Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / FilePresentation.cs / 1 / FilePresentation.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// Interacts with user on file based information for XpsViewer. // // // History: // 08/28/2005: [....]: Refactored some of the code from FileManagement.cs // Simplified responsiblity to user interaction //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Forms; using System.Windows.TrustUI; namespace MS.Internal.Documents.Application { ////// Interacts with user on file based information for XpsViewer. /// ////// Responsibility: /// Should be the only class that interacts with the user with or for file /// location information. /// internal static class FilePresentation { #region Internal Methods //------------------------------------------------------------------------- // Internal Methods //------------------------------------------------------------------------- ////// Prompts the user for the save location for the current XpsDocument. /// /// The token for the current document ////// /// Critical: /// - we access and set CriticalFileToken /// - we create a CriticalFileToken for the user specifed location /// - we access the RootBrowserWindow, which is critical /// - we assert to show the file save dialog /// - we make asserts to get the local filename specified by the /// the user; the file name is not stored /// TreatAsSafe: /// - the caller has the fileToken for the existing document /// - the data already exists on the system we are simply updating it /// with information provided by the user /// - the data is data the user already had access to /// - document is an XpsDocument a non executable extension that is /// safe to run in PartialTrust /// [SecurityCritical, SecurityTreatAsSafe] internal static bool ShowSaveFileDialog(ref CriticalFileToken fileToken) { string extension = SR.Get(SRID.FileManagementSaveExt); Trace.SafeWrite(Trace.File, "Showing SafeFileDialog."); bool result = false; SaveFileDialog save = new SaveFileDialog(); if (fileToken != null) { string originalLocation = fileToken.Location.LocalPath; new FileIOPermission(PermissionState.Unrestricted).Assert(); // BlessedAssert try { save.FileName = originalLocation; } finally { FileIOPermission.RevertAssert(); } } save.Filter = SR.Get(SRID.FileManagementSaveFilter); save.DefaultExt = extension; DialogResult dialogResult; // We need FileDialogPermissionAccess.Save and UIPermission.AllWindows // to invoke the ShowDialog method specifying a parent window. // We need to specify a parent window in order to avoid a Winforms // Common Dialog issue where the wrong window is used as the parent // which causes the Save dialog to be incorrectly localized. PermissionSet permissions = new PermissionSet(PermissionState.None); permissions.AddPermission(new UIPermission(UIPermissionWindow.AllWindows)); permissions.AddPermission(new FileDialogPermission(FileDialogPermissionAccess.Save)); // Get the root browser window, if it exists. IWin32Window rbw = null; if (DocumentApplicationDocumentViewer.Instance != null) { rbw = DocumentApplicationDocumentViewer.Instance.RootBrowserWindow; } permissions.Assert(); //BlessedAssert try { if (rbw != null) { dialogResult = save.ShowDialog(rbw); } else { dialogResult = save.ShowDialog(); } } finally { PermissionSet.RevertAssert(); } if (dialogResult == DialogResult.OK) { string filePath; // can not use OpenFile w/ stream as we need the name // to do a safe save as word does FileIOPermission getFileName = new FileIOPermission(PermissionState.None); // path is unknown at this time // get_FileName requires AllAccess; wish it was PathDiscovery imho getFileName.AllFiles = FileIOPermissionAccess.AllAccess; getFileName.Assert(); //BlessedAssert try { filePath = save.FileName; } finally { FileIOPermission.RevertAssert(); } // Add .xps extension if not already present. // This must be done manually since the file save dialog will automatically // add the extension only if the filename doesn't have a known extension. // For instance, homework.1 would become homework.1.xps, but if the user // gets up to homework.386, then the dialog would just pass it through as // is, requiring us to append the extension here. if (!extension.Equals( Path.GetExtension(filePath), StringComparison.OrdinalIgnoreCase)) { filePath = filePath + extension; } Uri file = new Uri(filePath); // as this is the only place we can verify the user authorized this // particular file we construct the token here new FileIOPermission( FileIOPermissionAccess.Read | FileIOPermissionAccess.Write, filePath) .Assert(); // BlessedAssert try { fileToken = new CriticalFileToken(file); } finally { FileIOPermission.RevertAssert(); } result = true; Trace.SafeWrite(Trace.File, "A save location was selected."); } return result; } ////// Notifies the user that the selected destination file is read-only, so /// we cannot save to that location. /// internal static void ShowDestinationIsReadOnly() { System.Windows.MessageBox.Show( SR.Get(SRID.FileManagementDestinationIsReadOnly), SR.Get(SRID.FileManagementTitleError), System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Exclamation ); } ////// Notifies the user of the failure to create temporary files, which /// prevents editing. /// internal static void ShowNoTemporaryFileAccess() { System.Windows.MessageBox.Show( SR.Get(SRID.FileManagementNoTemporaryFileAccess), SR.Get(SRID.FileManagementTitleError), System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Exclamation ); } ////// Notifies the user of the failure to open from a location. /// internal static void ShowNoAccessToSource() { System.Windows.MessageBox.Show( SR.Get(SRID.FileManagementNoAccessToSource), SR.Get(SRID.FileManagementTitleError), System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Exclamation ); } ////// Notifies the user of the failure to save to a location. /// internal static void ShowNoAccessToDestination() { System.Windows.MessageBox.Show( SR.Get(SRID.FileManagementNoAccessToDestination), SR.Get(SRID.FileManagementTitleError), System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Exclamation ); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
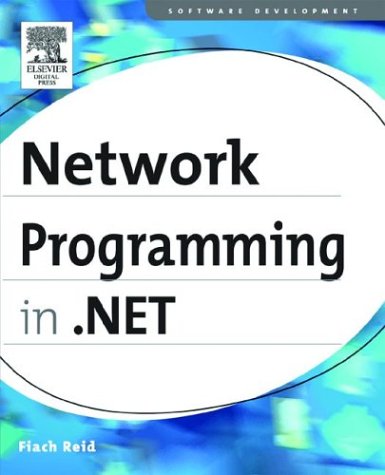
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyControlCollection.cs
- PeerApplication.cs
- BaseValidator.cs
- TextServicesHost.cs
- PropertyToken.cs
- ToolboxSnapDragDropEventArgs.cs
- EntityCommandDefinition.cs
- WorkflowHostingEndpoint.cs
- StrokeSerializer.cs
- COM2ComponentEditor.cs
- InputChannelAcceptor.cs
- DataGridAutoFormatDialog.cs
- CharacterMetricsDictionary.cs
- CannotUnloadAppDomainException.cs
- ByteRangeDownloader.cs
- XmlArrayItemAttribute.cs
- XmlDataDocument.cs
- LineInfo.cs
- ToolStripContainerDesigner.cs
- UnsafeNativeMethods.cs
- UriSectionData.cs
- LexicalChunk.cs
- RegexGroup.cs
- StringSorter.cs
- WebBrowserProgressChangedEventHandler.cs
- PersonalizationStateInfoCollection.cs
- ListSortDescriptionCollection.cs
- GetPageCompletedEventArgs.cs
- ChtmlCommandAdapter.cs
- AddInServer.cs
- SelectionRange.cs
- RuntimeArgumentHandle.cs
- MailHeaderInfo.cs
- httpstaticobjectscollection.cs
- XmlSequenceWriter.cs
- DesignerTransaction.cs
- DependencyProperty.cs
- StrokeDescriptor.cs
- Matrix.cs
- PropertyMappingExceptionEventArgs.cs
- CodeObjectCreateExpression.cs
- SizeChangedEventArgs.cs
- BaseTemplateBuildProvider.cs
- relpropertyhelper.cs
- unsafeIndexingFilterStream.cs
- FaultException.cs
- CategoryAttribute.cs
- SqlPersonalizationProvider.cs
- HostingMessageProperty.cs
- XmlSequenceWriter.cs
- Transform3D.cs
- ConstraintManager.cs
- ArrayTypeMismatchException.cs
- VisualStateGroup.cs
- BindingElementCollection.cs
- MouseBinding.cs
- DbException.cs
- NewArray.cs
- EUCJPEncoding.cs
- SerializationInfo.cs
- ServiceChannel.cs
- ExtensionWindow.cs
- EditingMode.cs
- MetadataUtil.cs
- HiddenField.cs
- ConfigurationFileMap.cs
- ThicknessAnimation.cs
- PropertyChangedEventArgs.cs
- Formatter.cs
- ApplicationSecurityInfo.cs
- FlowSwitch.cs
- RadioButton.cs
- DesignerActionGlyph.cs
- Events.cs
- BaseContextMenu.cs
- storagemappingitemcollection.viewdictionary.cs
- BindingExpressionBase.cs
- XmlSchemaComplexContentExtension.cs
- sqlser.cs
- Translator.cs
- FullTextState.cs
- WindowsSysHeader.cs
- MailAddressCollection.cs
- DataGridViewCell.cs
- DrawingAttributes.cs
- WorkflowShape.cs
- SpeechDetectedEventArgs.cs
- Journaling.cs
- WebControlAdapter.cs
- AsymmetricAlgorithm.cs
- CommandTreeTypeHelper.cs
- ContextQuery.cs
- MetaType.cs
- DbProviderSpecificTypePropertyAttribute.cs
- SubtreeProcessor.cs
- DynamicArgumentDesigner.xaml.cs
- FunctionCommandText.cs
- ConfigXmlComment.cs
- ContainsRowNumberChecker.cs
- _FtpControlStream.cs