Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Monitoring / system / Diagnosticts / InstanceDataCollectionCollection.cs / 1305376 / InstanceDataCollectionCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; using System.Collections; using System.Globalization; ////// The collection returned from the public class InstanceDataCollectionCollection : DictionaryBase { [Obsolete("This constructor has been deprecated. Please use System.Diagnostics.PerformanceCounterCategory.ReadCategory() to get an instance of this collection instead. http://go.microsoft.com/fwlink/?linkid=14202")] public InstanceDataCollectionCollection() : base() {} ///method. /// that contains all the counter and instance data. /// The collection contains an InstanceDataCollection object for each counter. Each InstanceDataCollection /// object contains the performance data for all counters for that instance. In other words the data is /// indexed by counter name and then by instance name. /// /// public InstanceDataCollection this[string counterName] { get { if (counterName == null) throw new ArgumentNullException("counterName"); object objectName = counterName.ToLower(CultureInfo.InvariantCulture); return (InstanceDataCollection) Dictionary[objectName]; } } ///[To be supplied.] ////// public ICollection Keys { get { return Dictionary.Keys; } } ///[To be supplied.] ////// public ICollection Values { get { return Dictionary.Values; } } internal void Add(string counterName, InstanceDataCollection value) { object objectName = counterName.ToLower(CultureInfo.InvariantCulture); Dictionary.Add(objectName, value); } ///[To be supplied.] ////// public bool Contains(string counterName) { if (counterName == null) throw new ArgumentNullException("counterName"); object objectName = counterName.ToLower(CultureInfo.InvariantCulture); return Dictionary.Contains(objectName); } ///[To be supplied.] ////// public void CopyTo(InstanceDataCollection[] counters, int index) { Dictionary.Values.CopyTo((Array)counters, index); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics { using System.Diagnostics; using System; using System.Collections; using System.Globalization; ////// The collection returned from the public class InstanceDataCollectionCollection : DictionaryBase { [Obsolete("This constructor has been deprecated. Please use System.Diagnostics.PerformanceCounterCategory.ReadCategory() to get an instance of this collection instead. http://go.microsoft.com/fwlink/?linkid=14202")] public InstanceDataCollectionCollection() : base() {} ///method. /// that contains all the counter and instance data. /// The collection contains an InstanceDataCollection object for each counter. Each InstanceDataCollection /// object contains the performance data for all counters for that instance. In other words the data is /// indexed by counter name and then by instance name. /// /// public InstanceDataCollection this[string counterName] { get { if (counterName == null) throw new ArgumentNullException("counterName"); object objectName = counterName.ToLower(CultureInfo.InvariantCulture); return (InstanceDataCollection) Dictionary[objectName]; } } ///[To be supplied.] ////// public ICollection Keys { get { return Dictionary.Keys; } } ///[To be supplied.] ////// public ICollection Values { get { return Dictionary.Values; } } internal void Add(string counterName, InstanceDataCollection value) { object objectName = counterName.ToLower(CultureInfo.InvariantCulture); Dictionary.Add(objectName, value); } ///[To be supplied.] ////// public bool Contains(string counterName) { if (counterName == null) throw new ArgumentNullException("counterName"); object objectName = counterName.ToLower(CultureInfo.InvariantCulture); return Dictionary.Contains(objectName); } ///[To be supplied.] ////// public void CopyTo(InstanceDataCollection[] counters, int index) { Dictionary.Values.CopyTo((Array)counters, index); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
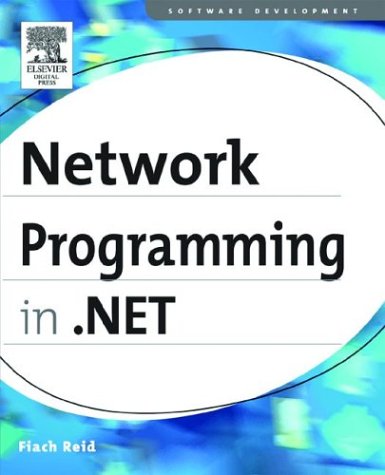
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberInfoSerializationHolder.cs
- SystemColors.cs
- RelatedPropertyManager.cs
- precedingquery.cs
- DecoderNLS.cs
- MediaScriptCommandRoutedEventArgs.cs
- Property.cs
- MdImport.cs
- MdImport.cs
- DBCSCodePageEncoding.cs
- MultiByteCodec.cs
- DataGridViewCellStateChangedEventArgs.cs
- WebPartUtil.cs
- CatalogPartCollection.cs
- VisualStyleInformation.cs
- TreeNodeBindingCollection.cs
- PerformanceCounterLib.cs
- SQLDecimalStorage.cs
- Rotation3DAnimationBase.cs
- PeerName.cs
- EntityProviderFactory.cs
- CategoryAttribute.cs
- HandlerFactoryCache.cs
- QilList.cs
- BrowserCapabilitiesFactory.cs
- XmlILConstructAnalyzer.cs
- EntityDataSourceWrapper.cs
- ControlDesignerState.cs
- InstanceKeyCollisionException.cs
- AndMessageFilterTable.cs
- SourceFilter.cs
- EqualityArray.cs
- ToolStripControlHost.cs
- ReliabilityContractAttribute.cs
- Context.cs
- HandleRef.cs
- MissingMemberException.cs
- FlowLayoutSettings.cs
- AVElementHelper.cs
- XmlReader.cs
- SimpleWorkerRequest.cs
- DependencyPropertyChangedEventArgs.cs
- EFAssociationProvider.cs
- DisplayToken.cs
- PenLineCapValidation.cs
- FontFamily.cs
- DataGridViewCellStyleChangedEventArgs.cs
- DataBindingHandlerAttribute.cs
- _UncName.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- LinkLabel.cs
- FormatException.cs
- NamedPipeConnectionPoolSettings.cs
- StringArrayConverter.cs
- FlowDocumentFormatter.cs
- ClockGroup.cs
- OdbcConnectionStringbuilder.cs
- LOSFormatter.cs
- RolePrincipal.cs
- _CacheStreams.cs
- UserInitiatedNavigationPermission.cs
- FormatterServices.cs
- StylusPlugin.cs
- SortFieldComparer.cs
- SessionPageStateSection.cs
- DataGridViewSelectedCellCollection.cs
- HitTestWithPointDrawingContextWalker.cs
- LinqDataSourceHelper.cs
- AlternationConverter.cs
- CustomDictionarySources.cs
- WorkflowRuntimeServiceElement.cs
- Listbox.cs
- InkPresenter.cs
- EventTrigger.cs
- AccessDataSource.cs
- WindowCollection.cs
- ContainsSearchOperator.cs
- XmlSchemaDatatype.cs
- BitConverter.cs
- ADMembershipUser.cs
- Expression.cs
- SQLSingle.cs
- BrushConverter.cs
- BindingContext.cs
- MailMessage.cs
- TearOffProxy.cs
- MessageDescriptionCollection.cs
- ViewBase.cs
- TablePattern.cs
- MultiView.cs
- BlockCollection.cs
- NullableConverter.cs
- XPathAxisIterator.cs
- DbConnectionHelper.cs
- DeferredSelectedIndexReference.cs
- SoapExtension.cs
- WebPageTraceListener.cs
- FontStyles.cs
- BlockUIContainer.cs
- PrtCap_Public_Simple.cs