Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapFrameEncode.cs / 1 / BitmapFrameEncode.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: BitmapFrameEncode.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; namespace System.Windows.Media.Imaging { #region BitmapFrameEncode ////// BitmapFrameEncode abstract class /// internal sealed class BitmapFrameEncode : BitmapFrame { #region Constructors ////// Internal constructor /// ////// SecurityCritical: Accesses unmanaged resources (_wicSource) /// SecurityTreatAsSafe: Inputs are verified and _wicSource and the get is Critical /// [SecurityCritical, SecurityTreatAsSafe] internal BitmapFrameEncode( BitmapSource source, BitmapSource thumbnail, BitmapMetadata metadata, ReadOnlyCollectioncolorContexts ) : base(true) { _bitmapInit.BeginInit(); Debug.Assert(source != null); _source = source; WicSourceHandle = _source.WicSourceHandle; IsSourceCached = _source.IsSourceCached; _isColorCorrected = _source._isColorCorrected; _thumbnail = thumbnail; _readOnlycolorContexts = colorContexts; InternalMetadata = metadata; _syncObject = source.SyncObject; _bitmapInit.EndInit(); FinalizeCreation(); } /// /// Do not allow construction /// This will be called for cloning /// private BitmapFrameEncode() : base(true) { } #endregion #region IUriContext ////// Provides the base uri of the current context. /// public override Uri BaseUri { get { ReadPreamble(); return null; } set { WritePreamble(); } } #endregion #region Public Properties ////// Accesses the Thumbnail property for this BitmapFrameEncode /// public override BitmapSource Thumbnail { get { ReadPreamble(); return _thumbnail; } } ////// Accesses the Metadata property for this BitmapFrameEncode /// public override ImageMetadata Metadata { get { ReadPreamble(); return InternalMetadata; } } ////// Accesses the Decoder property for this BitmapFrameEncode /// public override BitmapDecoder Decoder { get { ReadPreamble(); return null; } } ////// Accesses the ColorContext property for this BitmapFrameEncode /// public override ReadOnlyCollectionColorContexts { get { ReadPreamble(); return _readOnlycolorContexts; } } #endregion #region Public Methods /// /// Create an in-place bitmap metadata writer. /// public override InPlaceBitmapMetadataWriter CreateInPlaceBitmapMetadataWriter() { ReadPreamble(); return null; } #endregion #region Freezable ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BitmapFrameEncode(); } ////// Copy the fields not covered by DPs. This is used by /// CloneCore(), CloneCurrentValueCore(), GetAsFrozenCore() and /// GetCurrentValueAsFrozenCore(). /// private void CopyCommon(BitmapFrameEncode sourceBitmapFrameEncode) { _bitmapInit.BeginInit(); Debug.Assert(sourceBitmapFrameEncode._source != null); _source = sourceBitmapFrameEncode._source; _frameSource = sourceBitmapFrameEncode._frameSource; _thumbnail = sourceBitmapFrameEncode._thumbnail; _readOnlycolorContexts = sourceBitmapFrameEncode.ColorContexts; if (sourceBitmapFrameEncode.InternalMetadata != null) { InternalMetadata = sourceBitmapFrameEncode.InternalMetadata.Clone(); } _bitmapInit.EndInit(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } #endregion #region Internal Properties / Methods ///Freezable.GetCurrentValueAsFrozenCore . ////// Create the unmanaged resources /// ////// Critical - access critical resources /// TreatAsSafe - All inputs verified /// [SecurityCritical, SecurityTreatAsSafe] internal override void FinalizeCreation() { CreationCompleted = true; UpdateCachedSettings(); } ////// Internally stores the bitmap metadata /// ////// Critical - Access critical resource (_metadata) /// TreatAsSafe - site of origin is verified if possible. /// internal override BitmapMetadata InternalMetadata { [SecurityCritical, SecurityTreatAsSafe] get { // Demand Site Of Origin on the URI before usage of metadata. CheckIfSiteOfOrigin(); return _metadata; } [SecurityCritical, SecurityTreatAsSafe] set { // Demand Site Of Origin on the URI before usage of metadata. CheckIfSiteOfOrigin(); _metadata = value; } } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Source for this Frame private BitmapSource _source; #endregion } #endregion // BitmapFrameEncode } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: BitmapFrameEncode.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; namespace System.Windows.Media.Imaging { #region BitmapFrameEncode ////// BitmapFrameEncode abstract class /// internal sealed class BitmapFrameEncode : BitmapFrame { #region Constructors ////// Internal constructor /// ////// SecurityCritical: Accesses unmanaged resources (_wicSource) /// SecurityTreatAsSafe: Inputs are verified and _wicSource and the get is Critical /// [SecurityCritical, SecurityTreatAsSafe] internal BitmapFrameEncode( BitmapSource source, BitmapSource thumbnail, BitmapMetadata metadata, ReadOnlyCollectioncolorContexts ) : base(true) { _bitmapInit.BeginInit(); Debug.Assert(source != null); _source = source; WicSourceHandle = _source.WicSourceHandle; IsSourceCached = _source.IsSourceCached; _isColorCorrected = _source._isColorCorrected; _thumbnail = thumbnail; _readOnlycolorContexts = colorContexts; InternalMetadata = metadata; _syncObject = source.SyncObject; _bitmapInit.EndInit(); FinalizeCreation(); } /// /// Do not allow construction /// This will be called for cloning /// private BitmapFrameEncode() : base(true) { } #endregion #region IUriContext ////// Provides the base uri of the current context. /// public override Uri BaseUri { get { ReadPreamble(); return null; } set { WritePreamble(); } } #endregion #region Public Properties ////// Accesses the Thumbnail property for this BitmapFrameEncode /// public override BitmapSource Thumbnail { get { ReadPreamble(); return _thumbnail; } } ////// Accesses the Metadata property for this BitmapFrameEncode /// public override ImageMetadata Metadata { get { ReadPreamble(); return InternalMetadata; } } ////// Accesses the Decoder property for this BitmapFrameEncode /// public override BitmapDecoder Decoder { get { ReadPreamble(); return null; } } ////// Accesses the ColorContext property for this BitmapFrameEncode /// public override ReadOnlyCollectionColorContexts { get { ReadPreamble(); return _readOnlycolorContexts; } } #endregion #region Public Methods /// /// Create an in-place bitmap metadata writer. /// public override InPlaceBitmapMetadataWriter CreateInPlaceBitmapMetadataWriter() { ReadPreamble(); return null; } #endregion #region Freezable ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new BitmapFrameEncode(); } ////// Copy the fields not covered by DPs. This is used by /// CloneCore(), CloneCurrentValueCore(), GetAsFrozenCore() and /// GetCurrentValueAsFrozenCore(). /// private void CopyCommon(BitmapFrameEncode sourceBitmapFrameEncode) { _bitmapInit.BeginInit(); Debug.Assert(sourceBitmapFrameEncode._source != null); _source = sourceBitmapFrameEncode._source; _frameSource = sourceBitmapFrameEncode._frameSource; _thumbnail = sourceBitmapFrameEncode._thumbnail; _readOnlycolorContexts = sourceBitmapFrameEncode.ColorContexts; if (sourceBitmapFrameEncode.InternalMetadata != null) { InternalMetadata = sourceBitmapFrameEncode.InternalMetadata.Clone(); } _bitmapInit.EndInit(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { BitmapFrameEncode sourceBitmapFrameEncode = (BitmapFrameEncode)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmapFrameEncode); } #endregion #region Internal Properties / Methods ///Freezable.GetCurrentValueAsFrozenCore . ////// Create the unmanaged resources /// ////// Critical - access critical resources /// TreatAsSafe - All inputs verified /// [SecurityCritical, SecurityTreatAsSafe] internal override void FinalizeCreation() { CreationCompleted = true; UpdateCachedSettings(); } ////// Internally stores the bitmap metadata /// ////// Critical - Access critical resource (_metadata) /// TreatAsSafe - site of origin is verified if possible. /// internal override BitmapMetadata InternalMetadata { [SecurityCritical, SecurityTreatAsSafe] get { // Demand Site Of Origin on the URI before usage of metadata. CheckIfSiteOfOrigin(); return _metadata; } [SecurityCritical, SecurityTreatAsSafe] set { // Demand Site Of Origin on the URI before usage of metadata. CheckIfSiteOfOrigin(); _metadata = value; } } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members /// Source for this Frame private BitmapSource _source; #endregion } #endregion // BitmapFrameEncode } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
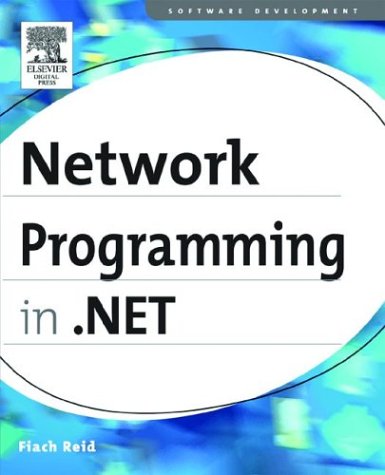
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaImporterExtension.cs
- ClientSponsor.cs
- PasswordTextNavigator.cs
- EventOpcode.cs
- VectorAnimationUsingKeyFrames.cs
- CodeSubDirectoriesCollection.cs
- ApplicationSecurityManager.cs
- CharacterBufferReference.cs
- StructuredTypeEmitter.cs
- Expressions.cs
- WebPartEditorApplyVerb.cs
- WinFormsComponentEditor.cs
- FrameworkElement.cs
- PrivilegeNotHeldException.cs
- GeometryCollection.cs
- RegexCompiler.cs
- BuildManagerHost.cs
- GlobalProxySelection.cs
- ValidatorCompatibilityHelper.cs
- BinaryExpression.cs
- DrawingImage.cs
- ControlBuilder.cs
- BinHexEncoder.cs
- SmiRecordBuffer.cs
- ProxyWebPartManager.cs
- AuthenticationModulesSection.cs
- ViewLoader.cs
- SchemaDeclBase.cs
- PathFigureCollection.cs
- BuildResultCache.cs
- DataControlPagerLinkButton.cs
- ConnectionConsumerAttribute.cs
- InstanceDataCollectionCollection.cs
- BoundsDrawingContextWalker.cs
- PropertyTabAttribute.cs
- GridItem.cs
- UserControlDesigner.cs
- TextLineResult.cs
- ModelItemCollectionImpl.cs
- ICollection.cs
- MediaElement.cs
- namescope.cs
- TabItem.cs
- FixedBufferAttribute.cs
- XmlSchemaAnyAttribute.cs
- FlowPosition.cs
- TickBar.cs
- SessionEndingCancelEventArgs.cs
- ReadWriteObjectLock.cs
- EnumMember.cs
- _ScatterGatherBuffers.cs
- NotifyCollectionChangedEventArgs.cs
- SchemaConstraints.cs
- RemoteWebConfigurationHost.cs
- WebPartTransformerCollection.cs
- TypeUnloadedException.cs
- SafeArrayTypeMismatchException.cs
- UnsafeNativeMethods.cs
- QueryTask.cs
- BindingValueChangedEventArgs.cs
- ErrorRuntimeConfig.cs
- Enlistment.cs
- Utils.cs
- SerializationException.cs
- PriorityChain.cs
- CollectionViewGroupInternal.cs
- RoutedEvent.cs
- CalendarTable.cs
- OutOfProcStateClientManager.cs
- MultiPartWriter.cs
- AuditLogLocation.cs
- XmlIncludeAttribute.cs
- CodeDelegateCreateExpression.cs
- SurrogateEncoder.cs
- SmiXetterAccessMap.cs
- LabelLiteral.cs
- PrintDialogException.cs
- AutoGeneratedField.cs
- RelationshipConverter.cs
- ConfigurationCollectionAttribute.cs
- DocumentStream.cs
- handlecollector.cs
- KeySplineConverter.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- Triplet.cs
- StackBuilderSink.cs
- DecoderBestFitFallback.cs
- EventHandlerService.cs
- Effect.cs
- DebugView.cs
- SignedXml.cs
- RegistryKey.cs
- StorageInfo.cs
- XmlSequenceWriter.cs
- TextEditorContextMenu.cs
- FrameworkContentElement.cs
- Types.cs
- WebBrowserNavigatingEventHandler.cs
- XmlBufferReader.cs
- ButtonBase.cs