Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / TextFormatting / LexicalChunk.cs / 1305600 / LexicalChunk.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: LexicalChunk.cs // // Contents: Lexical chunk represents the character analysis of a piece of raw character string. // // Created: 6-13-2005 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Windows.Media.TextFormatting; using MS.Internal.Generic; namespace MS.Internal.TextFormatting { ////// Lexical chunk represents the character analysis of a piece of raw character string. /// It contains the analyzing result of the string by the lexical services component i.e. /// word-breaker or hyphenator or both. /// /// The number of character indices represented by a chunk may not map exactly to the same number /// of LSCP in the LS character position space. This is because two adjacent character indices in /// a chunk may be mapped by two non-adjacent LSCP in the LS positions. Between two LSRun could /// exist a hidden-run which occupies real LSCP but represents no actual displayable character. /// /// The mapping between the character offsets and the offsets to the correspondent LSCP is retained /// in a span vector that is indexed by the character offsets. /// internal struct LexicalChunk { private TextLexicalBreaks _breaks; // lexical breaks of chunk characters private SpanVector_ichVector; // spans of offsets to the ich-correspondence LSCP internal TextLexicalBreaks Breaks { get { return _breaks; } } /// /// Boolean value indicates whether this chunk contains no valid break info /// internal bool IsNoBreak { get { return _breaks == null; } } ////// Contruct lexical chunk from character analysis /// internal LexicalChunk( TextLexicalBreaks breaks, SpanVectorichVector ) { Invariant.Assert(breaks != null); _breaks = breaks; _ichVector = ichVector; } /// /// Convert the specified LSCP to character index /// internal int LSCPToCharacterIndex(int lsdcp) { if (_ichVector.Count > 0) { int ich = 0; int cchLast = 0; int lsdcpLast = 0; for (int i = 0; i < _ichVector.Count; i++) { Spanspan = _ichVector[i]; int lsdcpCurrent = span.Value; if (lsdcpCurrent > lsdcp) { return ich - cchLast + Math.Min(cchLast, lsdcp - lsdcpLast); } ich += span.Length; cchLast = span.Length; lsdcpLast = lsdcpCurrent; } return ich - cchLast + Math.Min(cchLast, lsdcp - lsdcpLast); } return lsdcp; } /// /// Convert the specified character index to LSCP /// internal int CharacterIndexToLSCP(int ich) { if (_ichVector.Count > 0) { SpanRiderichRider = new SpanRider (_ichVector); ichRider.At(ich); return ichRider.CurrentValue + ich - ichRider.CurrentSpanStart; } return ich; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
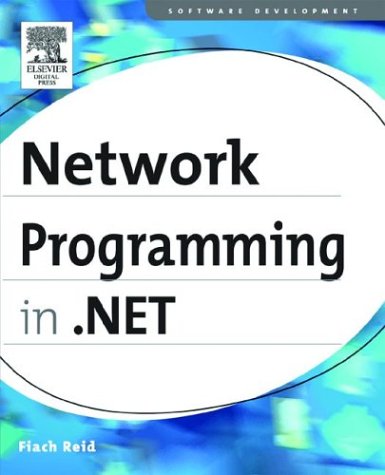
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceNotFoundException.cs
- HtmlImage.cs
- PageClientProxyGenerator.cs
- ResourceExpressionEditorSheet.cs
- PersonalizationStateQuery.cs
- BooleanFacetDescriptionElement.cs
- WebPartTransformerAttribute.cs
- ModuleBuilderData.cs
- CapacityStreamGeometryContext.cs
- UInt64Converter.cs
- PointAnimationClockResource.cs
- EntityProxyFactory.cs
- DetailsView.cs
- XmlValidatingReader.cs
- XPathDocument.cs
- RegionInfo.cs
- DetailsViewUpdateEventArgs.cs
- WebColorConverter.cs
- MultiByteCodec.cs
- PassportPrincipal.cs
- ProfileSettingsCollection.cs
- NullableFloatAverageAggregationOperator.cs
- And.cs
- DateTime.cs
- TreeView.cs
- BooleanKeyFrameCollection.cs
- DataListItemCollection.cs
- X509ChainPolicy.cs
- UniqueTransportManagerRegistration.cs
- InstanceDataCollection.cs
- SeparatorAutomationPeer.cs
- XmlName.cs
- XmlSiteMapProvider.cs
- QilStrConcat.cs
- DataBoundControlParameterTarget.cs
- HttpCacheVary.cs
- SrgsOneOf.cs
- UniqueIdentifierService.cs
- OptimizerPatterns.cs
- DataTemplateKey.cs
- UInt32Converter.cs
- XPathArrayIterator.cs
- GridViewRow.cs
- DocComment.cs
- ListView.cs
- Assembly.cs
- MiniAssembly.cs
- FlowNode.cs
- ContentPlaceHolder.cs
- XmlILConstructAnalyzer.cs
- ProfilePropertyNameValidator.cs
- DPTypeDescriptorContext.cs
- HelpKeywordAttribute.cs
- EventDescriptorCollection.cs
- ToolboxComponentsCreatedEventArgs.cs
- URL.cs
- FormattedText.cs
- GenericWebPart.cs
- BaseParaClient.cs
- StyleXamlParser.cs
- ETagAttribute.cs
- LicenseContext.cs
- ApplicationFileParser.cs
- RefType.cs
- CodeTypeParameter.cs
- NameValueFileSectionHandler.cs
- EntityWithKeyStrategy.cs
- UdpSocketReceiveManager.cs
- PageThemeParser.cs
- LoginView.cs
- Error.cs
- SimpleHandlerBuildProvider.cs
- AbsoluteQuery.cs
- ExceptionHelpers.cs
- TrackingQueryElement.cs
- EntityDataSourceQueryBuilder.cs
- IdentityManager.cs
- XamlSerializationHelper.cs
- SplitContainer.cs
- AttachedAnnotationChangedEventArgs.cs
- BoundColumn.cs
- MemberListBinding.cs
- RangeContentEnumerator.cs
- PaperSize.cs
- XPathNode.cs
- ResourcesBuildProvider.cs
- WindowHideOrCloseTracker.cs
- AsyncStreamReader.cs
- QuadraticBezierSegment.cs
- NavigationWindow.cs
- HotSpotCollection.cs
- WpfPayload.cs
- SweepDirectionValidation.cs
- ResXResourceSet.cs
- LostFocusEventManager.cs
- TemplateControlCodeDomTreeGenerator.cs
- PrintingPermissionAttribute.cs
- StateItem.cs
- Vector3DCollectionConverter.cs
- ExceptionUtil.cs