Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SpStreamWrapper.cs / 1 / SpStreamWrapper.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Maps an audio stream the SAPI ISpStreamFormat // // History: // 7/10/2005 [....] //----------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Speech.AudioFormat; using System.Speech.Internal.SapiInterop; using System.Speech.Internal.Synthesis; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. using STATSTG = System.Runtime.InteropServices.ComTypes.STATSTG; namespace System.Speech.Internal.SapiInterop { internal class SpStreamWrapper : IStream, IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SpStreamWrapper (Stream stream) { _stream = stream; _endOfStreamPosition = stream.Length; } ////// /// public void Dispose () { _stream.Dispose (); GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods #region ISpStreamFormat interface implementation public void Read (byte [] pv, int cb, IntPtr pcbRead) { if (_endOfStreamPosition >= 0 && _stream.Position + cb > _endOfStreamPosition) { cb = (int) (_endOfStreamPosition - _stream.Position); } int read = 0; try { read = _stream.Read (pv, 0, cb); } catch (EndOfStreamException) { read = 0; } if (pcbRead != IntPtr.Zero) { Marshal.WriteIntPtr (pcbRead, new IntPtr (read)); } } public void Write (byte [] pv, int cb, IntPtr pcbWritten) { throw new NotSupportedException (); } public void Seek (long offset, int seekOrigin, IntPtr plibNewPosition) { _stream.Seek (offset, (SeekOrigin) seekOrigin); if (plibNewPosition != IntPtr.Zero) { Marshal.WriteIntPtr (plibNewPosition, new IntPtr (_stream.Position)); } } public void SetSize (long libNewSize) { throw new NotSupportedException (); } public void CopyTo (IStream pstm, long cb, IntPtr pcbRead, IntPtr pcbWritten) { throw new NotSupportedException (); } public void Commit (int grfCommitFlags) { _stream.Flush (); } public void Revert () { throw new NotSupportedException (); } public void LockRegion (long libOffset, long cb, int dwLockType) { throw new NotSupportedException (); } public void UnlockRegion (long libOffset, long cb, int dwLockType) { throw new NotSupportedException (); } public void Stat (out STATSTG pstatstg, int grfStatFlag) { pstatstg = new STATSTG (); pstatstg.cbSize = _stream.Length; } public void Clone (out IStream ppstm) { throw new NotSupportedException (); } #endregion #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private Stream _stream; protected long _endOfStreamPosition = -1; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
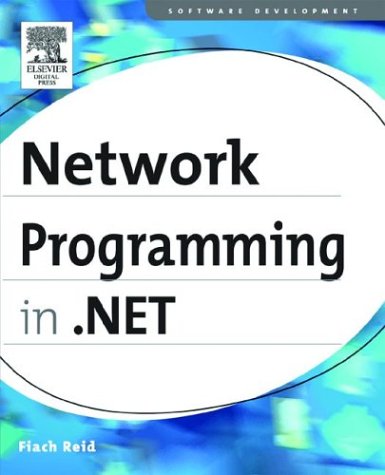
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageCodeDomTreeGenerator.cs
- SocketInformation.cs
- Rect3DValueSerializer.cs
- PolyLineSegment.cs
- DictionarySectionHandler.cs
- NamespaceEmitter.cs
- PersistenceTypeAttribute.cs
- ToolboxItemAttribute.cs
- ToolStripDropDown.cs
- DBConnectionString.cs
- XmlNamespaceDeclarationsAttribute.cs
- DataContractJsonSerializer.cs
- validationstate.cs
- MenuItemStyleCollection.cs
- TypeSystemProvider.cs
- ContainsRowNumberChecker.cs
- DatasetMethodGenerator.cs
- SchemaElementDecl.cs
- TableLayout.cs
- ControlPaint.cs
- SQLInt32Storage.cs
- ProtocolsConfigurationHandler.cs
- ColumnCollection.cs
- EventMappingSettingsCollection.cs
- AlphabetConverter.cs
- DataSourceCacheDurationConverter.cs
- SQLBoolean.cs
- XmlQualifiedName.cs
- TextEditorCharacters.cs
- OutputScope.cs
- UnwrappedTypesXmlSerializerManager.cs
- PreservationFileWriter.cs
- BmpBitmapEncoder.cs
- LayoutTable.cs
- Frame.cs
- AttributeEmitter.cs
- SqlDataSourceView.cs
- EntityViewContainer.cs
- SafeNativeMethods.cs
- MulticastDelegate.cs
- HtmlToClrEventProxy.cs
- MemberPathMap.cs
- WindowsSolidBrush.cs
- xmlsaver.cs
- KnownTypesHelper.cs
- TimeoutException.cs
- SmiRecordBuffer.cs
- EdmFunction.cs
- LineInfo.cs
- SqlFlattener.cs
- SQLGuidStorage.cs
- WebPartConnectionsDisconnectVerb.cs
- ViewStateException.cs
- DefaultParameterValueAttribute.cs
- AttributeInfo.cs
- DataGridViewCellValidatingEventArgs.cs
- EntityTemplateFactory.cs
- ListMarkerLine.cs
- XmlSchemaAnnotated.cs
- CustomTypeDescriptor.cs
- ClientRolePrincipal.cs
- TransformPatternIdentifiers.cs
- ISO2022Encoding.cs
- TextRange.cs
- StreamInfo.cs
- TextSearch.cs
- LiteralControl.cs
- EventMappingSettings.cs
- indexingfiltermarshaler.cs
- XslTransform.cs
- RenderDataDrawingContext.cs
- ErrorFormatter.cs
- SingleAnimation.cs
- RenderDataDrawingContext.cs
- QueueProcessor.cs
- ContentElement.cs
- DelegateSerializationHolder.cs
- ChtmlTextWriter.cs
- Reference.cs
- ZipIOModeEnforcingStream.cs
- ChangeNode.cs
- ScrollPattern.cs
- TreeNodeCollection.cs
- DbConnectionOptions.cs
- StrokeIntersection.cs
- CriticalExceptions.cs
- PageTheme.cs
- ModuleBuilderData.cs
- QueryInterceptorAttribute.cs
- DataTemplate.cs
- ScriptHandlerFactory.cs
- ControlCachePolicy.cs
- DynamicRenderer.cs
- AsymmetricAlgorithm.cs
- XmlDataProvider.cs
- WmlCalendarAdapter.cs
- BinaryNegotiation.cs
- Light.cs
- LightweightCodeGenerator.cs
- ViewgenGatekeeper.cs