Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / DataGridPagerStyle.cs / 1305376 / DataGridPagerStyle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Web; using System.Web.UI; ////// public sealed class DataGridPagerStyle : TableItemStyle { ///Specifies the ///pager style for the control. This class cannot be inherited. /// const int PROP_MODE = 0x00080000; ///Represents the Mode property. ////// const int PROP_NEXTPAGETEXT = 0x00100000; ///Represents the Next Page Text property. ////// const int PROP_PREVPAGETEXT = 0x00200000; ///Represents the Previous Page Text property. ////// const int PROP_PAGEBUTTONCOUNT = 0x00400000; ///Represents the Page Button Count property. ////// const int PROP_POSITION = 0x00800000; ///Represents the Position property. ////// const int PROP_VISIBLE = 0x01000000; private DataGrid owner; ///Represents the Visible property. ////// Creates a new instance of DataGridPagerStyle. /// internal DataGridPagerStyle(DataGrid owner) { this.owner = owner; } ////// internal bool IsPagerOnBottom { get { PagerPosition position = Position; return(position == PagerPosition.Bottom) || (position == PagerPosition.TopAndBottom); } } ////// internal bool IsPagerOnTop { get { PagerPosition position = Position; return(position == PagerPosition.Top) || (position == PagerPosition.TopAndBottom); } } ////// Gets or sets the type of Paging UI to use. /// [ WebCategory("Appearance"), DefaultValue(PagerMode.NextPrev), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Mode) ] public PagerMode Mode { get { if (IsSet(PROP_MODE)) { return(PagerMode)(ViewState["Mode"]); } return PagerMode.NextPrev; } set { if (value < PagerMode.NextPrev || value > PagerMode.NumericPages) { throw new ArgumentOutOfRangeException("value"); } ViewState["Mode"] = value; SetBit(PROP_MODE); owner.OnPagerChanged(); } } ////// Gets or sets the text to be used for the Next page /// button. /// [ Localizable(true), WebCategory("Appearance"), DefaultValue(">"), NotifyParentProperty(true), WebSysDescription(SR.PagerSettings_NextPageText) ] public string NextPageText { get { if (IsSet(PROP_NEXTPAGETEXT)) { return(string)(ViewState["NextPageText"]); } return ">"; } set { ViewState["NextPageText"] = value; SetBit(PROP_NEXTPAGETEXT); owner.OnPagerChanged(); } } ////// [ WebCategory("Behavior"), DefaultValue(10), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_PageButtonCount) ] public int PageButtonCount { get { if (IsSet(PROP_PAGEBUTTONCOUNT)) { return(int)(ViewState["PageButtonCount"]); } return 10; } set { if (value < 1) { throw new ArgumentOutOfRangeException("value"); } ViewState["PageButtonCount"] = value; SetBit(PROP_PAGEBUTTONCOUNT); owner.OnPagerChanged(); } } ///Gets or sets the number of pages to show in the /// paging UI when the mode is ////// . /// [ WebCategory("Layout"), DefaultValue(PagerPosition.Bottom), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Position) ] public PagerPosition Position { get { if (IsSet(PROP_POSITION)) { return(PagerPosition)(ViewState["Position"]); } return PagerPosition.Bottom; } set { if (value < PagerPosition.Bottom || value > PagerPosition.TopAndBottom) { throw new ArgumentOutOfRangeException("value"); } ViewState["Position"] = value; SetBit(PROP_POSITION); owner.OnPagerChanged(); } } ///Gets or sets the vertical /// position of the paging UI bar with /// respect to its associated control. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue("<"), NotifyParentProperty(true), WebSysDescription(SR.PagerSettings_PreviousPageText) ] public string PrevPageText { get { if (IsSet(PROP_PREVPAGETEXT)) { return(string)(ViewState["PrevPageText"]); } return "<"; } set { ViewState["PrevPageText"] = value; SetBit(PROP_PREVPAGETEXT); owner.OnPagerChanged(); } } ///Gets or sets the text to be used for the Previous /// page button. ////// [ WebCategory("Appearance"), DefaultValue(true), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Visible) ] public bool Visible { get { if (IsSet(PROP_VISIBLE)) { return(bool)(ViewState["PagerVisible"]); } return true; } set { ViewState["PagerVisible"] = value; SetBit(PROP_VISIBLE); owner.OnPagerChanged(); } } ///Gets or set whether the paging /// UI is to be shown. ////// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is DataGridPagerStyle) { DataGridPagerStyle ps = (DataGridPagerStyle)s; if (ps.IsSet(PROP_MODE)) this.Mode = ps.Mode; if (ps.IsSet(PROP_NEXTPAGETEXT)) this.NextPageText = ps.NextPageText; if (ps.IsSet(PROP_PREVPAGETEXT)) this.PrevPageText = ps.PrevPageText; if (ps.IsSet(PROP_PAGEBUTTONCOUNT)) this.PageButtonCount = ps.PageButtonCount; if (ps.IsSet(PROP_POSITION)) this.Position = ps.Position; if (ps.IsSet(PROP_VISIBLE)) this.Visible = ps.Visible; } } } ///Copies the data grid pager style from the specified ///. /// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, which // is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is DataGridPagerStyle) { DataGridPagerStyle ps = (DataGridPagerStyle)s; if (ps.IsSet(PROP_MODE) && !this.IsSet(PROP_MODE)) this.Mode = ps.Mode; if (ps.IsSet(PROP_NEXTPAGETEXT) && !this.IsSet(PROP_NEXTPAGETEXT)) this.NextPageText = ps.NextPageText; if (ps.IsSet(PROP_PREVPAGETEXT) && !this.IsSet(PROP_PREVPAGETEXT)) this.PrevPageText = ps.PrevPageText; if (ps.IsSet(PROP_PAGEBUTTONCOUNT) && !this.IsSet(PROP_PAGEBUTTONCOUNT)) this.PageButtonCount = ps.PageButtonCount; if (ps.IsSet(PROP_POSITION) && !this.IsSet(PROP_POSITION)) this.Position = ps.Position; if (ps.IsSet(PROP_VISIBLE) && !this.IsSet(PROP_VISIBLE)) this.Visible = ps.Visible; } } } ///Merges the data grid pager style from the specified ///. /// public override void Reset() { if (IsSet(PROP_MODE)) ViewState.Remove("Mode"); if (IsSet(PROP_NEXTPAGETEXT)) ViewState.Remove("NextPageText"); if (IsSet(PROP_PREVPAGETEXT)) ViewState.Remove("PrevPageText"); if (IsSet(PROP_PAGEBUTTONCOUNT)) ViewState.Remove("PageButtonCount"); if (IsSet(PROP_POSITION)) ViewState.Remove("Position"); if (IsSet(PROP_VISIBLE)) ViewState.Remove("PagerVisible"); base.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Restores the data grip pager style to the default values. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Web; using System.Web.UI; ////// public sealed class DataGridPagerStyle : TableItemStyle { ///Specifies the ///pager style for the control. This class cannot be inherited. /// const int PROP_MODE = 0x00080000; ///Represents the Mode property. ////// const int PROP_NEXTPAGETEXT = 0x00100000; ///Represents the Next Page Text property. ////// const int PROP_PREVPAGETEXT = 0x00200000; ///Represents the Previous Page Text property. ////// const int PROP_PAGEBUTTONCOUNT = 0x00400000; ///Represents the Page Button Count property. ////// const int PROP_POSITION = 0x00800000; ///Represents the Position property. ////// const int PROP_VISIBLE = 0x01000000; private DataGrid owner; ///Represents the Visible property. ////// Creates a new instance of DataGridPagerStyle. /// internal DataGridPagerStyle(DataGrid owner) { this.owner = owner; } ////// internal bool IsPagerOnBottom { get { PagerPosition position = Position; return(position == PagerPosition.Bottom) || (position == PagerPosition.TopAndBottom); } } ////// internal bool IsPagerOnTop { get { PagerPosition position = Position; return(position == PagerPosition.Top) || (position == PagerPosition.TopAndBottom); } } ////// Gets or sets the type of Paging UI to use. /// [ WebCategory("Appearance"), DefaultValue(PagerMode.NextPrev), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Mode) ] public PagerMode Mode { get { if (IsSet(PROP_MODE)) { return(PagerMode)(ViewState["Mode"]); } return PagerMode.NextPrev; } set { if (value < PagerMode.NextPrev || value > PagerMode.NumericPages) { throw new ArgumentOutOfRangeException("value"); } ViewState["Mode"] = value; SetBit(PROP_MODE); owner.OnPagerChanged(); } } ////// Gets or sets the text to be used for the Next page /// button. /// [ Localizable(true), WebCategory("Appearance"), DefaultValue(">"), NotifyParentProperty(true), WebSysDescription(SR.PagerSettings_NextPageText) ] public string NextPageText { get { if (IsSet(PROP_NEXTPAGETEXT)) { return(string)(ViewState["NextPageText"]); } return ">"; } set { ViewState["NextPageText"] = value; SetBit(PROP_NEXTPAGETEXT); owner.OnPagerChanged(); } } ////// [ WebCategory("Behavior"), DefaultValue(10), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_PageButtonCount) ] public int PageButtonCount { get { if (IsSet(PROP_PAGEBUTTONCOUNT)) { return(int)(ViewState["PageButtonCount"]); } return 10; } set { if (value < 1) { throw new ArgumentOutOfRangeException("value"); } ViewState["PageButtonCount"] = value; SetBit(PROP_PAGEBUTTONCOUNT); owner.OnPagerChanged(); } } ///Gets or sets the number of pages to show in the /// paging UI when the mode is ////// . /// [ WebCategory("Layout"), DefaultValue(PagerPosition.Bottom), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Position) ] public PagerPosition Position { get { if (IsSet(PROP_POSITION)) { return(PagerPosition)(ViewState["Position"]); } return PagerPosition.Bottom; } set { if (value < PagerPosition.Bottom || value > PagerPosition.TopAndBottom) { throw new ArgumentOutOfRangeException("value"); } ViewState["Position"] = value; SetBit(PROP_POSITION); owner.OnPagerChanged(); } } ///Gets or sets the vertical /// position of the paging UI bar with /// respect to its associated control. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue("<"), NotifyParentProperty(true), WebSysDescription(SR.PagerSettings_PreviousPageText) ] public string PrevPageText { get { if (IsSet(PROP_PREVPAGETEXT)) { return(string)(ViewState["PrevPageText"]); } return "<"; } set { ViewState["PrevPageText"] = value; SetBit(PROP_PREVPAGETEXT); owner.OnPagerChanged(); } } ///Gets or sets the text to be used for the Previous /// page button. ////// [ WebCategory("Appearance"), DefaultValue(true), NotifyParentProperty(true), WebSysDescription(SR.DataGridPagerStyle_Visible) ] public bool Visible { get { if (IsSet(PROP_VISIBLE)) { return(bool)(ViewState["PagerVisible"]); } return true; } set { ViewState["PagerVisible"] = value; SetBit(PROP_VISIBLE); owner.OnPagerChanged(); } } ///Gets or set whether the paging /// UI is to be shown. ////// public override void CopyFrom(Style s) { if (s != null && !s.IsEmpty) { base.CopyFrom(s); if (s is DataGridPagerStyle) { DataGridPagerStyle ps = (DataGridPagerStyle)s; if (ps.IsSet(PROP_MODE)) this.Mode = ps.Mode; if (ps.IsSet(PROP_NEXTPAGETEXT)) this.NextPageText = ps.NextPageText; if (ps.IsSet(PROP_PREVPAGETEXT)) this.PrevPageText = ps.PrevPageText; if (ps.IsSet(PROP_PAGEBUTTONCOUNT)) this.PageButtonCount = ps.PageButtonCount; if (ps.IsSet(PROP_POSITION)) this.Position = ps.Position; if (ps.IsSet(PROP_VISIBLE)) this.Visible = ps.Visible; } } } ///Copies the data grid pager style from the specified ///. /// public override void MergeWith(Style s) { if (s != null && !s.IsEmpty) { if (IsEmpty) { // merge into an empty style is equivalent to a copy, which // is more efficient CopyFrom(s); return; } base.MergeWith(s); if (s is DataGridPagerStyle) { DataGridPagerStyle ps = (DataGridPagerStyle)s; if (ps.IsSet(PROP_MODE) && !this.IsSet(PROP_MODE)) this.Mode = ps.Mode; if (ps.IsSet(PROP_NEXTPAGETEXT) && !this.IsSet(PROP_NEXTPAGETEXT)) this.NextPageText = ps.NextPageText; if (ps.IsSet(PROP_PREVPAGETEXT) && !this.IsSet(PROP_PREVPAGETEXT)) this.PrevPageText = ps.PrevPageText; if (ps.IsSet(PROP_PAGEBUTTONCOUNT) && !this.IsSet(PROP_PAGEBUTTONCOUNT)) this.PageButtonCount = ps.PageButtonCount; if (ps.IsSet(PROP_POSITION) && !this.IsSet(PROP_POSITION)) this.Position = ps.Position; if (ps.IsSet(PROP_VISIBLE) && !this.IsSet(PROP_VISIBLE)) this.Visible = ps.Visible; } } } ///Merges the data grid pager style from the specified ///. /// public override void Reset() { if (IsSet(PROP_MODE)) ViewState.Remove("Mode"); if (IsSet(PROP_NEXTPAGETEXT)) ViewState.Remove("NextPageText"); if (IsSet(PROP_PREVPAGETEXT)) ViewState.Remove("PrevPageText"); if (IsSet(PROP_PAGEBUTTONCOUNT)) ViewState.Remove("PageButtonCount"); if (IsSet(PROP_POSITION)) ViewState.Remove("Position"); if (IsSet(PROP_VISIBLE)) ViewState.Remove("PagerVisible"); base.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Restores the data grip pager style to the default values. ///
Link Menu
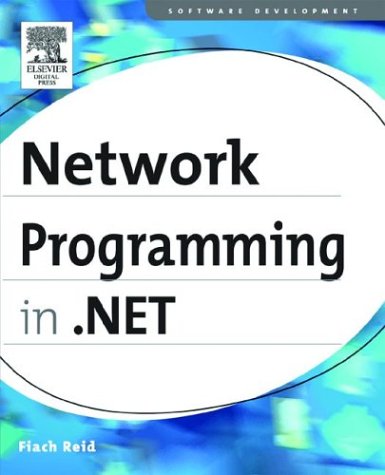
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CssTextWriter.cs
- CalendarDay.cs
- WebPartEditorApplyVerb.cs
- AttributeCollection.cs
- BitmapEffectRenderDataResource.cs
- WebPartCancelEventArgs.cs
- ColorTransformHelper.cs
- FormsAuthenticationTicket.cs
- BackgroundWorker.cs
- DrawingGroup.cs
- Attribute.cs
- ProviderBase.cs
- CompilerGlobalScopeAttribute.cs
- InputBuffer.cs
- DefaultAsyncDataDispatcher.cs
- StringUtil.cs
- EDesignUtil.cs
- TextEditorSpelling.cs
- DataSourceHelper.cs
- PropertyInformation.cs
- SpStreamWrapper.cs
- namescope.cs
- BaseParagraph.cs
- panel.cs
- CompiledQueryCacheEntry.cs
- TTSVoice.cs
- FilteredAttributeCollection.cs
- OleDbParameterCollection.cs
- VirtualDirectoryMappingCollection.cs
- RegexWorker.cs
- COM2PropertyBuilderUITypeEditor.cs
- SerializableAttribute.cs
- DeleteIndexBinder.cs
- ConnectionsZone.cs
- InteropExecutor.cs
- TimelineClockCollection.cs
- Literal.cs
- EpmTargetPathSegment.cs
- SmiRecordBuffer.cs
- MatrixIndependentAnimationStorage.cs
- Sql8ConformanceChecker.cs
- ADMembershipUser.cs
- NullReferenceException.cs
- DefaultTextStore.cs
- HandledMouseEvent.cs
- COAUTHINFO.cs
- X509Chain.cs
- Int64.cs
- StoryFragments.cs
- Visual.cs
- ComboBoxItem.cs
- PageThemeCodeDomTreeGenerator.cs
- WorkflowMessageEventArgs.cs
- PrimaryKeyTypeConverter.cs
- _BasicClient.cs
- IRCollection.cs
- RelationshipNavigation.cs
- DataStreams.cs
- DesignerProperties.cs
- Semaphore.cs
- Mapping.cs
- NameObjectCollectionBase.cs
- UriTemplateLiteralQueryValue.cs
- DataControlLinkButton.cs
- SignatureToken.cs
- ImageBrush.cs
- RightsManagementLicense.cs
- ListManagerBindingsCollection.cs
- InvalidPipelineStoreException.cs
- ExpandCollapsePattern.cs
- CodeEventReferenceExpression.cs
- RenderContext.cs
- FileBasedResourceGroveler.cs
- SqlDependencyUtils.cs
- DefaultPrintController.cs
- ClientConvert.cs
- TemplateField.cs
- XmlChoiceIdentifierAttribute.cs
- Configuration.cs
- EncoderParameters.cs
- TdsParserHelperClasses.cs
- ColumnClickEvent.cs
- MILUtilities.cs
- HashHelper.cs
- AllMembershipCondition.cs
- StrokeNodeOperations.cs
- ConsumerConnectionPoint.cs
- ColumnPropertiesGroup.cs
- PagedDataSource.cs
- HttpInputStream.cs
- DuplexChannelBinder.cs
- JavascriptXmlWriterWrapper.cs
- BindableTemplateBuilder.cs
- PreviousTrackingServiceAttribute.cs
- PatternMatchRules.cs
- FileNotFoundException.cs
- TrustLevel.cs
- Cursor.cs
- ActivityMarkupSerializer.cs
- SHA384Managed.cs