImmComposition.cs
- LogAppendAsyncResult.cs
- CommandField.cs
- ArgumentDesigner.xaml.cs
- WebPageTraceListener.cs
- DecimalConverter.cs
- cookiecontainer.cs
- PtsHost.cs
- TextRunProperties.cs
- SecurityKeyIdentifier.cs
- Perspective.cs
- ObjectSet.cs
- ProfilePropertySettings.cs
- Currency.cs
- LambdaCompiler.Logical.cs
- ParameterCollectionEditor.cs
- MonikerSyntaxException.cs
- XmlNodeWriter.cs
- mda.cs
- StoragePropertyMapping.cs
- CorePropertiesFilter.cs
- ContentPosition.cs
- LowerCaseStringConverter.cs
- CultureMapper.cs
- StaticFileHandler.cs
- DiagnosticTrace.cs
- TaskbarItemInfo.cs
- FontFamily.cs
- SecurityVersion.cs
- AnimationLayer.cs
- ReaderWriterLock.cs
- XmlQueryOutput.cs
- DoubleAnimationUsingPath.cs
- StoragePropertyMapping.cs
- SafeNativeMethods.cs
- ListItemCollection.cs
- AssemblyName.cs
- ADConnectionHelper.cs
- DataGridViewCellParsingEventArgs.cs
- ThreadSafeList.cs
- EntityDataSourceView.cs
- AppearanceEditorPart.cs
- TimeSpanValidator.cs
- GeometryHitTestParameters.cs
- DataGridViewRowsRemovedEventArgs.cs
- UrlPropertyAttribute.cs
- UnsafeNativeMethods.cs
- SerialPort.cs
- XmlSchemaGroup.cs
- WebConfigurationManager.cs
- InvalidComObjectException.cs
- UInt32.cs
- OleDbCommand.cs
- RuntimeWrappedException.cs
- PropertyTabChangedEvent.cs
- HashLookup.cs
- ColorDialog.cs
- LiteralControl.cs
- ItemTypeToolStripMenuItem.cs
- DrawingState.cs
- ConstructorExpr.cs
- MultipleViewProviderWrapper.cs
- Translator.cs
- TransformerInfo.cs
- CssClassPropertyAttribute.cs
- StringReader.cs
- TextTreeUndo.cs
- PlaceHolder.cs
- XmlSchemaComplexContent.cs
- AuthenticationManager.cs
- SamlAuthenticationClaimResource.cs
- DataGridPagerStyle.cs
- NoPersistScope.cs
- CurrencyManager.cs
- DataBindingHandlerAttribute.cs
- RichTextBox.cs
- ActivityValidationServices.cs
- ConfigurationProperty.cs
- OutputCacheSettings.cs
- NonParentingControl.cs
- SafeCancelMibChangeNotify.cs
- FacetChecker.cs
- XsltArgumentList.cs
- TriggerBase.cs
- WindowShowOrOpenTracker.cs
- XmlReflectionImporter.cs
- DecimalFormatter.cs
- SqlBuilder.cs
- TimeEnumHelper.cs
- BezierSegment.cs
- MD5.cs
- DesignerActionHeaderItem.cs
- BuildManager.cs
- TreePrinter.cs
- Atom10FeedFormatter.cs
- BoundColumn.cs
- ListComponentEditor.cs
- RadioButtonBaseAdapter.cs
- ReflectionUtil.cs