Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / PersistStreamTypeWrapper.cs / 1 / PersistStreamTypeWrapper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Runtime.Serialization; using System.CodeDom; using System.Reflection; using System.Collections.ObjectModel; using SafeHGlobalHandle = System.IdentityModel.SafeHGlobalHandle; [InterfaceType(ComInterfaceType.InterfaceIsIUnknown), Guid("0000010c-0000-0000-C000-000000000046")] internal interface IPersist { void GetClassID( /* [out] */ out Guid pClassID); }; [InterfaceType(ComInterfaceType.InterfaceIsIUnknown), Guid("00000109-0000-0000-C000-000000000046")] internal interface IPersistStream : IPersist { new void GetClassID(out Guid pClassID); [PreserveSig] int IsDirty( ); void Load([In] IStream pStm); void Save([In] IStream pStm, [In, MarshalAs(UnmanagedType.Bool)] bool fClearDirty); void GetSizeMax(out long pcbSize); }; internal class PersistHelper { internal static byte[] ConvertHGlobalToByteArray(SafeHGlobalHandle hGlobal) { // this has to be Int32, even on 64 bit machines since Marshal.Copy takes a 32 bit integer Int32 sizeOfByteArray = (SafeNativeMethods.GlobalSize(hGlobal)).ToInt32(); if (sizeOfByteArray > 0) { byte[] byteArray = new Byte[sizeOfByteArray]; IntPtr pBuff = SafeNativeMethods.GlobalLock(hGlobal); if (IntPtr.Zero == pBuff) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new OutOfMemoryException()); try { Marshal.Copy(pBuff, byteArray, 0, sizeOfByteArray); } finally { SafeNativeMethods.GlobalUnlock(hGlobal); } return byteArray; } return null; } internal static Byte [] PersistIPersistStreamToByteArray (IPersistStream persistableObject) { IStream stream = SafeNativeMethods.CreateStreamOnHGlobal(SafeHGlobalHandle.InvalidHandle, false); try { persistableObject.Save (stream, true); SafeHGlobalHandle hGlobal = SafeNativeMethods.GetHGlobalFromStream(stream); if (null == hGlobal || IntPtr.Zero == hGlobal.DangerousGetHandle()) { DiagnosticUtility.DebugAssert("HGlobal returned from GetHGlobalFromStream is NULL"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } return ConvertHGlobalToByteArray (hGlobal); } finally { Marshal.ReleaseComObject (stream); } } internal static void LoadIntoObjectFromByteArray (IPersistStream persistableObject, Byte [] byteStream) { SafeHGlobalHandle hGlobal = SafeHGlobalHandle.AllocHGlobal(byteStream.Length); IntPtr pBuff = SafeNativeMethods.GlobalLock (hGlobal); if (IntPtr.Zero == pBuff) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new OutOfMemoryException()); try { Marshal.Copy (byteStream, 0, pBuff, byteStream.Length); IStream stream = SafeNativeMethods.CreateStreamOnHGlobal (hGlobal, false); try { persistableObject.Load (stream); } finally { Marshal.ReleaseComObject (stream); } } finally { SafeNativeMethods.GlobalUnlock (hGlobal); } } internal static object ActivateAndLoadFromByteStream (Guid clsid, byte [] byteStream) { IPersistStream persistableObject = SafeNativeMethods.CoCreateInstance( clsid, null, CLSCTX.INPROC_SERVER, typeof (IPersistStream).GUID) as IPersistStream; if (null != persistableObject) { LoadIntoObjectFromByteArray (persistableObject, byteStream); return persistableObject; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new InvalidOperationException (SR.GetString (SR.CLSIDDoesNotSupportIPersistStream, clsid.ToString("B")))); } } [DataContract] public class PersistStreamTypeWrapper { [DataMember] internal Guid clsid; [DataMember] internal byte [] dataStream ; public PersistStreamTypeWrapper () {} public void SetObject(T obj) { if (Marshal.IsComObject(obj)) { IntPtr punk = Marshal.GetIUnknownForObject(obj); if (IntPtr.Zero == punk) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.UnableToRetrievepUnk))); } try { IntPtr persistStream = IntPtr.Zero; Guid iidPersistStream = typeof(IPersistStream).GUID; int hr = Marshal.QueryInterface(punk, ref iidPersistStream, out persistStream); if (HR.S_OK == hr) { try { if (IntPtr.Zero == persistStream) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.PersistWrapperIsNull))); } IPersistStream persistableObject = (IPersistStream)System.Runtime.Remoting.Services.EnterpriseServicesHelper.WrapIUnknownWithComObject(persistStream); try { this.dataStream = PersistHelper.PersistIPersistStreamToByteArray(persistableObject); this.clsid = typeof(T).GUID; } finally { Marshal.ReleaseComObject(persistableObject); } } finally { Marshal.Release(persistStream); } } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.CLSIDDoesNotSupportIPersistStream, typeof(T).GUID.ToString("B")))); } finally { Marshal.Release(punk); } } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.NotAComObject))); } } public void GetObject (ref T obj) { if (clsid == typeof (T).GUID ) { IntPtr punk = Marshal.GetIUnknownForObject(obj); if (IntPtr.Zero == punk) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.UnableToRetrievepUnk))); } try { IntPtr persistStream = IntPtr.Zero; Guid iidPersistStream = typeof(IPersistStream).GUID; int hr = Marshal.QueryInterface(punk, ref iidPersistStream, out persistStream); if (HR.S_OK == hr) { try { if (IntPtr.Zero == persistStream) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.PersistWrapperIsNull))); } IPersistStream persistableObject = (IPersistStream)System.Runtime.Remoting.Services.EnterpriseServicesHelper.WrapIUnknownWithComObject(persistStream); try { PersistHelper.LoadIntoObjectFromByteArray(persistableObject, dataStream); } finally { Marshal.ReleaseComObject(persistableObject); } } finally { Marshal.Release(persistStream); } } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.CLSIDDoesNotSupportIPersistStream, typeof(T).GUID.ToString("B")))); } finally { Marshal.Release(punk); } } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new InvalidOperationException (SR.GetString (SR.CLSIDOfTypeDoesNotMatch, typeof (T).GUID.ToString(), clsid.ToString("B")))); } } internal class DataContractSurrogateForPersistWrapper : IDataContractSurrogate { Guid [] allowedClasses ; public DataContractSurrogateForPersistWrapper (Guid [] allowedClasses ) { this.allowedClasses = allowedClasses; } bool IsAllowedClass (Guid clsid) { foreach (Guid classID in allowedClasses) if (clsid == classID) return true; return false; } public Type GetDataContractType(Type type) { if (type.IsInterface) return typeof (PersistStreamTypeWrapper); else return type; } public object GetObjectToSerialize(object obj, Type targetType) { if (targetType == typeof (object) || (targetType.IsInterface )) { IPersistStream streamableObject = obj as IPersistStream; if (null != streamableObject) { PersistStreamTypeWrapper objToSerialize = new PersistStreamTypeWrapper (); streamableObject.GetClassID (out objToSerialize.clsid); objToSerialize.dataStream = PersistHelper.PersistIPersistStreamToByteArray (streamableObject); return objToSerialize; } if (targetType.IsInterface ) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new InvalidOperationException (SR.GetString (SR.TargetObjectDoesNotSupportIPersistStream))); return obj; } return obj; } public object GetDeserializedObject(object obj, Type targetType) { if (targetType == typeof (object) || (targetType.IsInterface )) { PersistStreamTypeWrapper streamWrapper = obj as PersistStreamTypeWrapper; if (null != streamWrapper) { if (IsAllowedClass (streamWrapper.clsid)) { return PersistHelper.ActivateAndLoadFromByteStream (streamWrapper.clsid, streamWrapper.dataStream); } else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new InvalidOperationException (SR.GetString (SR.NotAllowedPersistableCLSID, streamWrapper.clsid.ToString("B")))); } if (targetType.IsInterface ) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new InvalidOperationException (SR.GetString (SR.TargetTypeIsAnIntefaceButCorrespoindingTypeIsNotPersistStreamTypeWrapper))); } return obj; } public object GetCustomDataToExport(MemberInfo memberInfo, Type dataContractType) { return null; } public object GetCustomDataToExport(Type clrType, Type dataContractType) { return null; } public void GetKnownCustomDataTypes(Collection customDataTypes) { customDataTypes.Add (typeof (PersistStreamTypeWrapper)); } public Type GetReferencedTypeOnImport(string typeName, string typeNamespace, object customData) { return null; } public CodeTypeDeclaration ProcessImportedType(CodeTypeDeclaration typeDeclaration, CodeCompileUnit compileUnit) { return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
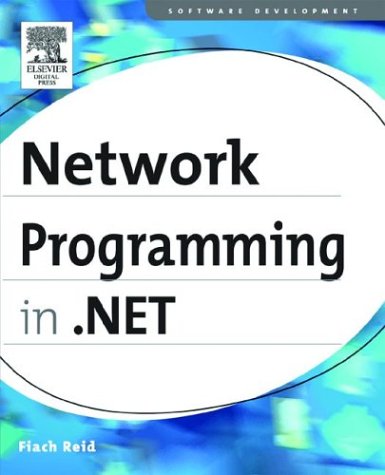
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GB18030Encoding.cs
- TouchFrameEventArgs.cs
- Single.cs
- FixedFlowMap.cs
- ExcCanonicalXml.cs
- RunInstallerAttribute.cs
- CachingHintValidation.cs
- XmlNamespaceDeclarationsAttribute.cs
- TextDecorations.cs
- FunctionParameter.cs
- XPathAncestorQuery.cs
- MetadataSerializer.cs
- KnownBoxes.cs
- MatrixUtil.cs
- SByte.cs
- TextRangeBase.cs
- SafeEventLogReadHandle.cs
- SocketInformation.cs
- EncodingDataItem.cs
- CurrencyManager.cs
- CodeExpressionRuleDeclaration.cs
- ScrollProviderWrapper.cs
- AssemblyCache.cs
- ObjectDisposedException.cs
- RuleSettings.cs
- TextSelectionHighlightLayer.cs
- CheckBoxStandardAdapter.cs
- KeySpline.cs
- GZipDecoder.cs
- HtmlElementCollection.cs
- WeakHashtable.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- SortDescriptionCollection.cs
- StringAnimationUsingKeyFrames.cs
- HtmlInputSubmit.cs
- EnumBuilder.cs
- ContentIterators.cs
- FileDialogCustomPlacesCollection.cs
- DataGrid.cs
- ProfileGroupSettings.cs
- RawUIStateInputReport.cs
- ClockController.cs
- MD5.cs
- DocumentViewerBase.cs
- ProtocolImporter.cs
- FilePresentation.cs
- MatrixUtil.cs
- FixedFindEngine.cs
- HandleCollector.cs
- AppDomainAttributes.cs
- NativeMethods.cs
- TextLine.cs
- MessageAction.cs
- NativeCppClassAttribute.cs
- NetPeerTcpBindingCollectionElement.cs
- TransactionFilter.cs
- TrackingMemoryStream.cs
- MasterPageCodeDomTreeGenerator.cs
- TypedServiceOperationListItem.cs
- WeakReadOnlyCollection.cs
- ProfileGroupSettings.cs
- OperationContext.cs
- XPathNodePointer.cs
- StringInfo.cs
- DesigntimeLicenseContext.cs
- CheckBoxFlatAdapter.cs
- CreationContext.cs
- Compiler.cs
- Scene3D.cs
- OletxCommittableTransaction.cs
- AttachedAnnotationChangedEventArgs.cs
- OleDbDataAdapter.cs
- PrimitiveXmlSerializers.cs
- InternalTypeHelper.cs
- SettingsAttributeDictionary.cs
- SHA1.cs
- GetPageNumberCompletedEventArgs.cs
- ListViewUpdatedEventArgs.cs
- MethodCallConverter.cs
- AnnotationDocumentPaginator.cs
- Menu.cs
- AliasedSlot.cs
- PageAsyncTaskManager.cs
- AnnotationResourceChangedEventArgs.cs
- ToolStripDropDownMenu.cs
- SetterBaseCollection.cs
- PermissionSetEnumerator.cs
- ProfileManager.cs
- CircleHotSpot.cs
- templategroup.cs
- handlecollector.cs
- BuildManagerHost.cs
- DataService.cs
- ConstructorNeedsTagAttribute.cs
- SafeTokenHandle.cs
- Identifier.cs
- CngKey.cs
- Utils.cs
- Command.cs
- WebPartsPersonalizationAuthorization.cs