Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / IlGen / OptimizerPatterns.cs / 1 / OptimizerPatterns.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Xsl.Qil; namespace System.Xml.Xsl.IlGen { internal enum OptimizerPatternName { None, DodReverse, // (Dod $reverse-axis:*) EqualityIndex, // ILGen will build an equality index when this pattern is recognized FilterAttributeKind, // (Filter $iter:(Content *) (IsType $iter Attribute)) FilterContentKind, // (Filter $iter:* (IsType $iter $kind:*)) FilterElements, // (Filter $iter:* (And (IsType $iter Element) (NameOf $iter (LiteralQName * * *)))) IsDocOrderDistinct, // True if the annotated expression always returns nodes in document order, with no duplicates IsPositional, // True if the annotated iterator should track current position during iteration JoinAndDod, // (Dod (Loop $path1:* $path2:*)), where $path2.ContextNode = $path1 MaxPosition, // True if the position range of the annoted iterator or length expression has a maximum SameDepth, // True if the annotated expression always returns nodes at the same depth in the tree Step, // True if the annotated expression returns nodes from one of the simple axis operators, or from a union of Content operators SingleTextRtf, // (RtfCtor (TextCtor *) *) Axis, // (AnyAxis *) MaybeSideEffects, // True if annotated expression might have side effects TailCall, // (Invoke * *) True if invocation can be compiled as using .tailcall DodMerge, // (Dod (Loop * (Invoke * *))), where invoked function returns nodes in document order } internal enum OptimizerPatternArgument { StepNode = 0, // Step, QilNode: The QilNode of the inner step expression (Content, DescendantOrSelf, XPathFollowing, Union, etc.) StepInput = 1, // Step, QilNode: The expression from which navigation begins ElementQName = 2, // FilterElements, QilLiteral: All but elements of this QName are filtered by FilterElements expression KindTestType = 2, // FilterContentKind, XmlType: All but nodes of this XmlType are filtered by FilterContentKind expression IndexedNodes = 0, // EqualityIndex, QilNode: Expression that returns the nodes to be indexed KeyExpression = 1, // EqualityIndex, QilNode: Expression that returns the keys for the index DodStep = 2, // JoinAndDod | DodReverse, QilNode: Last step in a JoinAndDod expression, or only step in DodReverse expression MaxPosition = 2, // MaxPosition, int: Maximum position of the annotated iterator or length expression RtfText = 2, // SingleTextRtf, QilNode: Expression that constructs the text of the simple text Rtf } ////// As the Qil graph is traversed, patterns are identified. Subtrees that match these patterns are /// annotated with this class, which identifies the matching patterns and their arguments. /// internal class OptimizerPatterns : IQilAnnotation { private static readonly int PatternCount = Enum.GetValues(typeof(OptimizerPatternName)).Length; private int patterns; // Set of patterns that the annotated Qil node and its subtree matches private bool isReadOnly; // True if setters are disabled in the case of singleton OptimizerPatterns private object arg0, arg1, arg2; // Arguments to the matching patterns private static OptimizerPatterns ZeroOrOneDefault; private static OptimizerPatterns MaybeManyDefault; private static OptimizerPatterns DodDefault; ////// Get OptimizerPatterns annotation for the specified node. Lazily create if necessary. /// public static OptimizerPatterns Read(QilNode nd) { XmlILAnnotation ann = nd.Annotation as XmlILAnnotation; OptimizerPatterns optPatt = (ann != null) ? ann.Patterns : null; if (optPatt == null) { if (!nd.XmlType.MaybeMany) { // Expressions with ZeroOrOne cardinality should always report IsDocOrderDistinct and NoContainedNodes if (ZeroOrOneDefault == null) { optPatt = new OptimizerPatterns(); optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.AddPattern(OptimizerPatternName.SameDepth); optPatt.isReadOnly = true; ZeroOrOneDefault = optPatt; } else { optPatt = ZeroOrOneDefault; } } else if (nd.XmlType.IsDod) { if (DodDefault == null) { optPatt = new OptimizerPatterns(); optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.isReadOnly = true; DodDefault = optPatt; } else { optPatt = DodDefault; } } else { if (MaybeManyDefault == null) { optPatt = new OptimizerPatterns(); optPatt.isReadOnly = true; MaybeManyDefault = optPatt; } else { optPatt = MaybeManyDefault; } } } return optPatt; } ////// Create and initialize OptimizerPatterns annotation for the specified node. /// public static OptimizerPatterns Write(QilNode nd) { XmlILAnnotation ann = XmlILAnnotation.Write(nd); OptimizerPatterns optPatt = ann.Patterns; if (optPatt == null || optPatt.isReadOnly) { optPatt = new OptimizerPatterns(); ann.Patterns = optPatt; if (!nd.XmlType.MaybeMany) { optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.AddPattern(OptimizerPatternName.SameDepth); } else if (nd.XmlType.IsDod) { optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); } } return optPatt; } ////// Create and initialize OptimizerPatterns annotation for the specified node. /// public static void Inherit(QilNode ndSrc, QilNode ndDst, OptimizerPatternName pattern) { OptimizerPatterns annSrc = OptimizerPatterns.Read(ndSrc); if (annSrc.MatchesPattern(pattern)) { OptimizerPatterns annDst = OptimizerPatterns.Write(ndDst); annDst.AddPattern(pattern); // Inherit pattern arguments switch (pattern) { case OptimizerPatternName.Step: annDst.AddArgument(OptimizerPatternArgument.StepNode, annSrc.GetArgument(OptimizerPatternArgument.StepNode)); annDst.AddArgument(OptimizerPatternArgument.StepInput, annSrc.GetArgument(OptimizerPatternArgument.StepInput)); break; case OptimizerPatternName.FilterElements: annDst.AddArgument(OptimizerPatternArgument.ElementQName, annSrc.GetArgument(OptimizerPatternArgument.ElementQName)); break; case OptimizerPatternName.FilterContentKind: annDst.AddArgument(OptimizerPatternArgument.KindTestType, annSrc.GetArgument(OptimizerPatternArgument.KindTestType)); break; case OptimizerPatternName.EqualityIndex: annDst.AddArgument(OptimizerPatternArgument.IndexedNodes, annSrc.GetArgument(OptimizerPatternArgument.IndexedNodes)); annDst.AddArgument(OptimizerPatternArgument.KeyExpression, annSrc.GetArgument(OptimizerPatternArgument.KeyExpression)); break; case OptimizerPatternName.DodReverse: case OptimizerPatternName.JoinAndDod: annDst.AddArgument(OptimizerPatternArgument.DodStep, annSrc.GetArgument(OptimizerPatternArgument.DodStep)); break; case OptimizerPatternName.MaxPosition: annDst.AddArgument(OptimizerPatternArgument.MaxPosition, annSrc.GetArgument(OptimizerPatternArgument.MaxPosition)); break; case OptimizerPatternName.SingleTextRtf: annDst.AddArgument(OptimizerPatternArgument.RtfText, annSrc.GetArgument(OptimizerPatternArgument.RtfText)); break; } } } ////// Add an argument to one of the matching patterns. /// public void AddArgument(OptimizerPatternArgument argId, object arg) { Debug.Assert(!this.isReadOnly, "This OptimizerPatterns instance is read-only."); switch ((int) argId) { case 0: this.arg0 = arg; break; case 1: this.arg1 = arg; break; case 2: this.arg2 = arg; break; default: Debug.Assert(false, "Cannot handle more than 2 arguments."); break; } } ////// Get an argument of one of the matching patterns. /// public object GetArgument(OptimizerPatternArgument argNum) { object arg = null; switch ((int) argNum) { case 0: arg = this.arg0; break; case 1: arg = this.arg1; break; case 2: arg = this.arg2; break; } Debug.Assert(arg != null, "There is no '" + argNum + "' argument."); return arg; } ////// Add a pattern to the list of patterns that the annotated node matches. /// public void AddPattern(OptimizerPatternName pattern) { Debug.Assert(Enum.IsDefined(typeof(OptimizerPatternName), pattern)); Debug.Assert((int) pattern < 32); Debug.Assert(!this.isReadOnly, "This OptimizerPatterns instance is read-only."); this.patterns |= (1 << (int) pattern); } ////// Return true if the annotated node matches the specified pattern. /// public bool MatchesPattern(OptimizerPatternName pattern) { Debug.Assert(Enum.IsDefined(typeof(OptimizerPatternName), pattern)); return (this.patterns & (1 << (int) pattern)) != 0; } ////// Return name of this annotation. /// public virtual string Name { get { return "Patterns"; } } ////// Return string representation of this annotation. /// public override string ToString() { string s = ""; for (int pattNum = 0; pattNum < PatternCount; pattNum++) { if (MatchesPattern((OptimizerPatternName) pattNum)) { if (s.Length != 0) s += ", "; s += ((OptimizerPatternName) pattNum).ToString(); } } return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Xsl.Qil; namespace System.Xml.Xsl.IlGen { internal enum OptimizerPatternName { None, DodReverse, // (Dod $reverse-axis:*) EqualityIndex, // ILGen will build an equality index when this pattern is recognized FilterAttributeKind, // (Filter $iter:(Content *) (IsType $iter Attribute)) FilterContentKind, // (Filter $iter:* (IsType $iter $kind:*)) FilterElements, // (Filter $iter:* (And (IsType $iter Element) (NameOf $iter (LiteralQName * * *)))) IsDocOrderDistinct, // True if the annotated expression always returns nodes in document order, with no duplicates IsPositional, // True if the annotated iterator should track current position during iteration JoinAndDod, // (Dod (Loop $path1:* $path2:*)), where $path2.ContextNode = $path1 MaxPosition, // True if the position range of the annoted iterator or length expression has a maximum SameDepth, // True if the annotated expression always returns nodes at the same depth in the tree Step, // True if the annotated expression returns nodes from one of the simple axis operators, or from a union of Content operators SingleTextRtf, // (RtfCtor (TextCtor *) *) Axis, // (AnyAxis *) MaybeSideEffects, // True if annotated expression might have side effects TailCall, // (Invoke * *) True if invocation can be compiled as using .tailcall DodMerge, // (Dod (Loop * (Invoke * *))), where invoked function returns nodes in document order } internal enum OptimizerPatternArgument { StepNode = 0, // Step, QilNode: The QilNode of the inner step expression (Content, DescendantOrSelf, XPathFollowing, Union, etc.) StepInput = 1, // Step, QilNode: The expression from which navigation begins ElementQName = 2, // FilterElements, QilLiteral: All but elements of this QName are filtered by FilterElements expression KindTestType = 2, // FilterContentKind, XmlType: All but nodes of this XmlType are filtered by FilterContentKind expression IndexedNodes = 0, // EqualityIndex, QilNode: Expression that returns the nodes to be indexed KeyExpression = 1, // EqualityIndex, QilNode: Expression that returns the keys for the index DodStep = 2, // JoinAndDod | DodReverse, QilNode: Last step in a JoinAndDod expression, or only step in DodReverse expression MaxPosition = 2, // MaxPosition, int: Maximum position of the annotated iterator or length expression RtfText = 2, // SingleTextRtf, QilNode: Expression that constructs the text of the simple text Rtf } ////// As the Qil graph is traversed, patterns are identified. Subtrees that match these patterns are /// annotated with this class, which identifies the matching patterns and their arguments. /// internal class OptimizerPatterns : IQilAnnotation { private static readonly int PatternCount = Enum.GetValues(typeof(OptimizerPatternName)).Length; private int patterns; // Set of patterns that the annotated Qil node and its subtree matches private bool isReadOnly; // True if setters are disabled in the case of singleton OptimizerPatterns private object arg0, arg1, arg2; // Arguments to the matching patterns private static OptimizerPatterns ZeroOrOneDefault; private static OptimizerPatterns MaybeManyDefault; private static OptimizerPatterns DodDefault; ////// Get OptimizerPatterns annotation for the specified node. Lazily create if necessary. /// public static OptimizerPatterns Read(QilNode nd) { XmlILAnnotation ann = nd.Annotation as XmlILAnnotation; OptimizerPatterns optPatt = (ann != null) ? ann.Patterns : null; if (optPatt == null) { if (!nd.XmlType.MaybeMany) { // Expressions with ZeroOrOne cardinality should always report IsDocOrderDistinct and NoContainedNodes if (ZeroOrOneDefault == null) { optPatt = new OptimizerPatterns(); optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.AddPattern(OptimizerPatternName.SameDepth); optPatt.isReadOnly = true; ZeroOrOneDefault = optPatt; } else { optPatt = ZeroOrOneDefault; } } else if (nd.XmlType.IsDod) { if (DodDefault == null) { optPatt = new OptimizerPatterns(); optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.isReadOnly = true; DodDefault = optPatt; } else { optPatt = DodDefault; } } else { if (MaybeManyDefault == null) { optPatt = new OptimizerPatterns(); optPatt.isReadOnly = true; MaybeManyDefault = optPatt; } else { optPatt = MaybeManyDefault; } } } return optPatt; } ////// Create and initialize OptimizerPatterns annotation for the specified node. /// public static OptimizerPatterns Write(QilNode nd) { XmlILAnnotation ann = XmlILAnnotation.Write(nd); OptimizerPatterns optPatt = ann.Patterns; if (optPatt == null || optPatt.isReadOnly) { optPatt = new OptimizerPatterns(); ann.Patterns = optPatt; if (!nd.XmlType.MaybeMany) { optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); optPatt.AddPattern(OptimizerPatternName.SameDepth); } else if (nd.XmlType.IsDod) { optPatt.AddPattern(OptimizerPatternName.IsDocOrderDistinct); } } return optPatt; } ////// Create and initialize OptimizerPatterns annotation for the specified node. /// public static void Inherit(QilNode ndSrc, QilNode ndDst, OptimizerPatternName pattern) { OptimizerPatterns annSrc = OptimizerPatterns.Read(ndSrc); if (annSrc.MatchesPattern(pattern)) { OptimizerPatterns annDst = OptimizerPatterns.Write(ndDst); annDst.AddPattern(pattern); // Inherit pattern arguments switch (pattern) { case OptimizerPatternName.Step: annDst.AddArgument(OptimizerPatternArgument.StepNode, annSrc.GetArgument(OptimizerPatternArgument.StepNode)); annDst.AddArgument(OptimizerPatternArgument.StepInput, annSrc.GetArgument(OptimizerPatternArgument.StepInput)); break; case OptimizerPatternName.FilterElements: annDst.AddArgument(OptimizerPatternArgument.ElementQName, annSrc.GetArgument(OptimizerPatternArgument.ElementQName)); break; case OptimizerPatternName.FilterContentKind: annDst.AddArgument(OptimizerPatternArgument.KindTestType, annSrc.GetArgument(OptimizerPatternArgument.KindTestType)); break; case OptimizerPatternName.EqualityIndex: annDst.AddArgument(OptimizerPatternArgument.IndexedNodes, annSrc.GetArgument(OptimizerPatternArgument.IndexedNodes)); annDst.AddArgument(OptimizerPatternArgument.KeyExpression, annSrc.GetArgument(OptimizerPatternArgument.KeyExpression)); break; case OptimizerPatternName.DodReverse: case OptimizerPatternName.JoinAndDod: annDst.AddArgument(OptimizerPatternArgument.DodStep, annSrc.GetArgument(OptimizerPatternArgument.DodStep)); break; case OptimizerPatternName.MaxPosition: annDst.AddArgument(OptimizerPatternArgument.MaxPosition, annSrc.GetArgument(OptimizerPatternArgument.MaxPosition)); break; case OptimizerPatternName.SingleTextRtf: annDst.AddArgument(OptimizerPatternArgument.RtfText, annSrc.GetArgument(OptimizerPatternArgument.RtfText)); break; } } } ////// Add an argument to one of the matching patterns. /// public void AddArgument(OptimizerPatternArgument argId, object arg) { Debug.Assert(!this.isReadOnly, "This OptimizerPatterns instance is read-only."); switch ((int) argId) { case 0: this.arg0 = arg; break; case 1: this.arg1 = arg; break; case 2: this.arg2 = arg; break; default: Debug.Assert(false, "Cannot handle more than 2 arguments."); break; } } ////// Get an argument of one of the matching patterns. /// public object GetArgument(OptimizerPatternArgument argNum) { object arg = null; switch ((int) argNum) { case 0: arg = this.arg0; break; case 1: arg = this.arg1; break; case 2: arg = this.arg2; break; } Debug.Assert(arg != null, "There is no '" + argNum + "' argument."); return arg; } ////// Add a pattern to the list of patterns that the annotated node matches. /// public void AddPattern(OptimizerPatternName pattern) { Debug.Assert(Enum.IsDefined(typeof(OptimizerPatternName), pattern)); Debug.Assert((int) pattern < 32); Debug.Assert(!this.isReadOnly, "This OptimizerPatterns instance is read-only."); this.patterns |= (1 << (int) pattern); } ////// Return true if the annotated node matches the specified pattern. /// public bool MatchesPattern(OptimizerPatternName pattern) { Debug.Assert(Enum.IsDefined(typeof(OptimizerPatternName), pattern)); return (this.patterns & (1 << (int) pattern)) != 0; } ////// Return name of this annotation. /// public virtual string Name { get { return "Patterns"; } } ////// Return string representation of this annotation. /// public override string ToString() { string s = ""; for (int pattNum = 0; pattNum < PatternCount; pattNum++) { if (MatchesPattern((OptimizerPatternName) pattNum)) { if (s.Length != 0) s += ", "; s += ((OptimizerPatternName) pattNum).ToString(); } } return s; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
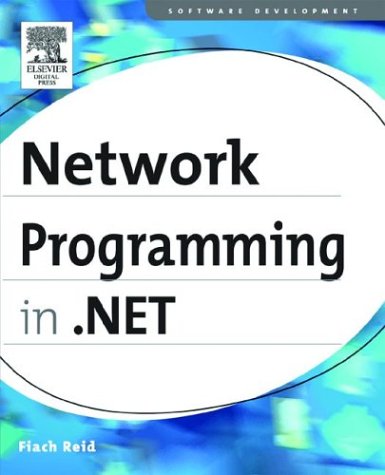
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceQueryProvider.cs
- SponsorHelper.cs
- IpcClientChannel.cs
- ExternalDataExchangeService.cs
- PackageFilter.cs
- ConnectivityStatus.cs
- WorkflowOperationInvoker.cs
- TemplateControl.cs
- Parser.cs
- UrlAuthorizationModule.cs
- XmlDigitalSignatureProcessor.cs
- DES.cs
- SpecularMaterial.cs
- TableLayoutRowStyleCollection.cs
- DataGridViewHeaderCell.cs
- XmlCharacterData.cs
- ServiceInfo.cs
- BitmapFrameEncode.cs
- SimpleRecyclingCache.cs
- CheckBoxFlatAdapter.cs
- GeometryCollection.cs
- DispatcherTimer.cs
- GenericEnumerator.cs
- dbenumerator.cs
- UidManager.cs
- RouteTable.cs
- InvalidPipelineStoreException.cs
- DbProviderSpecificTypePropertyAttribute.cs
- RuntimeEnvironment.cs
- XmlSerializerVersionAttribute.cs
- dsa.cs
- MethodImplAttribute.cs
- OutputCacheProfileCollection.cs
- TextPattern.cs
- DiscriminatorMap.cs
- WebPartMenu.cs
- StylusPoint.cs
- PeerApplicationLaunchInfo.cs
- TransformerConfigurationWizardBase.cs
- SwitchLevelAttribute.cs
- EntityContainer.cs
- CodeCatchClauseCollection.cs
- GridViewRowPresenter.cs
- DataSvcMapFile.cs
- DataServiceSaveChangesEventArgs.cs
- ReadOnlyDictionary.cs
- EventNotify.cs
- BindValidationContext.cs
- XmlDeclaration.cs
- EventSchemaTraceListener.cs
- PeerApplicationLaunchInfo.cs
- VoiceSynthesis.cs
- StrongTypingException.cs
- CqlWriter.cs
- MbpInfo.cs
- _SecureChannel.cs
- InvokeHandlers.cs
- StructuralCache.cs
- ConstraintEnumerator.cs
- MsmqAppDomainProtocolHandler.cs
- EmptyQuery.cs
- PeerInvitationResponse.cs
- AsyncStreamReader.cs
- ListViewGroupCollectionEditor.cs
- InternalsVisibleToAttribute.cs
- Stack.cs
- PrintDialogDesigner.cs
- SqlFunctions.cs
- RelationshipNavigation.cs
- FormsAuthenticationUserCollection.cs
- ArrangedElementCollection.cs
- OdbcUtils.cs
- AppearanceEditorPart.cs
- DataGridClipboardHelper.cs
- MatcherBuilder.cs
- DataException.cs
- Button.cs
- WorkflowNamespace.cs
- ValueCollectionParameterReader.cs
- TdsParserStaticMethods.cs
- PropertyMetadata.cs
- wpf-etw.cs
- SaveFileDialog.cs
- XmlTextReaderImpl.cs
- FieldTemplateFactory.cs
- FixedPageAutomationPeer.cs
- TextEditorParagraphs.cs
- WebPartCollection.cs
- Resources.Designer.cs
- SiteMembershipCondition.cs
- ReadOnlyTernaryTree.cs
- RegistryKey.cs
- PerformanceCounterPermission.cs
- DataGridViewRowsRemovedEventArgs.cs
- ResXFileRef.cs
- LabelLiteral.cs
- SchemaElement.cs
- CodeGotoStatement.cs
- ReachSerializationUtils.cs
- EntitySetBase.cs