Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / BlockUIContainer.cs / 1305600 / BlockUIContainer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: BlockUIContainer - a wrapper for embedded UIElements in text // flow content block collections // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Markup; // ContentProperty namespace System.Windows.Documents { ////// BlockUIContainer - a wrapper for embedded UIElements in text /// flow content block collections /// [ContentProperty("Child")] public class BlockUIContainer : Block { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of BlockUIContainer element. /// ////// The purpose of this element is to be a wrapper for UIElements /// when they are embedded into text flow - as items of /// BlockCollections. /// public BlockUIContainer() : base() { } ////// Initializes an BlockUIContainer specifying its child UIElement /// /// /// UIElement set as a child of this block item /// public BlockUIContainer(UIElement uiElement) : base() { if (uiElement == null) { throw new ArgumentNullException("uiElement"); } this.Child = uiElement; } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Properties ////// The content spanned by this TextElement. /// public UIElement Child { get { return this.ContentStart.GetAdjacentElement(LogicalDirection.Forward) as UIElement; } set { TextContainer textContainer = this.TextContainer; textContainer.BeginChange(); try { TextPointer contentStart = this.ContentStart; UIElement child = Child; if (child != null) { textContainer.DeleteContentInternal(contentStart, this.ContentEnd); ContainerTextElementField.ClearValue(child); } if (value != null) { ContainerTextElementField.SetValue(value, this); contentStart.InsertUIElement(value); } } finally { textContainer.EndChange(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: BlockUIContainer - a wrapper for embedded UIElements in text // flow content block collections // //--------------------------------------------------------------------------- using System.ComponentModel; // DesignerSerializationVisibility using System.Windows.Markup; // ContentProperty namespace System.Windows.Documents { ////// BlockUIContainer - a wrapper for embedded UIElements in text /// flow content block collections /// [ContentProperty("Child")] public class BlockUIContainer : Block { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Initializes a new instance of BlockUIContainer element. /// ////// The purpose of this element is to be a wrapper for UIElements /// when they are embedded into text flow - as items of /// BlockCollections. /// public BlockUIContainer() : base() { } ////// Initializes an BlockUIContainer specifying its child UIElement /// /// /// UIElement set as a child of this block item /// public BlockUIContainer(UIElement uiElement) : base() { if (uiElement == null) { throw new ArgumentNullException("uiElement"); } this.Child = uiElement; } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Properties ////// The content spanned by this TextElement. /// public UIElement Child { get { return this.ContentStart.GetAdjacentElement(LogicalDirection.Forward) as UIElement; } set { TextContainer textContainer = this.TextContainer; textContainer.BeginChange(); try { TextPointer contentStart = this.ContentStart; UIElement child = Child; if (child != null) { textContainer.DeleteContentInternal(contentStart, this.ContentEnd); ContainerTextElementField.ClearValue(child); } if (value != null) { ContainerTextElementField.SetValue(value, this); contentStart.InsertUIElement(value); } } finally { textContainer.EndChange(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
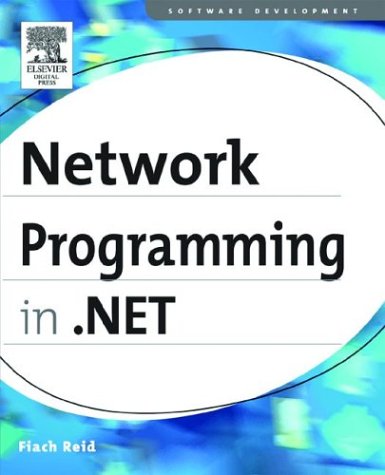
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputFile.cs
- HtmlWindowCollection.cs
- RangeEnumerable.cs
- PrinterUnitConvert.cs
- DataGridViewIntLinkedList.cs
- PublisherIdentityPermission.cs
- HostedTransportConfigurationBase.cs
- SystemEvents.cs
- ConnectionStringsExpressionBuilder.cs
- ScriptResourceInfo.cs
- GraphicsPathIterator.cs
- OleServicesContext.cs
- ModuleBuilderData.cs
- MessagePropertyVariants.cs
- XmlText.cs
- PersonalizableAttribute.cs
- CommonDialog.cs
- ObjectDataSourceStatusEventArgs.cs
- CodeDomLocalizationProvider.cs
- InvokeHandlers.cs
- FrugalMap.cs
- DynamicControlParameter.cs
- HMACSHA256.cs
- HttpDigestClientElement.cs
- AdRotator.cs
- AppSettingsExpressionBuilder.cs
- WindowExtensionMethods.cs
- DoubleLink.cs
- LeftCellWrapper.cs
- TcpAppDomainProtocolHandler.cs
- TableLayoutStyle.cs
- ConfigXmlComment.cs
- NetworkStream.cs
- SmtpDateTime.cs
- CompositeControl.cs
- UnsafeNativeMethods.cs
- ToolBar.cs
- DesignerDataSchemaClass.cs
- PrintPreviewControl.cs
- WebContext.cs
- XmlIgnoreAttribute.cs
- AnnotationService.cs
- InstanceDataCollectionCollection.cs
- Listen.cs
- DefaultObjectMappingItemCollection.cs
- AccessDataSourceView.cs
- securestring.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- GraphicsState.cs
- StyleBamlTreeBuilder.cs
- XmlSchemaDocumentation.cs
- AxHost.cs
- ExpressionStringBuilder.cs
- OleDbPermission.cs
- XmlnsDefinitionAttribute.cs
- VisualCollection.cs
- RequestCachingSection.cs
- RSAPKCS1SignatureFormatter.cs
- FormViewAutoFormat.cs
- AlternateViewCollection.cs
- InputGestureCollection.cs
- DesignerToolStripControlHost.cs
- TraceLevelStore.cs
- PropertyValueChangedEvent.cs
- HwndHostAutomationPeer.cs
- LineSegment.cs
- PartialCachingAttribute.cs
- PrintDocument.cs
- ButtonRenderer.cs
- SHA256Cng.cs
- FloaterParaClient.cs
- ToolStripSettings.cs
- DataSourceCache.cs
- sqlcontext.cs
- LinqToSqlWrapper.cs
- MsmqInputMessage.cs
- TdsEnums.cs
- SmtpFailedRecipientException.cs
- ResourceDisplayNameAttribute.cs
- safesecurityhelperavalon.cs
- TypeTypeConverter.cs
- MarkedHighlightComponent.cs
- AppDomainAttributes.cs
- ScrollChrome.cs
- AppSecurityManager.cs
- SignatureHelper.cs
- SimpleWebHandlerParser.cs
- ListItemCollection.cs
- PackUriHelper.cs
- MappedMetaModel.cs
- hresults.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- HotSpot.cs
- TimeoutStream.cs
- ControlPaint.cs
- WebRequestModuleElementCollection.cs
- QuaternionRotation3D.cs
- ClientRuntimeConfig.cs
- TreeNode.cs
- ConnectionInterfaceCollection.cs