Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Collections / Specialized / StringDictionaryWithComparer.cs / 1305376 / StringDictionaryWithComparer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // StringDictionary compares keys by converting them to lowercase first, using the Invariant culture. // This is not the right thing to do for file names, registry keys, environment variable etc. // This internal version of StringDictionary accepts an IEqualityComparer and enables you to // customize the string comparison to be StringComparer.OrdinalIgnoreCase for the above cases. namespace System.Collections.Specialized { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Globalization; [Serializable] internal class StringDictionaryWithComparer : StringDictionary { public StringDictionaryWithComparer() : this(StringComparer.OrdinalIgnoreCase) { } public StringDictionaryWithComparer(IEqualityComparer comparer) { ReplaceHashtable(new Hashtable(comparer)); } public override string this[string key] { get { if( key == null ) { throw new ArgumentNullException("key"); } return (string) contents[key]; } set { if( key == null ) { throw new ArgumentNullException("key"); } contents[key] = value; } } public override void Add(string key, string value) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Add(key, value); } public override bool ContainsKey(string key) { if( key == null ) { throw new ArgumentNullException("key"); } return contents.ContainsKey(key); } public override void Remove(string key) { if( key == null ) { throw new ArgumentNullException("key"); } contents.Remove(key); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
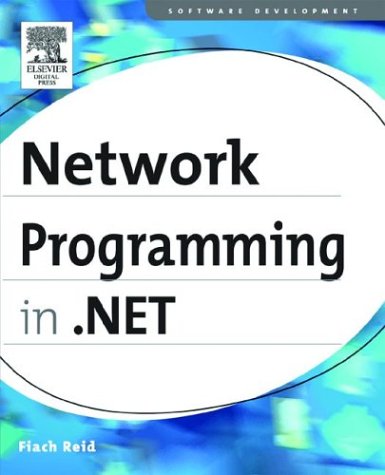
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeNativeMethodsMilCoreApi.cs
- SqlParameterizer.cs
- XmlSchemaSimpleTypeList.cs
- CacheEntry.cs
- ServiceChannelFactory.cs
- SID.cs
- TextElementAutomationPeer.cs
- ClientSettingsSection.cs
- XamlRtfConverter.cs
- ConstraintStruct.cs
- HttpRequestTraceRecord.cs
- TypeExtensionConverter.cs
- WebMessageEncodingBindingElement.cs
- SimpleTypeResolver.cs
- FrameworkElement.cs
- StructuredTypeInfo.cs
- InheritanceUI.cs
- URLMembershipCondition.cs
- TextElementEnumerator.cs
- SynchronizationScope.cs
- HotSpotCollection.cs
- SplineKeyFrames.cs
- OutputScope.cs
- DataBoundLiteralControl.cs
- CompoundFileStreamReference.cs
- DataReceivedEventArgs.cs
- XmlDataImplementation.cs
- Misc.cs
- FocusChangedEventArgs.cs
- LinkLabel.cs
- UnsignedPublishLicense.cs
- Cursor.cs
- ContainerUIElement3D.cs
- SelectionEditor.cs
- Faults.cs
- WebPartEditorOkVerb.cs
- ServerIdentity.cs
- TypePropertyEditor.cs
- SqlExpressionNullability.cs
- InteropBitmapSource.cs
- DomainUpDown.cs
- HtmlTernaryTree.cs
- SqlDataRecord.cs
- BindingElement.cs
- LayoutTableCell.cs
- EqualityComparer.cs
- EDesignUtil.cs
- SchemaElementLookUpTable.cs
- SqlDataSourceSelectingEventArgs.cs
- DbConnectionFactory.cs
- ImageAutomationPeer.cs
- SqlUtil.cs
- MSAAEventDispatcher.cs
- References.cs
- PlatformNotSupportedException.cs
- FamilyTypeface.cs
- Span.cs
- ToolStripLocationCancelEventArgs.cs
- BatchStream.cs
- _DomainName.cs
- ListControlConvertEventArgs.cs
- AxisAngleRotation3D.cs
- ReachDocumentSequenceSerializer.cs
- StringAttributeCollection.cs
- SimpleApplicationHost.cs
- PolicyManager.cs
- AppDomainGrammarProxy.cs
- Operators.cs
- XmlSchemas.cs
- DragEventArgs.cs
- ControlTemplate.cs
- ZoneButton.cs
- SqlNotificationEventArgs.cs
- DurableServiceAttribute.cs
- DataControlLinkButton.cs
- Token.cs
- DesignSurface.cs
- LinkConverter.cs
- DoubleUtil.cs
- DBSqlParserTableCollection.cs
- DesignerAutoFormat.cs
- PerfCounters.cs
- DataContext.cs
- WindowsEditBox.cs
- SafeWaitHandle.cs
- PathHelper.cs
- DataIdProcessor.cs
- XmlWriter.cs
- SoapReflectionImporter.cs
- TextServicesHost.cs
- ActiveDesignSurfaceEvent.cs
- _BufferOffsetSize.cs
- SymbolType.cs
- IsolatedStoragePermission.cs
- DictionarySurrogate.cs
- DateTimeFormat.cs
- ApplicationHost.cs
- DataControlCommands.cs
- FrugalMap.cs
- PlatformNotSupportedException.cs